How to Fix 'Line 5 Expecting Identifier' Error in Lua?
Introduction If you're encountering the error message "Line 5 Expecting Identifier" while attempting to run your Lua obfuscator, you're likely having trouble with how you've structured your code. Understanding the error message can help you fix it and get your obfuscator working correctly. In this article, we'll delve into the root causes of this error, step-by-step solutions, and ways to improve your Lua code for better functionality. Understanding the Error The error you're seeing is due to incorrect syntax in your Lua script. Specifically, this error message suggests that the Lua interpreter has encountered a piece of code it doesn't know how to interpret, often due to misspelled keywords or improper use of operators and functions. In your case, the use of wait() was typed with an uppercase 'W' instead of lowercase, which is essential as Lua keywords are case-sensitive. Common Causes of 'Expecting Identifier' Errors Incorrect Function Calls: Lua is strict about case sensitivity. If you use Wait() instead of wait(), the interpreter won't recognize it and will throw an error. Malformed strings: Ensure that all strings are properly closed and that there are no unmatched quotations. Invalid identifiers: Identifiers in Lua must begin with a letter or underscore and cannot start with a number or contain special characters (except for _). Incorrect function definitions: Ensure that your function syntax adheres to Lua's rules. Step-by-Step Solution to Fix Your Code Let’s walk through fixing your Lua code step-by-step and ensuring it’s functioning as intended. Step 1: Correct the wait() Function Call As you have identified, changing Wait() to wait() should solve the immediate issue causing the error. Here is the revised function call: local function Obfuscate(before, after) if string.match(Code, before) then Code = Code:gsub(before, after) end wait(0.01) -- Ensure 'wait' is in lowercase end Step 2: Use Proper String Matching Ensure that your string patterns in the Obfuscate function are defined correctly. This can significantly improve your code's performance. For example, the previous calls to Obfuscate could break down unused substitutions if not configured right. Here's a snippet: Obfuscate("%%A", "65") Obfuscate("%%B", "66") -- Add similar calls Each call should have the correct string expressions you want to manipulate. Step 3: Validate the Output After altering the function calls, run the script to see if there are any other issues indicated by errors. If it prints an excessively long string upon using the obfuscator, make sure to check if you've mistakenly included extra spaces or formatting. Step 4: Testing You can test the code snippets step-by-step to immediately catch errors or inefficiencies. Here's an example of how to test part of your code: print(Code) -- Check the output after manipulation Frequently Asked Questions Q1: What is the difference between wait() and Wait()? A1: In Lua, function names are case-sensitive. wait() is a built-in function in Roblox for pausing the script for a specified time, whereas Wait() is not recognized, leading to errors. Q2: What if my string output is too long? A2: Review your obfuscation replacements. Ensure that you're replacing the correct patterns to avoid unintended long outputs. Q3: Can I optimize this obfuscator further? A3: Yes! Consider using a mapping table for your character replacements instead of individual function calls for each character. This can greatly enhance the performance of your script. Conclusion Errors like "Line 5 Expecting Identifier" in Lua can often feel frustrating, especially when you’re in the thick of developing a project. Mistakes like incorrect case sensitivity can lead to these roadblocks. By following the steps outlined above and testing iteratively, you can successfully debug your obfuscator code and improve its efficiency. Remember, clear and structured code not only helps avoid errors but also enhances maintainability and readability. Happy coding!
