Context API + TypeScript: How to avoid prop drilling in React
Passing props down through many levels of components — also known as prop drilling — can quickly make your React app harder to maintain and understand. The good news? React's Context API can help — and with TypeScript, you can make it fully type-safe. In this post, I’ll show you how to create a context from scratch using React + TypeScript, and how it helps keep your code clean. ✅ The Problem: Prop drilling everywhere Imagine this scenario: You have a deeply nested component. You need to pass the same data (like user info or theme) through multiple layers. Without context, you end up doing something like this: function App() { const user = { name: 'Alice' }; return ; } function Page({ user }: { user: { name: string } }) { return ; } function Sidebar({ user }: { user: { name: string } }) { return ; } function UserInfo({ user }: { user: { name: string } }) { return Hello, {user.name}!; } Every component in the chain has to receive and forward the user prop. This is where Context shines.
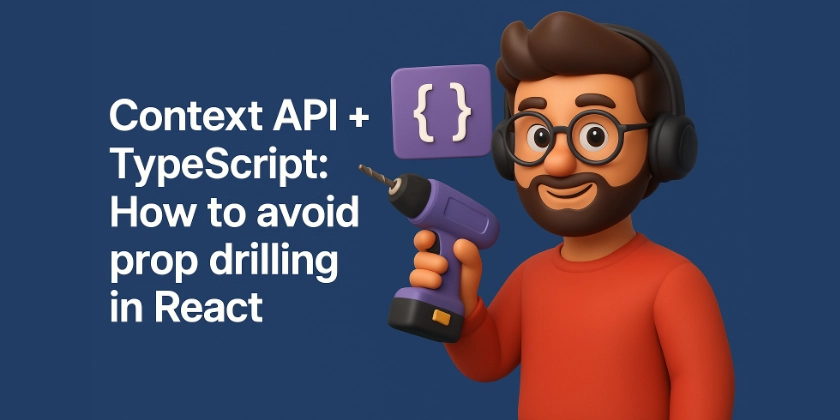
Passing props down through many levels of components — also known as prop drilling — can quickly make your React app harder to maintain and understand.
The good news? React's Context API can help — and with TypeScript, you can make it fully type-safe.
In this post, I’ll show you how to create a context from scratch using React + TypeScript, and how it helps keep your code clean.
✅ The Problem: Prop drilling everywhere
Imagine this scenario:
- You have a deeply nested component.
- You need to pass the same data (like user info or theme) through multiple layers.
Without context, you end up doing something like this:
function App() {
const user = { name: 'Alice' };
return <Page user={user} />;
}
function Page({ user }: { user: { name: string } }) {
return <Sidebar user={user} />;
}
function Sidebar({ user }: { user: { name: string } }) {
return <UserInfo user={user} />;
}
function UserInfo({ user }: { user: { name: string } }) {
return <p>Hello, {user.name}!p>;
}
Every component in the chain has to receive and forward the user
prop. This is where Context shines.