AbortController and Signal Handling
AbortController and Signal Handling in JavaScript 1. Historical and Technical Context The introduction of the AbortController API in the Fetch Standard was a response to the evolving needs for better control over network requests in web applications. Historically, web APIs, especially the Fetch API introduced in 2015, lacked mechanisms to cancel ongoing requests. In the early days of AJAX and XMLHttpRequest, developers either had to work with timeouts or manually manage the state of requests, leading to complex and often unmanageable code bases. The AbortController was introduced in the JavaScript API as part of the broader effort to bring more robust signal handling capabilities to developers. By providing an interoperable way to communicate with tasks running in the web environment, it enables developers to abort requests and effectively manage asynchronous operations. Overview The AbortController API consists of two main components: AbortController: An interface for creating abort signals that can be shared with one or more tasks. AbortSignal: A signal that notifies when an operation has been aborted. Prior to these APIs, developers relied on cumbersome techniques to manage cancellations. Libraries like jQuery implemented custom mechanisms for task management, which, while functional, were not standardized. 2. How AbortController Works The key classes involved in this paradigm include: AbortController: It is used to create an instance that is connected to an AbortSignal. Once an AbortController is created, you can call its abort() method to signal that the associated tasks should be canceled. AbortSignal: The signal instance provides an aborted property and an onabort event handler to react to abort events. Basic Example Here's a simple example demonstrating the basic use of the AbortController with the Fetch API: const controller = new AbortController(); const { signal } = controller; fetch('https://jsonplaceholder.typicode.com/posts', { signal }) .then(response => response.json()) .then(data => { console.log(data); }) .catch(err => { if (err.name === 'AbortError') { console.error('Fetch aborted'); } else { console.error('Fetch error:', err); } }); // Manually aborting the request after 1 second setTimeout(() => { controller.abort(); }, 1000); In this example, the AbortController allows us to abort the fetch request if it takes longer than one second. 3. Advanced Scenarios Multiple Requests Cancellation Consider a scenario where you have multiple requests but you want to cancel all of them when one of them succeeds. const controller = new AbortController(); const signal = controller.signal; const fetchPost = async (id) => { try { const response = await fetch(`https://jsonplaceholder.typicode.com/posts/${id}`, { signal }); const data = await response.json(); console.log(data); // Abort all other pending requests if this one is successful controller.abort(); } catch (err) { if (err.name === 'AbortError') { console.error(`Fetch for post ${id} aborted`); } } }; // Fetching multiple posts for (let id = 1; id setTimeout(resolve, 100)); // Simulating delay console.log(post); } } const controller = new AbortController(); const signal = controller.signal; // Fetching posts and aborting after 500ms setTimeout(() => { controller.abort(); }, 500); fetchAllPosts(signal) .catch((err) => { if (err.name === 'AbortError') { console.log('Fetch operation aborted'); } }); 4. Edge Cases and Advanced Implementation Techniques While simple implementations are straightforward, developers need to consider several edge cases: Signal: The signal must be passed to every fetch request; developers must ensure that they don't inadvertently create a new controller for a single operation that could cause other tasks to lose their reference. State Management: If using multiple controllers, be cautious about the state across various concurrent operations. Properly managing controller references is crucial to avoid unintentional abort behavior. Using Custom Event Handlers You can also extend functionality with custom events for more complex scenarios: const controller = new AbortController(); const signal = controller.signal; signal.addEventListener('abort', () => { console.log('Fetch has been aborted.'); }); fetch('https://jsonplaceholder.typicode.com/posts', { signal }) .then(response => response.json()) .then(data => { console.log('Data:', data); }) .catch(err => { if (err.name === 'AbortError') { console.log('The request was aborted'); } }); // Abort the request controller.abort(); This allows for more modular and reusable code by handling aborts in a dedicated function. 5. Comparing Alternatives XMLHttpRequest Before the Fetc
