Python Built-in Data Structures(I)
Recently, a Python instructor asked me whether a set is mutable or immutable. I can't clearly remember what I said, but I did get the last part of the words correct because I remember saying '-utable', which reminded me of the viral video of the backbenchers in a class answering that the pressure 'creases' instead of a comprehensive 'increases' or 'decreases'. This led me to researching the built-in data structures in Python, and below is the first part of a summary of my findings. Lists fruits = ["banana", "mango", "watermelon"] Lists are used to store multiple items in a single variable. They can be defined using square brackets [ ] or the list type function Key Features of Lists Ordered - maintain the order of elements. Mutable - items can be modified after creation. Heterogeneous - elements can be of different data types. Allow duplicates - can contain duplicate values. Indexed - accessing items can be done directly using their index, starting from 0. It is important to note that lists store references rather than values. Elements in a list are not stored directly in the list structure; rather, the list stores pointers to the actual objects in memory. Common List Operations Method Description count(x) Returns the number of times x appears in the list append(x) Adds x to the end of the list insert(i,x) Inserts x at index i remove(x) Remove the first item with the value x pop([i]) Remove item at position i and return it clear() Removes all items from the list reverse() Reverse the elements of the list in place index(x) Returns the index of the first value of x sort() Lists in ascending or descending order copy() Returns the shallow copy of the list List Comprehensions List comprehensions provide a concise way of creating new lists based on the values of an existing list. For example, we have a list of numbers and we want to generate the square of each element in the list. numbers = [1, 2, 3, 4] #List comprehension to create a new list squared_numbers = [num** 2 for num in numbers] print(squared_numbers) Output [1, 4, 9, 16] When to use Lists Storing collections of related items. For example, a program that tracks student names in a classroom would use a list like students = ["Njoroge", "Karen", "Fatuma", "Chebet", "Juma"] to maintain all names in one organized structure. Iterating through sequential data. For example, when sending email notifications to multiple recipients, a program would loop through a list of email addresses to send the same message to each person. Temporary storage of results. For example, when scraping a website, a program accumulates all URLs in a list before processing them further or saving them to a database. Lists allow programmers to create new data collections by transforming or filtering existing data. For example, automatically create a new list with just the scores above 70 for a list of test scores. Tuples my_tuple = ("Alice", 15, "Student") Tuples, like lists, store multiple items in a single variable. The primary difference, however, is that tuples cannot be changed after their creation. Key Features of Tuples Ordered - elements are in a specific sequence. Immutable - cannot be changed after their creation. Defined using parentheses(). Allow duplicates. Heterogeneous - can contain mixed data types. Common Tuple Operations Due to the immutability of tuples, there are only two tuple methods that a tuple object can call. Method Description count(x) Returns the number of times x appears in tuple index(x) Returns the index of the first occurrence of x When to use Tuples For efficient looping - useful for looping over fixed data like days of the week in a calendar app. Packing/unpacking values - for example, when processing student records, like extracting name and grade from a database tuple. Immutable records - good for creating read-only data structures like coordinates or database rows. Dictionary keys - tuples of coordinates (latitude, longitude) can be used as dictionary keys to store location details, ensuring consistency and suitability for lookup operations. As mentioned before, the fundamental difference between lists and tuples is that lists are mutable while tuples are not. As a result, list object provide more methods but at the cost of memory space. Take the example below: import sys a_list = ['Maina', 'Football', 1, 3.142, True] a_tuple = ('Chebet', 'Tennis', 2, 13.31, False) print('The list size:', sys.getsizeof(a_list), 'bytes') print('The tuple size:', sys.getsizeof(a_tuple), 'bytes') Outputs The list size: 104 bytes The tuple size: 80 bytes This difference becomes more apparent when working with big data. Immutability makes notable optimization as well as making processing much faster, which is efficient when working with millions of sequence objects. Thus far, can you explain when
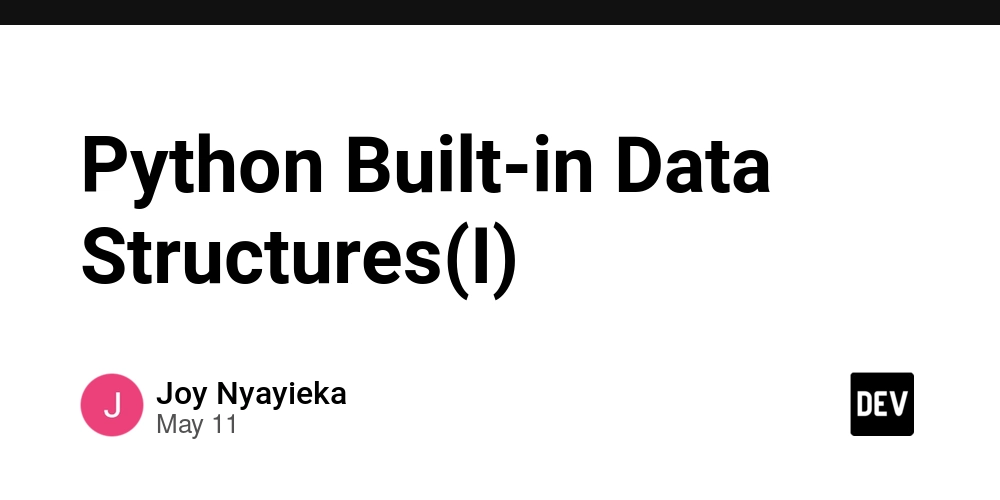
Recently, a Python instructor asked me whether a set is mutable or immutable. I can't clearly remember what I said, but I did get the last part of the words correct because I remember saying '-utable', which reminded me of the viral video of the backbenchers in a class answering that the pressure 'creases' instead of a comprehensive 'increases' or 'decreases'. This led me to researching the built-in data structures in Python, and below is the first part of a summary of my findings.
