60 Interview question-answer of Angular 19 with follow-up question. Everyone should know. (31 - 60)
31. What is ng-content and how does it work? Answer: ng-content is used for content projection, allowing you to insert external HTML into a component's template. Follow-up: How do you create multiple content slots? => Use named slots with select attribute, like. 32. What is the difference between ngClass and ngStyle? Answer: ngClass is used for adding or removing CSS classes dynamically, while ngStyle is used for applying inline styles dynamically. Follow-up: How do you conditionally apply a class in Angular? => Use ngClass with an object or array syntax, e.g., [ngClass]="{ 'active': isActive }". 33. What is a BehaviorSubject in Angular? Answer: BehaviorSubject is a type of RxJS Subject that stores the current value and emits it to new subscribers. Follow-up: When would you use a BehaviorSubject over a Subject? => Use BehaviorSubject when you need to emit the latest value to new subscribers. 34. How do you implement lazy loading in Angular? Answer: Lazy loading in Angular is implemented by using loadChildren in route defnitions, which loads modules only when needed. Follow-up: What is the advantage of lazy loading? => It reduces initial load time by only loading modules required for the current view. 35. What is the RouterLink directive? Answer: RouterLink is a directive used to navigate between views or routes in an Angular app. It binds the URL to a route. Follow-up: How do you pass route parameters with RouterLink? => Use routerLink="/path/:id" and routerLinkActive to add active class to links. 36. What is the ngFor directive? Answer: ngFor is a structural directive that iterates over an array or list and creates a template for each item. Follow-up: How do you track items in ngFor for performance optimization? => Use trackBy to track items by a unique identifer, improving rendering performance. 37. What are HostListener and HostBinding in Angular? Answer: HostListener allows you to listen for events on the host element, while HostBinding binds properties to the host element. Follow-up: How do you use HostListener to listen for mouse events? => Use @hostlistener('event', ['$event']) to bind to DOM events like click, mouseenter, etc. 38. What are static and dynamic queries in Angular? Answer: Static queries are resolved once, such as @ViewChild and @ContentChild with static: true. Dynamic queries are resolved during change detection with static: false. Follow-up: When would you use static: true in @ViewChild? => Use static: true when you need the query result before the component’s view is initialized. 39. How do you create a custom form control in Angular? Answer: To create a custom form control, implement the ControlValueAccessor interface to connect your custom component with Angular’s forms API. Follow-up: What are the steps to implement ControlValueAccessor? => Implement writeValue(), registerOnChange(), and registerOnTouched() methods in the custom component. 40. How do you optimize Angular applications for performance? Answer: Optimizing Angular apps can include techniques like lazy loading, Ahead-of-Time (AOT) compilation, tree-shaking, and using ChangeDetectionStrategy.OnPush. Follow-up: How does tree-shaking improve performance? => Tree-shaking removes unused code during the build process, reducing bundle size. 41. What is the ngModel directive and how does it work? Answer: ngModel is a directive used for two-way data binding in Angular. It binds input elements to component properties, enabling automatic synchronization between them. Follow-up: How does two-way binding work with ngModel? => Two-way binding in ngModel is achieved by using the syntax [(ngModel)]="property", which binds the property to both the model and the view. 42. What is the purpose of ngOnChanges() lifecycle hook? Answer: ngOnChanges() is called whenever any input property changes in a component. It receives a SimpleChanges object, which provides previous and current values of the inputs. Follow-up: When is ngOnChanges() triggered in a component? => It is triggered whenever Angular detects changes in bound input properties. 43. What is the difference between HttpClient.get() and HttpClient.post() methods? Answer: HttpClient.get() is used to retrieve data from a server, while HttpClient.post() is used to send data to the server. Follow-up: How would you pass parameters with HttpClient.get()? => You can pass parameters using HttpParams with the params option in the get() method. 44. What is the purpose of RxJS operators in Angular? Answer: RxJS operators are used to compose and manipulate streams of data in Angular. They allow for handling asynchronous events such as HTTP requests, user interactions, and more. Follow-up: What is the difference between map and mergeMap operators? => map transforms the emitted value, while mergeMap fattens inner Observables and merges their results. 45. How do you implement inter-component communication in Angular? Answer: Inter-component communication can be achieved using @Input()
