Turn Your React App into a PWA That Works Offline, Installs Like Magic & Feels Native
Introduction The first time I encountered a Progressive Web App (PWA), I was using Twitter on a painfully slow connection while traveling. I remember being impressed that Twitter still loaded, allowed me to scroll, and even let me post a tweet. Later, I discovered that what I was experiencing was the magic of a well-built PWA. PWAs are more than just websites. They combine the best of both web and mobile apps—lightweight, installable, offline-capable, and blazing fast. For developers, especially those already using React, building a PWA can be a game-changer. It means offering users the flexibility of the web with the performance and reliability of a native application. In this blog post, we’ll walk through the entire process of turning a regular React app into a robust PWA. We'll not only touch on the how, but also explain the why at each step. Whether you're building a portfolio site, a budget tracker, or a full-fledged social media app, understanding how to “PWA-ify” your React app is a skill worth learning. 1. Prerequisites Before we start writing any code, let’s take a quick look at what you'll need to follow along smoothly. Basic React Knowledge You don’t need to be an expert, but understanding React components, props, and hooks will help you a lot. If you’ve built a simple app like a to-do list or a weather app, you’re ready. Node.js and npm Installed We’ll use Node.js to run our development server and npm (or Yarn) to manage dependencies. Make sure you’ve got these installed. You can check by running: node -v npm -v If you’re seeing version numbers, you’re good to go. If not, download them from https://nodejs.org/. A React App to Work With We’ll use Create React App (CRA) in this guide because it comes with built-in PWA support. If you already have a CRA project, you can use it. Otherwise, we’ll create one in the next section. Tools and Libraries While the native service worker provided by CRA is great, we’ll also introduce Workbox, a powerful toolset by Google for managing caching and offline behavior. Real-World Use Case: Job Application Tracker For this walkthrough, imagine we’re building a Job Application Tracker—a simple app where users can add jobs they've applied to, mark statuses, and track interviews. Making this app a PWA ensures users can check their application statuses even when offline, such as during travel or in areas with spotty connections 2. Setting Up the Project To bring our Job Application Tracker idea to life, we’ll begin by setting up a fresh React project using Create React App. CRA is a solid starting point because it includes basic PWA configurations out of the box—especially the service worker setup that we’ll expand upon later. Step 1: Create a New React App Open your terminal and run: npx create-react-app job-tracker-pwa This command scaffolds a new React project in a folder called job-tracker-pwa with a working development setup. Navigate into your project folder: cd job-tracker-pwa Start the development server to make sure everything’s working: npm start Once the server starts, open http://localhost:3000 in your browser. You should see the default CRA homepage. At this point, we’ve got a basic React app running. Think of it as the foundation for our PWA. All the ingredients we need are here—we just need to fine-tune them. Step 2: Understand the Folder Structure Here’s a quick overview of the key folders and files we’ll be working with: public/manifest.json – describes your app to the browser (name, icons, colors, etc.) src/service-worker.js (or equivalent CRA abstraction) – handles caching and offline behavior src/index.js – where you register the service worker public/index.html – contains the link to the manifest file We'll gradually update each of these to turn our app into a PWA. Step 3: Clean Up the Boilerplate To focus on our job tracker, let’s clean up the default content: Delete the CRA logo and default content from App.js Remove unnecessary CSS or assets in App.css and logo.svg Replace it with a simple heading: function App() { return ( Job Application Tracker Track your job applications, interviews, and offers. ); } This gives us a clean slate to build from—just like clearing out a room before redecorating. 3. Understanding PWA Essentials Before we begin tweaking the code to turn this into a real PWA, let’s understand what makes a web app progressive. It boils down to a few key technologies and concepts: Web App Manifest The Web App Manifest is a simple JSON file that gives the browser information about your app—its name, icon, theme color, how it should be displayed, and more. It’s what allows a user to “install” your app to their home screen and what makes the app look like a native app when launched.
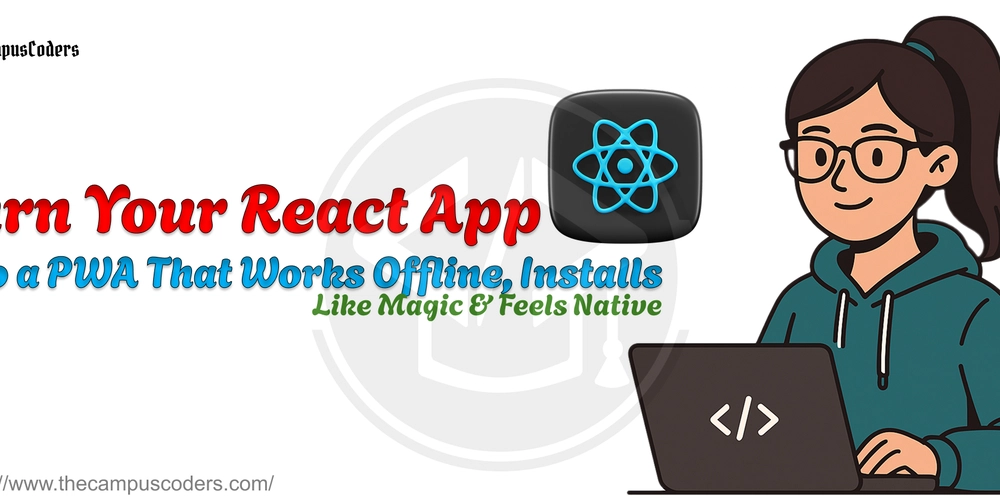
Introduction
The first time I encountered a Progressive Web App (PWA), I was using Twitter on a painfully slow connection while traveling. I remember being impressed that Twitter still loaded, allowed me to scroll, and even let me post a tweet. Later, I discovered that what I was experiencing was the magic of a well-built PWA.
