Designing for Scalability
Designing for Scalability Introduction: Scalability, the ability of a system to handle a growing amount of work, is crucial for software success. Poorly designed systems struggle under increased load, leading to performance bottlenecks and user dissatisfaction. Planning for scalability from the outset is vital, avoiding costly redesigns later. Prerequisites: Before embarking on scalable design, several prerequisites must be met. These include a thorough understanding of expected growth patterns (user base, data volume, transaction rates), selection of appropriate technologies (databases, servers, cloud platforms), and a well-defined architecture. Advantages: Scalable systems offer numerous advantages. They handle increased demand seamlessly, ensuring consistent performance and user experience. This leads to improved reliability, reduced downtime, and enhanced capacity for future growth. Scalability also facilitates easier deployment of new features and faster response to market changes. Disadvantages: While scalability offers significant benefits, challenges exist. Implementing scalable solutions often requires higher upfront investment in infrastructure and development. Managing a complex, distributed system can be more difficult than a monolithic one, demanding specialized skills and robust monitoring. Increased complexity can also lead to higher operational costs. Features of Scalable Systems: Scalable systems typically incorporate several key features: Modular Design: Breaking down the system into independent, reusable components allows for independent scaling. Horizontal Scaling: Adding more servers to handle increased load (e.g., using load balancers). Asynchronous Processing: Handling tasks in the background prevents blocking operations. Example using Python's asyncio: import asyncio async def my_task(): # Simulate a long-running task await asyncio.sleep(1) return "Task complete" async def main(): task = asyncio.create_task(my_task()) # ... other code can execute concurrently ... result = await task print(result) asyncio.run(main()) Caching: Storing frequently accessed data closer to the user reduces database load. Conclusion: Designing for scalability is not a one-size-fits-all solution; the optimal approach depends on specific needs and constraints. However, careful planning, appropriate technology choices, and incorporation of scalable design patterns are crucial for building systems capable of handling future growth and ensuring long-term success.
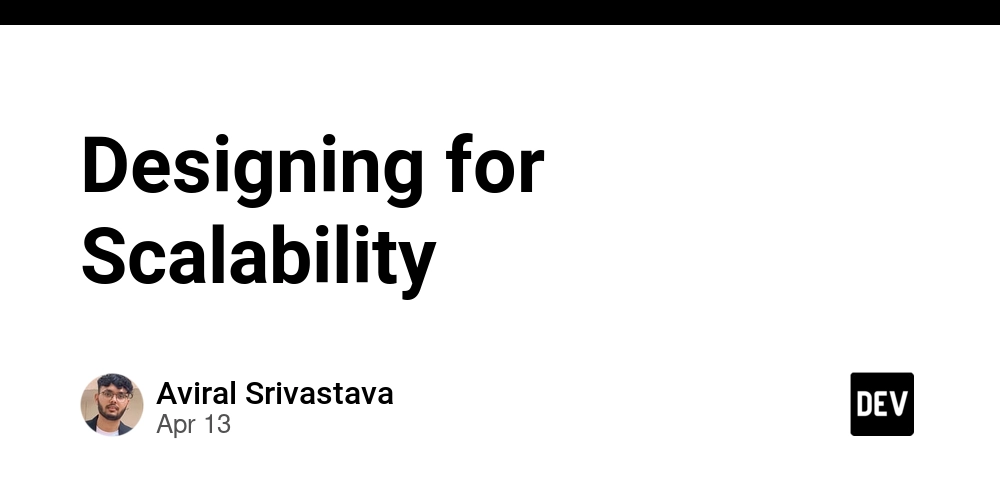
Designing for Scalability
Introduction:
Scalability, the ability of a system to handle a growing amount of work, is crucial for software success. Poorly designed systems struggle under increased load, leading to performance bottlenecks and user dissatisfaction. Planning for scalability from the outset is vital, avoiding costly redesigns later.
Prerequisites:
Before embarking on scalable design, several prerequisites must be met. These include a thorough understanding of expected growth patterns (user base, data volume, transaction rates), selection of appropriate technologies (databases, servers, cloud platforms), and a well-defined architecture.
Advantages:
Scalable systems offer numerous advantages. They handle increased demand seamlessly, ensuring consistent performance and user experience. This leads to improved reliability, reduced downtime, and enhanced capacity for future growth. Scalability also facilitates easier deployment of new features and faster response to market changes.
Disadvantages:
While scalability offers significant benefits, challenges exist. Implementing scalable solutions often requires higher upfront investment in infrastructure and development. Managing a complex, distributed system can be more difficult than a monolithic one, demanding specialized skills and robust monitoring. Increased complexity can also lead to higher operational costs.
Features of Scalable Systems:
Scalable systems typically incorporate several key features:
- Modular Design: Breaking down the system into independent, reusable components allows for independent scaling.
- Horizontal Scaling: Adding more servers to handle increased load (e.g., using load balancers).
-
Asynchronous Processing: Handling tasks in the background prevents blocking operations. Example using Python's
asyncio
:
import asyncio
async def my_task():
# Simulate a long-running task
await asyncio.sleep(1)
return "Task complete"
async def main():
task = asyncio.create_task(my_task())
# ... other code can execute concurrently ...
result = await task
print(result)
asyncio.run(main())
- Caching: Storing frequently accessed data closer to the user reduces database load.
Conclusion:
Designing for scalability is not a one-size-fits-all solution; the optimal approach depends on specific needs and constraints. However, careful planning, appropriate technology choices, and incorporation of scalable design patterns are crucial for building systems capable of handling future growth and ensuring long-term success.