Top 25 Tricky C# Interview Questions (With Explanations and Code Examples)
Preparing for a C# interview can be challenging, especially when the questions go beyond syntax and test your real-world problem-solving and conceptual understanding. Whether you're a beginner brushing up your skills or an experienced developer aiming for senior roles, mastering tricky C# questions is essential. In this blog, I’ve compiled 25 carefully selected C# interview questions that often trip up candidates—not just with clever wording, but with depth. Each question includes a clear explanation and a code example to help you not only understand the concept but also remember it in the long run. 1. Boxing/Unboxing Confusion object obj = 123; long l = (long)(int)obj; // Why the extra cast? Answer: Unboxing must match the exact type. You must unbox to int before casting to long. 2. Object Equality vs Reference Equality object a = 123; object b = 123; Console.WriteLine(a == b); // Output? Answer: False — reference equality for boxed values fails. 3. String Interning string a = "hello"; string b = "he" + "llo"; Console.WriteLine(object.ReferenceEquals(a, b)); // Output? Answer: True — compiler optimizes literals and interns strings. 4. Implicit vs Explicit Casting double d = 10.9; int i = (int)d; Console.WriteLine(i); // Output? Answer: 10 — explicit cast truncates (not rounds). 5. Nullable with Null-Coalescing int? x = null; int y = x ?? 99; Console.WriteLine(y); // Output? Answer: 99 — ?? provides fallback when null. 6. Struct vs Class Copy Behavior struct S { public int X; } S a = new S { X = 1 }; S b = a; b.X = 2; Console.WriteLine(a.X); // Output? Answer: 1 — value type gets copied. 7. Foreach Variable Capture var actions = new List(); for (int i = 0; i Console.Write(i)); } foreach (var act in actions) act(); // Output? Answer: 333 — all actions reference the same i. Fix: Use a local copy of i inside the loop. 8. Overriding vs Hiding class Base { public virtual void Show() => Console.Write("Base"); } class Derived : Base { public new void Show() => Console.Write("Derived"); } Base b = new Derived(); b.Show(); // Output? Answer: Base — method hiding, not overriding. 9. Params Keyword Quirk void Print(params int[] numbers) => Console.WriteLine(numbers.Length); Print(); // Output? Print(null); // Output? Answer: 0 — empty params System.NullReferenceException — null is passed directly. 10. Null with == Operator string a = null; Console.WriteLine(a == null); // Output? Answer: True — safe because == is overloaded for strings. 11. Readonly with Reference Type readonly List list = new List(); list.Add(1); // Is this allowed? Answer: Yes — readonly means reference can't change, not object contents. 12. Static Constructor Execution class A { static A() { Console.Write("Static"); } } A a1 = new A(); A a2 = new A(); // How many times will "Static" print? Answer: Once — static constructor runs only once per type. 13. Catching Exception Types try { throw new NullReferenceException(); } catch (Exception) { Console.Write("Caught"); } Answer: Caught — because NullReferenceException is a subclass of Exception. 