P1
/** * Tool widget to trigger the UI popup for Digital Identity Verification. */ com.cibc.go.myclient.view.form.widget.DigitalIdentityVerificationTool = (function($, superClass, global, utilities, DigitalIdentityVerificationView) { utilities.assertArrayElementsNotNull(arguments); /** * Constructor for DigitalIdentityVerificationTool. * @param {com.cibc.go.myclient.view.form.widget.WidgetFactory} widgetFactory The factory instance. * @param {com.cibc.go.myclient.view.form.widget.Container|jQuery} parent The parent container. * @param {Object} settings widget configuration settings. * @constructor * @extends com.cibc.go.myclient.view.form.widget.DataPicker */ var DivTool = function(widgetFactory, parent, settings) { var self = this; var newSettings = $.extend({ trigger: settings.trigger ? settings.trigger : "button", dialog: self.showDialog }, settings); superClass.call(self, widgetFactory, parent, newSettings); self.widgetFactory = widgetFactory; self.settings = newSettings; }; utilities.inherits(DivTool, superClass); /** * Shows the Digital Identity Verification dialog * @returns {DivTool} this instance for chaining */ DivTool.prototype.showDialog = function() { var self = this; var dialogId = 'DigitalIdentityVerificationDialog'; // Check if dialog already exists if ($('#' + dialogId).length > 0) { return self; } var title = global.Messages.get('DIV.Dialog.Title', 'Digital Identity Verification'); var $dialog = $(""); $dialog.dialog({ modal: true, width: $(window).width() * 0.7, minHeight: 250, resizable: false, open: function(event, ui) { $(this).html("Loading..."); // simple placeholder // Create the view and render it var view = new DigitalIdentityVerificationView({}); view.renderIn($(this)); $(this).closest('.ui-dialog').addClass('div-dialog-wrapper'); }, close: function(event, ui) { $(this).dialog("destroy"); $(this).remove(); } }); return self; }; /** * Overridden method from superclass - shows the editor * @param {Event} event The triggering event * @returns {DivTool} this instance for chaining */ DivTool.prototype.showEditor = function(event) { this.showDialog(); return this; }; /** * Overridden method from superclass - handles click on editor * @param {Event} event The click event * @returns {DivTool} this instance for chaining */ DivTool.prototype.clickEditor = function(event) { this.showDialog(); return this; }; return DivTool; })(jQuery, com.cibc.go.myclient.view.form.widget.DataPicker, com.cibc.go.myclient.global, com.cibc.go.Utilities, com.cibc.go.myclient.view.DigitalIdentityVerificationView); /** * View component responsible for rendering the content and handling interactions * within the Digital Identity Verification dialog. */ com.cibc.go.myclient.view.DigitalIdentityVerificationView = (function($, global, utilities) { utilities.assertArrayElementsNotNull(arguments); var soyTemplate = com.cibc.go.myclient.soy.view.DigitalIdentityVerificationView.main; /** * Constructor for the Digital Identity Verification View * @param {Object} settings Configuration settings * @constructor */ var DigitalIdentityVerificationView = function(settings) { var self = this; self.settings = $.extend({}, settings); self.clientModel = global.CurrentPage.client; self.errorMessages = []; }; /** * Renders the view into the specified container * @param {jQuery} container The container to render into * @returns {DigitalIdentityVerificationView} this instance for chaining */ DigitalIdentityVerificationView.prototype.renderIn = function(container) { var self = this; var clientDataForSoy = null; self.errorMessages = []; // Validate client model if (!self.clientModel) { self.errorMessages.push(global.Messages.get('DIV.Error.NoClientData', 'Client data is not available.')); container.html(soyTemplate({ messages: global.Messages, errorMessages: self.errorMessages })); } else { // Prepare client data for the Soy template clientDataForSoy = { iacode: self.clientModel.get('iacode'), firstName: self.clientModel.get('firstName'), lastName: self.clientModel.get('lastName'), dateOfBirth: self.clientModel.get('dob'), language: self.cl
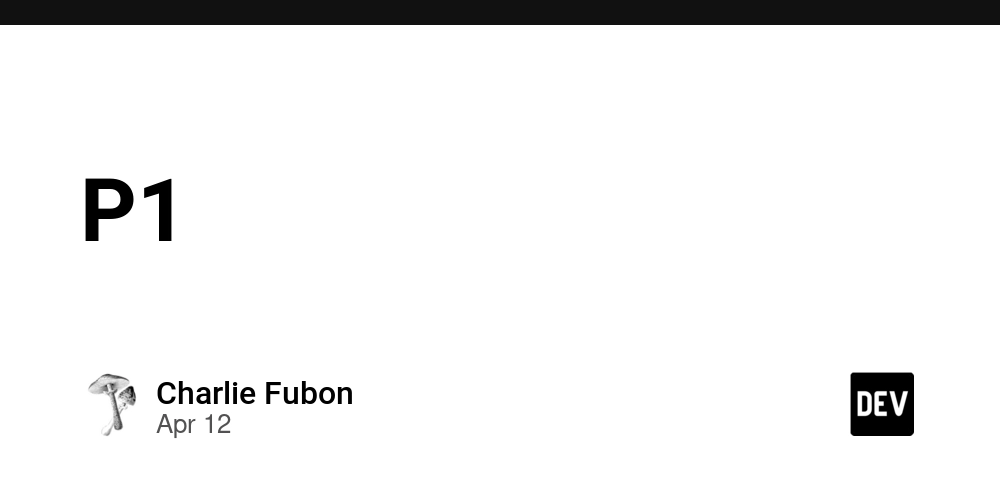
/**
* Tool widget to trigger the UI popup for Digital Identity Verification.