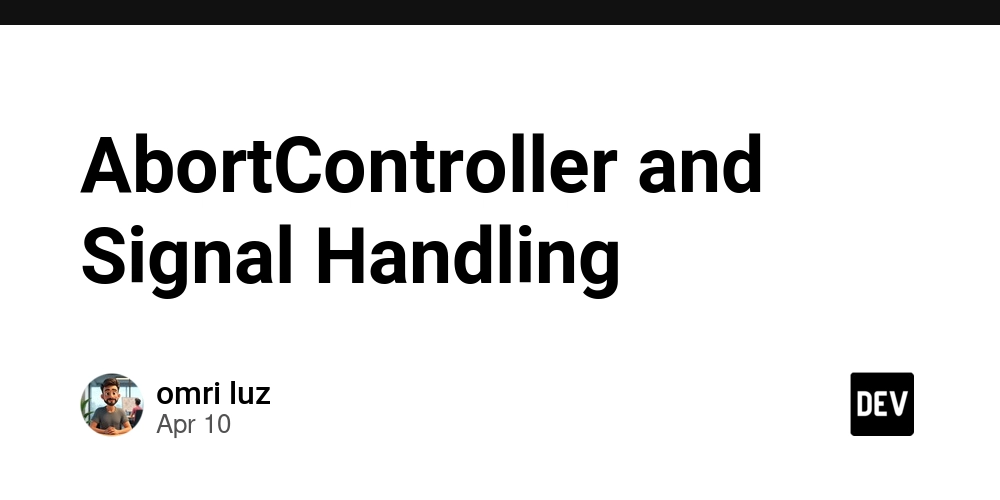
AbortController and Signal Handling in JavaScript
1. Historical and Technical Context
The introduction of the AbortController
API in the Fetch Standard was a response to the evolving needs for better control over network requests in web applications. Historically, web APIs, especially the Fetch API introduced in 2015, lacked mechanisms to cancel ongoing requests. In the early days of AJAX and XMLHttpRequest
, developers either had to work with timeouts or manually manage the state of requests, leading to complex and often unmanageable code bases.
The AbortController
was introduced in the JavaScript API as part of the broader effort to bring more robust signal handling capabilities to developers. By providing an interoperable way to communicate with tasks running in the web environment, it enables developers to abort requests and effectively manage asynchronous operations.
Overview
The AbortController
API consists of two main components:
- AbortController: An interface for creating abort signals that can be shared with one or more tasks.
- AbortSignal: A signal that notifies when an operation has been aborted.
Prior to these APIs, developers relied on cumbersome techniques to manage cancellations. Libraries like jQuery implemented custom mechanisms for task management, which, while functional, were not standardized.
2. How AbortController
Works
The key classes involved in this paradigm include:
AbortController: It is used to create an instance that is connected to an
AbortSignal
. Once anAbortController
is created, you can call itsabort()
method to signal that the associated tasks should be canceled.AbortSignal: The signal instance provides an
aborted
property and anonabort
event handler to react to abort events.
Basic Example
Here's a simple example demonstrating the basic use of the AbortController
with the Fetch API:
const controller = new AbortController();
const { signal } = controller;
fetch('https://jsonplaceholder.typicode.com/posts', { signal })
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(err => {
if (err.name === 'AbortError') {
console.error('Fetch aborted');
} else {
console.error('Fetch error:', err);
}
});
// Manually aborting the request after 1 second
setTimeout(() => {
controller.abort();
}, 1000);
In this example, the AbortController
allows us to abort the fetch request if it takes longer than one second.
3. Advanced Scenarios
Multiple Requests Cancellation
Consider a scenario where you have multiple requests but you want to cancel all of them when one of them succeeds.
const controller = new AbortController();
const signal = controller.signal;
const fetchPost = async (id) => {
try {
const response = await fetch(`https://jsonplaceholder.typicode.com/posts/${id}`, { signal });
const data = await response.json();
console.log(data);
// Abort all other pending requests if this one is successful
controller.abort();
} catch (err) {
if (err.name === 'AbortError') {
console.error(`Fetch for post ${id} aborted`);
}
}
};
// Fetching multiple posts
for (let id = 1; id <= 5; id++) {
fetchPost(id);
}
With Asynchronous Iterators
AbortController
can also be integrated with asynchronous iterators, providing cancellation in a stream of asynchronous operations.
async function fetchAllPosts(signal) {
const response = await fetch('https://jsonplaceholder.typicode.com/posts', { signal });
const data = await response.json();
for (const post of data) {
await new Promise((resolve) => setTimeout(resolve, 100)); // Simulating delay
console.log(post);
}
}
const controller = new AbortController();
const signal = controller.signal;
// Fetching posts and aborting after 500ms
setTimeout(() => {
controller.abort();
}, 500);
fetchAllPosts(signal)
.catch((err) => {
if (err.name === 'AbortError') {
console.log('Fetch operation aborted');
}
});
4. Edge Cases and Advanced Implementation Techniques
While simple implementations are straightforward, developers need to consider several edge cases:
Signal: The
signal
must be passed to every fetch request; developers must ensure that they don't inadvertently create a new controller for a single operation that could cause other tasks to lose their reference.State Management: If using multiple controllers, be cautious about the state across various concurrent operations. Properly managing controller references is crucial to avoid unintentional abort behavior.
