How to Fix Text Disappearing in C Backspace Functionality
Introduction If you're writing a simple text editor in C and encountering issues with your text disappearing when using the Backspace key at the beginning of a line, you're not alone. Many newcomers face unexpected behavior in their text manipulation functions. This article will dive into why this happens, particularly focusing on how Backspace interacts with your cursor position and text storage. Understanding the Issue In your C text editor code, when you press the Backspace key (ASCII 8), you're performing different operations depending on the cursor's current position. If the cursor is at the beginning of a line (cursor_x = 0), the function tries to merge the current line with the preceding line using strcat. Though this is an intended feature, it inadvertently leads to the upper line becoming invisible -- because the necessary conditions for displaying it are not met properly afterward. The Existing Code Logic Let's break down the section of code responsible for handling the Backspace key: else if (ch == 8) { int len = strlen(text[cursor_y]); if (cursor_x > 0) { // Shift characters left for (int i = cursor_x - 1; i < len; i++) { text[cursor_y][i] = text[cursor_y][i + 1]; } cursor_x--; } else { // Merge lines if (cursor_y != 0) { strcat(text[cursor_y - 1], text[cursor_y]); text[cursor_y][0] = '\0'; line_count--; cursor_x = strlen(text[cursor_y - 1]); cursor_y--; } } } Here, if cursor_x is zero, you're attempting to concatenate the current line to the line above. However, there's a missing piece to ensure that after this operation, the lines are redrawn correctly, resulting in the upper line sometimes disappearing. A Step-by-Step Solution To fix this issue, let’s introduce additional logic to handle cases where you delete characters at the start of a line more gracefully. Modifying the Backspace Block We can prevent the current line from disappearing by letting it resize properly and adjusting how we handle cursor movement. Here’s the revised code for the Backspace section: else if (ch == 8) { int len = strlen(text[cursor_y]); if (cursor_x > 0) { for (int i = cursor_x - 1; i < len; i++) { text[cursor_y][i] = text[cursor_y][i + 1]; } cursor_x--; } else { if (cursor_y > 0) { // Append the current line to the previous one, // ensuring we avoid data loss int prev_len = strlen(text[cursor_y - 1]); int curr_len = strlen(text[cursor_y]); // Avoiding overflow if (prev_len + curr_len < MAX_LINE_LEN - 1) { strcat(text[cursor_y - 1], text[cursor_y]); cursor_y--; cursor_x = strlen(text[cursor_y - 1]); } // Clear the current line so text does not appear twice text[cursor_y][0] = '\0'; line_count--; } } } Explanation of the Changes Boundary Check: Before concatenating lines, we check if the length of the previous line and current line together does not exceed the maximum line length. This ensures no data is lost. Cursor Positioning: After joining the lines correctly, we reset the cursor to the end of the new line, providing a seamless editing experience. Finally, we clear the current line to avoid duplication. Overall Robustness: These changes provide a more user-friendly experience, maintaining data integrity and preventing unexpected behavior. Testing the Solution Once you've integrated the changes into your text editor, give it some test runs: Navigate to the beginning of different lines and press Backspace multiple times to see if the text behaves as expected. Try deleting characters in the middle and end of lines, ensuring that all functionalities work correctly. This will confirm the issue has been addressed and enhance the usability of your text editor. Conclusion Programming in C can sometimes lead to troublesome bugs that may cause text to disappear or behave erratically. By understanding how cursor locations affect your text editing functions and implementing robust error handling, you can create a more reliable text editor. Testing your modifications thoroughly is key to achieving a stable and functional application. Frequently Asked Questions Q: What causes text to disappear when I press Backspace? A: It's typically due to mishandling line concatenation in your text buffer, particularly when the cursor is positioned at the start of a line. Q: How can I improve my text editing software? A: Focus on input handling, clear screen logic, and memory management to ensure stability and usability. Q: Are there better libraries for text editing in C? A: You might want to explore libraries like ncurses for more robust terminal handling capabilities, offering pre-built functionalities for text input and dis
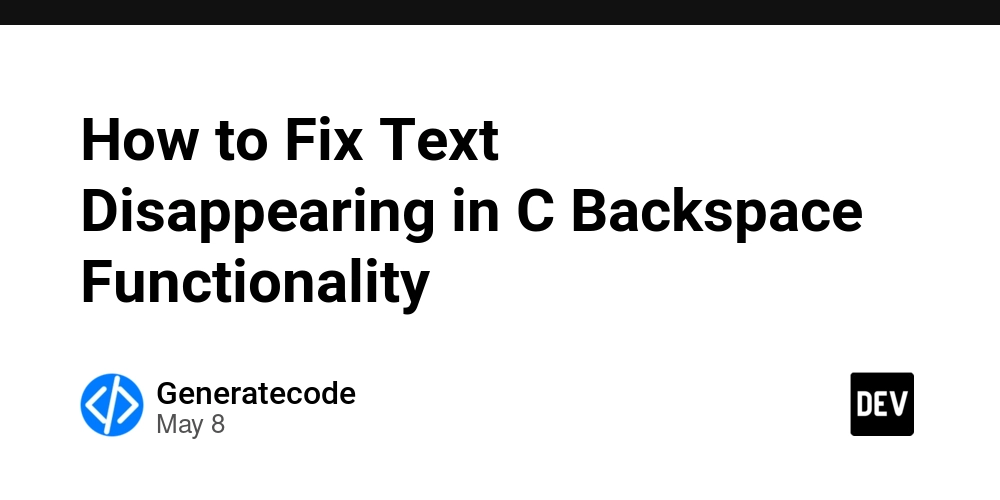
Introduction
If you're writing a simple text editor in C and encountering issues with your text disappearing when using the Backspace key at the beginning of a line, you're not alone. Many newcomers face unexpected behavior in their text manipulation functions. This article will dive into why this happens, particularly focusing on how Backspace interacts with your cursor position and text storage.
