Challenge: Deploy AWS Infrastructure with Pulumi (Python)
Challenge: Deploy AWS Infrastructure with Pulumi (Python) 1️⃣ Simple AWS S3 Bucket Deployment python Copy import pulumi from pulumi_aws import s3 Create a private S3 bucket with tags bucket = s3.Bucket( "my-pulumi-bucket", acl="private", tags={ "Name": "PulumiDemoBucket", "Environment": "Dev" } ) Enable bucket versioning s3.BucketVersioning( "bucket-versioning", bucket=bucket.id, versioning_configuration={ "status": "Enabled" } ) Export the bucket name and ARN pulumi.export("bucket_name", bucket.id) pulumi.export("bucket_arn", bucket.arn) Deployment Commands: bash Copy Initialize new Pulumi project (AWS Python template) pulumi new aws-python -y Deploy infrastructure pulumi up View outputs pulumi stack output bucket_name Destroy resources when done pulumi destroy -y 2️⃣ Multi-Cloud Example (AWS + Azure) python Copy import pulumi from pulumi_aws import s3 from pulumi_azure_native import storage, resources AWS S3 Bucket aws_bucket = s3.Bucket( "aws-demo-bucket", acl="private" ) Azure Resource Group resource_group = resources.ResourceGroup( "azure-demo-rg" ) Azure Storage Account storage_account = storage.StorageAccount( "azuredemostorage", resource_group_name=resource_group.name, sku=storage.SkuArgs( name="Standard_LRS" ), kind="StorageV2" ) Export resources pulumi.export("aws_bucket", aws_bucket.id) pulumi.export("azure_storage", storage_account.name) Prerequisites: bash Copy Install required providers pip install pulumi_aws pulumi_azure_native Configure both cloud providers aws configure az login 3️⃣ Advanced Example: Static Website on AWS (S3 + CloudFront + Route53) python Copy import pulumi from pulumi_aws import s3, cloudfront, route53 S3 Bucket for website content bucket = s3.Bucket( "website-bucket", website=s3.BucketWebsiteArgs( index_document="index.html" ), acl="public-read" ) Upload sample index.html s3.BucketObject( "index.html", bucket=bucket.id, content=" Hello Pulumi!", content_type="text/html", acl="public-read" ) CloudFront Distribution distribution = cloudfront.Distribution( "website-distribution", origins=[cloudfront.DistributionOriginArgs( domain_name=bucket.bucket_regional_domain_name, origin_id=bucket.arn )], enabled=True, default_root_object="index.html", default_cache_behavior=cloudfront.DistributionDefaultCacheBehaviorArgs( allowed_methods=["GET", "HEAD"], cached_methods=["GET", "HEAD"], target_origin_id=bucket.arn, viewer_protocol_policy="redirect-to-https" ), restrictions=cloudfront.DistributionRestrictionsArgs( geo_restriction=cloudfront.DistributionRestrictionsGeoRestrictionArgs( restriction_type="none" ) ), viewer_certificate=cloudfront.DistributionViewerCertificateArgs( cloudfront_default_certificate=True ) ) Export the website URL pulumi.export("website_url", distribution.domain_name) Deployment Steps: Save as main.py Run pulumi up Access website at the exported URL