PWAs are more than just websites. They combine the best of both web and mobile apps—lightweight, installable, offline-capable, and blazing fast. For developers, especially those already using React, building a PWA can be a game-changer. It means offering users the flexibility of the web with the performance and reliability of a native application.
In this blog post, we’ll walk through the entire process of turning a regular React app into a robust PWA. We'll not only touch on the how, but also explain the why at each step. Whether you're building a portfolio site, a budget tracker, or a full-fledged social media app, understanding how to “PWA-ify” your React app is a skill worth learning.
1. Prerequisites
Before we start writing any code, let’s take a quick look at what you'll need to follow along smoothly.
Basic React Knowledge
You don’t need to be an expert, but understanding React components, props, and hooks will help you a lot. If you’ve built a simple app like a to-do list or a weather app, you’re ready.
Node.js and npm Installed
We’ll use Node.js to run our development server and npm (or Yarn) to manage dependencies. Make sure you’ve got these installed. You can check by running:
node -v
npm -v
If you’re seeing version numbers, you’re good to go. If not, download them from https://nodejs.org/.
A React App to Work With
We’ll use Create React App (CRA) in this guide because it comes with built-in PWA support. If you already have a CRA project, you can use it. Otherwise, we’ll create one in the next section.
Tools and Libraries
While the native service worker provided by CRA is great, we’ll also introduce Workbox, a powerful toolset by Google for managing caching and offline behavior.
Real-World Use Case: Job Application Tracker
For this walkthrough, imagine we’re building a Job Application Tracker—a simple app where users can add jobs they've applied to, mark statuses, and track interviews. Making this app a PWA ensures users can check their application statuses even when offline, such as during travel or in areas with spotty connections
2. Setting Up the Project
To bring our Job Application Tracker idea to life, we’ll begin by setting up a fresh React project using Create React App. CRA is a solid starting point because it includes basic PWA configurations out of the box—especially the service worker setup that we’ll expand upon later.
Step 1: Create a New React App
Open your terminal and run:
npx create-react-app job-tracker-pwa
This command scaffolds a new React project in a folder called job-tracker-pwa
with a working development setup.
Navigate into your project folder:
cd job-tracker-pwa
Start the development server to make sure everything’s working:
npm start
Once the server starts, open http://localhost:3000 in your browser. You should see the default CRA homepage.
At this point, we’ve got a basic React app running. Think of it as the foundation for our PWA. All the ingredients we need are here—we just need to fine-tune them.
Step 2: Understand the Folder Structure
Here’s a quick overview of the key folders and files we’ll be working with:
-
public/manifest.json
– describes your app to the browser (name, icons, colors, etc.) -
src/service-worker.js
(or equivalent CRA abstraction) – handles caching and offline behavior -
src/index.js
– where you register the service worker -
public/index.html
– contains the link to the manifest file
We'll gradually update each of these to turn our app into a PWA.
Step 3: Clean Up the Boilerplate
To focus on our job tracker, let’s clean up the default content:
- Delete the CRA logo and default content from
App.js
- Remove unnecessary CSS or assets in
App.css
andlogo.svg
- Replace it with a simple heading:
function App() {
return (
<div className="App">
<h1>Job Application Trackerh1>
<p>Track your job applications, interviews, and offers.p>
div>
);
}
This gives us a clean slate to build from—just like clearing out a room before redecorating.
3. Understanding PWA Essentials
Before we begin tweaking the code to turn this into a real PWA, let’s understand what makes a web app progressive. It boils down to a few key technologies and concepts:
Web App Manifest
The Web App Manifest is a simple JSON file that gives the browser information about your app—its name, icon, theme color, how it should be displayed, and more.
It’s what allows a user to “install” your app to their home screen and what makes the app look like a native app when launched.
Service Workers
A Service Worker is a script that runs in the background, separate from your React app. It acts like a network proxy and can intercept requests, cache assets, and provide content even when the user is offline.
Service workers power the offline capabilities and performance boost that make PWAs so impressive. They’re what allowed Twitter to load even when I was halfway through a train tunnel.
HTTPS is Required
Service workers only work on secure origins. So once we deploy, the app must be served over HTTPS. Most platforms like Vercel, Netlify, and Firebase Hosting handle this for you automatically.
App Shell Model
PWAs typically use the App Shell model—essentially caching the core layout of the app (header, footer, routing, etc.) so it loads instantly even if the network is slow or unavailable. The actual content (like job application data) can be fetched and updated when the connection returns.
4. Creating the Web Manifest File
Imagine your app is a product on a shelf. The manifest file is its label—telling the browser what it’s called, what it looks like, and how it should behave when someone installs it.
The manifest is a JSON file located in the public/
folder of your project. Let’s open the one that CRA generated for us: public/manifest.json
.
You’ll see some default settings like:
{
"short_name": "React App",
"name": "Create React App Sample",
"icons": [...],
"start_url": ".",
"display": "standalone",
"theme_color": "#000000",
"background_color": "#ffffff"
}
Let’s customize this to fit our Job Application Tracker:
{
"short_name": "JobTracker",
"name": "Job Application Tracker",
"icons": [
{
"src": "icons/icon-192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icons/icon-512.png",
"sizes": "512x512",
"type": "image/png"
}
],
"start_url": "/",
"display": "standalone",
"theme_color": "#0d9488",
"background_color": "#ffffff"
}