Selenium vs Puppeteer vs Playwright: Choosing the Right Tool for Web Automation
In today's fast-paced world of web development, automation has become essential for tasks like testing, web scraping, and performance monitoring. With several powerful tools available, choosing the right one can be overwhelming. This blog post will delve into the differences between three popular web automation frameworks: Selenium, Puppeteer, and Playwright, to help you make an informed decision. Selenium: The Veteran Selenium is the oldest and most established player in the web automation field. Initially conceived in 2004 by Jason Huggins, it has evolved into a comprehensive suite of tools with a large and active community. Selenium supports a wide range of browsers and programming languages, making it a versatile choice for cross-browser testing and web scraping. One of Selenium's greatest strengths is its ability to support a wide range of browsers and operating systems. This makes it an ideal choice for ensuring that web applications are compatible with different environments and provide a consistent user experience across various platforms. Key Features of Selenium: Cross-browser compatibility: Selenium supports all major browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer. Multi-language support: You can write Selenium scripts in various programming languages like Java, Python, C#, Ruby, JavaScript, and more. Selenium WebDriver: This powerful component provides a programming interface to interact with web elements, simulate user actions, and perform assertions. Selenium Grid: Enables parallel test execution across different browsers and machines, significantly speeding up testing. Selenium Grid allows for running tests in parallel on multiple machines and managing different browser versions. This is particularly useful for large-scale testing and for reducing the overall testing time. Open-source and free: Selenium is a community-driven project, making it accessible and cost-effective. Use Cases of Selenium: Automated testing: Selenium is widely used for automating functional and regression tests for web applications. Cross-browser compatibility testing: Ensure your web application works seamlessly across different browsers and versions. Web scraping: Extract data from websites that don't offer APIs. Data migration: Automate data transfer between web applications. Code Example: Navigating to a Webpage with Selenium import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; import org.testng.annotations.Test; public class BrowserStackDemo { WebDriver driver; @Test public void verifyTitle() { driver = new ChromeDriver(); driver.get("https://www.browserstack.com/"); Assert.assertEquals(driver.getTitle(), "Most Reliable App & Cross Browser Testing Platform | BrowserStack"); driver.quit(); } } This code snippet demonstrates how to initialize a ChromeDriver, navigate to a URL, and verify the page title using Selenium with Java. Puppeteer: The Chrome Specialist Puppeteer, a Node.js library developed by the Google Chrome team, provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It excels in tasks specifically designed for Chrome and Chromium, offering excellent performance and deep integration with Chrome's DevTools. While Puppeteer has a strong focus on Chrome, it also supports Firefox, expanding its capabilities beyond just Chrome-based automation. Puppeteer also supports cross-browser and cross-platform testing, making it compatible with a variety of operating systems and programming languages. This allows developers to use Puppeteer for testing web applications across different environments. Key Features of Puppeteer: Headless browsing: Run Chrome or Chromium in headless mode (without a graphical user interface) for faster execution and efficient resource utilization. Chrome DevTools integration: Provides fine-grained control over Chrome's features and functionalities. Easy setup and configuration: Puppeteer is relatively easy to set up and use, especially for developers familiar with JavaScript. Performance-oriented: Puppeteer is known for its speed and efficiency in Chrome environments. Screenshot and PDF generation: Capture screenshots and generate PDFs of web pages with ease. Timeline capture: Puppeteer can capture timeline traces of your site to help diagnose performance issues. This allows developers to analyze website performance and identify areas for improvement. Use Cases of Puppeteer: Web scraping: Extract data from websites, including single-page applications (SPAs) that rely heavily on JavaScript. UI testing: Automate form submissions, keyboard input, and other UI interactions. Performance testing: Capture timeline traces and analyze website performance metrics. SEO testing: Analyze how web pa
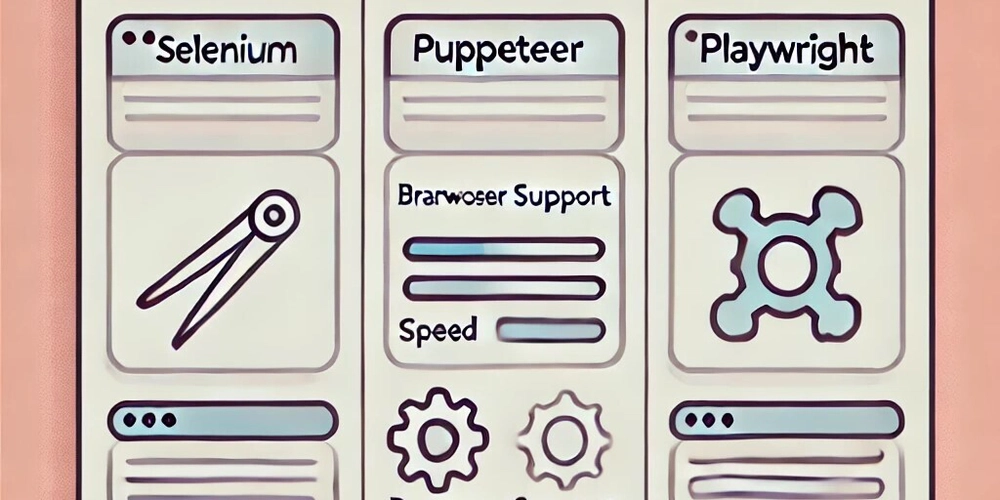
In today's fast-paced world of web development, automation has become essential for tasks like testing, web scraping, and performance monitoring. With several powerful tools available, choosing the right one can be overwhelming. This blog post will delve into the differences between three popular web automation frameworks: Selenium, Puppeteer, and Playwright, to help you make an informed decision.
