NestJS Design Patterns
Analytical Perspectives and Strategic Applications for Scalable Architectures This document serves as an advanced reference for software architects and backend engineers utilizing the NestJS framework to design robust, scalable, and maintainable systems. It systematically explores a broad spectrum of software design patterns, each tailored for application within the context of NestJS. With a focus on theoretical foundations, practical use cases, scalability considerations, and illustrative code examples, this guide bridges conceptual architecture with real-world development practices. 1. Modular Architecture Pattern Application Context: Facilitates the decomposition of a system into discrete, feature-centric modules, each encapsulating domain logic and dependencies. Scalability Assessment: Highly effective for scaling large codebases by promoting bounded contexts and autonomous development cycles. Use Cases: Domain-driven design, feature toggling, microservice-aligned code separation. 2. Dependency Injection (DI) Pattern Application Context: Enables decoupled class instantiation and promotes inversion of control, enhancing flexibility in managing dependencies. Scalability Assessment: Foundational to scalable NestJS systems; facilitates composability and unit testing. Use Cases: Dynamic configuration loading, multi-tenant environments, and mocking in unit tests. 3. Controller-Service-Repository Pattern Application Context: Establishes a hierarchical structure for separating HTTP handling, business logic, and data access. Scalability Assessment: Promotes maintainability, reusability, and abstraction—vital in large-scale applications. Use Cases: RESTful APIs, layered services, structured domain-driven implementations. 4. Repository Pattern Application Context: Abstracts data layer interactions, allowing substitution of storage backends without impacting business logic. Scalability Assessment: Essential for persistence-agnostic design; simplifies testing and data access refactoring. Use Cases: ORM abstraction, mocking data sources, transitioning between databases. 5. Factory Pattern Application Context: Constructs instances dynamically based on runtime criteria or configurations. Scalability Assessment: Highly beneficial in multi-environment setups or pluggable architectures. Use Cases: Logging configuration, dependency instantiation based on context, strategy selectors. 6. Strategy Pattern Application Context: Encapsulates algorithmic behavior and enables dynamic substitution without modifying consuming logic. Scalability Assessment: Supports extensibility and modular business logic injection. Use Cases: Authentication flows, payment gateway integrations, behavior-driven services. 7. Builder Pattern Application Context: Constructs complex data objects or query parameters incrementally and fluently. Scalability Assessment: Enhances readability and maintainability of configuration logic. Use Cases: Dynamic query generation, DTO assembly, chained configuration utilities. 8. Observer Pattern Application Context: Facilitates loosely coupled event-driven communication between disparate modules. Scalability Assessment: Pivotal in reactive and event-oriented architectures. Use Cases: Domain event propagation, audit logging, notification broadcasting. 9. Middleware Pattern Application Context: Implements cross-cutting concerns at the request-processing pipeline stage. Scalability Assessment: Suitable for centralizing security and operational layers. Use Cases: Authentication, request logging, metrics injection. 10. Interceptor Pattern Application Context: Enables interception and transformation of method responses and error flows. Scalability Assessment: Ideal for implementing AOP-like constructs and reusable business rules. Use Cases: Response shaping, exception wrapping, conditional caching. 11. Adapter Pattern Application Context: Translates external APIs or systems into interfaces that conform to the application's expectations. Scalability Assessment: Indispensable for isolating infrastructure changes from domain logic. Use Cases: External payment services, cloud storage APIs, third-party logging. 12. Proxy Pattern Application Context: Intercepts method calls to augment behavior transparently. Scalability Assessment: Valuable in performance tuning and service orchestration. Use Cases: Rate limiting, lazy loading, distributed cache facades. 13. CQRS Pattern (Command Query Responsibility Segregation) Application Context: Segregates the responsibilities of data mutation and data retrieval. Scalability Assessment: Enables optimization of read/write models, crucial for high-throughput systems. Use Cases: Financial transactions, e-commerce systems, audit-tracked updates. 14. Ev
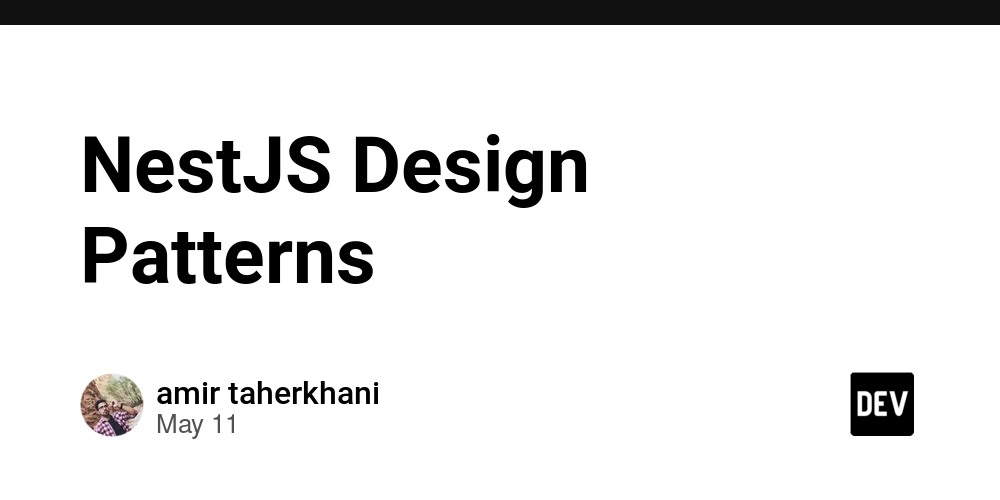
Analytical Perspectives and Strategic Applications for Scalable Architectures
This document serves as an advanced reference for software architects and backend engineers utilizing the NestJS framework to design robust, scalable, and maintainable systems. It systematically explores a broad spectrum of software design patterns, each tailored for application within the context of NestJS. With a focus on theoretical foundations, practical use cases, scalability considerations, and illustrative code examples, this guide bridges conceptual architecture with real-world development practices.
