Key Software Development Principles and Acronyms
Modern programming is full of principles and acronyms that help developers write maintainable, readable, and robust code. Here’s a quick guide to the most popular and widely used ones: 1. KISS (Keep It Simple, Stupid) Favor simplicity: solutions should be as simple as possible, avoiding unnecessary complexity. Simplicity makes code easier to maintain, test, and understand. 2. DRY (Don’t Repeat Yourself) Avoid duplication: don’t repeat information or logic. Duplicated code is harder to maintain and more error-prone. 3. YAGNI (You Aren’t Gonna Need It) Don’t build features until you actually need them. Avoid implementing functionality “just in case.” 4. BDUF (Big Design Up Front) Design everything before you start coding. This principle is often contrasted with agile, iterative approaches. 5. SOLID A set of five core object-oriented design principles: Single Responsibility Principle (SRP) – a class should have only one reason to change Open/Closed Principle (OCP) – open for extension, closed for modification Liskov Substitution Principle (LSP) – subclasses should be substitutable for their base classes Interface Segregation Principle (ISP) – many client-specific interfaces are better than one general-purpose interface Dependency Inversion Principle (DIP) – depend on abstractions, not concretions 6. APO (Avoid Premature Optimization) Don’t optimize code before it’s necessary. Premature optimization can make code more complicated without proven need. 7. GRASP (General Responsibility Assignment Software Patterns) A set of patterns for assigning responsibilities to classes and objects in OOP. 8. PSR (PHP Standard Recommendations) A set of coding standards for PHP (if you’re working with PHP). 9. Law of Demeter Principle of least knowledge: “don’t talk to strangers.” Objects should only interact with their immediate collaborators. 10. Occam’s Razor The simplest solution is usually the correct one. 11. Principle of Least Astonishment (POLA) Systems should behave in a way that least surprises users. 12. Convention over Configuration Favor sensible defaults and conventions to minimize configuration and boilerplate code. 13. Composition over Inheritance Prefer composing objects over class inheritance to increase flexibility and code reuse. 14. Single Source of Truth (SSOT) Data should have a single, unambiguous source. Conclusion There are many principles and acronyms in software development that help create maintainable and high-quality code. In addition to KISS, DRY, YAGNI, BDUF, SOLID, and APO, you’ll often encounter GRASP, PSR, Law of Demeter, Occam’s Razor, POLA, Convention over Configuration, Composition over Inheritance, and more. Mastering and applying these principles is a hallmark of a professional developer.
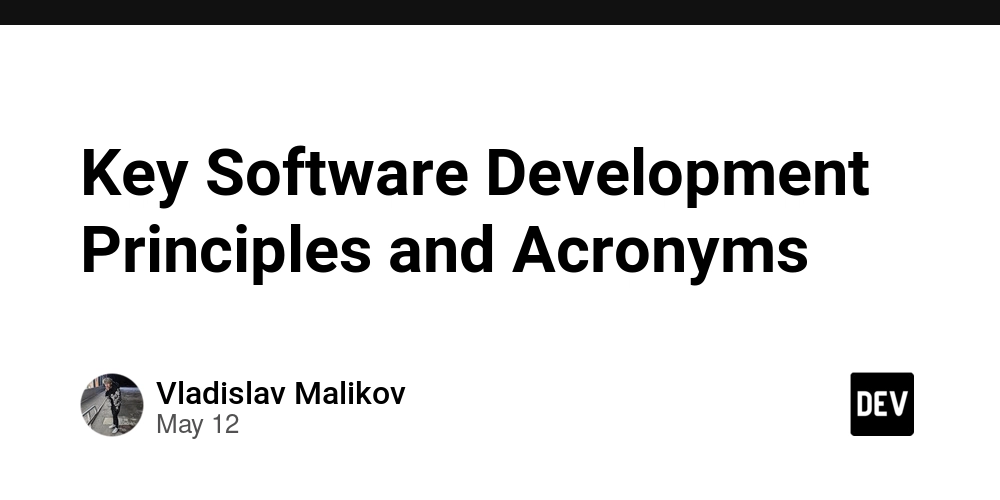
Modern programming is full of principles and acronyms that help developers write maintainable, readable, and robust code. Here’s a quick guide to the most popular and widely used ones:
1. KISS (Keep It Simple, Stupid)
Favor simplicity: solutions should be as simple as possible, avoiding unnecessary complexity. Simplicity makes code easier to maintain, test, and understand.
2. DRY (Don’t Repeat Yourself)
Avoid duplication: don’t repeat information or logic. Duplicated code is harder to maintain and more error-prone.
3. YAGNI (You Aren’t Gonna Need It)
Don’t build features until you actually need them. Avoid implementing functionality “just in case.”
4. BDUF (Big Design Up Front)
Design everything before you start coding. This principle is often contrasted with agile, iterative approaches.
5. SOLID
A set of five core object-oriented design principles:
- Single Responsibility Principle (SRP) – a class should have only one reason to change
- Open/Closed Principle (OCP) – open for extension, closed for modification
- Liskov Substitution Principle (LSP) – subclasses should be substitutable for their base classes
- Interface Segregation Principle (ISP) – many client-specific interfaces are better than one general-purpose interface
- Dependency Inversion Principle (DIP) – depend on abstractions, not concretions
6. APO (Avoid Premature Optimization)
Don’t optimize code before it’s necessary. Premature optimization can make code more complicated without proven need.
7. GRASP (General Responsibility Assignment Software Patterns)
A set of patterns for assigning responsibilities to classes and objects in OOP.
8. PSR (PHP Standard Recommendations)
A set of coding standards for PHP (if you’re working with PHP).
9. Law of Demeter
Principle of least knowledge: “don’t talk to strangers.” Objects should only interact with their immediate collaborators.
10. Occam’s Razor
The simplest solution is usually the correct one.
11. Principle of Least Astonishment (POLA)
Systems should behave in a way that least surprises users.
12. Convention over Configuration
Favor sensible defaults and conventions to minimize configuration and boilerplate code.
13. Composition over Inheritance
Prefer composing objects over class inheritance to increase flexibility and code reuse.
14. Single Source of Truth (SSOT)
Data should have a single, unambiguous source.
Conclusion
There are many principles and acronyms in software development that help create maintainable and high-quality code. In addition to KISS, DRY, YAGNI, BDUF, SOLID, and APO, you’ll often encounter GRASP, PSR, Law of Demeter, Occam’s Razor, POLA, Convention over Configuration, Composition over Inheritance, and more. Mastering and applying these principles is a hallmark of a professional developer.