Understanding the Issue
In your C text editor code, when you press the Backspace key (ASCII 8), you're performing different operations depending on the cursor's current position. If the cursor is at the beginning of a line (cursor_x = 0
), the function tries to merge the current line with the preceding line using strcat
. Though this is an intended feature, it inadvertently leads to the upper line becoming invisible -- because the necessary conditions for displaying it are not met properly afterward.
The Existing Code Logic
Let's break down the section of code responsible for handling the Backspace key:
else if (ch == 8) {
int len = strlen(text[cursor_y]);
if (cursor_x > 0) {
// Shift characters left
for (int i = cursor_x - 1; i < len; i++) {
text[cursor_y][i] = text[cursor_y][i + 1];
}
cursor_x--;
} else {
// Merge lines
if (cursor_y != 0) {
strcat(text[cursor_y - 1], text[cursor_y]);
text[cursor_y][0] = '\0';
line_count--;
cursor_x = strlen(text[cursor_y - 1]);
cursor_y--;
}
}
}
Here, if cursor_x
is zero, you're attempting to concatenate the current line to the line above. However, there's a missing piece to ensure that after this operation, the lines are redrawn correctly, resulting in the upper line sometimes disappearing.
A Step-by-Step Solution
To fix this issue, let’s introduce additional logic to handle cases where you delete characters at the start of a line more gracefully.
Modifying the Backspace Block
We can prevent the current line from disappearing by letting it resize properly and adjusting how we handle cursor movement. Here’s the revised code for the Backspace section:
else if (ch == 8) {
int len = strlen(text[cursor_y]);
if (cursor_x > 0) {
for (int i = cursor_x - 1; i < len; i++) {
text[cursor_y][i] = text[cursor_y][i + 1];
}
cursor_x--;
} else {
if (cursor_y > 0) {
// Append the current line to the previous one,
// ensuring we avoid data loss
int prev_len = strlen(text[cursor_y - 1]);
int curr_len = strlen(text[cursor_y]);
// Avoiding overflow
if (prev_len + curr_len < MAX_LINE_LEN - 1) {
strcat(text[cursor_y - 1], text[cursor_y]);
cursor_y--;
cursor_x = strlen(text[cursor_y - 1]);
}
// Clear the current line so text does not appear twice
text[cursor_y][0] = '\0';
line_count--;
}
}
}
Explanation of the Changes
- Boundary Check: Before concatenating lines, we check if the length of the previous line and current line together does not exceed the maximum line length. This ensures no data is lost.
- Cursor Positioning: After joining the lines correctly, we reset the cursor to the end of the new line, providing a seamless editing experience. Finally, we clear the current line to avoid duplication.
- Overall Robustness: These changes provide a more user-friendly experience, maintaining data integrity and preventing unexpected behavior.
Testing the Solution
Once you've integrated the changes into your text editor, give it some test runs:
- Navigate to the beginning of different lines and press Backspace multiple times to see if the text behaves as expected.
- Try deleting characters in the middle and end of lines, ensuring that all functionalities work correctly.
- This will confirm the issue has been addressed and enhance the usability of your text editor.
Conclusion
Programming in C can sometimes lead to troublesome bugs that may cause text to disappear or behave erratically. By understanding how cursor locations affect your text editing functions and implementing robust error handling, you can create a more reliable text editor. Testing your modifications thoroughly is key to achieving a stable and functional application.
Frequently Asked Questions
Q: What causes text to disappear when I press Backspace?
A: It's typically due to mishandling line concatenation in your text buffer, particularly when the cursor is positioned at the start of a line.
Q: How can I improve my text editing software?
A: Focus on input handling, clear screen logic, and memory management to ensure stability and usability.
Q: Are there better libraries for text editing in C?
A: You might want to explore libraries like ncurses for more robust terminal handling capabilities, offering pre-built functionalities for text input and display.