1. Modular Architecture Pattern
Application Context: Facilitates the decomposition of a system into discrete, feature-centric modules, each encapsulating domain logic and dependencies.
Scalability Assessment: Highly effective for scaling large codebases by promoting bounded contexts and autonomous development cycles.
Use Cases: Domain-driven design, feature toggling, microservice-aligned code separation.
2. Dependency Injection (DI) Pattern
Application Context: Enables decoupled class instantiation and promotes inversion of control, enhancing flexibility in managing dependencies.
Scalability Assessment: Foundational to scalable NestJS systems; facilitates composability and unit testing.
Use Cases: Dynamic configuration loading, multi-tenant environments, and mocking in unit tests.
3. Controller-Service-Repository Pattern
Application Context: Establishes a hierarchical structure for separating HTTP handling, business logic, and data access.
Scalability Assessment: Promotes maintainability, reusability, and abstraction—vital in large-scale applications.
Use Cases: RESTful APIs, layered services, structured domain-driven implementations.
4. Repository Pattern
Application Context: Abstracts data layer interactions, allowing substitution of storage backends without impacting business logic.
Scalability Assessment: Essential for persistence-agnostic design; simplifies testing and data access refactoring.
Use Cases: ORM abstraction, mocking data sources, transitioning between databases.
5. Factory Pattern
Application Context: Constructs instances dynamically based on runtime criteria or configurations.
Scalability Assessment: Highly beneficial in multi-environment setups or pluggable architectures.
Use Cases: Logging configuration, dependency instantiation based on context, strategy selectors.
6. Strategy Pattern
Application Context: Encapsulates algorithmic behavior and enables dynamic substitution without modifying consuming logic.
Scalability Assessment: Supports extensibility and modular business logic injection.
Use Cases: Authentication flows, payment gateway integrations, behavior-driven services.
7. Builder Pattern
Application Context: Constructs complex data objects or query parameters incrementally and fluently.
Scalability Assessment: Enhances readability and maintainability of configuration logic.
Use Cases: Dynamic query generation, DTO assembly, chained configuration utilities.
8. Observer Pattern
Application Context: Facilitates loosely coupled event-driven communication between disparate modules.
Scalability Assessment: Pivotal in reactive and event-oriented architectures.
Use Cases: Domain event propagation, audit logging, notification broadcasting.
9. Middleware Pattern
Application Context: Implements cross-cutting concerns at the request-processing pipeline stage.
Scalability Assessment: Suitable for centralizing security and operational layers.
Use Cases: Authentication, request logging, metrics injection.
10. Interceptor Pattern
Application Context: Enables interception and transformation of method responses and error flows.
Scalability Assessment: Ideal for implementing AOP-like constructs and reusable business rules.
Use Cases: Response shaping, exception wrapping, conditional caching.
11. Adapter Pattern
Application Context: Translates external APIs or systems into interfaces that conform to the application's expectations.
Scalability Assessment: Indispensable for isolating infrastructure changes from domain logic.
Use Cases: External payment services, cloud storage APIs, third-party logging.
12. Proxy Pattern
Application Context: Intercepts method calls to augment behavior transparently.
Scalability Assessment: Valuable in performance tuning and service orchestration.
Use Cases: Rate limiting, lazy loading, distributed cache facades.
