Screen Orientation API for Responsive Design
Comprehensive Guide to the Screen Orientation API for Responsive Design Introduction In our increasingly mobile-centric world, the way we interact with devices has fundamentally transformed the nature of web design. Today’s responsive applications must adapt not only to a wide range of screen sizes but also to different orientations. The Screen Orientation API, introduced with the Web Platform in recent years, provides developers with the tools necessary to build dynamic, orientation-aware applications. This article will delve deeply into the Screen Orientation API, providing historical context, implementation techniques, use cases, performance considerations, and debugging strategies, making it an invaluable resource for senior developers. Historical Context The evolution of web development has been marked by a shift towards mobile-first design. With the advent of smartphones and tablets, developers began to recognize the need for responsive applications that adapt to varying viewport sizes and orientations. This movement was primarily propelled by frameworks like Bootstrap and Flexbox, as well as the introduction of CSS media queries in Level 2 of the CSS specification. However, media queries alone did not take device orientation into account. With devices able to switch orientation between landscape and portrait modes, the necessity for a more granular control over screen orientation emerged. Thus, the Screen Orientation API was developed, allowing developers to programmatically retrieve and control the viewport orientation of an application. Technical Overview of the Screen Orientation API The Screen Orientation API provides functionality to retrieve orientation information and control how an application behaves when the device's orientation changes. The API consists of the following key features: Screen Orientation Types: These include: portrait-primary and portrait-secondary: Representing the portrait orientation with a primary (home button on the bottom) and secondary (home button on the top). landscape-primary and landscape-secondary: Representing the landscape orientation with single and dual configurations. any-portrait and any-landscape: Allowing the app to adapt to any orientation in portrait or landscape mode. Properties: screen.orientation.type: Returns the current orientation type. screen.orientation.lock(): Locks the current orientation to one of the predefined types. screen.orientation.unlock(): Unlocks the screen to enable orientation changes. Event Handlers: The API provides an onchange event that notifies developers when the orientation changes. Basic Usage Example Here’s a simple example that demonstrates how to implement the Screen Orientation API in a web application: if ('orientation' in screen) { document.addEventListener('DOMContentLoaded', () => { // Get the current orientation const setOrientation = () => { const orientation = screen.orientation.type; console.log(`Current screen orientation: ${orientation}`); }; // Set orientation change event listener screen.orientation.addEventListener('change', setOrientation); // Lock screen orientation to portrait screen.orientation.lock('portrait').then(() => { console.log('Orientation locked to portrait'); }).catch((error) => { console.error('Failed to lock orientation:', error); }); }); } Handling Orientation Changes In real-world applications, handling orientation changes smoothly is crucial, especially for media applications or games: function updateLayoutForOrientation() { const orientation = screen.orientation.type; if (orientation.includes('portrait')) { // Apply portrait-specific styles