Lists
fruits = ["banana", "mango", "watermelon"]
Lists are used to store multiple items in a single variable. They can be defined using square brackets [ ] or the list type function
Key Features of Lists
- Ordered - maintain the order of elements.
- Mutable - items can be modified after creation.
- Heterogeneous - elements can be of different data types.
- Allow duplicates - can contain duplicate values.
- Indexed - accessing items can be done directly using their index, starting from 0.
It is important to note that lists store references rather than values. Elements in a list are not stored directly in the list structure; rather, the list stores pointers to the actual objects in memory.
Common List Operations
Method | Description |
---|---|
count(x) |
Returns the number of times x appears in the list |
append(x) |
Adds x to the end of the list |
insert(i,x) |
Inserts x at index i |
remove(x) |
Remove the first item with the value x |
pop([i]) |
Remove item at position i and return it |
clear() |
Removes all items from the list |
reverse() |
Reverse the elements of the list in place |
index(x) |
Returns the index of the first value of x |
sort() |
Lists in ascending or descending order |
copy() |
Returns the shallow copy of the list |
List Comprehensions
List comprehensions provide a concise way of creating new lists based on the values of an existing list.
For example, we have a list of numbers and we want to generate the square of each element in the list.
numbers = [1, 2, 3, 4]
#List comprehension to create a new list
squared_numbers = [num** 2 for num in numbers]
print(squared_numbers)
Output
[1, 4, 9, 16]
When to use Lists
Storing collections of related items. For example, a program that tracks student names in a classroom would use a list like
students = ["Njoroge", "Karen", "Fatuma", "Chebet", "Juma"]
to maintain all names in one organized structure.Iterating through sequential data. For example, when sending email notifications to multiple recipients, a program would loop through a list of email addresses to send the same message to each person.
Temporary storage of results. For example, when scraping a website, a program accumulates all URLs in a list before processing them further or saving them to a database.
Lists allow programmers to create new data collections by transforming or filtering existing data. For example, automatically create a new list with just the scores above 70 for a list of test scores.
Tuples
my_tuple = ("Alice", 15, "Student")
Tuples, like lists, store multiple items in a single variable. The primary difference, however, is that tuples cannot be changed after their creation.
Key Features of Tuples
- Ordered - elements are in a specific sequence.
- Immutable - cannot be changed after their creation.
- Defined using parentheses().
- Allow duplicates.
- Heterogeneous - can contain mixed data types.
Common Tuple Operations
Due to the immutability of tuples, there are only two tuple methods that a tuple object can call.
Method | Description |
---|---|
count(x) |
Returns the number of times x appears in tuple |
index(x) |
Returns the index of the first occurrence of x
|
When to use Tuples
- For efficient looping - useful for looping over fixed data like days of the week in a calendar app.
- Packing/unpacking values - for example, when processing student records, like extracting name and grade from a database tuple.
- Immutable records - good for creating read-only data structures like coordinates or database rows.
- Dictionary keys - tuples of coordinates (latitude, longitude) can be used as dictionary keys to store location details, ensuring consistency and suitability for lookup operations.
As mentioned before, the fundamental difference between lists and tuples is that lists are mutable while tuples are not. As a result, list object provide more methods but at the cost of memory space. Take the example below:
import sys
a_list = ['Maina', 'Football', 1, 3.142, True]
a_tuple = ('Chebet', 'Tennis', 2, 13.31, False)
print('The list size:', sys.getsizeof(a_list), 'bytes')
print('The tuple size:', sys.getsizeof(a_tuple), 'bytes')
Outputs
The list size: 104 bytes
The tuple size: 80 bytes
This difference becomes more apparent when working with big data. Immutability makes notable optimization as well as making processing much faster, which is efficient when working with millions of sequence objects.
Thus far, can you explain when to use a list over a tuple and vice versa? And more importantly, for the people at the back, are tuples mutable or immutable?