Exploring the New Features of Vue 3 Composition API
Vue 3 brought major architectural changes to how developers write Vue applications, with the Composition API being the most transformative. It offers an alternative to the Options API and gives developers more powerful, scalable ways to manage logic, reactivity, and component organization. Let’s dive into what makes the Composition API such a game changer — with real code examples, comparisons, and performance benefits for modern frontend projects. Why the Composition API? In Vue 2, we wrote components using the Options API, which was intuitive but had limitations when scaling an app. Logic was split across data, methods, computed, etc., making it harder to maintain and reuse. The Composition API allows you to group related logic together and reuse it across components with ease. This aligns well with modern practices like composability, code reuse, and TypeScript integration. Basic Setup Here’s how a simple counter looks using the Composition API: import { ref } from 'vue' const count = ref(0) function increment() { count.value++ } Count is: {{ count }} What’s happening here? ref() creates a reactive value. Logic and state are colocated, not spread across options. The syntax simplifies component setup and improves DX (Developer Experience). Key Features & Concepts 1. ref and reactive Vue 3 provides two ways to declare reactive state. import { ref, reactive } from 'vue' const count = ref(0) // primitive reactivity const user = reactive({ name: 'John', age: 30 }) // object reactivity 2. computed Properties Just like in the Options API, but now imported and used directly: import { computed } from 'vue' const fullName = computed(() => `${user.firstName} ${user.lastName}`) 3. watch and watchEffect Watchers allow you to react to changes: watch(count, (newVal, oldVal) => { console.log(`Count changed from ${oldVal} to ${newVal}`) }) watchEffect(() => { console.log(`Count is now ${count.value}`) }) 4. Custom Composables One of the biggest strengths of the Composition API is creating reusable logic with custom hooks, called composables: // useToggle.ts import { ref } from 'vue' export function useToggle(initial = false) { const state = ref(initial) const toggle = () => (state.value = !state.value) return { state, toggle } } Usage in a component: const { state: isVisible, toggle } = useToggle() Improved DX and TypeScript Support The Composition API is fully compatible with TypeScript. The flexibility of function-based logic enables better type inference, auto-completion, and maintainability in IDEs. With , DX is improved by: Eliminating boilerplate Auto-importing variables into templates Enhanced readability and performance Options API vs. Composition API Feature Options API Composition API Code Organization Split by option Grouped by feature Reusability Mixins (limited) Composables (flexible) TypeScript Support Partial Excellent Learning Curve Lower for beginners Steeper but more powerful DX with Not supported Fully supported When to Use Composition API? Use Composition API when: Your component logic is growing complex You want to reuse logic across components You need better TypeScript support You're using modern tooling like Vite or Nuxt 3 Stick to Options API when: You're building small apps or MVPs Your team prefers simpler onboarding Final Thoughts Vue 3’s Composition API empowers developers to build more scalable, reusable, and maintainable frontend applications. While it introduces a learning curve, the long-term gains in productivity and code quality are worth the shift — especially for teams building modern apps at scale. If you're still using the Options API, try rewriting a small component with the Composition API. You might be surprised how much cleaner and intuitive your code becomes.
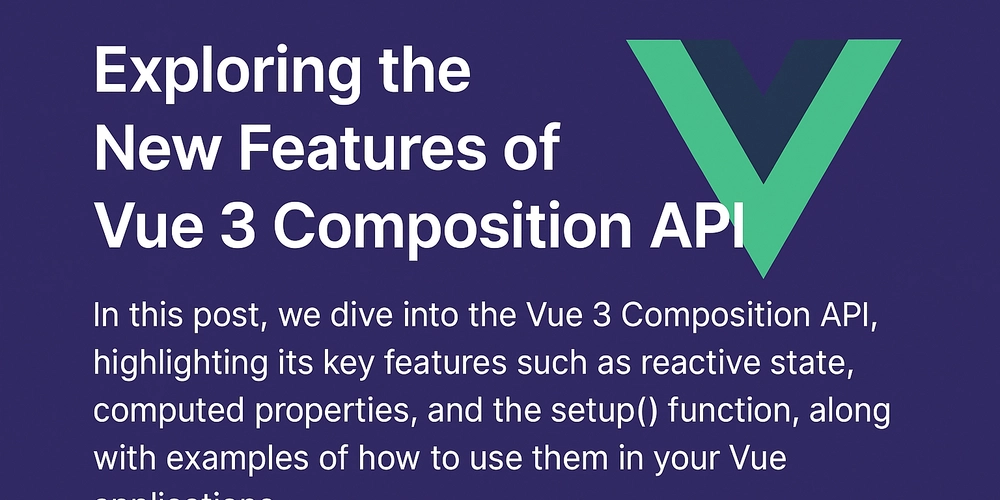
Vue 3 brought major architectural changes to how developers write Vue applications, with the Composition API being the most transformative. It offers an alternative to the Options API and gives developers more powerful, scalable ways to manage logic, reactivity, and component organization.