or layout document.body.classList.add('portrait'); document.body.classList.remove('landscape'); } else { // Apply landscape-specific styles or layout document.body.classList.add('landscape'); document.body.classList.remove('portrait'); } } // Event listener for orientation change screen.orientation.addEventListener('change', updateLayoutForOrientation); updateLayoutForOrientation(); // Initial call Advanced Implementation: Locking Orientation Based on User Input A more advanced implementation requires developers to lock orientation based on user specifications. Here’s an example that locks the orientation to landscape only when a user clicks a button: Lock to Landscape Unlock Orientation document.getElementById('landscape').addEventListener('click', () => { screen.orientation.lock('landscape').catch((error) => { console.error('Error locking orientation:', error); }); }); document.getElementById('unlock').addEventListener('click', () => { screen.orientation.unlock().then(() => {
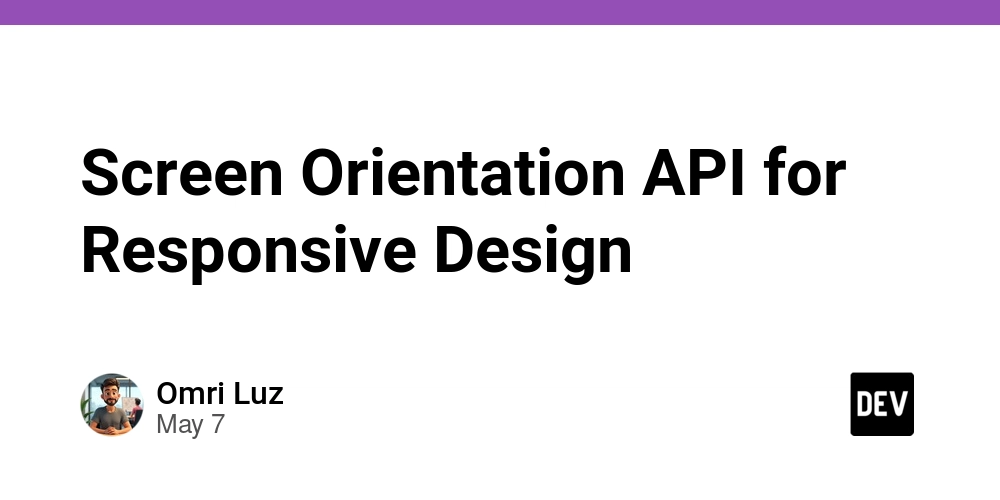
Comprehensive Guide to the Screen Orientation API for Responsive Design
Introduction
In our increasingly mobile-centric world, the way we interact with devices has fundamentally transformed the nature of web design. Today’s responsive applications must adapt not only to a wide range of screen sizes but also to different orientations. The Screen Orientation API, introduced with the Web Platform in recent years, provides developers with the tools necessary to build dynamic, orientation-aware applications. This article will delve deeply into the Screen Orientation API, providing historical context, implementation techniques, use cases, performance considerations, and debugging strategies, making it an invaluable resource for senior developers.
Historical Context
The evolution of web development has been marked by a shift towards mobile-first design. With the advent of smartphones and tablets, developers began to recognize the need for responsive applications that adapt to varying viewport sizes and orientations. This movement was primarily propelled by frameworks like Bootstrap and Flexbox, as well as the introduction of CSS media queries in Level 2 of the CSS specification.
However, media queries alone did not take device orientation into account. With devices able to switch orientation between landscape and portrait modes, the necessity for a more granular control over screen orientation emerged. Thus, the Screen Orientation API was developed, allowing developers to programmatically retrieve and control the viewport orientation of an application.
Technical Overview of the Screen Orientation API
The Screen Orientation API provides functionality to retrieve orientation information and control how an application behaves when the device's orientation changes. The API consists of the following key features:
-
Screen Orientation Types: These include:
-
portrait-primary
andportrait-secondary
: Representing the portrait orientation with a primary (home button on the bottom) and secondary (home button on the top). -
landscape-primary
andlandscape-secondary
: Representing the landscape orientation with single and dual configurations. -
any-portrait
andany-landscape
: Allowing the app to adapt to any orientation in portrait or landscape mode.
-
-
Properties:
-
screen.orientation.type
: Returns the current orientation type. -
screen.orientation.lock()
: Locks the current orientation to one of the predefined types. -
screen.orientation.unlock()
: Unlocks the screen to enable orientation changes.
-
Event Handlers: The API provides an
onchange
event that notifies developers when the orientation changes.