13. CQRS Pattern (Command Query Responsibility Segregation)
Application Context: Segregates the responsibilities of data mutation and data retrieval.
Scalability Assessment: Enables optimization of read/write models, crucial for high-throughput systems.
Use Cases: Financial transactions, e-commerce systems, audit-tracked updates.
14. Event Bus (Pub/Sub) Pattern
Application Context: Disseminates domain events to loosely coupled subscribers asynchronously.
Scalability Assessment: Empowers event-driven and eventually consistent distributed systems.
Use Cases: Workflow orchestration, integration events, reactive microservices.
15. Singleton Pattern
Application Context: Guarantees a single shared instance across the application lifecycle.
Scalability Assessment: Effective for shared configurations and resource pools.
Use Cases: Configuration services, logging services, environment-sensitive utilities.
16. Hexagonal Architecture (Ports and Adapters)
Application Context: Organizes systems into inbound/outbound interfaces surrounding a core domain model.
Scalability Assessment: Highly adaptable for long-term evolution and infrastructure independence.
Use Cases: Domain-driven applications, API and CLI adapters, decoupling infrastructure dependencies.
17. Bridge Pattern
Application Context: Decouples abstraction from its implementation, allowing both to evolve independently.
Scalability Assessment: Useful in building extensible service bridges with multiple layers of variation.
Use Cases: Logging frameworks, external storage abstractions, service mediators.
18. Decorator Pattern
Application Context: Augments class behavior dynamically via metadata and reflection.
Scalability Assessment: Core to NestJS metaprogramming; highly composable.
Use Cases: Guards, interceptors, pipes, dependency tagging.
19. Template Method Pattern
Application Context: Defines skeleton workflows with customizable sub-steps.
Scalability Assessment: Facilitates uniform operations while permitting domain-specific overrides.
Use Cases: Job pipelines, ETL routines, test scenario execution.
20. Chain of Responsibility Pattern
Application Context: Processes a request through a sequence of handlers.
Scalability Assessment: Ideal for modular middleware pipelines and dynamic command resolution.
Use Cases: Authorization stacks, validation layers, dynamic event routers.
21. Command Pattern
Application Context: Encapsulates operations as discrete objects, enabling queuing, logging, and replayability.
Scalability Assessment: Well-suited for task-oriented and distributed command processing systems.
Use Cases: CQRS command dispatchers, job queues, undo/redo stacks.
Example Structures and Usage
1. Modular Architecture Pattern
Example:
// users.module.ts
@Module({
controllers: [UsersController],
providers: [UsersService],
exports: [UsersService],
})
export class UsersModule {}
2. Dependency Injection (DI) Pattern
Example:
@Injectable()
export class AuthService {
constructor(private readonly usersService: UsersService) {}
}
3. Controller-Service-Repository Pattern
Example:
@Controller('users')
export class UsersController {
constructor(private readonly usersService: UsersService) {}
@Get()
findAll() {
return this.usersService.findAll();
}
}
4. Repository Pattern
Example:
@Injectable()
export class UserRepository {
constructor(private readonly prisma: PrismaService) {}
async findById(id: string): Promise<User | null> {
return this.prisma.user.findUnique({ where: { id } });
}
async create(data: CreateUserDto): Promise<User> {
return this.prisma.user.create({ data });
}
}
5. Factory Pattern
Example:
@Module({
providers: [
{
provide: 'ASYNC_LOGGER',
useFactory: async (configService: ConfigService) => {
return new LoggerService(configService.get('LOG_LEVEL'));
},
inject: [ConfigService],
},
],
})
export class LoggingModule {}
6. Strategy Pattern
Example:
@Injectable()
export class JwtStrategy extends PassportStrategy(Strategy) {
constructor(private configService: ConfigService) {
super({
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
secretOrKey: configService.get('JWT_SECRET'),
});
}
}
7. Builder Pattern
Example:
const query = new UserQueryBuilder()
.withAge(30)
.withStatus('active')
.build();
8. Observer Pattern
Example:
@OnEvent('user.created')
handleUserCreated(user: User) {
this.mailService.sendWelcomeEmail(user.email);
}
9. Middleware Pattern
Example:
@Injectable()
export class AuthMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: Function) {
console.log('Checking auth...');
next();
}
}
10. Interceptor Pattern
Example:
@Injectable()
export class ResponseInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable<any> {
return next.handle().pipe(map(data => ({ success: true, data })));
}
}
11. Adapter Pattern
Example:
export class StripePaymentAdapter implements PaymentInterface {
charge(amount: number) {