Selenium: The Veteran
Selenium is the oldest and most established player in the web automation field. Initially conceived in 2004 by Jason Huggins, it has evolved into a comprehensive suite of tools with a large and active community. Selenium supports a wide range of browsers and programming languages, making it a versatile choice for cross-browser testing and web scraping.
One of Selenium's greatest strengths is its ability to support a wide range of browsers and operating systems. This makes it an ideal choice for ensuring that web applications are compatible with different environments and provide a consistent user experience across various platforms.
Key Features of Selenium:
Cross-browser compatibility: Selenium supports all major browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer.
Multi-language support: You can write Selenium scripts in various programming languages like Java, Python, C#, Ruby, JavaScript, and more.
Selenium WebDriver: This powerful component provides a programming interface to interact with web elements, simulate user actions, and perform assertions.
Selenium Grid: Enables parallel test execution across different browsers and machines, significantly speeding up testing. Selenium Grid allows for running tests in parallel on multiple machines and managing different browser versions. This is particularly useful for large-scale testing and for reducing the overall testing time.
Open-source and free: Selenium is a community-driven project, making it accessible and cost-effective.
Use Cases of Selenium:
Automated testing: Selenium is widely used for automating functional and regression tests for web applications.
Cross-browser compatibility testing: Ensure your web application works seamlessly across different browsers and versions.
Web scraping: Extract data from websites that don't offer APIs.
Data migration: Automate data transfer between web applications.
Code Example: Navigating to a Webpage with Selenium
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.Test;
public class BrowserStackDemo {
WebDriver driver;
@Test
public void verifyTitle() {
driver = new ChromeDriver();
driver.get("https://www.browserstack.com/");
Assert.assertEquals(driver.getTitle(), "Most Reliable App & Cross Browser Testing Platform | BrowserStack");
driver.quit();
}
}
This code snippet demonstrates how to initialize a ChromeDriver, navigate to a URL, and verify the page title using Selenium with Java.
Puppeteer: The Chrome Specialist
Puppeteer, a Node.js library developed by the Google Chrome team, provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It excels in tasks specifically designed for Chrome and Chromium, offering excellent performance and deep integration with Chrome's DevTools. While Puppeteer has a strong focus on Chrome, it also supports Firefox, expanding its capabilities beyond just Chrome-based automation.
Puppeteer also supports cross-browser and cross-platform testing, making it compatible with a variety of operating systems and programming languages. This allows developers to use Puppeteer for testing web applications across different environments.
Key Features of Puppeteer:
Headless browsing: Run Chrome or Chromium in headless mode (without a graphical user interface) for faster execution and efficient resource utilization.
Chrome DevTools integration: Provides fine-grained control over Chrome's features and functionalities.
Easy setup and configuration: Puppeteer is relatively easy to set up and use, especially for developers familiar with JavaScript.
Performance-oriented: Puppeteer is known for its speed and efficiency in Chrome environments.
Screenshot and PDF generation: Capture screenshots and generate PDFs of web pages with ease.
Timeline capture: Puppeteer can capture timeline traces of your site to help diagnose performance issues. This allows developers to analyze website performance and identify areas for improvement.
Use Cases of Puppeteer:
Web scraping: Extract data from websites, including single-page applications (SPAs) that rely heavily on JavaScript.
UI testing: Automate form submissions, keyboard input, and other UI interactions.
Performance testing: Capture timeline traces and analyze website performance metrics.
SEO testing: Analyze how web pages are rendered and indexed by search engines.
