Can Spring Boot Main Thread Spawn Multiple Child Threads?
Introduction Creating a Spring Boot project where the main thread spawns multiple threads, which in turn can spawn their own child threads, is indeed possible. This architecture can effectively leverage the multi-threading capabilities of Java, enabling applications to perform concurrent tasks efficiently. In this article, we'll explore how to implement such a scenario in Spring Boot, examine whether it’s a good practice for your application, and provide step-by-step implementation guidance. Understanding Multi-threading in Spring Boot Multi-threading allows a single application to run multiple threads simultaneously. In Java, the Thread class and the Runnable interface can be utilized to create and manage multiple threads. Spring Boot simplifies managing threads through the use of annotations and configuration classes, such as the @EnableAsync annotation you have included in your configuration class. Why Utilize Multi-threading? There are several advantages to using multi-threading in your applications: Improved Performance: Execute multiple tasks in parallel, which reduces latency. Better Resource Utilization: Utilize CPU resources more efficiently by running background tasks. Responsive Applications: Enhance user experience in web applications by offloading long-running tasks to background threads. However, it’s crucial to note that, while multi-threading can enhance performance, it also introduces complexity to your application. Improper handling can lead to issues such as race conditions, deadlocks, and increased difficulty in debugging. Is This a Good Practice? Leveraging multi-threading can be beneficial, but it’s best to assess your specific use case: If your application performs multiple independent tasks concurrently, using multi-threading is a good practice. If tasks are heavily inter-dependent or require a lot of shared resource access, you might want to reconsider, as this can lead to complications. If you decide multi-threading suits your needs, let’s dive into the implementation. Below, we'll implement spawning multiple threads from the main thread and subsequently creating child threads. Implementation Steps Step 1: Create the Spring Boot Application First, make sure you have a Spring Boot application set up. If you haven’t done so, create a simple Spring Boot project using Spring Initializr or your IDE. Step 2: Update the Async Configuration Class You already have an AsyncConfig class. Ensure it looks like this: @Configuration @EnableAsync public class AsyncConfig { @Bean(name = "taskExecutor") Executor taskExecutor() { ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor(); executor.setCorePoolSize(15); executor.setMaxPoolSize(40); executor.setQueueCapacity(100); executor.setThreadNamePrefix("AsyncThread-"); executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy()); executor.initialize(); return executor; } } This configuration allows Spring to manage thread execution using a thread pool. Step 3: Create a Service to Handle Multi-threading Next, let’s create a service that will be responsible for spawning threads. Here’s an example service class: import org.springframework.beans.factory.annotation.Autowired; import org.springframework.scheduling.annotation.Async; import org.springframework.stereotype.Service; import java.util.concurrent.Executor; @Service public class ThreadService { @Autowired private Executor taskExecutor; public void executeTasks() { for (int i = 0; i < 5; i++) { int finalI = i; taskExecutor.execute(() -> spawnChildThreads(finalI)); } } @Async public void spawnChildThreads(int parentId) { for (int i = 0; i < 3; i++) { int childId = i; System.out.println("Parent Thread ID: " + parentId + " spawning Child Thread ID: " + childId); taskExecutor.execute(() -> doWork(childId)); } } @Async public void doWork(int threadId) { try { Thread.sleep(1000); System.out.println("Work done by Child Thread ID: " + threadId); } catch (InterruptedException e) { Thread.currentThread().interrupt(); System.out.println("Thread interrupted: " + threadId); } } } In this code: The executeTasks() method spawns multiple threads (5 in this case), each of which can spawn 3 child threads. Each child thread performs a simple doWork() method which simulates work by sleeping for 1 second. Step 4: Triggering Your Threads You’ll need to invoke the executeTasks method to initiate the process. You can do this in your main application class or a controller: import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure
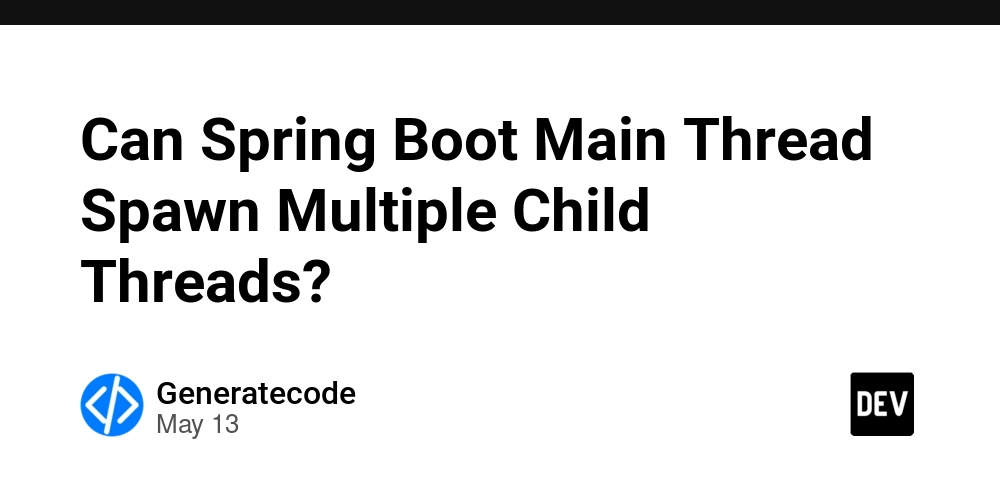
Introduction
Creating a Spring Boot project where the main thread spawns multiple threads, which in turn can spawn their own child threads, is indeed possible. This architecture can effectively leverage the multi-threading capabilities of Java, enabling applications to perform concurrent tasks efficiently.
