Why gRPC is a Great Choice
Introduction gRPC (Google Remote Procedure Call) is a high-performance, open-source framework that enables efficient communication between applications and services. Built on HTTP/2, it leverages Protocol Buffers (protobuf) for serialisation, making it a compelling alternative to traditional REST APIs, WebSockets (WS), or plain HTTP communication. This article explores why gRPC is a strong choice, when to use it, and the advantages of streaming events via gRPC compared to WebSockets or HTTP. Why Choose gRPC? gRPC provides several advantages over traditional API communication methods: 1. Performance & Efficiency gRPC uses Protocol Buffers (protobuf), a compact binary format that is much smaller and faster to process than JSON or XML. It runs over HTTP/2, enabling multiplexing, which allows multiple requests and responses over the same connection, reducing latency. 2. Strongly Typed Contracts gRPC defines service interfaces using .proto files, ensuring a strict, well-defined API contract. Auto-generates client and server code for multiple languages, reducing development effort and errors. 3. Streaming Capabilities Unlike REST, which follows a request-response model, gRPC supports bidirectional streaming, allowing continuous data flow between the client and server. This is ideal for real-time applications like chat apps, telemetry, stock price updates, and monitoring systems. 4. Multi-Language Support gRPC is language-agnostic, allowing seamless communication between applications written in different programming languages. Supports TypeScript, Python, Java, Go, C++, and more. 5. Authentication & Security Supports built-in TLS encryption for secure communication. Integrates easily with OAuth, JWT, and API keys for authentication. When to Use gRPC gRPC is a great choice when: You Need High-Performance, Low-Latency Communication Ideal for microservices architectures, where services communicate frequently. Suitable for high-frequency API calls, reducing overhead compared to REST. Streaming Large or Continuous Data Perfect for scenarios where real-time event streaming is required, such as live analytics or stock market feeds. You Need a Strongly Typed API Ensures strict API definitions, reducing errors between client and server implementations. Inter-Service Communication in Microservices gRPC is widely used for microservices communication, especially within Kubernetes-based environments. You Require Multi-Language Compatibility Ideal when different services are written in multiple programming languages, as gRPC can generate client and server stubs automatically. Why Use gRPC for Streaming Events Over WebSockets or HTTP? Streaming data efficiently between services is a common requirement, and gRPC provides key advantages over WebSockets (WS) and HTTP: 1. Efficient Binary Protocol gRPC uses Protocol Buffers, which are significantly smaller and more efficient than JSON payloads used in WebSockets or RESTful APIs. WebSockets send raw data, often requiring additional parsing and handling on both ends. 2. Multiplexing & HTTP/2 WebSockets use a single TCP connection, which can lead to congestion under heavy loads. gRPC operates over HTTP/2, allowing multiple requests and responses to be sent over the same connection without blocking. 3. Built-in Backpressure Handling gRPC handles flow control automatically, preventing the server from overwhelming the client with too much data at once. WebSockets lack native backpressure handling, requiring manual implementation. 4. Better Error Handling & Retries gRPC has built-in error codes and automatic retries. WebSockets lack standardised error handling, requiring custom implementations. 