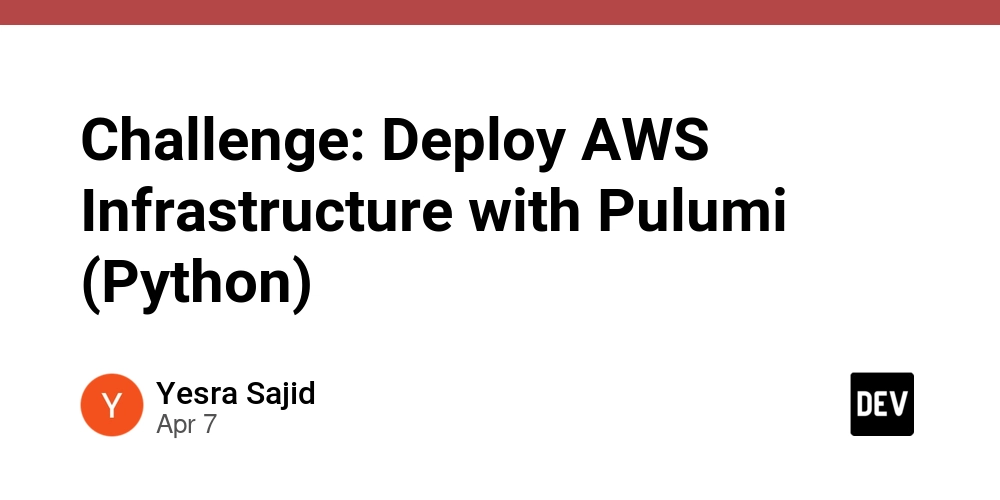
Challenge: Deploy AWS Infrastructure with Pulumi (Python)
1️⃣ Simple AWS S3 Bucket Deployment
python
Copy
import pulumi
from pulumi_aws import s3
Create a private S3 bucket with tags
bucket = s3.Bucket(
"my-pulumi-bucket",
acl="private",
tags={
"Name": "PulumiDemoBucket",
"Environment": "Dev"
}
)
Enable bucket versioning
s3.BucketVersioning(
"bucket-versioning",
bucket=bucket.id,
versioning_configuration={
"status": "Enabled"
}
)
Export the bucket name and ARN
pulumi.export("bucket_name", bucket.id)
pulumi.export("bucket_arn", bucket.arn)
Deployment Commands:
bash
Copy
Initialize new Pulumi project (AWS Python template)
pulumi new aws-python -y
Deploy infrastructure
pulumi up
View outputs
pulumi stack output bucket_name
Destroy resources when done
pulumi destroy -y
2️⃣ Multi-Cloud Example (AWS + Azure)
python
Copy
import pulumi
from pulumi_aws import s3
from pulumi_azure_native import storage, resources
AWS S3 Bucket
aws_bucket = s3.Bucket(
"aws-demo-bucket",
acl="private"
)
Azure Resource Group
resource_group = resources.ResourceGroup(
"azure-demo-rg"
)
Azure Storage Account
storage_account = storage.StorageAccount(
"azuredemostorage",
resource_group_name=resource_group.name,
sku=storage.SkuArgs(
name="Standard_LRS"
),
kind="StorageV2"
)
Export resources
pulumi.export("aws_bucket", aws_bucket.id)
pulumi.export("azure_storage", storage_account.name)
Prerequisites:
bash
Copy
Install required providers
pip install pulumi_aws pulumi_azure_native
Configure both cloud providers
aws configure
az login
3️⃣ Advanced Example: Static Website on AWS (S3 + CloudFront + Route53)
python
Copy
import pulumi
from pulumi_aws import s3, cloudfront, route53
S3 Bucket for website content
bucket = s3.Bucket(
"website-bucket",
website=s3.BucketWebsiteArgs(
index_document="index.html"
),
acl="public-read"
)
Upload sample index.html
s3.BucketObject(
"index.html",
bucket=bucket.id,
content="
Hello Pulumi!
",content_type="text/html",
acl="public-read"
)
CloudFront Distribution
distribution = cloudfront.Distribution(
"website-distribution",
origins=[cloudfront.DistributionOriginArgs(
domain_name=bucket.bucket_regional_domain_name,
origin_id=bucket.arn
)],
enabled=True,
default_root_object="index.html",
default_cache_behavior=cloudfront.DistributionDefaultCacheBehaviorArgs(
allowed_methods=["GET", "HEAD"],
cached_methods=["GET", "HEAD"],
target_origin_id=bucket.arn,
viewer_protocol_policy="redirect-to-https"
),
restrictions=cloudfront.DistributionRestrictionsArgs(
geo_restriction=cloudfront.DistributionRestrictionsGeoRestrictionArgs(
restriction_type="none"
)
),
viewer_certificate=cloudfront.DistributionViewerCertificateArgs(
cloudfront_default_certificate=True
)
)
Export the website URL
pulumi.export("website_url", distribution.domain_name)
Deployment Steps:
Save as main.py
Run pulumi up
Access website at the exported URL