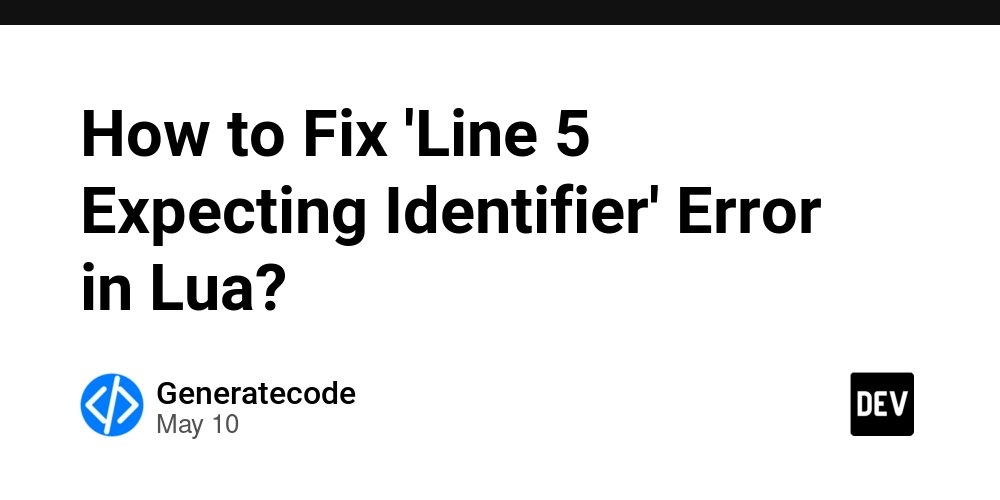
Introduction
If you're encountering the error message "Line 5 Expecting Identifier" while attempting to run your Lua obfuscator, you're likely having trouble with how you've structured your code. Understanding the error message can help you fix it and get your obfuscator working correctly. In this article, we'll delve into the root causes of this error, step-by-step solutions, and ways to improve your Lua code for better functionality.
Understanding the Error
The error you're seeing is due to incorrect syntax in your Lua script. Specifically, this error message suggests that the Lua interpreter has encountered a piece of code it doesn't know how to interpret, often due to misspelled keywords or improper use of operators and functions. In your case, the use of wait()
was typed with an uppercase 'W' instead of lowercase, which is essential as Lua keywords are case-sensitive.
Common Causes of 'Expecting Identifier' Errors
-
Incorrect Function Calls: Lua is strict about case sensitivity. If you use
Wait()
instead ofwait()
, the interpreter won't recognize it and will throw an error. - Malformed strings: Ensure that all strings are properly closed and that there are no unmatched quotations.
-
Invalid identifiers: Identifiers in Lua must begin with a letter or underscore and cannot start with a number or contain special characters (except for
_
). - Incorrect function definitions: Ensure that your function syntax adheres to Lua's rules.
Step-by-Step Solution to Fix Your Code
Let’s walk through fixing your Lua code step-by-step and ensuring it’s functioning as intended.
Step 1: Correct the wait()
Function Call
As you have identified, changing Wait()
to wait()
should solve the immediate issue causing the error. Here is the revised function call:
local function Obfuscate(before, after)
if string.match(Code, before) then
Code = Code:gsub(before, after)
end
wait(0.01) -- Ensure 'wait' is in lowercase
end
Step 2: Use Proper String Matching
Ensure that your string patterns in the Obfuscate
function are defined correctly. This can significantly improve your code's performance. For example, the previous calls to Obfuscate
could break down unused substitutions if not configured right. Here's a snippet:
Obfuscate("%%A", "65")
Obfuscate("%%B", "66")
-- Add similar calls
Each call should have the correct string expressions you want to manipulate.
Step 3: Validate the Output
After altering the function calls, run the script to see if there are any other issues indicated by errors. If it prints an excessively long string upon using the obfuscator, make sure to check if you've mistakenly included extra spaces or formatting.
Step 4: Testing
You can test the code snippets step-by-step to immediately catch errors or inefficiencies. Here's an example of how to test part of your code:
print(Code) -- Check the output after manipulation
Frequently Asked Questions
Q1: What is the difference between wait()
and Wait()
?
A1: In Lua, function names are case-sensitive. wait()
is a built-in function in Roblox for pausing the script for a specified time, whereas Wait()
is not recognized, leading to errors.
Q2: What if my string output is too long?
A2: Review your obfuscation replacements. Ensure that you're replacing the correct patterns to avoid unintended long outputs.
Q3: Can I optimize this obfuscator further?
A3: Yes! Consider using a mapping table for your character replacements instead of individual function calls for each character. This can greatly enhance the performance of your script.
Conclusion
Errors like "Line 5 Expecting Identifier" in Lua can often feel frustrating, especially when you’re in the thick of developing a project. Mistakes like incorrect case sensitivity can lead to these roadblocks. By following the steps outlined above and testing iteratively, you can successfully debug your obfuscator code and improve its efficiency. Remember, clear and structured code not only helps avoid errors but also enhances maintainability and readability. Happy coding!