Let’s dive into what makes the Composition API such a game changer — with real code examples, comparisons, and performance benefits for modern frontend projects.
Why the Composition API?
In Vue 2, we wrote components using the Options API, which was intuitive but had limitations when scaling an app. Logic was split across data
, methods
, computed
, etc., making it harder to maintain and reuse.
The Composition API allows you to group related logic together and reuse it across components with ease. This aligns well with modern practices like composability, code reuse, and TypeScript integration.
Basic Setup
Here’s how a simple counter looks using the Composition API:
<script setup>
import { ref } from 'vue'
const count = ref(0)
function increment() {
count.value++
}
script>
<template>
template>
What’s happening here?
-
ref()
creates a reactive value. - Logic and state are colocated, not spread across options.
- The
syntax simplifies component setup and improves DX (Developer Experience).
Key Features & Concepts
1. ref
and reactive
Vue 3 provides two ways to declare reactive state.
import { ref, reactive } from 'vue'
const count = ref(0) // primitive reactivity
const user = reactive({ name: 'John', age: 30 }) // object reactivity
2. computed
Properties
Just like in the Options API, but now imported and used directly:
import { computed } from 'vue'
const fullName = computed(() => `${user.firstName} ${user.lastName}`)
3. watch
and watchEffect
Watchers allow you to react to changes:
watch(count, (newVal, oldVal) => {
console.log(`Count changed from ${oldVal} to ${newVal}`)
})
watchEffect(() => {
console.log(`Count is now ${count.value}`)
})
4. Custom Composables
One of the biggest strengths of the Composition API is creating reusable logic with custom hooks, called composables:
// useToggle.ts
import { ref } from 'vue'
export function useToggle(initial = false) {
const state = ref(initial)
const toggle = () => (state.value = !state.value)
return { state, toggle }
}
Usage in a component:
const { state: isVisible, toggle } = useToggle()
Improved DX and TypeScript Support
The Composition API is fully compatible with TypeScript. The flexibility of function-based logic enables better type inference, auto-completion, and maintainability in IDEs.
With , DX is improved by:
- Eliminating boilerplate
- Auto-importing variables into templates
- Enhanced readability and performance
Options API vs. Composition API
Feature | Options API | Composition API |
---|---|---|
Code Organization | Split by option | Grouped by feature |
Reusability | Mixins (limited) | Composables (flexible) |
TypeScript Support | Partial | Excellent |
Learning Curve | Lower for beginners | Steeper but more powerful |
DX with
|
Not supported | Fully supported |
When to Use Composition API?
Use Composition API when:
- Your component logic is growing complex
- You want to reuse logic across components
- You need better TypeScript support
- You're using modern tooling like Vite or Nuxt 3
Stick to Options API when:
- You're building small apps or MVPs
- Your team prefers simpler onboarding
Final Thoughts
Vue 3’s Composition API empowers developers to build more scalable, reusable, and maintainable frontend applications. While it introduces a learning curve, the long-term gains in productivity and code quality are worth the shift — especially for teams building modern apps at scale.
If you're still using the Options API, try rewriting a small component with the Composition API. You might be surprised how much cleaner and intuitive your code becomes.