How to solve coding problems
Photo by Olav Ahrens Røtne on Unsplash One of the most important skills in programming and software development is problem solving. This is something that I've enjoyed learning more about over the years and that I feel will always be improving. However, as I continue trying to improve my skills on solving coding interview problems, I've decided to dedicate some time to the strategy and concepts of problem solving. Here we go! The General Strategy Most videos and articles I've come across seem to reference the approach outlined in the book "How To Solve It" by George Polya: Understand the problem Formulate a plan Execute the plan Look back (Review and Refine) Let's look at these in detail. Step 1. Understand the Problem Read it multiple times and try to define any terms you don't fully understand, to the point that you should be able to teach the problem to someone else or at least be able to fully explain it in your own words. Additionally, here are some helpful points to keep in mind during this step: What are the inputs and expected outputs? Restate the problem in simple terms (ie. "I need to convert X into Y"). If you're having trouble understanding "how" the inputs are transformed manually walk through a test case. During interviews ask lots of questions. This can help you catch any edge cases. Step 2. Formulate a Plan Using pseudocode write out each step that you'll take in detail. Don't worry about using any code languguage specific syntax during this stage since you're essentially "prototyping" your solution. Using plain every-day language focus on how you would convert the given data into the desired output. Separating the "planning/problem solving" part from the "coding" part helps you avoid mistakes and can even help when naming variables and functions later based on what that step of the plan is intended to do. Here are some additional tips to keep in mind: Break down the problem into small steps Make your own examples. The ones provided could be a trick. Feel free to draw it out if needed. If problem provides any constraints these can hint at what data structure or algorithm to use. See if you can refine your pseudocode algorithm before moving on to the implementation step. Step 3. Execute the Plan Now it's time to implement your carefully crafted plan. Do so carefully and line-by-line; don't take any shortcuts. You can also test each line (ie. console.log'ing) as you implement it to ensure you're getting the desired results and avoid costly mistakes down the road. Alex Mitchell from Expert Beacon suggests "emphasizing correctness over efficiency and clarity over conciseness" during this stage. Make sure the core logic is right before optimizing. Step 4. Look Back (Review and Refine) At this point you've implemented an algorithm that solves the problem. But you're not done yet. Here's when you can go back and look for ways to optimize your solution: Optimize for time/space complexity Are there edge cases you might've missed? Can you improve the code's readability? BONUS
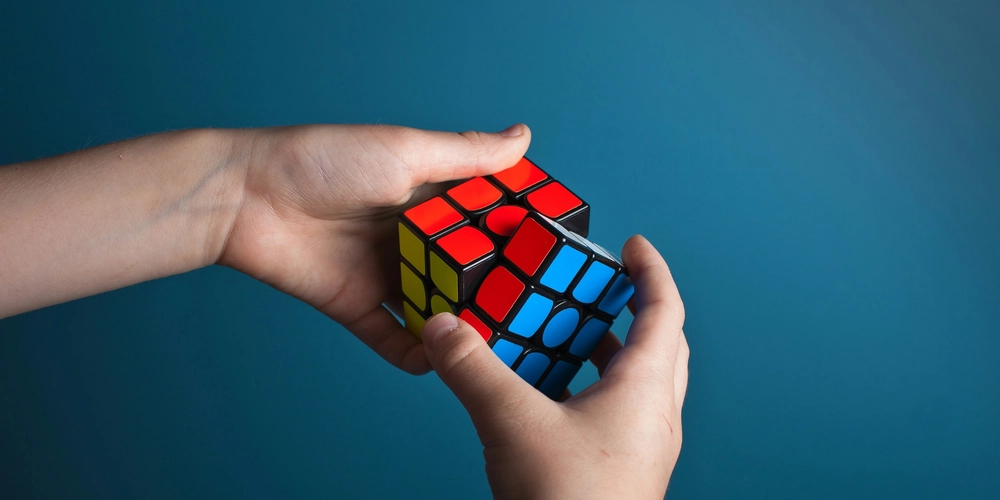
Photo by Olav Ahrens Røtne on Unsplash
One of the most important skills in programming and software development is problem solving. This is something that I've enjoyed learning more about over the years and that I feel will always be improving. However, as I continue trying to improve my skills on solving coding interview problems, I've decided to dedicate some time to the strategy and concepts of problem solving. Here we go!
The General Strategy
Most videos and articles I've come across seem to reference the approach outlined in the book "How To Solve It" by George Polya:
- Understand the problem
- Formulate a plan
- Execute the plan
- Look back (Review and Refine)
Let's look at these in detail.
Step 1. Understand the Problem
Read it multiple times and try to define any terms you don't fully understand, to the point that you should be able to teach the problem to someone else or at least be able to fully explain it in your own words. Additionally, here are some helpful points to keep in mind during this step:
- What are the inputs and expected outputs?
- Restate the problem in simple terms (ie. "I need to convert X into Y").
- If you're having trouble understanding "how" the inputs are transformed manually walk through a test case.
- During interviews ask lots of questions. This can help you catch any edge cases.
Step 2. Formulate a Plan
Using pseudocode write out each step that you'll take in detail. Don't worry about using any code languguage specific syntax during this stage since you're essentially "prototyping" your solution. Using plain every-day language focus on how you would convert the given data into the desired output. Separating the "planning/problem solving" part from the "coding" part helps you avoid mistakes and can even help when naming variables and functions later based on what that step of the plan is intended to do. Here are some additional tips to keep in mind:
- Break down the problem into small steps
- Make your own examples. The ones provided could be a trick. Feel free to draw it out if needed.
- If problem provides any constraints these can hint at what data structure or algorithm to use.
- See if you can refine your pseudocode algorithm before moving on to the implementation step.
Step 3. Execute the Plan
Now it's time to implement your carefully crafted plan. Do so carefully and line-by-line; don't take any shortcuts. You can also test each line (ie. console.log'ing) as you implement it to ensure you're getting the desired results and avoid costly mistakes down the road. Alex Mitchell from Expert Beacon suggests "emphasizing correctness over efficiency and clarity over conciseness" during this stage. Make sure the core logic is right before optimizing.
Step 4. Look Back (Review and Refine)
At this point you've implemented an algorithm that solves the problem. But you're not done yet. Here's when you can go back and look for ways to optimize your solution:
- Optimize for time/space complexity
- Are there edge cases you might've missed?
- Can you improve the code's readability?