How to Define the FUN Parameter for lsqcurvefit in MATLAB?
If you're working with MATLAB’s built-in lsqcurvefit function to fit a series of irregular Lorentzian peaks, you might have come across the challenge of correctly defining your custom model function. Your goal is to ensure that MATLAB can interact with your function without errors, particularly the one stating 'function is undefined for arguments of type "double".' In this article, we'll explore how to properly set up the FUN parameter in lsqcurvefit and ensure your data is fit appropriately. Understanding lsqcurvefit lsqcurvefit is designed to solve curve fitting problems by minimizing the sum of squares of nonlinear functions. It requires several inputs, including an initial guess for the parameters, the data points you want to fit, and a function that calculates the model predictions. Defining the Function FUN What is FUN? In the context of lsqcurvefit, the FUN parameter is a function handle that calculates the value of the model you want to fit to your data set. This model should take parameters as inputs and return the model values evaluated at the given x-data. Here’s a basic overview of how FUN should be structured: It must accept two inputs: the coefficients (parameters) and the data points. It must return a column vector containing the model's predictions for those data points. Correct Structure of myfun Considering your existing function, myfun, we should ensure it defines the expected input arguments correctly. You may need to adjust your myfun definition like so: function F = myfun(c, xdata) % Ensure xdata is a column vector xdata = xdata(:); F = c(1) + c(2) * exp(-1 * (xdata - c(3)).^2 / (c(4).^2)); end Explanation of Adjustments Input Verification: Adding xdata = xdata(:); ensures that xdata is a column vector. This is essential because lsqcurvefit expects the model output to be of a specific shape. Using lsqcurvefit with Your Function Next, you will want to call lsqcurvefit using your modified function. Here’s how you could structure that: % Example data XDATA = linspace(-10, 10, 100); % x data YDATA = [insert_your_data_here]; % y data (measured) % Initial guess for parameters: [offset, amplitude, center, width] X0 = [1, 1, 0, 1]; % Perform curve fitting X = lsqcurvefit(@myfun, X0, XDATA, YDATA); Troubleshooting Common Errors Function Not Defined: Ensure your myfun function is correctly defined in the same script or in a separate file named myfun.m in MATLAB’s current folder. Nonscalar Outputs: Ensure that your function returns a column vector, and that your input data is in the correct shape. Conclusion With the proper definition of your FUN parameter, using lsqcurvefit to fit your irregular Lorentzian peaks should be straightforward. By ensuring that the shape of your inputs matches with expectations in MATLAB, you'll avoid common pitfalls and errors such as those indicating that the function is undefined for certain argument types. Good luck with your fitting, and don't hesitate to ask if you encounter further challenges!
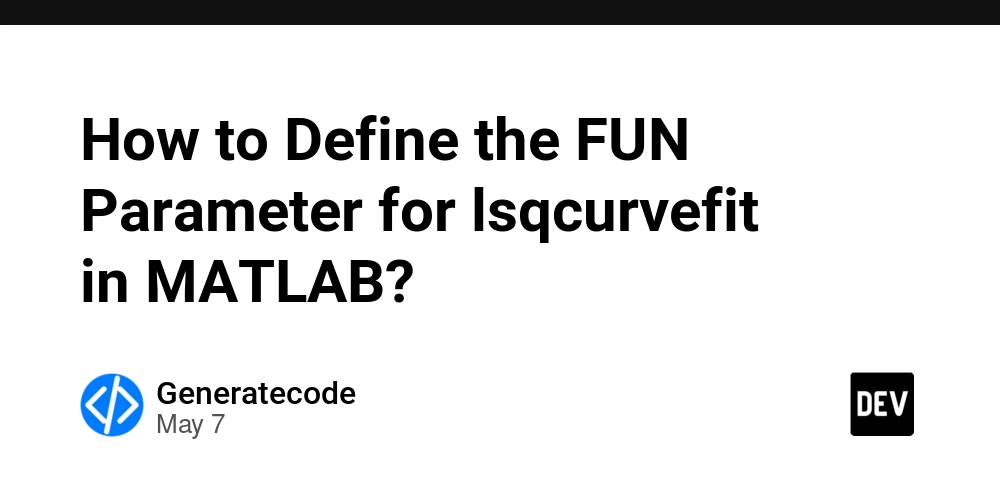
If you're working with MATLAB’s built-in lsqcurvefit
function to fit a series of irregular Lorentzian peaks, you might have come across the challenge of correctly defining your custom model function. Your goal is to ensure that MATLAB can interact with your function without errors, particularly the one stating 'function is undefined for arguments of type "double".' In this article, we'll explore how to properly set up the FUN
parameter in lsqcurvefit
and ensure your data is fit appropriately.
Understanding lsqcurvefit
lsqcurvefit
is designed to solve curve fitting problems by minimizing the sum of squares of nonlinear functions. It requires several inputs, including an initial guess for the parameters, the data points you want to fit, and a function that calculates the model predictions.
Defining the Function FUN
What is FUN
?
In the context of lsqcurvefit
, the FUN
parameter is a function handle that calculates the value of the model you want to fit to your data set. This model should take parameters as inputs and return the model values evaluated at the given x-data.
Here’s a basic overview of how FUN
should be structured:
- It must accept two inputs: the coefficients (parameters) and the data points.
- It must return a column vector containing the model's predictions for those data points.
Correct Structure of myfun
Considering your existing function, myfun
, we should ensure it defines the expected input arguments correctly. You may need to adjust your myfun
definition like so:
function F = myfun(c, xdata)
% Ensure xdata is a column vector
xdata = xdata(:);
F = c(1) + c(2) * exp(-1 * (xdata - c(3)).^2 / (c(4).^2));
end
Explanation of Adjustments
-
Input Verification: Adding
xdata = xdata(:);
ensures thatxdata
is a column vector. This is essential becauselsqcurvefit
expects the model output to be of a specific shape.
Using lsqcurvefit
with Your Function
Next, you will want to call lsqcurvefit
using your modified function. Here’s how you could structure that:
% Example data
XDATA = linspace(-10, 10, 100); % x data
YDATA = [insert_your_data_here]; % y data (measured)
% Initial guess for parameters: [offset, amplitude, center, width]
X0 = [1, 1, 0, 1];
% Perform curve fitting
X = lsqcurvefit(@myfun, X0, XDATA, YDATA);
Troubleshooting Common Errors
-
Function Not Defined: Ensure your
myfun
function is correctly defined in the same script or in a separate file namedmyfun.m
in MATLAB’s current folder. - Nonscalar Outputs: Ensure that your function returns a column vector, and that your input data is in the correct shape.
Conclusion
With the proper definition of your FUN
parameter, using lsqcurvefit
to fit your irregular Lorentzian peaks should be straightforward. By ensuring that the shape of your inputs matches with expectations in MATLAB, you'll avoid common pitfalls and errors such as those indicating that the function is undefined for certain argument types.
Good luck with your fitting, and don't hesitate to ask if you encounter further challenges!