In this article, we'll explore how to implement such a scenario in Spring Boot, examine whether it’s a good practice for your application, and provide step-by-step implementation guidance.
Understanding Multi-threading in Spring Boot
Multi-threading allows a single application to run multiple threads simultaneously. In Java, the Thread
class and the Runnable
interface can be utilized to create and manage multiple threads. Spring Boot simplifies managing threads through the use of annotations and configuration classes, such as the @EnableAsync
annotation you have included in your configuration class.
Why Utilize Multi-threading?
There are several advantages to using multi-threading in your applications:
- Improved Performance: Execute multiple tasks in parallel, which reduces latency.
- Better Resource Utilization: Utilize CPU resources more efficiently by running background tasks.
- Responsive Applications: Enhance user experience in web applications by offloading long-running tasks to background threads.
However, it’s crucial to note that, while multi-threading can enhance performance, it also introduces complexity to your application. Improper handling can lead to issues such as race conditions, deadlocks, and increased difficulty in debugging.
Is This a Good Practice?
Leveraging multi-threading can be beneficial, but it’s best to assess your specific use case:
- If your application performs multiple independent tasks concurrently, using multi-threading is a good practice.
- If tasks are heavily inter-dependent or require a lot of shared resource access, you might want to reconsider, as this can lead to complications.
If you decide multi-threading suits your needs, let’s dive into the implementation. Below, we'll implement spawning multiple threads from the main thread and subsequently creating child threads.
Implementation Steps
Step 1: Create the Spring Boot Application
First, make sure you have a Spring Boot application set up. If you haven’t done so, create a simple Spring Boot project using Spring Initializr or your IDE.
Step 2: Update the Async Configuration Class
You already have an AsyncConfig
class. Ensure it looks like this:
@Configuration
@EnableAsync
public class AsyncConfig {
@Bean(name = "taskExecutor")
Executor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(15);
executor.setMaxPoolSize(40);
executor.setQueueCapacity(100);
executor.setThreadNamePrefix("AsyncThread-");
executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());
executor.initialize();
return executor;
}
}
This configuration allows Spring to manage thread execution using a thread pool.
Step 3: Create a Service to Handle Multi-threading
Next, let’s create a service that will be responsible for spawning threads. Here’s an example service class:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import java.util.concurrent.Executor;
@Service
public class ThreadService {
@Autowired
private Executor taskExecutor;
public void executeTasks() {
for (int i = 0; i < 5; i++) {
int finalI = i;
taskExecutor.execute(() -> spawnChildThreads(finalI));
}
}
@Async
public void spawnChildThreads(int parentId) {
for (int i = 0; i < 3; i++) {
int childId = i;
System.out.println("Parent Thread ID: " + parentId + " spawning Child Thread ID: " + childId);
taskExecutor.execute(() -> doWork(childId));
}
}
@Async
public void doWork(int threadId) {
try {
Thread.sleep(1000);
System.out.println("Work done by Child Thread ID: " + threadId);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
System.out.println("Thread interrupted: " + threadId);
}
}
}
In this code:
- The
executeTasks()
method spawns multiple threads (5 in this case), each of which can spawn 3 child threads. - Each child thread performs a simple
doWork()
method which simulates work by sleeping for 1 second.
Step 4: Triggering Your Threads
You’ll need to invoke the executeTasks
method to initiate the process. You can do this in your main application class or a controller:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootMultiThreadApplication implements CommandLineRunner {
@Autowired
private ThreadService threadService;
public static void main(String[] args) {
SpringApplication.run(SpringBootMultiThreadApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
threadService.executeTasks();
}
}
This will run the multithreading example as soon as you start your application.
Frequently Asked Questions (FAQ)
1. Can I spawn unlimited threads in Spring Boot?
While you can spawn many threads, there's a limit based on your system resources. The configured thread pool size should balance performance and system limits.
2. How do I handle exceptions in child threads?
You can use a try-catch
block in your child thread methods to manage exceptions effectively.
3. Is Spring’s async support suitable for all use cases?
Not necessarily. If you have CPU-bound tasks, consider using more advanced parallel processing strategies instead of simple threading.
Conclusion
In conclusion, spawning multiple threads from a Spring Boot application is not only possible but can also be a practical approach to handling concurrent tasks. With careful management and the use of Spring’s built-in support for asynchronous processing, you can create highly efficient applications. Just ensure that you evaluate your specific use case to decide if this pattern fits well.