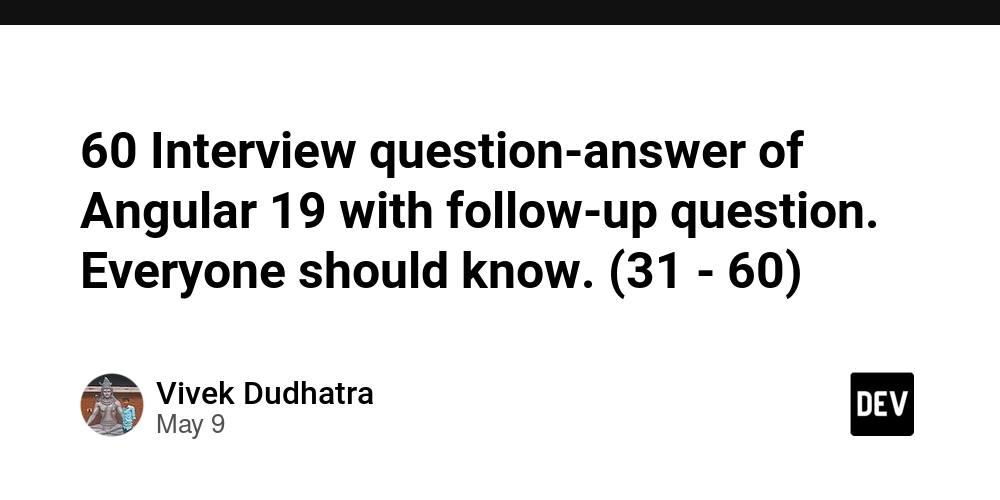
31. What is ng-content and how does it work?
Answer: ng-content is used for content projection, allowing you to insert external HTML into a component's template.
Follow-up: How do you create multiple content slots?
=> Use named slots with select attribute, like.
32. What is the difference between ngClass and ngStyle?
Answer: ngClass is used for adding or removing CSS classes dynamically, while ngStyle is used for applying inline styles dynamically.
Follow-up: How do you conditionally apply a class in Angular?
=> Use ngClass with an object or array syntax, e.g., [ngClass]="{ 'active': isActive }".
33. What is a BehaviorSubject in Angular?
Answer: BehaviorSubject is a type of RxJS Subject that stores the current value and emits it to new subscribers.
Follow-up: When would you use a BehaviorSubject over a Subject?
=> Use BehaviorSubject when you need to emit the latest value to new
subscribers.
34. How do you implement lazy loading in Angular?
Answer: Lazy loading in Angular is implemented by using loadChildren in route defnitions, which loads modules only when needed.
Follow-up: What is the advantage of lazy loading?
=> It reduces initial load time by only loading modules required for the current view.
35. What is the RouterLink directive?
Answer: RouterLink is a directive used to navigate between views or routes in an Angular app. It binds the URL to a route.
Follow-up: How do you pass route parameters with RouterLink?
=> Use routerLink="/path/:id" and routerLinkActive to add active class to links.
36. What is the ngFor directive?
Answer: ngFor is a structural directive that iterates over an array or list and creates a template for each item.
Follow-up: How do you track items in ngFor for performance optimization?
=> Use trackBy to track items by a unique identifer, improving rendering performance.
37. What are HostListener and HostBinding in Angular?
Answer: HostListener allows you to listen for events on the host element, while HostBinding binds properties to the host element.
Follow-up: How do you use HostListener to listen for mouse events?
=> Use @hostlistener('event', ['$event']) to bind to DOM events like click, mouseenter, etc.
38. What are static and dynamic queries in Angular?
Answer: Static queries are resolved once, such as @ViewChild and @ContentChild with static: true. Dynamic queries are resolved during change detection with static: false.
Follow-up: When would you use static: true in @ViewChild?
=> Use static: true when you need the query result before the component’s view is initialized.
39. How do you create a custom form control in Angular?
Answer: To create a custom form control, implement the ControlValueAccessor interface to connect your custom component with Angular’s forms API.
Follow-up: What are the steps to implement ControlValueAccessor?
=> Implement writeValue(), registerOnChange(), and registerOnTouched()
methods in the custom component.
40. How do you optimize Angular applications for performance?
Answer: Optimizing Angular apps can include techniques like lazy loading, Ahead-of-Time (AOT) compilation, tree-shaking, and using
ChangeDetectionStrategy.OnPush.
Follow-up: How does tree-shaking improve performance?
=> Tree-shaking removes unused code during the build process, reducing bundle size.
41. What is the ngModel directive and how does it work?
Answer: ngModel is a directive used for two-way data binding in Angular. It binds input elements to component properties, enabling automatic synchronization between them.
Follow-up: How does two-way binding work with ngModel?
=> Two-way binding in ngModel is achieved by using the syntax [(ngModel)]="property", which binds the property to both the model and the view.
42. What is the purpose of ngOnChanges() lifecycle hook?
Answer: ngOnChanges() is called whenever any input property changes in a component. It receives a SimpleChanges object, which provides previous and current values of the inputs.
Follow-up: When is ngOnChanges() triggered in a component?
=> It is triggered whenever Angular detects changes in bound input properties.
43. What is the difference between HttpClient.get() and HttpClient.post() methods?
Answer: HttpClient.get() is used to retrieve data from a server, while HttpClient.post() is used to send data to the server.
Follow-up: How would you pass parameters with HttpClient.get()?
=> You can pass parameters using HttpParams with the params option in the
get() method.
44. What is the purpose of RxJS operators in Angular?
Answer: RxJS operators are used to compose and manipulate streams of data in Angular. They allow for handling asynchronous events such as
HTTP requests, user interactions, and more.
Follow-up: What is the difference between map and mergeMap operators?
=> map transforms the emitted value, while mergeMap fattens inner
Observables and merges their results.