Code Example: Navigating to a Webpage with Puppeteer
const puppeteer = require('puppeteer');
puppeteer.launch().then(async browser => {
const page = await browser.newPage();
await page.setViewport({ width: 1280, height: 800 })
await page.goto('https://www.google.com/');
await page.screenshot({ path: 'myscreenshot.png', fullPage: true });
await browser.close();
});
This code snippet demonstrates how to launch a headless Chrome instance, navigate to a URL, and take a screenshot using Puppeteer.
Playwright: The Modern Contender
Playwright, a relatively new framework developed by Microsoft, builds upon the strengths of Puppeteer and expands its capabilities. It offers cross-browser support, including Chrome, Firefox, and WebKit, with a single API, making it a strong contender in the web automation landscape. Playwright was developed by the same team that created Puppeteer, but they have since moved from Google to Microsoft. This shared heritage is evident in Playwright's architecture and features, but Playwright distinguishes itself with its broader browser support and focus on modern web application testing.
Playwright's architecture is designed to address some of the limitations of Selenium and Puppeteer. It uses a WebSocket connection instead of the WebDriver API and HTTP, which allows for faster execution speeds and more reliable test execution.
Key Features of Playwright:
Cross-browser support: Playwright supports all modern rendering engines, including Chromium, WebKit, and Firefox.
Multi-language support: Provides APIs for JavaScript, TypeScript, Python, .NET, and Java.
Auto-wait: Playwright automatically waits for elements to be actionable before performing actions, reducing test flakiness. This feature eliminates the need for manual waits and makes tests more reliable by ensuring that elements are in the expected state before interactions occur.
Browser contexts: Create isolated browser contexts for each test, ensuring full test isolation and preventing interference.
Powerful tooling: Playwright offers tools like Codegen, Playwright Inspector, and Trace Viewer for test generation, debugging, and analysis.
One-time login: Playwright allows you to save the authentication state of a browser context and reuse it in multiple tests, saving time and effort.
Iframe and shadow DOM handling: Playwright can seamlessly handle iframes and shadow DOMs, making it easier to interact with complex web applications.
Use Cases of Playwright:
End-to-end testing: Test user flows across different browsers with the same script.
Visual regression testing: Compare screenshots over time to detect UI changes.
Mobile web testing: Simulate mobile environments within browsers to test responsive designs.
API testing: Playwright can also be used for API testing, expanding its capabilities beyond UI automation.
Code Example: Navigating to a Webpage with Playwright
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://www.example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
This code snippet demonstrates how to launch a Chromium browser, navigate to a URL, and take a screenshot using Playwright.
Head-to-Head Comparison
Feature | Selenium | Puppeteer | Playwright |
---|---|---|---|
Browser Support | All major browsers | Chrome and Firefox | Chrome, Firefox, and WebKit |
Language Support | Java, Python, C#, Ruby, JavaScript, and more | Primarily JavaScript | JavaScript, TypeScript, Python, .NET, Java |
Performance | Can be slower | Fast in Chrome environments | Fast and efficient |
Setup | Can be complex | Easy | Easy |
Debugging | Requires external tools | Integrated with Chrome DevTools | Powerful debugging tools |
Community | Large and active | Growing | Growing |
Ease of Use | Can be complex | Relatively easy | Easy |
Ideal for | Cross-browser testing, legacy browser support | Chrome-specific tasks, performance testing | Modern web apps, end-to-end testing |
Choosing the Right Tool
Criteria | Selenium | Puppeteer | Playwright |
---|---|---|---|
Cross-browser compatibility | Best choice if you need to test across a wide range of browsers, including older versions like Internet Explorer. | Limited to Chrome and Firefox. | Supports Chrome, Firefox, and WebKit, covering most modern browsers. |
Language support | Offers the widest range of language options. | Primarily focused on JavaScript. | Supports multiple languages, including JavaScript, TypeScript, Python, .NET, and Java. |
Performance | Can be slower compared to Puppeteer and Playwright. | Excellent performance in Chrome environments. | Generally fast and efficient across all supported browsers. |
Ease of use | Can have a steeper learning curve, especially for beginners. | Relatively easy to set up and use, especially for JavaScript developers. | Easy to learn and use, with a user-friendly API. |
Project requirements | Ideal for projects that require extensive cross-browser testing and support for legacy browsers. | Well-suited for projects focused on Chrome or Chromium, such as performance testing and web scraping. | A good all-around choice for modern web applications, especially those with complex interactions and dynamic content. |
Ultimately, the best tool for you will depend on your specific needs and priorities. Consider the factors listed above and choose the framework that best aligns with your project requirements and technical expertise.