Creating Custom Classes in Laravel Without Custom Commands
As a Laravel developer, I often encounter repetitive tasks that slow down development and add unnecessary complexity. One such challenge is creating custom class types, such as Services, Repositories, or Data Transfer Objects (DTOs). While Laravel’s Artisan commands are excellent for generating standard components like models or controllers, they don’t natively support custom class types. This leads to a common problem: manually creating Artisan commands for each class type, which is time, consuming and difficult to maintain. In this post, I’ll share my experience with this issue and introduce a practical solution, the usmanzahid/laravel-custom-make package, that streamlines the process with minimal configuration. The Challenge: Managing Custom Artisan Commands In many Laravel projects, I need to create custom classes to keep my code organized and maintainable. For example, I might use a Service class for business logic, a Repository for data access, or a DTO for structured data transfer. Without built-in Artisan commands for these, I initially resorted to creating custom commands, such as make:service or make:repository. While this approach worked, it was far from ideal. Each command required its own class and a stub file for the template. For a single class type, this might take an hour to set up. As the project grew, I found myself maintaining multiple commands, each with its own logic and stub files. Updating a stub meant editing the corresponding command’s code, and debugging issues across several commands became a hassle. This repetitive boilerplate felt inefficient and distracted me from focusing on the actual application logic. I needed a solution that would allow me to define custom class types quickly, without the overhead of creating and managing individual Artisan commands. That’s when I created the usmanzahid/laravel-custom-make package, which elegantly solves this problem. The laravel-custom-make package provides a lightweight and efficient way to generate custom class types in Laravel. Instead of writing a new Artisan command for each class type, you can define your custom types in a single configuration file, specify their output paths and stub templates, and let the package handle the rest. With just two lines of configuration per class type, you can create a new generator, making it a seamless addition to Laravel’s ecosystem. This package is particularly valuable because it eliminates the need for repetitive command creation while maintaining flexibility. It’s compatible with Laravel 10, 11, or 12 and requires PHP 8.0 or higher, ensuring broad applicability. Usage Guide: Setting Up laravel-custom-make Below, I’ll walk through the steps to install and configure the package, demonstrating how to create custom class types efficiently. Step 1: Install the Package Begin by installing the package via Composer: composer require usmanzahid/laravel-custom-make This command adds the package to your Laravel project, along with its dependencies. Step 2: Publish Configuration and Stubs Next, publish the package’s configuration file and stub templates: php artisan vendor:publish --provider="Usmanzahid\LaravelCustomMake\LaravelCustomMakeProvider" OR php artisan vendor:publish --tag=config php artisan vendor:publish --tag=stubs This creates a config/laravel_custom_maker.php file and a stubs/ directory in your project’s root. The configuration file defines your custom class types, while the stubs directory contains the templates for generated classes. Step 3: Configure Custom Class Types Open the config/laravel_custom_maker.php file. Here, you’ll define each custom class type by specifying its output path and stub file. For example, to configure Service and DTO class types: return [ 'service' => [ 'path' => app_path('Services'), 'stub' => base_path('stubs/service.stub'), ], 'dto' => [ 'path' => app_path('DTOs'), 'stub' => base_path('stubs/dto.stub'), ], ]; Each class type requires only two settings: path (where the generated file will be saved) and stub (the template file to use). This simplicity makes it easy to add new class types as needed. Step 4: Create Stub Templates Navigate to the stubs/ directory, where you can create or modify stub files. For a Service class, create a service.stub:
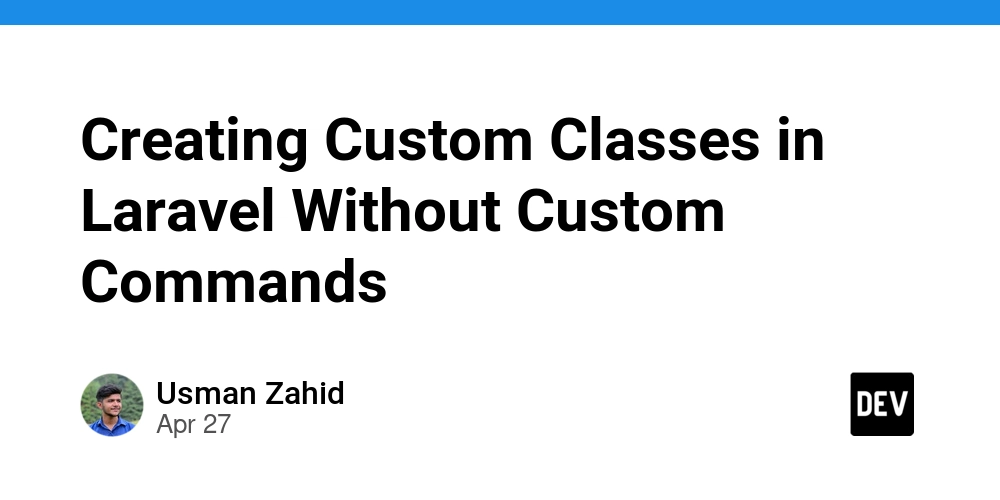
As a Laravel developer, I often encounter repetitive tasks that slow down development and add unnecessary complexity. One such challenge is creating custom class types, such as Services, Repositories, or Data Transfer Objects (DTOs).
While Laravel’s Artisan commands are excellent for generating standard components like models or controllers, they don’t natively support custom class types. This leads to a common problem: manually creating Artisan commands for each class type, which is time, consuming and difficult to maintain.
