How to Fix SDL_QueueAudio Delay When Playing Sounds?
Playing sounds in real-time applications like games is crucial for enhancing user experience. If you're experiencing roughly half a second delay each time you play a sound using SDL_QueueAudio, you're not alone. This issue often arises due to how the audio is processed and queued in SDL, which can lead to frustrating lag during gameplay. In this article, we'll explore what causes this delay and present you with effective solutions to minimize it, ensuring your application provides a smooth audio experience. Understanding the Delay in SDL Audio When you queue audio using SDL_QueueAudio, several processes take place before sound is heard. The audio data must be copied to a buffer and processed by the audio driver, which can introduce latency. This delay is especially noticeable in applications like games where immediate feedback is critical. Several factors can contribute to this delay: Buffer Size: A larger audio buffer can lead to increased latency, as more sound data is processed at once. Audio Format: The format that your sound files are in may not match what your audio device expects, causing additional processing time. Queueing Strategy: Depending on how sounds are queued and played, there could be inefficiencies causing delays. Step-by-Step Solutions to Reduce Audio Delay in SDL Let's focus on practical adjustments that can be made within your code to tackle this delay. Here are a few strategies: 1. Adjusting Buffer Size One of the simplest changes you can make is to adjust the buffer size when opening the audio device. Smaller buffer sizes can help reduce the delay: // Adjusting buffer size in SoundPrep function void SoundPrep(SDL_AudioSpec wavSpec) { const int bufferSize = 512; // Smaller buffer size for less latency soundDevice = SDL_OpenAudioDevice(NULL, 0, &wavSpec, NULL, SDL_AUDIO_ALLOW_FORMAT_CHANGE); SDL_QueueAudio(soundDevice, d->wavBuffer, d->wavLength); if (soundDevice) { soundPrepped = true; } else { printf("Cannot open sound device\n"); } } 2. Use SDL Audio Device Parameters Make sure the audio device parameters match the specifications of your audio files. This can help improve audio processing times: // Modify SoundPrep to specify preferred audio format audioSpec.freq = 44100; // Sample rate audioSpec.format = AUDIO_S16SYS; // 16-bit signed audio audioSpec.channels = 2; // Stereo SoundPrep(audioSpec); 3. Preload Sounds for Faster Access Preloading sounds essentially keeps the audio data readily accessible, which can help reduce delays when a sound is played. Instead of loading sounds in runtime, you can load them at the beginning of your program: // Load sound files in main function Sound* noise = LoadSound("Poonk.wav"); if (!noise) { printf("Error loading sound\n"); } 4. Implementing Real-Time Audio Mixing For more advanced use cases, consider implementing real-time mixing for your audio engine. This allows multiple sounds to be processed together, reducing delays further: // Example of integrating mixing with SDL_Mixer Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048); Mix_Chunk *soundEffect = Mix_LoadWAV("Poonk.wav"); Mix_PlayChannel(-1, soundEffect, 0); 5. Limiting Event Polling Interval If you have multiple sounds playing in a short time span, limiting how frequently you poll for events can smooth out performance, thereby managing audio delays: // Limiting event polling in SoundCheck function while (true) { SDL_Delay(16); // Roughly 60 FPS bool success = SDL_PollEvent(&event); if (success && event.type == SDL_KEYDOWN) { PlaySound(noise); } } Frequently Asked Questions Q: Why is there still a delay even after adjustments? A: If you continue to experience delays, consider checking the audio file format, optimizing your system performance, or testing on different hardware. Q: Can using stereo files instead of mono improve playback? A: Typically, using mono files can reduce processing time, as they require less data than stereo files. However, stereo might be more immersive depending on your game design. Conclusion Addressing audio delays in SDL using SDL_QueueAudio is critical for creating an engaging gaming experience. By implementing the techniques discussed — from adjusting buffer sizes to preloading sounds and fine-tuning event polling intervals — you can minimize the delay encountered when playing sounds. Testing these strategies on your specific system can yield varied results, so it's always beneficial to experiment with these techniques and see what fits best. Reducing audio lag not only enhances the gameplay experience but also ensures that your application runs smoothly on all platforms. Keep exploring and optimizing for better sound performance in your SDL applications!