5. Native Language Support & Auto-Generated Code gRPC clients are automatically generated for multiple programming languages. WebSockets require custom implementations for each language. TypeScript Example: Streaming Events via gRPC The following TypeScript implementation demonstrates a gRPC server and client using streaming. gRPC Server (server.ts) import * as grpc from "@grpc/grpc-js"; import * as protoLoader from "@grpc/proto-loader"; import { EventMessage } from "./proto/EventControllerPackage/EventMessage"; import path from "path"; import fs from "fs"; import { ProtoGrpcType } from "./proto/event"; const _PROTO_PATH = path.join(__dirname, "proto", "event.proto"); const found = fs.existsSync(_PROTO_PATH); console.log(found); const packageDefinition = protoLoader.loadSync(_PROTO_PATH); const grpcObj = grpc.loadPackageDefinition(packageDefinition) as unknown as ProtoGrpcType; const eventController = grpcObj.EventControllerPackage; const server = new grpc.Server(); server.addService(eventController.Controller.service, { StreamEvent: (client: any
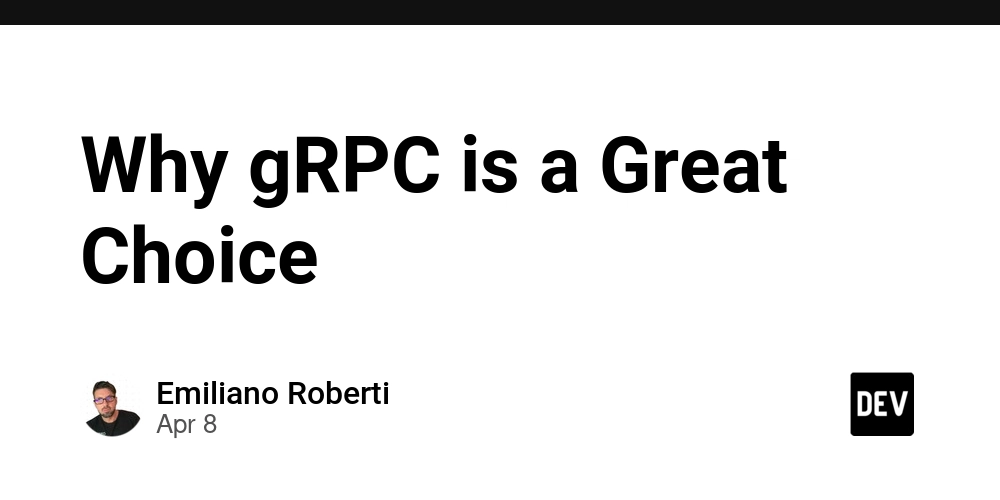
Introduction
gRPC (Google Remote Procedure Call) is a high-performance, open-source framework that enables efficient communication between applications and services. Built on HTTP/2, it leverages Protocol Buffers (protobuf) for serialisation, making it a compelling alternative to traditional REST APIs, WebSockets (WS), or plain HTTP communication.
This article explores why gRPC is a strong choice, when to use it, and the advantages of streaming events via gRPC compared to WebSockets or HTTP.
Why Choose gRPC?
gRPC provides several advantages over traditional API communication methods:
1. Performance & Efficiency
- gRPC uses Protocol Buffers (protobuf), a compact binary format that is much smaller and faster to process than JSON or XML.
- It runs over HTTP/2, enabling multiplexing, which allows multiple requests and responses over the same connection, reducing latency.
2. Strongly Typed Contracts
- gRPC defines service interfaces using .proto files, ensuring a strict, well-defined API contract.
- Auto-generates client and server code for multiple languages, reducing development effort and errors.
3. Streaming Capabilities
- Unlike REST, which follows a request-response model, gRPC supports bidirectional streaming, allowing continuous data flow between the client and server.
- This is ideal for real-time applications like chat apps, telemetry, stock price updates, and monitoring systems.
4. Multi-Language Support
- gRPC is language-agnostic, allowing seamless communication between applications written in different programming languages.
- Supports TypeScript, Python, Java, Go, C++, and more.
5. Authentication & Security
- Supports built-in TLS encryption for secure communication.
- Integrates easily with OAuth, JWT, and API keys for authentication.
When to Use gRPC
gRPC is a great choice when:
-
You Need High-Performance, Low-Latency Communication
- Ideal for microservices architectures, where services communicate frequently.
- Suitable for high-frequency API calls, reducing overhead compared to REST.
-
Streaming Large or Continuous Data
- Perfect for scenarios where real-time event streaming is required, such as live analytics or stock market feeds.
-
You Need a Strongly Typed API
- Ensures strict API definitions, reducing errors between client and server implementations.
-
Inter-Service Communication in Microservices
- gRPC is widely used for microservices communication, especially within Kubernetes-based environments.
-
You Require Multi-Language Compatibility
- Ideal when different services are written in multiple programming languages, as gRPC can generate client and server stubs automatically.
