Managing Form State in React: Separate State Variables vs. Single State Object
Managing form state is an essential part of building interactive React applications. When handling forms, you have two common approaches: using separate useState calls for each input or storing all form data in a single useState object. Each method has its advantages and trade-offs, so let’s break them down to help you decide which approach best fits your needs. The Separate State Variables Approach In this approach, each input field gets its own useState variable. This keeps things simple and explicit, making it easy to control each field independently. This is a straightforward way to manage form state, especially for small forms. Here’s an example of a simple form that adds a pet and its information to a list of pets: function AddPetForm() { // Each input has its own state const [species, setSpecies] = useState(""); const [name, setName] = useState(""); const [breed, setBreed] = useState(""); const [age, setAge] = useState(""); const handleSubmit = (e) => { e.preventDefault(); console.log({ species, name, breed, age }); // Logs all values separately }; return ( {/* Each input updates a specific state variable */} setSpecies(e.target.value)} placeholder="Species" /> setName(e.target.value)} placeholder="Name" /> setBreed(e.target.value)} placeholder="Breed" /> setAge(e.target.value)} placeholder="Age" /> Add Pet ); } Why Use This Approach? This approach keeps each input field separate, making state management clear and explicit. If an issue arises, debugging is easier since you can pinpoint exactly which state variable is affected. Additionally, it allows for detailed control, enabling you to update or validate individual fields without impacting others. Where It Falls Short: This approach can lead to repetitive code, as you'll need to write multiple similar useState calls and event handlers for each input. It's also not ideal for larger forms, as maintaining separate states for each field can become cumbersome as the form and its data grows. The Single State Object Approach Instead of using multiple state variables, you can consolidate all form data into a single object. This makes managing larger forms more efficient. Here’s the same AddPetForm component using a single state object: function AddPetForm() { // All form fields are stored in the single state object formData const [formData, setFormData] = useState({ species: "", name: "", breed: "", age: "" }); // Updates only the changed field while keeping the rest of the state intact const handleChange = (e) => { setFormData({ ...formData, [e.target.name]: e.target.value }); }; const handleSubmit = (e) => { e.preventDefault(); console.log(formData); // Logs all values as an object }; return ( {/* Each input updates the corresponding property in formData */} Add Pet ); } Why Use This Approach? Single State Objects are less repetitive, You only need one useState call, which is easier to manage as forms grow in size. It's also potentially easier to scale—when new fields are added, you don’t need to create additional state variables; you can simply add them to the object and create new input fields in your returned JSX. Where It Falls Short: Managing state with a single object can introduce complexities. You need to use object spread (...formData) to preserve the previous state while updating just one field, errors here might lead to overwriting other fields. Additionally, handling a single object requires managing dynamic keys and field changes, which can make the logic more complex, especially for simpler forms. Key Differences & When to Use Each Approach Separate State Variables: Best for: Smaller, simpler forms or cases where individual inputs need separate handling. When to Use: When you want explicit control over each field’s state, or when you’re dealing with a small form that won’t grow too large. Single State Object: Best for: Larger forms with many fields or when you need to keep related data together in a central object. When to Use: When scalability is important, or when managing form state as a group is more logical (e.g., user profile forms). Final Thoughts Both approaches are valid, and the best choice depends on your specific use case. The separate state variables method is easy to implement and works well for small forms, but as forms grow, managing state in a single object can make things more scalable and maintainable. Understanding both methods will help you decide which one fits your project best. If you're just starting out, experiment with both in different contexts (such as fetching and updating data from an api) and see what works best for you.