45. How do you implement inter-component communication in Angular?
Answer: Inter-component communication can be achieved using @Input() and @Output() decorators, services, or a shared store.
Follow-up: When would you use a service for inter-component communication?
=> When you need to share data or functionality across components without directly passing data through inputs/outputs.
46. What is a singleton service in Angular?
Answer: A singleton service is a service that is instantiated once and shared across multiple components and other services.
Follow-up: How do you create a singleton service in Angular?
=> Use the providedIn: 'root' confguration in the @Injectable() decorator.
47. What is the role of ChangeDetectionStrategy in Angular?
Answer: ChangeDetectionStrategy determines how Angular checks for changes in the application. The default strategy is ChangeDetectionStrategy.Default, but ChangeDetectionStrategy.OnPush improves performance by checking for changes only when certain conditions are met.
Follow-up: How does OnPush change detection strategy improve performance?
=> It only checks components when their inputs change or events occur within them, reducing unnecessary checks.
48. What are Angular pipes and how do you use them?
Answer: Pipes are used for transforming data in templates. They can be used for formatting, fltering, or transforming values displayed in the
view.
Follow-up: How do you create a custom pipe in Angular?
=> Create a class with the @pipe decorator and implement the PipeTransform interface.
49. What is the difference between ViewChild and ContentChild?
Answer: ViewChild is used to query elements within the component's view, while ContentChild is used to query projected content inside the
component (via ng-content).
Follow-up: When would you use ViewChild over ContentChild?
=> Use ViewChild when you need to access elements in the component's view, not projected content.
50. What is the difference between Injector and ngOnInit() in Angular?
Answer: Injector is an Angular service used for accessing dependency injections manually, whereas ngOnInit() is a lifecycle hook that initializes component properties.
Follow-up: What is the use case for using an Injector?
=> You can use Injector when you need to manually instantiate a dependency at runtime.
51. What is the ngFor directive in Angular?
Answer: The ngFor directive is used to iterate over a list or array and create a template for each item in the list.
Follow-up: How do you optimize ngFor for large lists?
=> Use the trackBy function to track items by a unique identifer, improving rendering performance.
52. What is the difference between template-driven forms and reactive forms?
Answer: Template-driven forms are created using Angular directives in the template and are more declarative, while reactive forms are created
in the component and provide more control over the form's structure and validation.
Follow-up: When would you use template-driven forms over reactive forms?
=> Template-driven forms are better for simple forms, while reactive
forms are more suitable for complex, dynamic forms.
53. How do you create an Angular service?
Answer: Angular services are created by using the @Injectable() decorator and are typically provided in the root or module to be injected into components or other services.
Follow-up: How do you provide a service at the component level?
=> Use the providers array in the component's metadata to provide the service locally.
54. What is the purpose of the @Injectable() decorator in Angular?
Answer: The @Injectable() decorator marks a class as a service that can be injected into other components or services via Angular’s
dependency injection system.
Follow-up: How does Angular handle dependency injection?
=> Angular uses the @Inject() decorator or constructor injection to provide the required dependencies.
55. What is the difference between a module and a component in Angular?
Answer: A module is a collection of components, services, and other Angular elements that work together, while a component is a unit of the
user interface with its own view and logic.
Follow-up: How are modules useful in large Angular applications?
=> Modules help organize code into cohesive blocks, making it easier to
manage and scale the application.
56. What are the advantages of Angular over other frameworks like React and Vue?
Answer: Angular is a complete, opinionated framework that offers tools like two-way data binding, routing, and state management. It is a full fledged solution for building enterprise-scale applications.
Follow-up: What makes Angular more suitable for large applications?
=> Its powerful features like dependency injection, strong typing (via
TypeScript), and built-in tools for forms, routing, and HTTP.
57. How do you enable lazy loading in Angular?
Answer: Lazy loading is enabled by defining route configurations with loadChildren in Angular's router module, which loads a module only when
a route is accessed.
Follow-up: What is the beneft of using lazy loading?
=> It reduces the initial loading time of the application by loading modules on demand.
58. What is an Observable in Angular?
Answer: An Observable is a stream of data that can emit multiple values over time. In Angular, it is used to handle asynchronous operations
such as HTTP requests, user inputs, or events.
Follow-up: How do you handle an Observable in Angular?
=> Use RxJS operators like subscribe(), map(), catchError, etc., to process the emitted
values.
59. What are Angular modules and how are they used? **
**Answer: Angular modules are containers for related components, services, and other Angular entities. They provide an organized structure for the app.
Follow-up: What is the role of AppModule in an Angular application?
=> AppModule is the root module that bootstraps the Angular application.
60. What is the role of Angular's ngZone?
Answer: ngZone is used to manage Angular's change detection mechanism. It allows the app to run outside of Angular's zone, helping to
improve performance, particularly for long-running tasks like animations or WebSocket subscriptions.
Follow-up: How does ngZone affect performance in Angular?
=> ngZone helps in reducing unnecessary change detection cycles, optimizing performance by only triggering updates when necessary.