Frontend Development Using Vue.js
Vue.js is one of the most popular JavaScript frameworks for building interactive user interfaces and single-page applications. It’s lightweight, flexible, and beginner-friendly — making it an excellent choice for frontend developers of all skill levels. What is Vue.js? Vue.js is an open-source JavaScript framework for building UIs. It was created by Evan You and is designed to be incrementally adoptable. That means you can use it to enhance parts of your webpage or build full-featured SPAs (Single Page Applications). Why Choose Vue.js? Easy to Learn: Simple syntax and comprehensive documentation. Component-Based: Encourages reusable and maintainable code. Lightweight: Fast to load and quick to execute. Reactive Data Binding: Automatically updates the DOM when data changes. Great Tooling: Vue CLI, Devtools, and a strong ecosystem. Getting Started with Vue.js You can include Vue.js via CDN or use Vue CLI for a full project setup. Using CDN (Quick Start) {{ message }} var app = new Vue({ el: '#app', data: { message: 'Hello Vue!' } }); Using Vue CLI (Recommended for Projects) npm install -g @vue/cli vue create my-vue-app cd my-vue-app npm run serve Key Vue.js Concepts Templates: HTML-like syntax with embedded expressions. Directives: Special attributes like v-if, v-for, v-model. Components: Reusable, encapsulated UI blocks. Props & Events: Communication between parent and child components. Reactivity System: Automatically updates DOM when data changes. Vue Router: For building SPAs with routing. Vuex: State management for complex applications. Example: Creating a Component Vue.component('todo-item', { props: ['item'], template: '{{ item.text }}' }); Popular Vue.js Libraries & Tools Vue Router: Adds navigation and routing features. Vuex: Centralized state management. Vuetify / BootstrapVue: UI frameworks for Vue.js. Vite: Lightning-fast development server and build tool for Vue 3. Best Practices Break your UI into small, reusable components. Use Vue CLI or Vite for project scaffolding. Keep components clean and focused. Use Vue DevTools for debugging. Organize files using standard folder structures (components, views, assets, store). Conclusion Vue.js is a powerful and approachable framework for frontend development. Whether you're enhancing a small section of your site or building a full-scale web app, Vue offers the tools and flexibility you need. Dive into Vue and take your frontend skills to the next level!
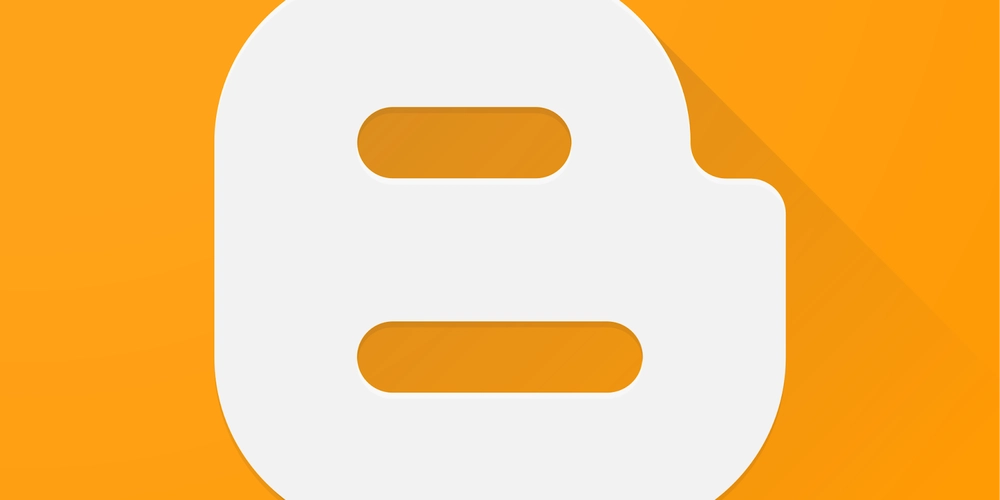
Vue.js is one of the most popular JavaScript frameworks for building interactive user interfaces and single-page applications. It’s lightweight, flexible, and beginner-friendly — making it an excellent choice for frontend developers of all skill levels.
What is Vue.js?
Vue.js is an open-source JavaScript framework for building UIs. It was created by Evan You and is designed to be incrementally adoptable. That means you can use it to enhance parts of your webpage or build full-featured SPAs (Single Page Applications).
Why Choose Vue.js?
- Easy to Learn: Simple syntax and comprehensive documentation.
- Component-Based: Encourages reusable and maintainable code.
- Lightweight: Fast to load and quick to execute.
- Reactive Data Binding: Automatically updates the DOM when data changes.
- Great Tooling: Vue CLI, Devtools, and a strong ecosystem.
Getting Started with Vue.js
You can include Vue.js via CDN or use Vue CLI for a full project setup.
Using CDN (Quick Start)
>
{{ message }}
Using Vue CLI (Recommended for Projects)
npm install -g @vue/cli
vue create my-vue-app
cd my-vue-app
npm run serve
Key Vue.js Concepts
- Templates: HTML-like syntax with embedded expressions.
-
Directives: Special attributes like
v-if
,v-for
,v-model
. - Components: Reusable, encapsulated UI blocks.
- Props & Events: Communication between parent and child components.
- Reactivity System: Automatically updates DOM when data changes.
- Vue Router: For building SPAs with routing.
- Vuex: State management for complex applications.
Example: Creating a Component
Vue.component('todo-item', {
props: ['item'],
template: '
});
Popular Vue.js Libraries & Tools
- Vue Router: Adds navigation and routing features.
- Vuex: Centralized state management.
- Vuetify / BootstrapVue: UI frameworks for Vue.js.
- Vite: Lightning-fast development server and build tool for Vue 3.
Best Practices
- Break your UI into small, reusable components.
- Use Vue CLI or Vite for project scaffolding.
- Keep components clean and focused.
- Use Vue DevTools for debugging.
- Organize files using standard folder structures (components, views, assets, store).
Conclusion
Vue.js is a powerful and approachable framework for frontend development. Whether you're enhancing a small section of your site or building a full-scale web app, Vue offers the tools and flexibility you need. Dive into Vue and take your frontend skills to the next level!