Method Overloading
Method Overloading in Java Method overloading allows a class to have multiple methods with the same name but different parameters. It is a way to achieve compile-time polymorphism. Key Points: Same method name, but different: Number of parameters Types of parameters Order of parameters Return type alone cannot differentiate overloaded methods. Can be done within the same class or between parent and child classes. Compile-time (static) polymorphism – the correct method is chosen at compile time. Examples of Method Overloading 1. Different Number of Parameters class Calculator { int add(int a, int b) { return a + b; } int add(int a, int b, int c) { // Overloaded method return a + b + c; } } 2. Different Data Types class Printer { void print(int num) { System.out.println("Printing int: " + num); } void print(String text) { // Overloaded method System.out.println("Printing string: " + text); } } 3. Different Order of Parameters class Display { void show(int a, double b) { System.out.println("int first, then double"); } void show(double a, int b) { // Overloaded method System.out.println("double first, then int"); } } 4. Invalid Overloading (Return Type Alone is Not Enough) ❌ Compile Error – Ambiguous method call. int multiply(int a, int b) { return a * b; } double multiply(int a, int b) { return a * b; } // ❌ Not valid overloading Why Use Method Overloading? Improves code readability (same method name for similar actions). Allows flexibility in method calls. Avoids complex method names like addTwoNumbers(), addThreeNumbers(). Method Overloading vs. Method Overriding Feature Method Overloading Method Overriding Definition Same name, different parameters Same name & parameters in subclass Polymorphism Compile-time Runtime (dynamic) Inheritance Not required Required (subclass overrides parent) Return Type Can vary (but not just return type) Must be same or covariant FAQ Q1. Can we overload main() in Java? ✅ Yes, but JVM only calls public static void main(String[] args). public class Main { public static void main(String[] args) { System.out.println("Standard main"); main(10); // Calls overloaded main } public static void main(int x) { // Overloaded main System.out.println("Overloaded main: " + x); } } Q2. Can we overload static methods? ✅ Yes, static methods can be overloaded just like instance methods. Q3. Can constructors be overloaded? ✅ Yes, constructors support overloading (e.g., new Student() and new Student("Alice")). Conclusion Method overloading improves flexibility and readability by allowing multiple methods with the same name but different parameters. It is resolved at compile time based on the method signature. Would you like a practical example or quiz to test your understanding?
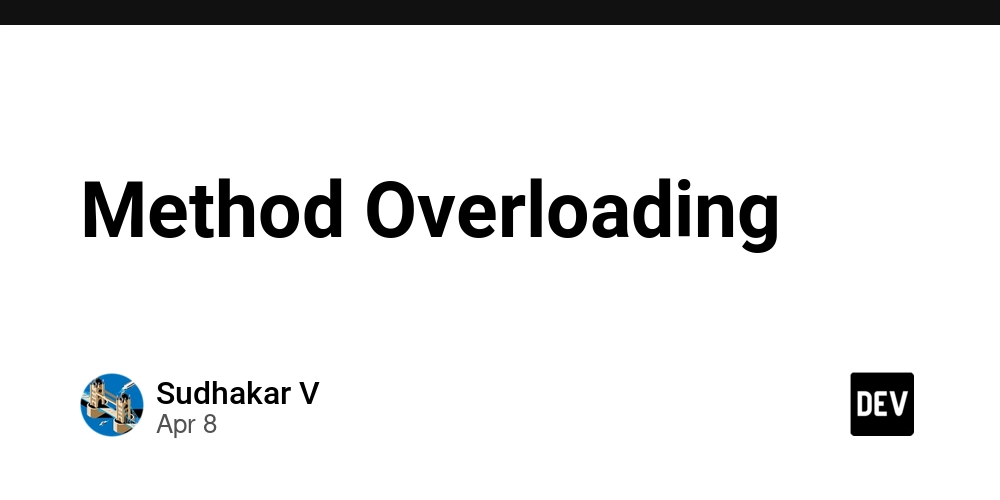
Method Overloading in Java
Method overloading allows a class to have multiple methods with the same name but different parameters. It is a way to achieve compile-time polymorphism.
Key Points:
-
Same method name, but different:
- Number of parameters
- Types of parameters
- Order of parameters
- Return type alone cannot differentiate overloaded methods.
- Can be done within the same class or between parent and child classes.
- Compile-time (static) polymorphism – the correct method is chosen at compile time.
Examples of Method Overloading
1. Different Number of Parameters
class Calculator {
int add(int a, int b) {
return a + b;
}
int add(int a, int b, int c) { // Overloaded method
return a + b + c;
}
}
2. Different Data Types
class Printer {
void print(int num) {
System.out.println("Printing int: " + num);
}
void print(String text) { // Overloaded method
System.out.println("Printing string: " + text);
}
}
3. Different Order of Parameters
class Display {
void show(int a, double b) {
System.out.println("int first, then double");
}
void show(double a, int b) { // Overloaded method
System.out.println("double first, then int");
}
}
4. Invalid Overloading (Return Type Alone is Not Enough)
❌ Compile Error – Ambiguous method call.
int multiply(int a, int b) { return a * b; }
double multiply(int a, int b) { return a * b; } // ❌ Not valid overloading
Why Use Method Overloading?
- Improves code readability (same method name for similar actions).
- Allows flexibility in method calls.
- Avoids complex method names like
addTwoNumbers()
,addThreeNumbers()
.
Method Overloading vs. Method Overriding
Feature | Method Overloading | Method Overriding |
---|---|---|
Definition | Same name, different parameters | Same name & parameters in subclass |
Polymorphism | Compile-time | Runtime (dynamic) |
Inheritance | Not required | Required (subclass overrides parent) |
Return Type | Can vary (but not just return type) | Must be same or covariant |
FAQ
Q1. Can we overload main()
in Java?
✅ Yes, but JVM only calls public static void main(String[] args)
.
public class Main {
public static void main(String[] args) {
System.out.println("Standard main");
main(10); // Calls overloaded main
}
public static void main(int x) { // Overloaded main
System.out.println("Overloaded main: " + x);
}
}
Q2. Can we overload static methods?
✅ Yes, static methods can be overloaded just like instance methods.
Q3. Can constructors be overloaded?
✅ Yes, constructors support overloading (e.g., new Student()
and new Student("Alice")
).
Conclusion
Method overloading improves flexibility and readability by allowing multiple methods with the same name but different parameters. It is resolved at compile time based on the method signature.
Would you like a practical example or quiz to test your understanding?