TS1388: Constructor type notation must be parenthesized when used in an intersection type
TS1388: Constructor type notation must be parenthesized when used in an intersection type TypeScript is a powerful programming language that builds on JavaScript by adding static type definitions. This means that you can define types for variables, function parameters, and return values, which helps catch errors at compile time rather than at runtime. Types in TypeScript include primitive types like string and number, as well as more complex structures like objects and arrays. If you're looking to deepen your understanding of TypeScript or explore AI tools to improve your coding skills, I highly recommend subscribing to my blog or checking out gpteach for interactive learning. To understand more about TypeScript, it's essential to grasp what types are. In programming, a type defines a category of data, dictating the operations that can be performed on it and the way it's structured. For example, a variable can be of type string, which means it can only hold textual data, or type number, meaning it can store numeric values. TS1388: Constructor type notation must be parenthesized when used in an intersection type The error specified in TS1388: Constructor type notation must be parenthesized when used in an intersection type occurs when you try to use constructor type notation in an intersection type but forget to enclose it in parentheses. Here's a breakdown of what this means. Constructor types allow you to create types based on constructors. For example: class MyClass { constructor(public value: number) {} } type MyConstructorType = new (value: number) => MyClass; // This is valid However, if you try to create an intersection type without proper parentheses, you'll encounter error TS1388. For instance: type MyType = MyConstructorType & { someProperty: string }; // Error: TS1388 In this case, the TypeScript compiler is confused because it expects the constructor type notation to be surrounded by parentheses. To fix this error, you simply need to modify the code like this: type MyType = (new (value: number) => MyClass) & { someProperty: string }; // Corrected Important to know! Always use parentheses around constructor types in intersection types to avoid TS1388. Constructor types describe how instances of a class can be created, which is crucial for type safety in TypeScript. More Examples of TS1388 Let’s look at another example that generates error TS1388: class AnotherClass { constructor(public name: string) {} } type AnotherConstructorType = new (name: string) => AnotherClass; type CombinedType = AnotherConstructorType & { age: number }; // Error: TS1388 To resolve this, you need to use parentheses: type CombinedType = (new (name: string) => AnotherClass) & { age: number }; // Corrected FAQ Q: Why do I need to use parentheses in intersection types? A: Using parentheses clarifies the order of operations and ensures TypeScript understands your intentions regarding constructor type notation. Q: Can I use constructor types anywhere in TypeScript? A: Constructor types are specific to classes and can be used when defining types that involve instance creation. Q: What happens if I don't fix the TS1388 error? A: If the error TS1388 is not fixed, TypeScript will not compile your code, preventing you from running your application. Important to know! Always test your types with TypeScript's compiler. This helps catch errors like TS1388 early on in development. In summary, TS1388: Constructor type notation must be parenthesized when used in an intersection type is an important rule in TypeScript that ensures you use correct syntax when working with constructor types. Always remember to use parentheses around your constructor types when combining them in intersection types to maintain the integrity of your code and prevent errors. Understanding such syntactical rules can greatly enhance your TypeScript development experience, making your code more robust and error-free.