*/
com.cibc.go.myclient.view.form.widget.DigitalIdentityVerificationTool = (function($, superClass, global, utilities, DigitalIdentityVerificationView) {
utilities.assertArrayElementsNotNull(arguments);
/**
* Constructor for DigitalIdentityVerificationTool.
* @param {com.cibc.go.myclient.view.form.widget.WidgetFactory} widgetFactory The factory instance.
* @param {com.cibc.go.myclient.view.form.widget.Container|jQuery} parent The parent container.
* @param {Object} settings widget configuration settings.
* @constructor
* @extends com.cibc.go.myclient.view.form.widget.DataPicker
*/
var DivTool = function(widgetFactory, parent, settings) {
var self = this;
var newSettings = $.extend({
trigger: settings.trigger ? settings.trigger : "button",
dialog: self.showDialog
}, settings);
superClass.call(self, widgetFactory, parent, newSettings);
self.widgetFactory = widgetFactory;
self.settings = newSettings;
};
utilities.inherits(DivTool, superClass);
/**
* Shows the Digital Identity Verification dialog
* @returns {DivTool} this instance for chaining
*/
DivTool.prototype.showDialog = function() {
var self = this;
var dialogId = 'DigitalIdentityVerificationDialog';
// Check if dialog already exists
if ($('#' + dialogId).length > 0) {
return self;
}
var title = global.Messages.get('DIV.Dialog.Title', 'Digital Identity Verification');
var $dialog = $("");
$dialog.dialog({
modal: true,
width: $(window).width() * 0.7,
minHeight: 250,
resizable: false,
open: function(event, ui) {
$(this).html("Loading..."); // simple placeholder
// Create the view and render it
var view = new DigitalIdentityVerificationView({});
view.renderIn($(this));
$(this).closest('.ui-dialog').addClass('div-dialog-wrapper');
},
close: function(event, ui) {
$(this).dialog("destroy");
$(this).remove();
}
});
return self;
};
/**
* Overridden method from superclass - shows the editor
* @param {Event} event The triggering event
* @returns {DivTool} this instance for chaining
*/
DivTool.prototype.showEditor = function(event) {
this.showDialog();
return this;
};
/**
* Overridden method from superclass - handles click on editor
* @param {Event} event The click event
* @returns {DivTool} this instance for chaining
*/
DivTool.prototype.clickEditor = function(event) {
this.showDialog();
return this;
};
return DivTool;
})(jQuery,
com.cibc.go.myclient.view.form.widget.DataPicker,
com.cibc.go.myclient.global,
com.cibc.go.Utilities,
com.cibc.go.myclient.view.DigitalIdentityVerificationView);
/**
* View component responsible for rendering the content and handling interactions
* within the Digital Identity Verification dialog.