14. IEnumerable vs IEnumerator Execution IEnumerable Foo() { Console.Write("Start"); yield return 1; } var result = Foo(); // Output? Answer: Nothing — execution deferred until iteration begins. 15. Integer Overflow int a = int.MaxValue; int b = a + 1; Console.WriteLine(b); // Output? Answer: -2147483648 — overflow with wraparound. 16. LINQ Deferred Execution var numbers = new List { 1, 2, 3 }; var result = numbers.Select(x => x * 2); numbers.Add(4); foreach (var x in result) Console.Write(x); // Output? Answer: 2468 — because of deferred execution. 17. StringBuilder vs String Concatenation string s = ""; for (int i = 0; i
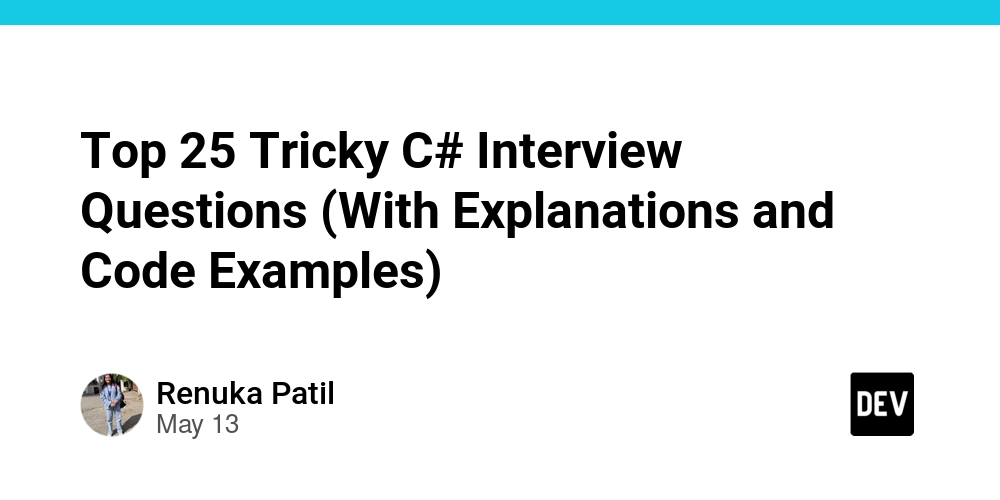
Preparing for a C# interview can be challenging, especially when the questions go beyond syntax and test your real-world problem-solving and conceptual understanding. Whether you're a beginner brushing up your skills or an experienced developer aiming for senior roles, mastering tricky C# questions is essential. In this blog, I’ve compiled 25 carefully selected C# interview questions that often trip up candidates—not just with clever wording, but with depth. Each question includes a clear explanation and a code example to help you not only understand the concept but also remember it in the long run.
1. Boxing/Unboxing Confusion
object obj = 123;
long l = (long)(int)obj; // Why the extra cast?
Answer: Unboxing must match the exact type. You must unbox to int
before casting to long
.
2. Object Equality vs Reference Equality
object a = 123;
object b = 123;
Console.WriteLine(a == b); // Output?
Answer: False
— reference equality for boxed values fails.
3. String Interning
string a = "hello";
string b = "he" + "llo";
Console.WriteLine(object.ReferenceEquals(a, b)); // Output?
Answer: True
— compiler optimizes literals and interns strings.
4. Implicit vs Explicit Casting
double d = 10.9;
int i = (int)d;
Console.WriteLine(i); // Output?
Answer: 10
— explicit cast truncates (not rounds).
5. Nullable with Null-Coalescing
int? x = null;
int y = x ?? 99;
Console.WriteLine(y); // Output?
Answer: 99
— ??
provides fallback when null.
6. Struct vs Class Copy Behavior
struct S { public int X; }
S a = new S { X = 1 };
S b = a;
b.X = 2;
Console.WriteLine(a.X); // Output?
Answer: 1
— value type gets copied.
7. Foreach Variable Capture
var actions = new List<Action>();
for (int i = 0; i < 3; i++)
{
actions.Add(() => Console.Write(i));
}
foreach (var act in actions) act(); // Output?
Answer: 333
— all actions reference the same i
.
Fix: Use a local copy of i
inside the loop.
8. Overriding vs Hiding
class Base { public virtual void Show() => Console.Write("Base"); }
class Derived : Base { public new void Show() => Console.Write("Derived"); }
Base b = new Derived(); b.Show(); // Output?
Answer: Base
— method hiding, not overriding.
9. Params Keyword Quirk
void Print(params int[] numbers) => Console.WriteLine(numbers.Length);
Print(); // Output?
Print(null); // Output?
Answer:
-
0
— empty params -
System.NullReferenceException
—null
is passed directly.
10. Null with ==
Operator
string a = null;
Console.WriteLine(a == null); // Output?
Answer: True
— safe because ==
is overloaded for strings.
11. Readonly with Reference Type
readonly List<int> list = new List<int>();
list.Add(1); // Is this allowed?
