Database Management System (DBMS) Development
Databases are at the heart of almost every software system. Whether it's a social media app, e-commerce platform, or business software, data must be stored, retrieved, and managed efficiently. A Database Management System (DBMS) is software designed to handle these tasks. In this post, we’ll explore how DBMSs are developed and what you need to know as a developer. What is a DBMS? A Database Management System is software that provides an interface for users and applications to interact with data. It supports operations like CRUD (Create, Read, Update, Delete), query processing, concurrency control, and data integrity. Types of DBMS Relational DBMS (RDBMS): Organizes data into tables. Examples: MySQL, PostgreSQL, Oracle. NoSQL DBMS: Used for non-relational or schema-less data. Examples: MongoDB, Cassandra, CouchDB. In-Memory DBMS: Optimized for speed, storing data in RAM. Examples: Redis, Memcached. Distributed DBMS: Handles data across multiple nodes or locations. Examples: Apache Cassandra, Google Spanner. Core Components of a DBMS Query Processor: Interprets SQL queries and converts them to low-level instructions. Storage Engine: Manages how data is stored and retrieved on disk or memory. Transaction Manager: Ensures consistency and handles ACID properties (Atomicity, Consistency, Isolation, Durability). Concurrency Control: Manages simultaneous transactions safely. Buffer Manager: Manages data caching between memory and disk. Indexing System: Enhances data retrieval speed. Languages Used in DBMS Development C/C++: For low-level operations and high-performance components. Rust: Increasingly popular due to safety and concurrency features. Python: Used for prototyping or scripting. Go: Ideal for building scalable and concurrent systems. Example: Building a Simple Key-Value Store in Python class KeyValueDB: def init(self): self.store = {} def insert(self, key, value): self.store[key] = value def get(self, key): return self.store.get(key) def delete(self, key): if key in self.store: del self.store[key] db = KeyValueDB() db.insert('name', 'Alice') print(db.get('name')) # Output: Alice Challenges in DBMS Development Efficient query parsing and execution Data consistency and concurrency issues Crash recovery and durability Scalability for large data volumes Security and user access control Popular Open Source DBMS Projects to Study SQLite: Lightweight and embedded relational DBMS. PostgreSQL: Full-featured, open-source RDBMS with advanced functionality. LevelDB: High-performance key-value store from Google. RethinkDB: Real-time NoSQL database. Conclusion Understanding how DBMSs work internally is not only intellectually rewarding but also extremely useful for optimizing application performance and managing data. Whether you're designing your own lightweight DBMS or just exploring how your favorite database works, these fundamentals will guide you in the right direction.
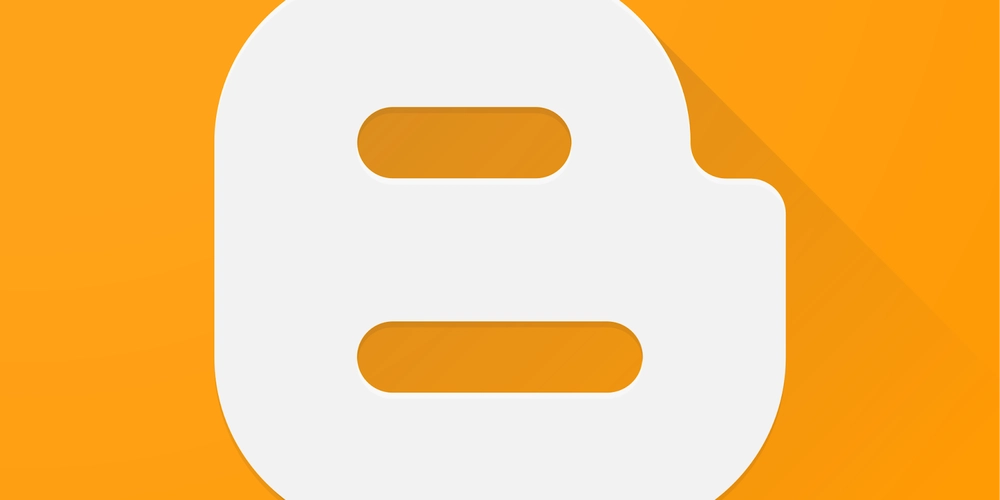
Databases are at the heart of almost every software system. Whether it's a social media app, e-commerce platform, or business software, data must be stored, retrieved, and managed efficiently. A Database Management System (DBMS) is software designed to handle these tasks. In this post, we’ll explore how DBMSs are developed and what you need to know as a developer.
What is a DBMS?
A Database Management System is software that provides an interface for users and applications to interact with data. It supports operations like CRUD (Create, Read, Update, Delete), query processing, concurrency control, and data integrity.
Types of DBMS
- Relational DBMS (RDBMS): Organizes data into tables. Examples: MySQL, PostgreSQL, Oracle.
- NoSQL DBMS: Used for non-relational or schema-less data. Examples: MongoDB, Cassandra, CouchDB.
- In-Memory DBMS: Optimized for speed, storing data in RAM. Examples: Redis, Memcached.
- Distributed DBMS: Handles data across multiple nodes or locations. Examples: Apache Cassandra, Google Spanner.
Core Components of a DBMS
- Query Processor: Interprets SQL queries and converts them to low-level instructions.
- Storage Engine: Manages how data is stored and retrieved on disk or memory.
- Transaction Manager: Ensures consistency and handles ACID properties (Atomicity, Consistency, Isolation, Durability).
- Concurrency Control: Manages simultaneous transactions safely.
- Buffer Manager: Manages data caching between memory and disk.
- Indexing System: Enhances data retrieval speed.
Languages Used in DBMS Development
- C/C++: For low-level operations and high-performance components.
- Rust: Increasingly popular due to safety and concurrency features.
- Python: Used for prototyping or scripting.
- Go: Ideal for building scalable and concurrent systems.
Example: Building a Simple Key-Value Store in Python
class KeyValueDB:
def init(self):
self.store = {}
def insert(self, key, value):
self.store[key] = value
def get(self, key):
return self.store.get(key)
def delete(self, key):
if key in self.store:
del self.store[key]
db = KeyValueDB()
db.insert('name', 'Alice')
print(db.get('name')) # Output: Alice
Challenges in DBMS Development
- Efficient query parsing and execution
- Data consistency and concurrency issues
- Crash recovery and durability
- Scalability for large data volumes
- Security and user access control
Popular Open Source DBMS Projects to Study
- SQLite: Lightweight and embedded relational DBMS.
- PostgreSQL: Full-featured, open-source RDBMS with advanced functionality.
- LevelDB: High-performance key-value store from Google.
- RethinkDB: Real-time NoSQL database.
Conclusion
Understanding how DBMSs work internally is not only intellectually rewarding but also extremely useful for optimizing application performance and managing data. Whether you're designing your own lightweight DBMS or just exploring how your favorite database works, these fundamentals will guide you in the right direction.