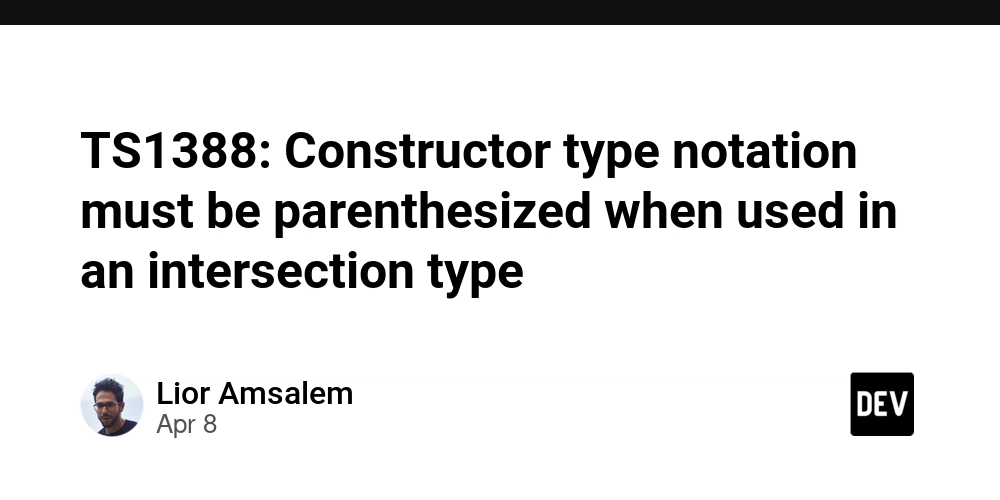
TS1388: Constructor type notation must be parenthesized when used in an intersection type
TypeScript is a powerful programming language that builds on JavaScript by adding static type definitions. This means that you can define types for variables, function parameters, and return values, which helps catch errors at compile time rather than at runtime. Types in TypeScript include primitive types like string
and number
, as well as more complex structures like objects and arrays.
If you're looking to deepen your understanding of TypeScript or explore AI tools to improve your coding skills, I highly recommend subscribing to my blog or checking out gpteach for interactive learning.
To understand more about TypeScript, it's essential to grasp what types are. In programming, a type defines a category of data, dictating the operations that can be performed on it and the way it's structured. For example, a variable can be of type string
, which means it can only hold textual data, or type number
, meaning it can store numeric values.
TS1388: Constructor type notation must be parenthesized when used in an intersection type
The error specified in TS1388: Constructor type notation must be parenthesized when used in an intersection type occurs when you try to use constructor type notation in an intersection type but forget to enclose it in parentheses.
Here's a breakdown of what this means. Constructor types allow you to create types based on constructors. For example:
class MyClass {
constructor(public value: number) {}
}
type MyConstructorType = new (value: number) => MyClass; // This is valid
However, if you try to create an intersection type without proper parentheses, you'll encounter error TS1388. For instance:
type MyType = MyConstructorType & { someProperty: string }; // Error: TS1388
In this case, the TypeScript compiler is confused because it expects the constructor type notation to be surrounded by parentheses. To fix this error, you simply need to modify the code like this:
type MyType = (new (value: number) => MyClass) & { someProperty: string }; // Corrected
Important to know!
- Always use parentheses around constructor types in intersection types to avoid TS1388.
- Constructor types describe how instances of a class can be created, which is crucial for type safety in TypeScript.
More Examples of TS1388
Let’s look at another example that generates error TS1388:
class AnotherClass {
constructor(public name: string) {}
}
type AnotherConstructorType = new (name: string) => AnotherClass;
type CombinedType = AnotherConstructorType & { age: number }; // Error: TS1388
To resolve this, you need to use parentheses:
type CombinedType = (new (name: string) => AnotherClass) & { age: number }; // Corrected
FAQ
Q: Why do I need to use parentheses in intersection types?
A: Using parentheses clarifies the order of operations and ensures TypeScript understands your intentions regarding constructor type notation.
Q: Can I use constructor types anywhere in TypeScript?
A: Constructor types are specific to classes and can be used when defining types that involve instance creation.
Q: What happens if I don't fix the TS1388 error?
A: If the error TS1388 is not fixed, TypeScript will not compile your code, preventing you from running your application.
Important to know!
- Always test your types with TypeScript's compiler. This helps catch errors like TS1388 early on in development.
In summary, TS1388: Constructor type notation must be parenthesized when used in an intersection type is an important rule in TypeScript that ensures you use correct syntax when working with constructor types. Always remember to use parentheses around your constructor types when combining them in intersection types to maintain the integrity of your code and prevent errors. Understanding such syntactical rules can greatly enhance your TypeScript development experience, making your code more robust and error-free.