Using Custom Event Handlers
You can also extend functionality with custom events for more complex scenarios:
const controller = new AbortController();
const signal = controller.signal;
signal.addEventListener('abort', () => {
console.log('Fetch has been aborted.');
});
fetch('https://jsonplaceholder.typicode.com/posts', { signal })
.then(response => response.json())
.then(data => {
console.log('Data:', data);
})
.catch(err => {
if (err.name === 'AbortError') {
console.log('The request was aborted');
}
});
// Abort the request
controller.abort();
This allows for more modular and reusable code by handling aborts in a dedicated function.
5. Comparing Alternatives
XMLHttpRequest
Before the Fetch API, developers mainly used XMLHttpRequest
. While it supports cancellation, it is less intuitive and requires more boilerplate. AbortController
, along with the Fetch API, simplifies the API surface area and reduces the cognitive load on developers.
Promises and Async/Await
While using Promises or async functions, nested cancellation may require sophisticated patterns through chaining. The AbortController
approach centralizes the cancellation logic and improves readability and maintainability.
RxJS or Other Libraries
For applications that require reactive programming, libraries like RxJS allow cancellation but can introduce additional complexity due to their abstractions. AbortController
is native and thus simpler for those unfamiliar with reactive paradigms.
6. Real-World Use Cases
User Input Contexts
In applications where user input triggers network requests (e.g., typing in a search bar), using AbortController
reduces unnecessary network load and improves application responsiveness.
let controller;
const searchInput = document.getElementById('search');
searchInput.addEventListener('input', async (event) => {
if (controller) {
controller.abort(); // Cancel the previous request
}
controller = new AbortController();
const signal = controller.signal;
try {
const response = await fetch(`https://api.example.com/search?q=${event.target.value}`, { signal });
const data = await response.json();
console.log(data);
} catch (err) {
if (err.name === 'AbortError') {
console.log('Previous request canceled to improve user experience');
}
}
});
Application Performance
Using AbortController
to cancel unnecessary requests can lead to improved performance metrics in web applications. Reduce the number of active network connections by effectively managing requests can allow for better resource allocation and enhanced user experiences.
7. Performance Considerations and Optimization Strategies
Minimizing Memory Leaks
Ensure that listeners added via addEventListener
are also removed when they no longer need to be active. Memory leaks due to lingering references can degrade performance, particularly in long-running applications.
Throttling Requests
In scenarios with rapid user input, implementing a throttling mechanism can alleviate immediate request overload and work subtly with the AbortController
. This would prevent launching a fetch operation on every keystroke:
let timeoutId;
searchInput.addEventListener('input', (event) => {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
// Trigger your fetch here
}, 300); // Adjust delay as necessary
});
8. Debugging Techniques for Advanced Scenarios
Logging and Monitoring
Integrate extensive logging around abort events—this can be essential in debugging both application behavior and potential performance hurdles. Use tools like Sentry or similar for monitoring.
DevTools
Utilize browser developer tools to inspect network activity. By watching the Network tab, you can identify when requests are being aborted and the associated circumstances leading to them.
Unit Tests
Implement unit tests for your cancellation logic, especially when handling multiple requests. Mocking AbortController
behavior can assist in building a robust test suite around your user interactions.
Performance Profiling
Use performance profiling tools to measure the impact of request cancellation on application runtime. Monitoring interaction times before and after restructuring code with AbortController
allows for concrete data representation of its efficiency.
9. Conclusion
The AbortController
brings a powerful paradigm shift to managing asynchronous tasks in JavaScript. It simplifies cancellation management and provides developers with a more straightforward API for handling complex asynchronous behaviors. By understanding its intricacies and potential pitfalls, combined with solid performance strategies, developers can create applications that are not only more responsive but also better at managing resources effectively.
Incorporating real-world scenarios and advanced optimizations, this guide provides a comprehensive approach to mastering AbortController
and signal handling. For further reading, consult the official MDN documentation and the Fetch API resources to deepen your understanding and mastery of these advanced JavaScript techniques.