useReducer is a React Hook that provides an alternative to useState for managing complex state logic. It's particularly useful when state transitions depend on the previous state or involve multiple sub-states. const [state, dispatch] = useReducer(reducer, initialState); reducer: A function that takes the current state and an action, then returns the new state. Based on the action.type, it returns the new state. initialState: The initial value of the state. state: holds the current state value dispatch: A function used to send actions to the reducer. const initialState = {count : 0}; function reducer(state, action) { switch (action.type) { case "increment": // Return a NEW object with the updated property return { ...state, count: state.count + action.payload }; case "decrement": return { ...state, count: state.count - action.payload }; // ❌ ❌ BAD: Returns a number, not the state object shape! AVOID // case "increment": // return state.count + action.payload; default: throw new Error("Unknown action type"); } } function Component(){ const [state, dispatch] = useReducer(reducer, initialState); const incrementCount = function(){ //sends an action to the reducer, updating the state accordingly. dispatch({ type: "increment", payload: 1 }) } const decrementCount = function(){ //sends an action to the reducer, updating the state accordingly dispatch({ type: "decrement", payload: 1 }) } return ( + Count: {state.count} - ) }
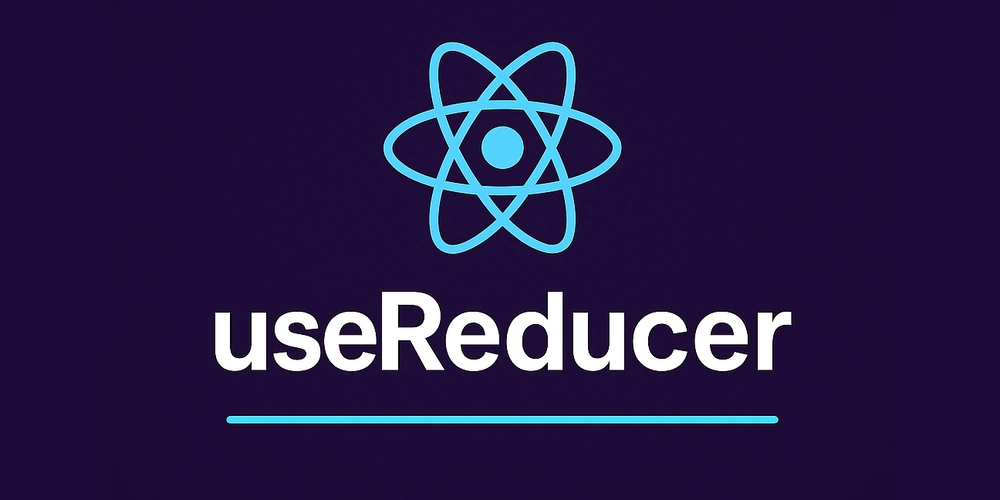
useReducer
is a React Hook that provides an alternative to useState
for managing complex state logic. It's particularly useful when state transitions depend on the previous state or involve multiple sub-states.
const [state, dispatch] = useReducer(reducer, initialState);
- reducer: A function that takes the current state and an action, then returns the new state. Based on the action.type, it returns the new state.
- initialState: The initial value of the state.
- state: holds the current state value
- dispatch: A function used to send actions to the reducer.
const initialState = {count : 0};
function reducer(state, action) {
switch (action.type) {
case "increment":
// Return a NEW object with the updated property
return { ...state, count: state.count + action.payload };
case "decrement":
return { ...state, count: state.count - action.payload };
// ❌ ❌ BAD: Returns a number, not the state object shape! AVOID
// case "increment":
// return state.count + action.payload;
default:
throw new Error("Unknown action type");
}
}
function Component(){
const [state, dispatch] = useReducer(reducer, initialState);
const incrementCount = function(){
//sends an action to the reducer, updating the state accordingly.
dispatch({ type: "increment", payload: 1 })
}
const decrementCount = function(){
//sends an action to the reducer, updating the state accordingly
dispatch({ type: "decrement", payload: 1 })
}
return (
<button onClick={incrementCount}>+button>
<span>Count: {state.count}span>
<button onClick={decrementCount}>-button>
)
}