Web Services Development
In today’s connected world, web services act as the backbone of communication between applications. Whether you're building mobile apps, web platforms, or enterprise systems, understanding how to develop robust web services is essential for modern developers. What are Web Services? A web service is a software component that enables applications to communicate with each other over the internet using standardized protocols like HTTP, XML, or JSON. Web services allow interoperability between different software applications running on various platforms. Types of Web Services RESTful Web Services: Use HTTP methods (GET, POST, PUT, DELETE) and are based on REST architecture. Lightweight and widely used. SOAP Web Services: Use XML-based messaging and offer more rigid structure and security, often used in enterprise systems. GraphQL: A newer alternative that allows clients to request exactly the data they need. Common Tools and Frameworks Node.js (Express): Great for building lightweight REST APIs. Spring Boot (Java): A robust framework for REST and SOAP services. Django (Python): Offers built-in support for creating APIs via Django REST Framework. ASP.NET (C#): Common in enterprise-level SOAP/REST applications. Sample REST API in Node.js const express = require('express'); const app = express(); app.get('/api/hello', (req, res) => { res.json({ message: 'Hello from the web service!' }); }); app.listen(3000, () => { console.log('Server running on port 3000'); }); Key Concepts to Understand HTTP Methods and Status Codes Authentication (API keys, OAuth, JWT) Data Serialization (JSON, XML) Cross-Origin Resource Sharing (CORS) API Documentation (Swagger/OpenAPI) Best Practices Design APIs with clear endpoints and meaningful names. Use proper status codes to indicate success or errors. Secure your APIs using authentication and rate limiting. Provide thorough documentation for developers. Test APIs using tools like Postman or Insomnia. Use Cases of Web Services Mobile App Backends Payment Gateways Cloud-Based Services Weather or Location APIs Social Media Integration Conclusion Web services are critical for building scalable and flexible software systems. By mastering web service development, you’ll be able to connect applications, share data, and build feature-rich platforms that serve users across devices and platforms.
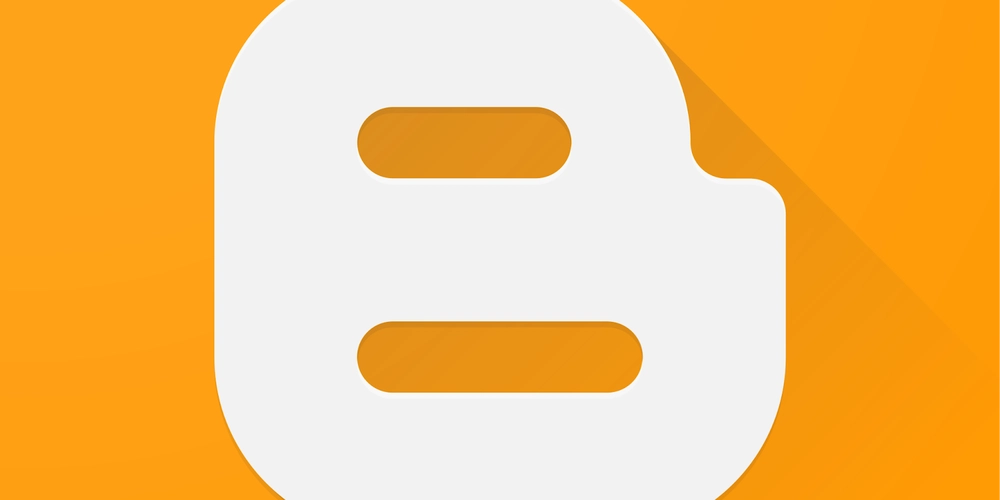
In today’s connected world, web services act as the backbone of communication between applications. Whether you're building mobile apps, web platforms, or enterprise systems, understanding how to develop robust web services is essential for modern developers.
What are Web Services?
A web service is a software component that enables applications to communicate with each other over the internet using standardized protocols like HTTP, XML, or JSON. Web services allow interoperability between different software applications running on various platforms.
Types of Web Services
- RESTful Web Services: Use HTTP methods (GET, POST, PUT, DELETE) and are based on REST architecture. Lightweight and widely used.
- SOAP Web Services: Use XML-based messaging and offer more rigid structure and security, often used in enterprise systems.
- GraphQL: A newer alternative that allows clients to request exactly the data they need.
Common Tools and Frameworks
- Node.js (Express): Great for building lightweight REST APIs.
- Spring Boot (Java): A robust framework for REST and SOAP services.
- Django (Python): Offers built-in support for creating APIs via Django REST Framework.
- ASP.NET (C#): Common in enterprise-level SOAP/REST applications.
Sample REST API in Node.js
const express = require('express');
const app = express();
app.get('/api/hello', (req, res) => {
res.json({ message: 'Hello from the web service!' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Key Concepts to Understand
- HTTP Methods and Status Codes
- Authentication (API keys, OAuth, JWT)
- Data Serialization (JSON, XML)
- Cross-Origin Resource Sharing (CORS)
- API Documentation (Swagger/OpenAPI)
Best Practices
- Design APIs with clear endpoints and meaningful names.
- Use proper status codes to indicate success or errors.
- Secure your APIs using authentication and rate limiting.
- Provide thorough documentation for developers.
- Test APIs using tools like Postman or Insomnia.
Use Cases of Web Services
- Mobile App Backends
- Payment Gateways
- Cloud-Based Services
- Weather or Location APIs
- Social Media Integration
Conclusion
Web services are critical for building scalable and flexible software systems. By mastering web service development, you’ll be able to connect applications, share data, and build feature-rich platforms that serve users across devices and platforms.