Readable and Writable Streams: Advanced Concepts
Readable and Writable Streams in JavaScript: Advanced Concepts Introduction The Node.js runtime has revolutionized how developers approach I/O operations, primarily through its implementation of streams. Streams facilitate the efficient handling of reading and writing data, enabling developers to process data incrementally rather than loading everything into memory at once. This article will delve deep into Readable and Writable Streams in Node.js, providing historical context, technical details, and advanced use cases. Historical Context Streams are not a new concept; they borrow heavily from the Unix philosophy of piping data between processes. Node.js introduced the stream module early in its development, effectively allowing non-blocking I/O operations and fostering a simpler, more efficient way to handle data that may not fit into memory altogether. Streams provide a foundation for readable and writable interfaces to abstract the complexities of I/O operations. The initial implementation in Node.js aimed to reflect the asynchronous nature understood by JavaScript developers, leveraging callbacks and event-driven logic. With the arrival of Promises and async/await in ES2015 and later, the landscape changed, allowing for even more sophisticated handling of streams. The Basics: Readable and Writable Streams Readable Streams In Node.js, a Readable Stream emits events that allow you to listen for data chunks, end-of-stream, and errors. The two primary methods are read() and pipe(). The read() method is crucial for manually controlling flow, while pipe() automatically handles the flow from a readable to a writable stream. Example: const { Readable } = require('stream'); const readable = new Readable({ read(size) { this.push('Hello, '); this.push('world!'); this.push(null); // signals EOF } }); readable.on('data', (chunk) => { console.log(chunk.toString()); }); In the above example, we create a simple Readable Stream emitting "Hello, world!" before signaling the end of the stream using null. Writable Streams Writable Streams provide a way to write data to an underlying resource, such as a file or network socket. Utilizing the write() and end() methods, Writable Streams can accept data over time. Example: const { Writable } = require('stream'); const writable = new Writable({ write(chunk, encoding, callback) { console.log(`Writing: ${chunk.toString()}`); callback(); // call when done } }); writable.write('Hello, '); writable.write('world!'); writable.end(); // signals completion Here, we create a Writable Stream that writes data to the console. Advanced Concepts 1. HighWaterMark and Buffering Streams operate with an internal buffer that helps manage the flow of data. The highWaterMark property establishes the buffer size, determining how much data can be queued before stopping the flow. When stream data exceeds the buffer, the producer will essentially pause until the consumer processes some of the buffered data. const { Readable } = require('stream'); const readable = new Readable({ read(size) { this.push('Data chunk'); }, highWaterMark: 5 }); readable.on('data', (chunk) => { console.log(`Buffered Data: ${chunk.toString()}`); }); Adjusting highWaterMark can optimize memory usage and performance. A fuller buffer may lead to higher throughput, but at the cost of increased memory consumption. 2. Implementing Transform Streams Transform Streams extend Writable and Readable Streams, enabling the modification of incoming data. The transform() method processes the input and can provide transformed data through the push() method. Example: const { Transform } = require('stream'); const transform = new Transform({ transform(chunk, encoding, callback) { const transformed = chunk.toString().toUpperCase(); this.push(transformed); callback(); } }); process.stdin.pipe(transform).pipe(process.stdout); In this scenario, input from stdin is transformed to uppercase before being output to stdout. Real-World Use Cases File Processing: Applications that read files (like log processors) benefit from streams, minimizing memory load. Network Operations: Efficiently stream data over the network in real time without overwhelming server resources. Data Transformation Pipelines: Streaming transforms enable parsing, filtering, and modifying data seamlessly between different sources and sinks. Performance Considerations When optimizing streams, consider the following areas: Buffer Management: Tweaking the highWaterMark can significantly impact performance based on your application’s requirements. Back Pressure: Handle back pressure effectively to prevent memory leaks. Utilize the drain event on Writable Streams to manage when it is safe to write ag
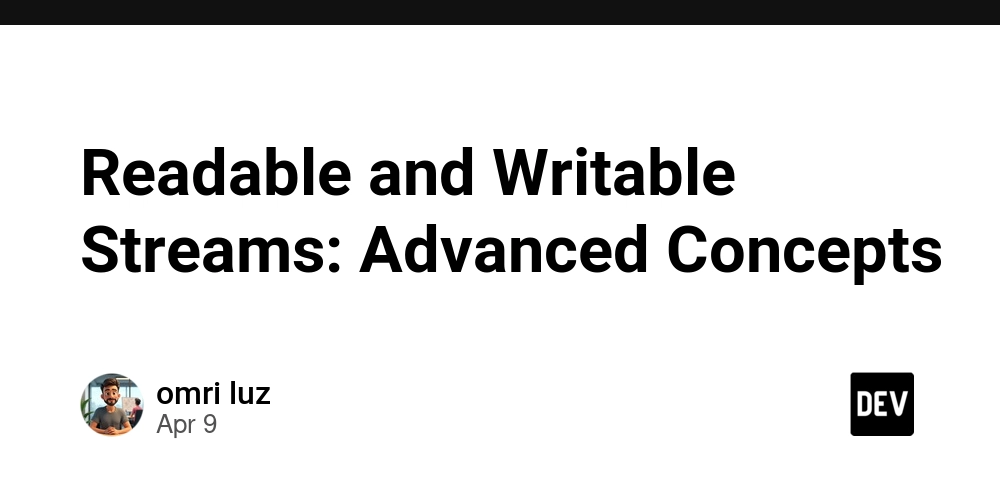
Readable and Writable Streams in JavaScript: Advanced Concepts
Introduction
The Node.js runtime has revolutionized how developers approach I/O operations, primarily through its implementation of streams. Streams facilitate the efficient handling of reading and writing data, enabling developers to process data incrementally rather than loading everything into memory at once. This article will delve deep into Readable and Writable Streams in Node.js, providing historical context, technical details, and advanced use cases.