In this post, I’ll share my experience with this issue and introduce a practical solution, the usmanzahid/laravel-custom-make
package, that streamlines the process with minimal configuration.
The Challenge: Managing Custom Artisan Commands
In many Laravel projects, I need to create custom classes to keep my code organized and maintainable. For example, I might use a Service class for business logic, a Repository for data access, or a DTO for structured data transfer. Without built-in Artisan commands for these, I initially resorted to creating custom commands, such as make:service
or make:repository
.
While this approach worked, it was far from ideal. Each command required its own class and a stub file for the template. For a single class type, this might take an hour to set up.
As the project grew, I found myself maintaining multiple commands, each with its own logic and stub files. Updating a stub meant editing the corresponding command’s code, and debugging issues across several commands became a hassle.
This repetitive boilerplate felt inefficient and distracted me from focusing on the actual application logic.
I needed a solution that would allow me to define custom class types quickly, without the overhead of creating and managing individual Artisan commands. That’s when I created the usmanzahid/laravel-custom-make
package, which elegantly solves this problem.
The laravel-custom-make
package provides a lightweight and efficient way to generate custom class types in Laravel. Instead of writing a new Artisan command for each class type, you can define your custom types in a single configuration file, specify their output paths and stub templates, and let the package handle the rest. With just two lines of configuration per class type, you can create a new generator, making it a seamless addition to Laravel’s ecosystem.
This package is particularly valuable because it eliminates the need for repetitive command creation while maintaining flexibility. It’s compatible with Laravel 10, 11, or 12 and requires PHP 8.0 or higher, ensuring broad applicability.
Usage Guide: Setting Up laravel-custom-make
Below, I’ll walk through the steps to install and configure the package, demonstrating how to create custom class types efficiently.
Step 1: Install the Package
Begin by installing the package via Composer:
composer require usmanzahid/laravel-custom-make
This command adds the package to your Laravel project, along with its dependencies.
Step 2: Publish Configuration and Stubs
Next, publish the package’s configuration file and stub templates:
php artisan vendor:publish --provider="Usmanzahid\LaravelCustomMake\LaravelCustomMakeProvider"
OR
php artisan vendor:publish --tag=config
php artisan vendor:publish --tag=stubs
This creates a config/laravel_custom_maker.php
file and a stubs/
directory in your project’s root. The configuration file defines your custom class types, while the stubs directory contains the templates for generated classes.
Step 3: Configure Custom Class Types
Open the config/laravel_custom_maker.php
file. Here, you’ll define each custom class type by specifying its output path and stub file. For example, to configure Service and DTO class types:
return [
'service' => [
'path' => app_path('Services'),
'stub' => base_path('stubs/service.stub'),
],
'dto' => [
'path' => app_path('DTOs'),
'stub' => base_path('stubs/dto.stub'),
],
];
Each class type requires only two settings: path (where the generated file will be saved) and stub (the template file to use). This simplicity makes it easy to add new class types as needed.
Step 4: Create Stub Templates
Navigate to the stubs/
directory, where you can create or modify stub files. For a Service class, create a service.stub:
namespace App\Services;
class {{ class }}
{
public function __construct()
{
// Initialize service
}
// Define service methods
}
For a DTO class, create a dto.stub
:
namespace App\DTOs;
class {{ class }}
{
// Define DTO properties
}
The {{ class }}
placeholder is automatically replaced with the class name provided during generation. Additional placeholders, such as {{ namespace }}
, can be used for more complex templates.
Step 5: Generate Custom Classes
With the configuration and stubs in place, you can generate classes using the make:custom
Artisan command. For example, to create an AuthService class:
php artisan make:custom service AuthService
This generates app/Services/AuthService.php
based on the service.stub
:
namespace App\Services;
class AuthService
{
public function __construct()
{
// Initialize service
}
// Define service methods
}
Similarly, to create a UserDTO
:
php artisan make:custom dto UserDTO
This produces app/DTOs/UserDTO.php
:
namespace App\DTOs;
class UserDTO
{
// Define DTO properties
}
The process is straightforward and eliminates the need for custom command classes.
Step 6: Extend and Customize
To add a new class type, such as a Repository, update the configuration:
'repository' => [
'path' => app_path('Repositories'),
'stub' => base_path('stubs/repository.stub'),
],
Create a repository.stub
, and you can generate Repository classes using the same make:custom
command. The package supports unlimited class types, and stubs can be customized with additional placeholders or logic as needed.
Benefits of laravel-custom-make
The laravel-custom-make package addresses the pain points of custom class generation by offering:
Efficiency: Define new class types with minimal configuration, avoiding the need for custom Artisan commands.
Flexibility: Customize stub templates to match your project’s conventions.
Maintainability: Centralize class type definitions in a single configuration file, simplifying updates.
Seamless Integration: Works naturally within Laravel’s Artisan ecosystem.
This package has significantly reduced the time I spend on boilerplate code, allowing me to focus on developing core application features.
Conclusion
For Laravel developers seeking a streamlined approach to generating custom class types, the usmanzahid/laravel-custom-make package is an invaluable tool. By replacing repetitive command creation with a simple configuration-based system, it saves time and reduces maintenance overhead. I encourage you to explore the package, experiment with custom stubs, and integrate it into your workflow.
You can find more details and contribute to the package on GitHub or install it via Packagist. If you have questions or insights about custom class generation in Laravel, feel free to share them in the comments—I’d love to hear your thoughts.
Thank you for reading, and happy coding!