*/
com.cibc.go.myclient.view.DigitalIdentityVerificationView = (function($, global, utilities) {
utilities.assertArrayElementsNotNull(arguments);
var soyTemplate = com.cibc.go.myclient.soy.view.DigitalIdentityVerificationView.main;
/**
* Constructor for the Digital Identity Verification View
* @param {Object} settings Configuration settings
* @constructor
*/
var DigitalIdentityVerificationView = function(settings) {
var self = this;
self.settings = $.extend({}, settings);
self.clientModel = global.CurrentPage.client;
self.errorMessages = [];
};
/**
* Renders the view into the specified container
* @param {jQuery} container The container to render into
* @returns {DigitalIdentityVerificationView} this instance for chaining
*/
DigitalIdentityVerificationView.prototype.renderIn = function(container) {
var self = this;
var clientDataForSoy = null;
self.errorMessages = [];
// Validate client model
if (!self.clientModel) {
self.errorMessages.push(global.Messages.get('DIV.Error.NoClientData', 'Client data is not available.'));
container.html(soyTemplate({
messages: global.Messages,
errorMessages: self.errorMessages
}));
} else {
// Prepare client data for the Soy template
clientDataForSoy = {
iacode: self.clientModel.get('iacode'),
firstName: self.clientModel.get('firstName'),
lastName: self.clientModel.get('lastName'),
dateOfBirth: self.clientModel.get('dob'),
language: self.clientModel.get('preferredLanguage'),
emails: self.clientModel.get('emails')
};
// Check if emails are available
if (!clientDataForSoy.emails || clientDataForSoy.emails.length === 0) {
self.errorMessages.push(global.Messages.get('DIV.No.Email.Available', 'No email addresses are available.'));
}
// Render the template with client data
container.html(soyTemplate({
messages: global.Messages,
clientData: clientDataForSoy,
errorMessages: self.errorMessages
}));
// Setup UI event handlers
self.setupUIDetails(container, self);
}
return self;
};
/**
* Sets up UI event handlers
* @param {jQuery} container The container with the rendered UI
* @param {DigitalIdentityVerificationView} self Reference to this instance
*/
DigitalIdentityVerificationView.prototype.setupUIDetails = function(container, self) {
var dialogId = '#DigitalIdentityVerificationDialog';
// Setup cancel button
container.find('#div-cancel-button').on('click', function(e) {
e.preventDefault();
$(dialogId).dialog('close');
});
// Setup initiate button
container.find('#div-initiate-button').on('click', function(e) {
e.preventDefault();
var $button = $(this);
var selectedEmail = null;
self.errorMessages = [];
// Disable button during processing
$button.prop('disabled', true);
// Get selected email
var $emailRadios = container.find('input[name="divEmailSelection"]');
if ($emailRadios.length > 1) { // Multiple emails presented as radios
var $checkedRadio = $emailRadios.filter(':checked');
if ($checkedRadio.length === 0) {
self.errorMessages.push(global.Messages.get('DIV.Error.EmailRequired', 'Please select an email address.'));
} else {
selectedEmail = $checkedRadio.val();
}
} else if ($emailRadios.length === 1) {
// Single email (hidden input)
selectedEmail = $emailRadios.val();
}
// Validate email selection
if (!selectedEmail && ($emailRadios.length > 0 ||
(self.clientModel &&
self.clientModel.get('emails') &&
self.clientModel.get('emails').length > 0))) {
if (self.errorMessages.length === 0) {
self.errorMessages.push(global.Messages.get('DIV.Error.EmailRequired', 'Please select an email address.'));
}
}
// Display errors or proceed
if (self.errorMessages.length > 0) {
self.renderIn(container);
container.find('#div-initiate-button').prop('disabled', false);
return;
}
// Prepare data for API call
var initiationData = {
clientId: self.clientModel.get('clientId'),
sin: self.clientModel.get('sin'),
firstName: self.clientModel.get('firstName'),
lastName: self.clientModel.get('lastName'),
selectedEmail: selectedEmail,
iacode: self.clientModel.get('iacode')
};
// Make API call to initiate DIV process
com.cibc.go.Utilities.post(
global.urls.get('div.initiation.url'), // URL for DIV initiation API
initiationData,
function(response) { // Success callback
// Show success message if needed
$(dialogId).dialog('close');
},
function(error) { // Error callback
// Handle error
self.errorMessages.push(global.Messages.get('DIV.Error.ApiError', 'An error occurred during verification initiation.'));
self.renderIn(container); // Re-render to show API error
container.find('#div-initiate-button').prop('disabled', false); // Re-enable button
}
);
});
};
// Return the constructor function
return DigitalIdentityVerificationView;
})(jQuery, com.cibc.go.myclient.global, com.cibc.go.Utilities); // Pass dependencies
{namespace com.cibc.go.myclient.soy.view.DigitalIdentityVerificationView}
/**
* Main template for the Digital Identity Verification popup content.
* @param messages The I18N messages map.
* @param clientData Map containing client details. Expected keys:
* iacode, firstName, lastName, dateOfBirth, language, emails (list)
* @param errorMessages List of error strings to display.
*/
{template .main}
{if $errorMessages and length($errorMessages) > 0}
{foreach $errorMsg in $errorMessages}
{$errorMsg}
{/foreach}
{/if}
{if $clientData}
{$messages['client.details.name'] ?: 'Name'}:
{$clientData.firstName} {$clientData.lastName}
{$messages['client.details.dob'] ?: 'Date of Birth'}:
{$clientData.dateOfBirth}
{$messages['client.details.language'] ?: 'Language'}:
{$clientData.language}
{$messages['client.details.iacode'] ?: 'IA Code'}:
{$clientData.iacode}
{* -- Email Selection -- *}
{if $clientData.emails and length($clientData.emails) > 1}
{$emailValue}
{else}
{/if}
{else}
{elseif $clientData.emails and length($clientData.emails) == 1}
{let $emailValue: $clientData.emails[0].address ? $clientData.emails[0].address : $clientData.emails[0] /}
{$messages['div.loading.error'] ?: 'Could not load client details.'}
{/if}
{/template}