Answer: Yes — readonly
means reference can't change, not object contents.
12. Static Constructor Execution
class A { static A() { Console.Write("Static"); } }
A a1 = new A(); A a2 = new A(); // How many times will "Static" print?
Answer: Once — static constructor runs only once per type.
13. Catching Exception Types
try { throw new NullReferenceException(); }
catch (Exception) { Console.Write("Caught"); }
Answer: Caught
— because NullReferenceException
is a subclass of Exception
.
14. IEnumerable vs IEnumerator Execution
IEnumerable<int> Foo()
{
Console.Write("Start");
yield return 1;
}
var result = Foo(); // Output?
Answer: Nothing — execution deferred until iteration begins.
15. Integer Overflow
int a = int.MaxValue;
int b = a + 1;
Console.WriteLine(b); // Output?
Answer: -2147483648
— overflow with wraparound.
16. LINQ Deferred Execution
var numbers = new List<int> { 1, 2, 3 };
var result = numbers.Select(x => x * 2);
numbers.Add(4);
foreach (var x in result) Console.Write(x); // Output?
Answer: 2468
— because of deferred execution.
17. StringBuilder vs String Concatenation
string s = "";
for (int i = 0; i < 1000; i++) s += i.ToString();
Problem: Performance. String is immutable — use StringBuilder
.
18. Static vs Instance Members
class A { public static int Count = 0; }
A.Count++;
Answer: Valid. Static members belong to the class, not instance.
19. Locking on this
lock (this) { } // Is this safe?
Answer: No — it's publicly accessible. Lock on private object instead.
20. Implicit Type with Null
var x = null; // Compile-time error
Answer: var
needs an initializer with a specific type.
21. Boxing & Unboxing Tricky Question
object obj = 10;
Console.WriteLine(obj == 10); // What will this print?
Answer:
True
.
Even though obj
is boxed, the ==
operator for int
is used here, so it unboxes obj
and compares the values.
But now try this:
object a = 10;
object b = 10;
Console.WriteLine(a == b); // What will this print?
Answer:
False
.
Now you're comparing two boxed objects — a
and b
are references to two different boxes in memory. Reference comparison fails.
22. Nullable Types & Null Coalescing
int? x = null;
int y = x ?? 5;
Console.WriteLine(y); // Output?
Answer:
5
— Because x
is null, the ??
operator provides a default.
23. Implicit & Explicit Conversion Tricky
double d = 10.5;
int i = (int)d;
Console.WriteLine(i); // Output?
Answer:
10
— Because (int)
casts truncate, not round.
24. Value vs Reference Types
struct MyStruct
{
public int Value;
}
class MyClass
{
public int Value;
}
Now test this behavior:
MyStruct s1 = new MyStruct { Value = 5 };
MyStruct s2 = s1;
s2.Value = 10;
Console.WriteLine(s1.Value); // Output?
Answer:
5
— because structs are value types, s2
is a copy of s1
.
Now compare:
MyClass c1 = new MyClass { Value = 5 };
MyClass c2 = c1;
c2.Value = 10;
Console.WriteLine(c1.Value); // Output?
Answer:
10
— because classes are reference types, c2
and c1
point to the same object.
25. String Interning and Equality
string a = "hello";
string b = "he" + "llo";
Console.WriteLine(object.ReferenceEquals(a, b)); // Output?
Answer:
True
— because of string interning in C#.
But this will be different:
string a = "hello";
string b = string.Concat("he", "llo");
Console.WriteLine(object.ReferenceEquals(a, b)); // Output?
Answer:
False
— different memory locations, even though content is same.
Cracking C# interviews requires more than just memorizing syntax—it’s about understanding the “why” behind each concept. The questions in this list are designed to help you think like a developer, not just a test taker. By practicing these tricky scenarios and analyzing the provided explanations and code samples, you'll be better prepared to tackle real-world problems and impress your interviewers. Keep coding, stay curious, and continue refining your skills—because that’s what sets great developers apart.
Happy Learning!