Avoiding Common Eloquent Pitfalls in Laravel Projects at Scale
When you're working with Laravel at scale, Eloquent — Laravel's beloved ORM — can be a double-edged sword. It’s expressive and elegant, but it can lead to subtle and costly performance issues if not used with caution. Over the years, I’ve worked on large Laravel applications where data integrity, response time, and system load were mission-critical. This post explores the common pitfalls I’ve encountered with Eloquent and how to avoid them. The N+1 Query Problem One of the most infamous issues is the N+1 query problem. If you've ever looped through a collection and accessed a relationship inside the loop, you’ve likely caused this. For example: $users = User::all(); foreach ($users as $user) { echo $user->profile->bio; } This results in 1 query to fetch users, and 1 query per user to fetch the profile — causing potentially hundreds of queries. The fix? Eager load the relationship: $users = User::with('profile')->get(); Use Laravel Debugbar or Clockwork to identify where this happens. Overusing toArray() and pluck() Fetching large datasets and calling toArray() or pluck() can lead to memory bloat, especially if you don’t filter the columns you need. Instead of: $users = User::all()->pluck('email'); Use: $users = User::select('email')->get()->pluck('email'); Missing Indexes Relying on Eloquent means relying on queries being generated for you — but are your columns indexed? For large tables, missing indexes on foreign keys, commonly filtered columns, or joined columns can kill performance. Use MySQL’s EXPLAIN keyword to analyze queries, and tools like Laravel Telescope to inspect slow database operations. Inappropriate Use of Relationships Eloquent relationships are powerful but sometimes unnecessary. For instance, defining hasManyThrough relationships for occasional admin views can be overkill. Consider using raw SQL or DB queries in those edge cases instead. Query Batching with Chunking If you're processing large datasets in the background (e.g., updating a status on a million records), don’t load all records at once. Use chunk(): User::chunk(1000, function ($users) { foreach ($users as $user) { $user->update(['status' => 'inactive']); } }); This approach avoids memory exhaustion and is queue-friendly. Avoiding Logic in Accessors/Mutators While accessors/mutators are elegant, putting heavy logic inside them can lead to unexpected performance bottlenecks — especially when models are serialized or when lists are transformed using them. Be mindful of what you're hiding under the hood. Use Resource Classes for API Responses Don’t pass entire models to the frontend. Use API Resource classes to sanitize and streamline your output. This ensures better control over data exposure and reduces payload size. return new UserResource($user); Conclusion Eloquent is a fantastic tool, but like every abstraction, it hides complexity. Understanding what’s happening under the hood — and where performance can break down — makes you a better Laravel developer. At scale, these differences can be the reason your app runs smoothly or crumbles under load.
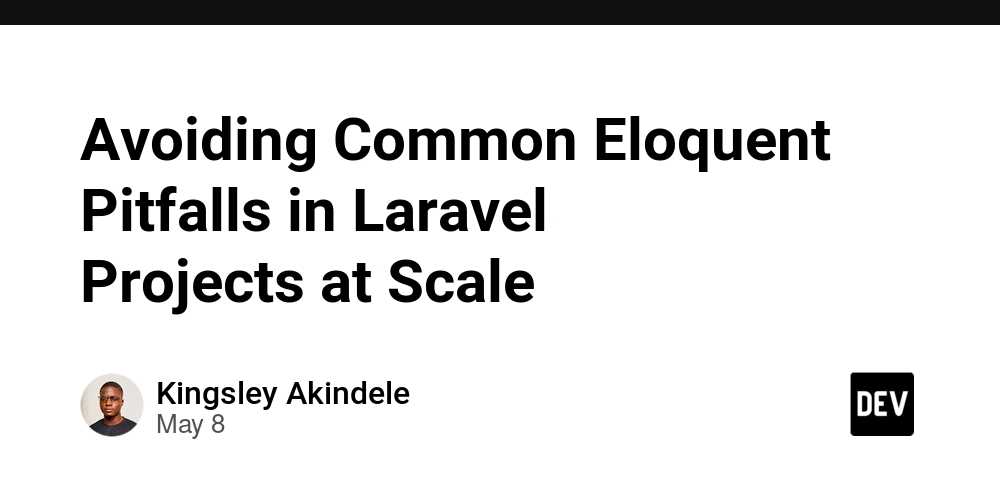
When you're working with Laravel at scale, Eloquent — Laravel's beloved ORM — can be a double-edged sword. It’s expressive and elegant, but it can lead to subtle and costly performance issues if not used with caution. Over the years, I’ve worked on large Laravel applications where data integrity, response time, and system load were mission-critical. This post explores the common pitfalls I’ve encountered with Eloquent and how to avoid them.
The N+1 Query Problem
One of the most infamous issues is the N+1 query problem. If you've ever looped through a collection and accessed a relationship inside the loop, you’ve likely caused this. For example:
$users = User::all();
foreach ($users as $user) {
echo $user->profile->bio;
}
This results in 1 query to fetch users, and 1 query per user to fetch the profile — causing potentially hundreds of queries. The fix? Eager load the relationship:
$users = User::with('profile')->get();
Use Laravel Debugbar or Clockwork to identify where this happens.
Overusing toArray() and pluck()
Fetching large datasets and calling toArray() or pluck() can lead to memory bloat, especially if you don’t filter the columns you need. Instead of:
$users = User::all()->pluck('email');
Use:
$users = User::select('email')->get()->pluck('email');
Missing Indexes
Relying on Eloquent means relying on queries being generated for you — but are your columns indexed? For large tables, missing indexes on foreign keys, commonly filtered columns, or joined columns can kill performance. Use MySQL’s EXPLAIN keyword to analyze queries, and tools like Laravel Telescope to inspect slow database operations.
Inappropriate Use of Relationships
Eloquent relationships are powerful but sometimes unnecessary. For instance, defining hasManyThrough relationships for occasional admin views can be overkill. Consider using raw SQL or DB queries in those edge cases instead.
Query Batching with Chunking
If you're processing large datasets in the background (e.g., updating a status on a million records), don’t load all records at once. Use chunk():
User::chunk(1000, function ($users) {
foreach ($users as $user) {
$user->update(['status' => 'inactive']);
}
});
This approach avoids memory exhaustion and is queue-friendly.
Avoiding Logic in Accessors/Mutators
While accessors/mutators are elegant, putting heavy logic inside them can lead to unexpected performance bottlenecks — especially when models are serialized or when lists are transformed using them. Be mindful of what you're hiding under the hood.
Use Resource Classes for API Responses
Don’t pass entire models to the frontend. Use API Resource classes to sanitize and streamline your output. This ensures better control over data exposure and reduces payload size.
return new UserResource($user);
Conclusion
Eloquent is a fantastic tool, but like every abstraction, it hides complexity. Understanding what’s happening under the hood — and where performance can break down — makes you a better Laravel developer. At scale, these differences can be the reason your app runs smoothly or crumbles under load.