Historical Context
Streams are not a new concept; they borrow heavily from the Unix philosophy of piping data between processes. Node.js introduced the stream module early in its development, effectively allowing non-blocking I/O operations and fostering a simpler, more efficient way to handle data that may not fit into memory altogether. Streams provide a foundation for readable and writable interfaces to abstract the complexities of I/O operations.
The initial implementation in Node.js aimed to reflect the asynchronous nature understood by JavaScript developers, leveraging callbacks and event-driven logic. With the arrival of Promises and async/await in ES2015 and later, the landscape changed, allowing for even more sophisticated handling of streams.
The Basics: Readable and Writable Streams
Readable Streams
In Node.js, a Readable Stream emits events that allow you to listen for data chunks, end-of-stream, and errors. The two primary methods are read()
and pipe()
. The read()
method is crucial for manually controlling flow, while pipe()
automatically handles the flow from a readable to a writable stream.
Example:
const { Readable } = require('stream');
const readable = new Readable({
read(size) {
this.push('Hello, ');
this.push('world!');
this.push(null); // signals EOF
}
});
readable.on('data', (chunk) => {
console.log(chunk.toString());
});
In the above example, we create a simple Readable Stream emitting "Hello, world!" before signaling the end of the stream using null
.
Writable Streams
Writable Streams provide a way to write data to an underlying resource, such as a file or network socket. Utilizing the write()
and end()
methods, Writable Streams can accept data over time.
Example:
const { Writable } = require('stream');
const writable = new Writable({
write(chunk, encoding, callback) {
console.log(`Writing: ${chunk.toString()}`);
callback(); // call when done
}
});
writable.write('Hello, ');
writable.write('world!');
writable.end(); // signals completion
Here, we create a Writable Stream that writes data to the console.
Advanced Concepts
1. HighWaterMark and Buffering
Streams operate with an internal buffer that helps manage the flow of data. The highWaterMark
property establishes the buffer size, determining how much data can be queued before stopping the flow. When stream data exceeds the buffer, the producer will essentially pause until the consumer processes some of the buffered data.
const { Readable } = require('stream');
const readable = new Readable({
read(size) {
this.push('Data chunk');
},
highWaterMark: 5
});
readable.on('data', (chunk) => {
console.log(`Buffered Data: ${chunk.toString()}`);
});
Adjusting highWaterMark
can optimize memory usage and performance. A fuller buffer may lead to higher throughput, but at the cost of increased memory consumption.
2. Implementing Transform Streams
Transform Streams extend Writable and Readable Streams, enabling the modification of incoming data. The transform()
method processes the input and can provide transformed data through the push()
method.
Example:
const { Transform } = require('stream');
const transform = new Transform({
transform(chunk, encoding, callback) {
const transformed = chunk.toString().toUpperCase();
this.push(transformed);
callback();
}
});
process.stdin.pipe(transform).pipe(process.stdout);
In this scenario, input from stdin
is transformed to uppercase before being output to stdout
.
Real-World Use Cases
File Processing: Applications that read files (like log processors) benefit from streams, minimizing memory load.
Network Operations: Efficiently stream data over the network in real time without overwhelming server resources.
Data Transformation Pipelines: Streaming transforms enable parsing, filtering, and modifying data seamlessly between different sources and sinks.
Performance Considerations
When optimizing streams, consider the following areas:
Buffer Management: Tweaking the
highWaterMark
can significantly impact performance based on your application’s requirements.Back Pressure: Handle back pressure effectively to prevent memory leaks. Utilize the
drain
event on Writable Streams to manage when it is safe to write again.Pipeline Management: Use
pipeline()
from thestream
module to manage error handling and clean closure of streams.
const { pipeline } = require('stream');
pipeline(
readableStream,
transformStream,
writableStream,
(err) => {
if (err) {
console.error('Pipeline failed.', err);
} else {
console.log('Pipeline succeeded.');
}
}
);
Advanced Debugging Techniques
Logging: Implement strong logging of data events and errors using middleware.
Event Listeners: Leverage all events (data, error, finish, end) to gain insights.
Debugging Tools: Tools like
node --inspect
can help you analyze how data flows through streams.
Potential Pitfalls
Memory Leaks: Be cautious with unhandled buffers. Events like
drain
, or using theclose
event, can help you manage memory effectively.Error Handling: Ensure that you have included robust error handling in your streams. Failing to handle errors can lead to unresponsive applications.
Complexity: Avoid overly complex stream chains that are hard to maintain. Use modular functions to simplify your transformations.
Conclusion
Readable and Writable Streams in Node.js are powerful constructs that allow for effective, efficient, and performant data handling. As applications grow in complexity, understanding the advanced concepts and intricacies of streams becomes essential for any senior developer. This comprehensive examination provides a solid foundation and serves as a reference for deeper exploration and understanding of JavaScript's streaming capabilities.
References
- Official Node.js documentation on Streams: Node.js Stream Documentation
- A deep dive into performance optimization: Performance in Node.js
- Learn about how to handle streams: Learn Node.js Streams
By leveraging these advanced concepts and best practices, developers can create scalable, high-performance applications that effectively manage and manipulate data streams in real-time.