Python Doesn’t Support True Constants – So I Built “setconstant” to Fix That
If you’ve ever written code in C, C++, or Java, then you’ve probably enjoyed the luxury of constants—unchangeable variables that provide structure, safety, and clarity to your programs. But then there's Python—a language that espouses flexibility and simplicity, but too often at the expense of immutability. There is no const keyword. No built-in mechanism to avoid variables being overwritten. It's too easy to overwrite a value by mistake, and suddenly your application starts doing crazy things. That's not only a nuisance—it's unsafe. So I created something to solve that. Introducing setconstant: True Constants for Python Having encountered this problem one too many times, I finally had enough and decided to stop waiting for someone to fix it. Say hello to setconstant—a simple, powerful Python package that allows you to declare constants which cannot be changed or overridden. No more typos. No longer magic numbers scattered throughout your code. Merely crystal-clear, unchanging values. Why setconstant Matters Let's be honest: Python is adored for its dynamism. But when constructing dependable, large-scale systems, we require some values to never alter. A math constant such as PI. A global config such as APP_NAME. A gravity constant for a physics engine. With setconstant, now you can: Declare constants in seconds – integers, floats, or strings. Impose immutability – a constant cannot be altered once declared. Get instant feedback – try to reassign it, and you’ll get an error. Keep code clean – avoid hardcoding or duplicate values everywhere. How It Works import setconstant setconstant.const_i("PI", 3) setconstant.const_f("GRAVITY", 9.81) setconstant.const_s("APP_NAME", "CodeHaven") print(setconstant.get_constant("PI")) # Output: 3 print(setconstant.get_constant("GRAVITY")) # Output: 9.81 print(setconstant.get_constant("APP_NAME")) # Output: CodeHaven Trying to redefine? This will throw an error! setconstant.const_i("PI", 4) # Raises ValueError Just import, define, and use. It's that simple. Why You’ll Love It No more surprises – accidental overwrites are gone. Cleaner code – no more magic numbers or string literals. Safer logic – constants behave like they should. Simple setup – install and use immediately. Ready to Try It? Just install it with: pip install setconstant Whether you're developing a machine learning model, a physics engine, a web application, or simply writing good code, this package might make your life easier—and your code more stable. I made setconstant because I needed it as a developer. If it's helpful for you too, I'd appreciate your feedback. Let's create a better Python experience together. Let’s keep coding, cleanly and clearly. — Anuraj R
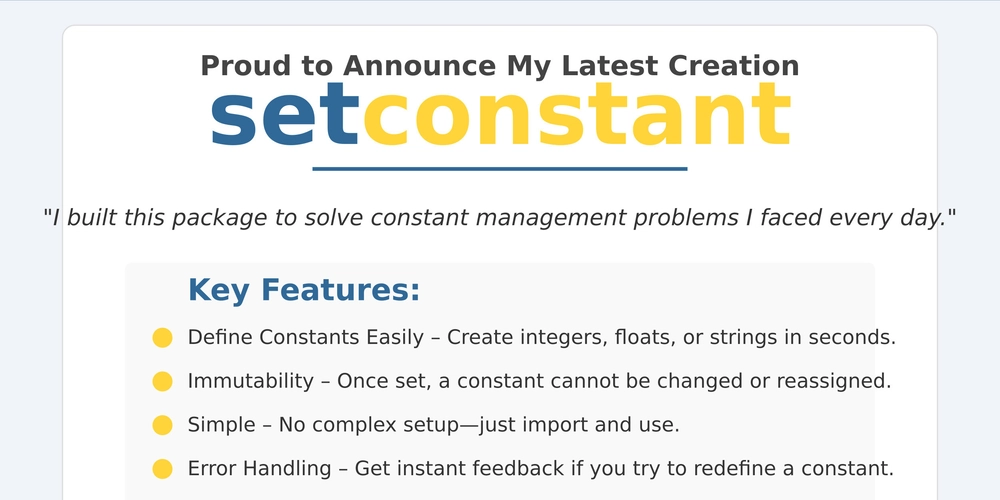
If you’ve ever written code in C, C++, or Java, then you’ve probably enjoyed the luxury of constants—unchangeable variables that provide structure, safety, and clarity to your programs.
But then there's Python—a language that espouses flexibility and simplicity, but too often at the expense of immutability. There is no const keyword. No built-in mechanism to avoid variables being overwritten. It's too easy to overwrite a value by mistake, and suddenly your application starts doing crazy things.
That's not only a nuisance—it's unsafe.
So I created something to solve that.
Introducing setconstant: True Constants for Python
Having encountered this problem one too many times, I finally had enough and decided to stop waiting for someone to fix it.
Say hello to setconstant—a simple, powerful Python package that allows you to declare constants which cannot be changed or overridden.
No more typos.
No longer magic numbers scattered throughout your code.
Merely crystal-clear, unchanging values.
Why setconstant Matters
Let's be honest: Python is adored for its dynamism. But when constructing dependable, large-scale systems, we require some values to never alter. A math constant such as PI. A global config such as APP_NAME. A gravity constant for a physics engine.
With setconstant, now you can:
Declare constants in seconds – integers, floats, or strings.
Impose immutability – a constant cannot be altered once declared.
Get instant feedback – try to reassign it, and you’ll get an error.
Keep code clean – avoid hardcoding or duplicate values everywhere.
How It Works
import setconstant
setconstant.const_i("PI", 3)
setconstant.const_f("GRAVITY", 9.81)
setconstant.const_s("APP_NAME", "CodeHaven")
print(setconstant.get_constant("PI")) # Output: 3
print(setconstant.get_constant("GRAVITY")) # Output: 9.81
print(setconstant.get_constant("APP_NAME")) # Output: CodeHaven
Trying to redefine? This will throw an error!
setconstant.const_i("PI", 4) # Raises ValueError
Just import, define, and use. It's that simple.
Why You’ll Love It
No more surprises – accidental overwrites are gone.
Cleaner code – no more magic numbers or string literals.
Safer logic – constants behave like they should.
Simple setup – install and use immediately.
Ready to Try It?
Just install it with:
pip install setconstant
Whether you're developing a machine learning model, a physics engine, a web application, or simply writing good code, this package might make your life easier—and your code more stable.
I made setconstant because I needed it as a developer. If it's helpful for you too, I'd appreciate your feedback. Let's create a better Python experience together.
Let’s keep coding, cleanly and clearly.