# Day 4 JavaScript Practice: Beginner Exercises Cheatsheet
Welcome to Day 4 of your JavaScript learning journey! Today is all about practice — putting your basic knowledge into action with real beginner-friendly exercises. By the end of this article, you’ll be comfortable with variables, operators, conditions, loops, arrays, and basic functions. Let’s dive in! 1. Variables & Basic Math Operations Let’s start by working with variables and numbers. You'll use basic arithmetic to perform calculations. // Declare two numbers let num1 = 10; let num2 = 5; // Perform operations let sum = num1 + num2; // Addition let difference = num1 - num2; // Subtraction let product = num1 * num2; // Multiplication let quotient = num1 / num2; // Division // Output results console.log("Sum:", sum); console.log("Difference:", difference); console.log("Product:", product); console.log("Quotient:", quotient); ✅ Challenge: Try changing num1 and num2 to different values. Add a new variable for modulus (%) to get the remainder. 2. Conditions & User Input Practice Here we’ll use prompt() to get user input, and if...else to make decisions based on the input. // Ask the user for their age let age = prompt("Enter your age:"); // Use if/else to check age if(age >= 18) { console.log("✅ You are an adult."); } else { console.log("⛔ You are underage."); } // Ask for a number and check if it’s even or odd let number = prompt("Enter a number:"); if(number % 2 === 0) { console.log("The number is Even."); } else { console.log("The number is Odd."); } ✅ Challenge: Add another if block to check if age is exactly 18. Write a custom message for age below 10. 3. Loops, Arrays & Functions Now let’s get hands-on with arrays, loops, and reusable functions. // Create an array let fruits = ["Mango", "Banana", "Orange"]; // Use a loop to display all fruits for(let i = 0; i
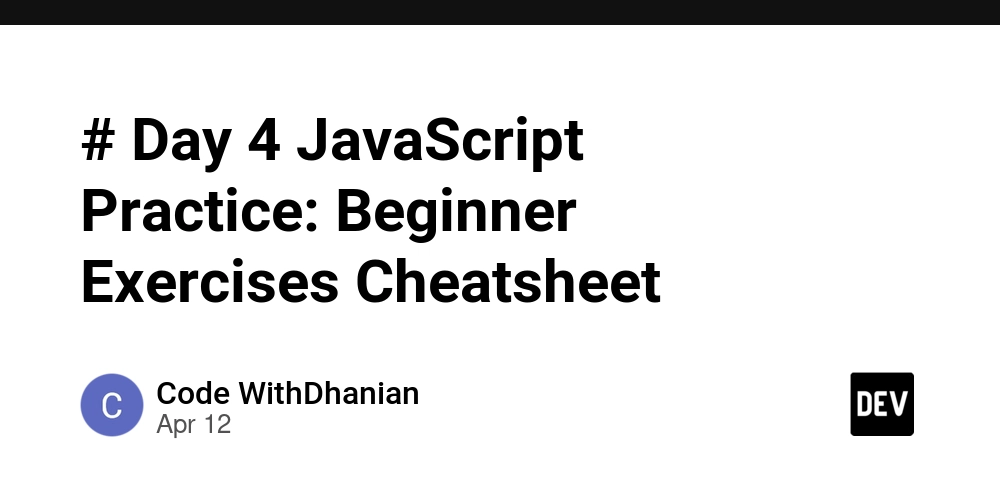
Welcome to Day 4 of your JavaScript learning journey!
Today is all about practice — putting your basic knowledge into action with real beginner-friendly exercises.
By the end of this article, you’ll be comfortable with variables, operators, conditions, loops, arrays, and basic functions.
Let’s dive in!
1. Variables & Basic Math Operations
Let’s start by working with variables and numbers. You'll use basic arithmetic to perform calculations.
// Declare two numbers
let num1 = 10;
let num2 = 5;
// Perform operations
let sum = num1 + num2; // Addition
let difference = num1 - num2; // Subtraction
let product = num1 * num2; // Multiplication
let quotient = num1 / num2; // Division
// Output results
console.log("Sum:", sum);
console.log("Difference:", difference);
console.log("Product:", product);
console.log("Quotient:", quotient);
✅ Challenge:
- Try changing
num1
andnum2
to different values. - Add a new variable for modulus (
%
) to get the remainder.
2. Conditions & User Input Practice
Here we’ll use prompt()
to get user input, and if...else
to make decisions based on the input.
// Ask the user for their age
let age = prompt("Enter your age:");
// Use if/else to check age
if(age >= 18) {
console.log("✅ You are an adult.");
} else {
console.log("⛔ You are underage.");
}
// Ask for a number and check if it’s even or odd
let number = prompt("Enter a number:");
if(number % 2 === 0) {
console.log("The number is Even.");
} else {
console.log("The number is Odd.");
}
✅ Challenge:
- Add another
if
block to check if age is exactly 18. - Write a custom message for age below 10.
3. Loops, Arrays & Functions
Now let’s get hands-on with arrays, loops, and reusable functions.
// Create an array
let fruits = ["Mango", "Banana", "Orange"];
// Use a loop to display all fruits
for(let i = 0; i < fruits.length; i++) {
console.log("