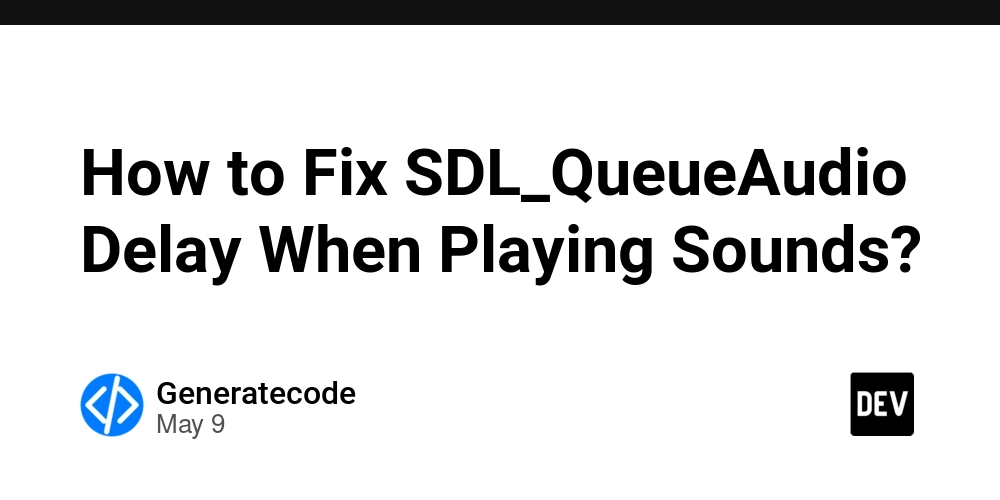
Playing sounds in real-time applications like games is crucial for enhancing user experience. If you're experiencing roughly half a second delay each time you play a sound using SDL_QueueAudio
, you're not alone. This issue often arises due to how the audio is processed and queued in SDL, which can lead to frustrating lag during gameplay. In this article, we'll explore what causes this delay and present you with effective solutions to minimize it, ensuring your application provides a smooth audio experience.
Understanding the Delay in SDL Audio
When you queue audio using SDL_QueueAudio
, several processes take place before sound is heard. The audio data must be copied to a buffer and processed by the audio driver, which can introduce latency. This delay is especially noticeable in applications like games where immediate feedback is critical. Several factors can contribute to this delay:
- Buffer Size: A larger audio buffer can lead to increased latency, as more sound data is processed at once.
- Audio Format: The format that your sound files are in may not match what your audio device expects, causing additional processing time.
- Queueing Strategy: Depending on how sounds are queued and played, there could be inefficiencies causing delays.
Step-by-Step Solutions to Reduce Audio Delay in SDL
Let's focus on practical adjustments that can be made within your code to tackle this delay. Here are a few strategies:
1. Adjusting Buffer Size
One of the simplest changes you can make is to adjust the buffer size when opening the audio device. Smaller buffer sizes can help reduce the delay:
// Adjusting buffer size in SoundPrep function
void SoundPrep(SDL_AudioSpec wavSpec) {
const int bufferSize = 512; // Smaller buffer size for less latency
soundDevice = SDL_OpenAudioDevice(NULL, 0, &wavSpec, NULL, SDL_AUDIO_ALLOW_FORMAT_CHANGE);
SDL_QueueAudio(soundDevice, d->wavBuffer, d->wavLength);
if (soundDevice) {
soundPrepped = true;
} else {
printf("Cannot open sound device\n");
}
}
2. Use SDL Audio Device Parameters
Make sure the audio device parameters match the specifications of your audio files. This can help improve audio processing times:
// Modify SoundPrep to specify preferred audio format
audioSpec.freq = 44100; // Sample rate
audioSpec.format = AUDIO_S16SYS; // 16-bit signed audio
audioSpec.channels = 2; // Stereo
SoundPrep(audioSpec);
3. Preload Sounds for Faster Access
Preloading sounds essentially keeps the audio data readily accessible, which can help reduce delays when a sound is played. Instead of loading sounds in runtime, you can load them at the beginning of your program:
// Load sound files in main function
Sound* noise = LoadSound("Poonk.wav");
if (!noise) {
printf("Error loading sound\n");
}
4. Implementing Real-Time Audio Mixing
For more advanced use cases, consider implementing real-time mixing for your audio engine. This allows multiple sounds to be processed together, reducing delays further:
// Example of integrating mixing with SDL_Mixer
Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048);
Mix_Chunk *soundEffect = Mix_LoadWAV("Poonk.wav");
Mix_PlayChannel(-1, soundEffect, 0);
5. Limiting Event Polling Interval
If you have multiple sounds playing in a short time span, limiting how frequently you poll for events can smooth out performance, thereby managing audio delays:
// Limiting event polling in SoundCheck function
while (true) {
SDL_Delay(16); // Roughly 60 FPS
bool success = SDL_PollEvent(&event);
if (success && event.type == SDL_KEYDOWN) {
PlaySound(noise);
}
}
Frequently Asked Questions
Q: Why is there still a delay even after adjustments?
A: If you continue to experience delays, consider checking the audio file format, optimizing your system performance, or testing on different hardware.
Q: Can using stereo files instead of mono improve playback?
A: Typically, using mono files can reduce processing time, as they require less data than stereo files. However, stereo might be more immersive depending on your game design.
Conclusion
Addressing audio delays in SDL using SDL_QueueAudio
is critical for creating an engaging gaming experience. By implementing the techniques discussed — from adjusting buffer sizes to preloading sounds and fine-tuning event polling intervals — you can minimize the delay encountered when playing sounds. Testing these strategies on your specific system can yield varied results, so it's always beneficial to experiment with these techniques and see what fits best.
Reducing audio lag not only enhances the gameplay experience but also ensures that your application runs smoothly on all platforms. Keep exploring and optimizing for better sound performance in your SDL applications!