return this.stripe.charge({ amount });
}
}
12. Proxy Pattern
Example:
@Injectable()
export class CachingProxy {
constructor(private readonly redisService: RedisService) {}
async getData(key: string, fallback: () => Promise<any>) {
const cached = await this.redisService.get(key);
if (cached) return JSON.parse(cached);
const result = await fallback();
await this.redisService.set(key, JSON.stringify(result));
return result;
}
}
13. CQRS Pattern (Command Query Responsibility Segregation)
Example:
export class CreateUserCommand {
constructor(public readonly username: string) {}
}
@CommandHandler(CreateUserCommand)
export class CreateUserHandler implements ICommandHandler<CreateUserCommand> {
async execute(command: CreateUserCommand) {
// handle logic
}
}
14. Event Bus (Pub/Sub) Pattern
Example:
export class UserCreatedEvent {
constructor(public readonly userId: string) {}
}
@EventsHandler(UserCreatedEvent)
export class SendEmailOnUserCreated {
handle(event: UserCreatedEvent) {
// send email logic
}
}
15. Singleton Pattern
Example:
@Injectable()
export class ConfigService {
private readonly config = new Map();
get(key: string): string {
return this.config.get(key);
}
}
16. Hexagonal Architecture (Ports and Adapters)
Example:
// Port interface
export interface NotificationPort {
send(email: string, message: string): Promise<void>;
}
// Adapter implementation
@Injectable()
export class EmailService implements NotificationPort {
async send(email: string, message: string): Promise<void> {
// send email
}
}
17. Bridge Pattern
Example:
abstract class Logger {
constructor(protected transport: Transport) {}
abstract log(message: string): void;
}
class FileLogger extends Logger {
log(message: string) {
this.transport.writeToFile(message);
}
}
18. Decorator Pattern
Example:
@UseGuards(AuthGuard)
@Get('profile')
getProfile(@Request() req) {
return req.user;
}
19. Template Method Pattern
Example:
abstract class ReportGenerator {
generate() {
this.fetchData();
this.formatData();
this.export();
}
abstract fetchData(): void;
abstract formatData(): void;
export() {
console.log('Exporting report...');
}
}
20. Chain of Responsibility Pattern
Example:
@Injectable()
export class AuthHandler {
setNext(handler: AuthHandler): AuthHandler {
this.nextHandler = handler;
return handler;
}
handle(request: any) {
if (this.canHandle(request)) {
return this.process(request);
} else if (this.nextHandler) {
return this.nextHandler.handle(request);
}
}
}
21. Command Pattern
Example:
export class DeleteUserCommand {
constructor(public readonly userId: string) {}
}
@CommandHandler(DeleteUserCommand)
export class DeleteUserHandler implements ICommandHandler<DeleteUserCommand> {
async execute(command: DeleteUserCommand) {
// delete user logic
}
}
Design Pattern Popularity Chart
Modular Architecture ██████████████████████████████████████ 95
Dependency Injection ███████████████████████████████████ 90
Controller-Service-Repo ████████████████████████████████ 88
CQRS █████████████████████████████ 85
Hexagonal Architecture ██████████████████████████ 80
Observer █████████████████████████ 78
Strategy ████████████████████████ 75
Repository █████████████████████ 70
Factory ████████████████████ 68
Event Bus ███████████████████ 65
Design Pattern Categories and Grouping
Category | Design Patterns |
---|---|
Architectural | - Modular Architecture - Hexagonal Architecture |
Structural | - Controller-Service-Repository - Repository - Adapter - Bridge - Decorator |
Creational | - Factory - Singleton - Builder |
Behavioral | - Strategy - Observer - Interceptor - Middleware - Chain of Responsibility - Command - Template Method - Proxy |
Distributed/Event | - Event Bus - CQRS |
Cross-Cutting | - Dependency Injection |
Summary Table
Pattern | Representative Context |
---|---|
Modular | Feature separation |
Dependency Injection | Configurable service instantiation |
Controller-Service-Repo | HTTP, logic, and persistence layers |
Factory | Dynamic provider instantiation |
Strategy | Behavior switching |
Builder | Complex query generation |
Observer | Event broadcast |
Adapter | External integration normalization |
Proxy | Caching, throttling |
Singleton | Shared runtime service |
Interceptor | Response transformation |
Middleware | Request preprocessing |
CQRS / Command / Event | Separated query/command processing |
Decorator | Class-level metadata annotation |
Chain of Responsibility | Sequential handler pipeline |
Hexagonal | Core-domain isolation |
Conclusion:
The NestJS framework inherently supports various architectural and design patterns that enable scalable, modular, and testable system construction. Mastery and strategic application of these patterns are vital in addressing complexity, maintaining separation of concerns, and facilitating agile development cycles across distributed and enterprise-grade software platforms.