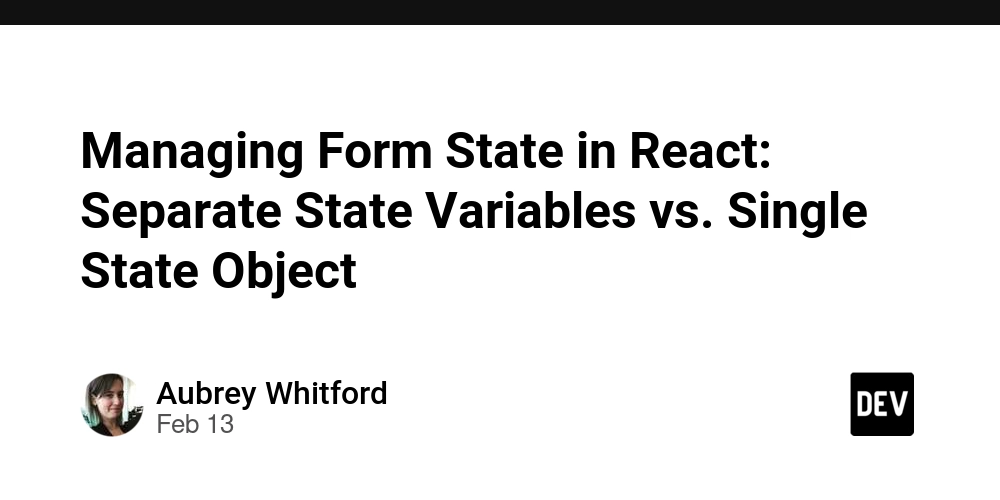
Managing form state is an essential part of building interactive React applications. When handling forms, you have two common approaches: using separate useState calls for each input or storing all form data in a single useState object. Each method has its advantages and trade-offs, so let’s break them down to help you decide which approach best fits your needs.
The Separate State Variables Approach
In this approach, each input field gets its own useState variable. This keeps things simple and explicit, making it easy to control each field independently. This is a straightforward way to manage form state, especially for small forms.
Here’s an example of a simple form that adds a pet and its information to a list of pets:
function AddPetForm() {
// Each input has its own state
const [species, setSpecies] = useState("");
const [name, setName] = useState("");
const [breed, setBreed] = useState("");
const [age, setAge] = useState("");
const handleSubmit = (e) => {
e.preventDefault();
console.log({ species, name, breed, age }); // Logs all values separately
};
return (
);
}
Why Use This Approach?
This approach keeps each input field separate, making state management clear and explicit. If an issue arises, debugging is easier since you can pinpoint exactly which state variable is affected. Additionally, it allows for detailed control, enabling you to update or validate individual fields without impacting others.
Where It Falls Short:
This approach can lead to repetitive code, as you'll need to write multiple similar useState calls and event handlers for each input. It's also not ideal for larger forms, as maintaining separate states for each field can become cumbersome as the form and its data grows.
The Single State Object Approach
Instead of using multiple state variables, you can consolidate all form data into a single object. This makes managing larger forms more efficient.
Here’s the same AddPetForm component using a single state object:
function AddPetForm() {
// All form fields are stored in the single state object formData
const [formData, setFormData] = useState({ species: "", name: "", breed: "", age: "" });
// Updates only the changed field while keeping the rest of the state intact
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
const handleSubmit = (e) => {
e.preventDefault();
console.log(formData); // Logs all values as an object
};
return (
);
}
Why Use This Approach?
Single State Objects are less repetitive, You only need one useState call, which is easier to manage as forms grow in size. It's also potentially easier to scale—when new fields are added, you don’t need to create additional state variables; you can simply add them to the object and create new input fields in your returned JSX.
Where It Falls Short:
Managing state with a single object can introduce complexities. You need to use object spread (...formData) to preserve the previous state while updating just one field, errors here might lead to overwriting other fields. Additionally, handling a single object requires managing dynamic keys and field changes, which can make the logic more complex, especially for simpler forms.
Key Differences & When to Use Each Approach
Separate State Variables:
Best for: Smaller, simpler forms or cases where individual inputs need separate handling.
When to Use: When you want explicit control over each field’s state, or when you’re dealing with a small form that won’t grow too large.
Single State Object:
Best for: Larger forms with many fields or when you need to keep related data together in a central object.
When to Use: When scalability is important, or when managing form state as a group is more logical (e.g., user profile forms).
Final Thoughts
Both approaches are valid, and the best choice depends on your specific use case. The separate state variables method is easy to implement and works well for small forms, but as forms grow, managing state in a single object can make things more scalable and maintainable.
Understanding both methods will help you decide which one fits your project best. If you're just starting out, experiment with both in different contexts (such as fetching and updating data from an api) and see what works best for you.