Using marisa.cr for Efficient String Storage in Crystal
The marisa.cr Crystal shard gives you access to the powerful Marisa Trie data structure, perfect for storing and searching strings efficiently. Let's look at how to use it. First, install the shard by adding it to your shard.yml: dependencies: marisa: git: https://codeberg.org/bendangelo/marisa.cr.git Basic Usage Create a trie and add some strings: require "marisa" trie = Marisa::Trie.new trie.add("snow") trie.add("snow cone") trie true Working with Weights Add strings with weights (useful for prioritization): trie.add("ice", 1_f32) trie.get_weight("ice") # => 1.0e-45_f32 Bulk Operations Add multiple strings at once: trie.add_many(["icicle", "snowball"]) Iterate through all keys: trie.each do |key| puts key end Saving and Loading Save your trie to disk: trie.save("winter.trie") Load it later: trie = Marisa::Trie.new trie.load("winter.trie") Specialized Tries For binary data: bytes_trie = Marisa::BytesTrie.new("one" => "1", "two" => "2") bytes_trie["one"] # => "1" For integer values: int_trie = Marisa::IntTrie.new("one" => 1, "two" => 2) int_trie["one"] # => 1 int_trie.sum("one") # => 4 (sums all matching entries) Advanced Options Customize your trie: trie = Marisa::Trie.new( ["test"], [1.0_f32], binary: true, num_tries: 10, cache_size: :large, order: :weight ) The marisa.cr shard is a great choice when you need compact, efficient string storage with fast lookup capabilities. Give it a try for your next autocomplete or search feature!
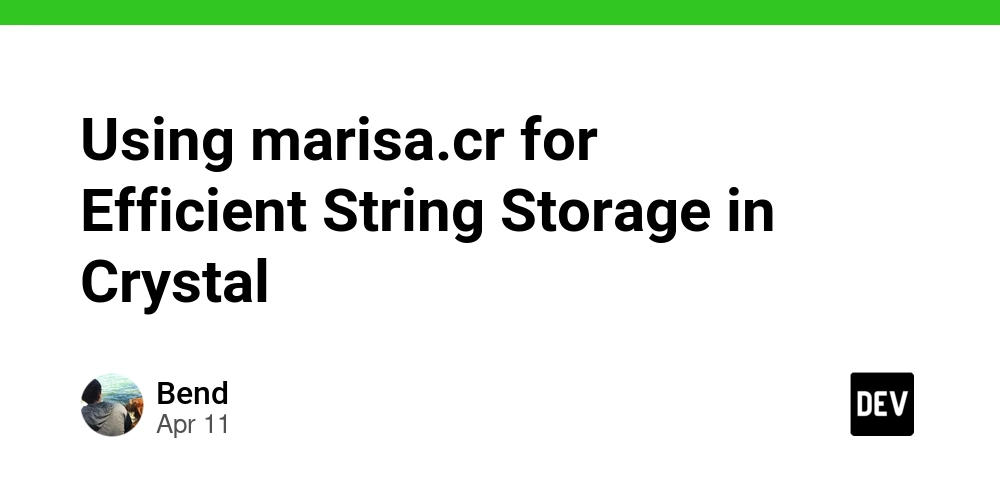
The marisa.cr
Crystal shard gives you access to the powerful Marisa Trie data structure, perfect for storing and searching strings efficiently. Let's look at how to use it.
First, install the shard by adding it to your shard.yml
:
dependencies:
marisa:
git: https://codeberg.org/bendangelo/marisa.cr.git
Basic Usage
Create a trie and add some strings:
require "marisa"
trie = Marisa::Trie.new
trie.add("snow")
trie.add("snow cone")
trie << "ice cream" # same as add
You can search for strings:
trie.search("ice").keys
# => ["ice", "ice cream"]
Check if a string exists:
trie.include?("snow") # => true
Working with Weights
Add strings with weights (useful for prioritization):
trie.add("ice", 1_f32)
trie.get_weight("ice") # => 1.0e-45_f32
Bulk Operations
Add multiple strings at once:
trie.add_many(["icicle", "snowball"])
Iterate through all keys:
trie.each do |key|
puts key
end
Saving and Loading
Save your trie to disk:
trie.save("winter.trie")
Load it later:
trie = Marisa::Trie.new
trie.load("winter.trie")
Specialized Tries
For binary data:
bytes_trie = Marisa::BytesTrie.new("one" => "1", "two" => "2")
bytes_trie["one"] # => "1"
For integer values:
int_trie = Marisa::IntTrie.new("one" => 1, "two" => 2)
int_trie["one"] # => 1
int_trie.sum("one") # => 4 (sums all matching entries)
Advanced Options
Customize your trie:
trie = Marisa::Trie.new(
["test"],
[1.0_f32],
binary: true,
num_tries: 10,
cache_size: :large,
order: :weight
)
The marisa.cr shard is a great choice when you need compact, efficient string storage with fast lookup capabilities. Give it a try for your next autocomplete or search feature!