Basic Usage Example
Here’s a simple example that demonstrates how to implement the Screen Orientation API in a web application:
if ('orientation' in screen) {
document.addEventListener('DOMContentLoaded', () => {
// Get the current orientation
const setOrientation = () => {
const orientation = screen.orientation.type;
console.log(`Current screen orientation: ${orientation}`);
};
// Set orientation change event listener
screen.orientation.addEventListener('change', setOrientation);
// Lock screen orientation to portrait
screen.orientation.lock('portrait').then(() => {
console.log('Orientation locked to portrait');
}).catch((error) => {
console.error('Failed to lock orientation:', error);
});
});
}
Handling Orientation Changes
In real-world applications, handling orientation changes smoothly is crucial, especially for media applications or games:
function updateLayoutForOrientation() {
const orientation = screen.orientation.type;
if (orientation.includes('portrait')) {
// Apply portrait-specific styles or layout
document.body.classList.add('portrait');
document.body.classList.remove('landscape');
} else {
// Apply landscape-specific styles or layout
document.body.classList.add('landscape');
document.body.classList.remove('portrait');
}
}
// Event listener for orientation change
screen.orientation.addEventListener('change', updateLayoutForOrientation);
updateLayoutForOrientation(); // Initial call
Advanced Implementation: Locking Orientation Based on User Input
A more advanced implementation requires developers to lock orientation based on user specifications. Here’s an example that locks the orientation to landscape only when a user clicks a button:
document.getElementById('landscape').addEventListener('click', () => {
screen.orientation.lock('landscape').catch((error) => {
console.error('Error locking orientation:', error);
});
});
document.getElementById('unlock').addEventListener('click', () => {
screen.orientation.unlock().then(() => {
console.log('Screen orientation unlocked');
}).catch((error) => {
console.error('Error unlocking orientation:', error);
});
});
Edge Cases and Advanced Techniques
Several edge cases can arise when implementing the Screen Orientation API:
User Permissions: Some browsers may require explicit user permissions to lock orientations. Always check for support and handle rejections gracefully.
Browser Compatibility: While most modern browsers support the Screen Orientation API, it's essential to have fallbacks for browsers that do not. Always check for support before using the API:
if (!screen.orientation || typeof screen.orientation.lock !== 'function') {
console.warn('Screen Orientation API not supported');
}
- Dynamic Components: When working with dynamic content (like iframes or components loaded via JavaScript), make sure the handling of orientation updates properly reflects changes across scopes.
Performance Considerations
When utilizing the Screen Orientation API in complex applications, performance should be a primary concern. Here are some strategies:
- Debounce Orientation Change Events: When the orientation changes, this can trigger a number of layout adjustments. Debouncing these events can prevent performance bottlenecks.
let debounceTimer;
screen.orientation.addEventListener('change', () => {
clearTimeout(debounceTimer);
debounceTimer = setTimeout(updateLayoutForOrientation, 100);
});
- Minimize Reflows: Whenever an orientation change occurs, avoid excessive DOM manipulations that can lead to layout reflows. Batch updates and apply styles conditionally.
Real-World Use Cases
Games: Mobile games often utilize the Screen Orientation API to lock the orientation for the best user experience.
Video Applications: Streaming applications can lock orientation to landscape for watching videos, providing a more immersive experience.
Photography Apps: Apps like Instagram utilize this API to ensure that the orientation matches the way users hold the device when capturing photos.
Comparison with Alternative Approaches
Before the Screen Orientation API, developers often relied purely on CSS media queries or JavaScript event listeners to detect orientation changes. Here are some key differences:
- Control: The Screen Orientation API allows for explicit control over the orientation, whereas CSS media queries can only provide styles based on current orientation.
- JavaScript Efficiency: In scenarios where multiple elements adapt to changes in orientation, having a dedicated API improves performance over raw CSS media queries that might trigger multiple reflows.
Debugging Techniques
Debugging issues related to the Screen Orientation API requires observing the behavior of the API across different devices and browsers. Here are some advanced strategies:
- Console Logging: Use detailed console logs to identify the order of operations when orientation changes.
- Browser Developer Tools: Inspect elements as they change styles, watching out for layout shifts.
- Network Throttling: Simulate different devices and orientations with throttled network conditions to study application responsiveness.
Conclusion
The Screen Orientation API is a powerful tool that aids in creating responsive and immersive web applications for mobile devices. By understanding its intricacies, leveraging its features, and employing best practices, developers can craft applications that adapt dynamically to user inputs and device behavior.
References
For further reading and advanced topics regarding the Screen Orientation API, consider consulting:
- MDN Web Docs: Screen Orientation API
- W3C Screen Orientation Specification
- CSS Tricks: Responsive Design
This comprehensive exploration of the Screen Orientation API highlights its capabilities and practical implications, cementing its importance in modern web development. As devices continue to evolve, so too will the tools that aid in crafting exemplary user experiences.