Why Use gRPC for Streaming Events Over WebSockets or HTTP?
Streaming data efficiently between services is a common requirement, and gRPC provides key advantages over WebSockets (WS) and HTTP:
1. Efficient Binary Protocol
- gRPC uses Protocol Buffers, which are significantly smaller and more efficient than JSON payloads used in WebSockets or RESTful APIs.
- WebSockets send raw data, often requiring additional parsing and handling on both ends.
2. Multiplexing & HTTP/2
- WebSockets use a single TCP connection, which can lead to congestion under heavy loads.
- gRPC operates over HTTP/2, allowing multiple requests and responses to be sent over the same connection without blocking.
3. Built-in Backpressure Handling
- gRPC handles flow control automatically, preventing the server from overwhelming the client with too much data at once.
- WebSockets lack native backpressure handling, requiring manual implementation.
4. Better Error Handling & Retries
- gRPC has built-in error codes and automatic retries.
- WebSockets lack standardised error handling, requiring custom implementations.
5. Native Language Support & Auto-Generated Code
- gRPC clients are automatically generated for multiple programming languages.
- WebSockets require custom implementations for each language.
TypeScript Example: Streaming Events via gRPC
The following TypeScript implementation demonstrates a gRPC server and client using streaming.
gRPC Server (server.ts
)
import * as grpc from "@grpc/grpc-js";
import * as protoLoader from "@grpc/proto-loader";
import { EventMessage } from "./proto/EventControllerPackage/EventMessage";
import path from "path";
import fs from "fs";
import { ProtoGrpcType } from "./proto/event";
const _PROTO_PATH = path.join(__dirname, "proto", "event.proto");
const found = fs.existsSync(_PROTO_PATH);
console.log(found);
const packageDefinition = protoLoader.loadSync(_PROTO_PATH);
const grpcObj = grpc.loadPackageDefinition(packageDefinition) as unknown as ProtoGrpcType;
const eventController = grpcObj.EventControllerPackage;
const server = new grpc.Server();
server.addService(eventController.Controller.service, {
StreamEvent: (client: any) => {
console.log("Connected", client.request.client_id);
let count = 0;
const interval = setInterval(() => {
const response: EventMessage = {
id: client.clientId,
message: JSON.stringify({ message: (count += 1) }),
timeStamp: new Date().toISOString(),
};
client.write(response);
if (count > 5) {
clearInterval(interval);
client.end();
}
}, 2000);
client.on("cancelled", () => {
console.log("Disconnected client", client.request.client_id);
});
},
});
const PORT = 50051;
server.bindAsync(
`0.0.0.0:${PORT}`,
grpc.ServerCredentials.createInsecure(),
() => {
console.log("gRPC server running on PORT", PORT);
}
);
gRPC Client (client.ts
)
import * as grpc from "@grpc/grpc-js";
import * as protoLoader from "@grpc/proto-loader";
import path from "path";
import { ProtoGrpcType } from "./proto/event";
import { EventRequest } from "./proto/EventControllerPackage/EventRequest";
const _PROTO_PATH = path.join(__dirname, "proto", "event.proto");
const packageDefinition = protoLoader.loadSync(_PROTO_PATH);
const grpcObj = grpc.loadPackageDefinition(packageDefinition) as unknown as ProtoGrpcType;
const client = new grpcObj.EventControllerPackage.Controller(
"localhost:50051",
grpc.credentials.createInsecure()
);
const stream = client.StreamEvent({ client_id: "client_emi" } as EventRequest);
stream.on("data", (response: any) => {
console.log(
`Received event: ${response.event_id} - ${response.message} at ${response.timestamp}`
);
});
stream.on("end", () => {
console.log("Stream ended by server.");
});
Conclusion
gRPC is a powerful alternative to REST, WebSockets, and HTTP for modern applications. Its efficiency, strong typing, and superior streaming capabilities make it the ideal choice for real-time, high-performance applications. Whether you're building microservices, IoT applications, or real-time event-driven systems, gRPC provides a fast, reliable, and scalable communication solution.