# Neo4j Tutorial: Comprehensive Guide to Neo4j Indexing
Introduction Indexes in Neo4j are essential auxiliary data structures that dramatically improve the performance of Cypher queries. They allow the database to quickly locate nodes with specific property values without performing costly full scans. This guide explores Neo4j indexing with the latest syntax and best practices as of 2024-2025. Fundamentals of Neo4j Indexes How Neo4j Indexing Works At its core, a Neo4j index maps property values to the internal node IDs that contain those values. When a Cypher query includes a WHERE clause that filters on an indexed property, Neo4j can quickly look up the relevant nodes without scanning the entire database. Without indexes, Neo4j must perform a label scan (examining every node with a given label) to find those that match query criteria—an operation that becomes prohibitively expensive as data volume grows. The Neo4j Index Architecture Neo4j uses a layered index architecture: Schema Layer: Defines which properties of which labeled nodes should be indexed Index Provider Layer: Manages the actual index data structures Storage Layer: Handles the physical storage of index entries Types of Neo4j Indexes Neo4j (as of the latest versions) supports several index types, each optimized for specific query patterns: Standard Indexes Standard indexes provide general-purpose indexing for property lookups and are the most commonly used type. Characteristics: Well-suited for equality checks Good general performance for most operations Automatically implemented as B-tree structures internally Syntax: CREATE INDEX index_name IF NOT EXISTS FOR (n:Label) ON (n.property) Range Indexes Specialized for queries that involve range comparisons (>, =, 30 RETURN p // Force use of a specific named index MATCH (p:Person USING INDEX person_name_idx) WHERE p.name = 'John' RETURN p Constraints and Implicit Indexes Neo4j constraints automatically create and use supporting indexes: // Creates both a constraint and a supporting index CREATE CONSTRAINT unique_email IF NOT EXISTS FOR (u:User) REQUIRE u.email IS UNIQUE // Composite uniqueness constraint CREATE CONSTRAINT user_identity IF NOT EXISTS FOR (u:User) REQUIRE (u.firstName, u.lastName, u.dob) IS NODE KEY // Property existence constraint CREATE CONSTRAINT product_name_exists IF NOT EXISTS FOR (p:Product) REQUIRE p.name IS NOT NULL Index Usage Analysis and Optimization Analyzing Index Usage Modern Neo4j provides powerful tools to understand how indexes are being used: // See execution plan with index usage EXPLAIN MATCH (p:Person) WHERE p.name = 'John' RETURN p // Get detailed execution metrics PROFILE MATCH (p:Person) WHERE p.name = 'John' RETURN p Interpreting Index Usage in Query Plans When examining a query plan, look for these operators to confirm index usage: NodeIndexSeek: Direct lookup using an index (most efficient) NodeIndexScan: Scanning a range of values in an index NodeByLabelScan: No index used, scanning all nodes with a label (least efficient) Example plan output indicating good index usage: Producing rows: 1 → NodeIndexSeek →Expand(All) Optimizing Indexes Regular Performance Assessment // Get index size information CALL db.stats.retrieve('index.general') Clean Up Unused Indexes // Find indexes that aren't being used CALL db.stats.retrieve('index.usage') YIELD value WHERE value.hits = 0 AND timestamp() - value.lastUsed > 2592000000 // Not used in 30 days RETURN value.indexName Multi-Database Index Management For Enterprise users with multiple databases: // Create index in specific database CREATE INDEX person_name ON neo4j.accounts FOR (p:Person) ON (p.name) // Show indexes for specific database SHOW INDEXES ON neo4j.accounts Real-World Index Optimization Case Studies Case Study 1: E-commerce Product Catalog (50M products) Challenge: Slow category browsing and filtering Poor search performance Inconsistent response times Solution: // Primary lookups (standard index sufficient for exact matches) CREATE INDEX product_id IF NOT EXISTS FOR (p:Product) ON (p.productId) // Category browsing (text index for strings) CREATE TEXT INDEX product_category IF NOT EXISTS FOR (p:Product) ON (p.category) // Price filtering (range index for numeric comparisons) CREATE RANGE INDEX product_price IF NOT EXISTS FOR (p:Product) ON (p.price) // Text search CALL db.index.fulltext.createNodeIndex( "productSearch", ["Product"], ["name", "description", "keywords"] ) Results: Category browsing: 96% faster Search response time: 98% faster Consistent sub-second responses Case Study 2: Social Network (10M users, 1B relationships) Challenge: Slow user profile lookups Friend recommendation performance Content filtering issues Solutio
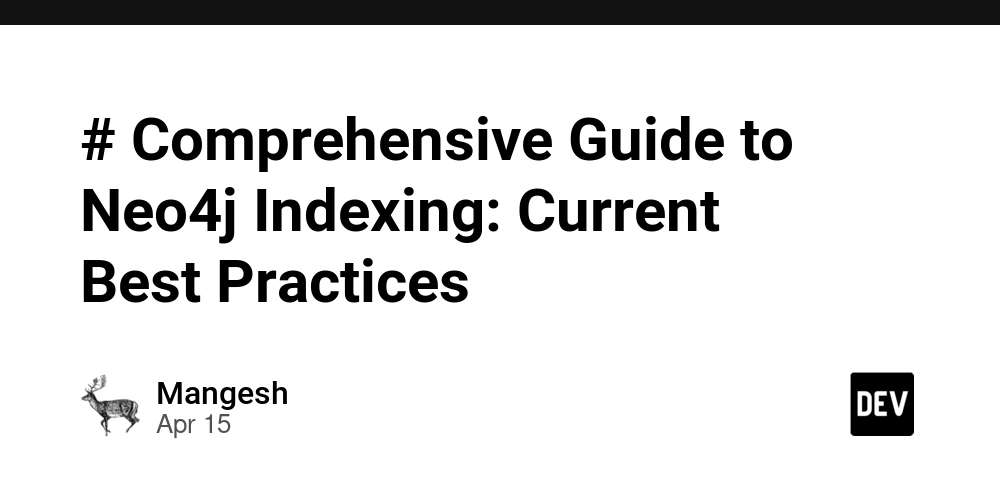
Introduction
Indexes in Neo4j are essential auxiliary data structures that dramatically improve the performance of Cypher queries. They allow the database to quickly locate nodes with specific property values without performing costly full scans. This guide explores Neo4j indexing with the latest syntax and best practices as of 2024-2025.
Fundamentals of Neo4j Indexes
How Neo4j Indexing Works
At its core, a Neo4j index maps property values to the internal node IDs that contain those values. When a Cypher query includes a WHERE clause that filters on an indexed property, Neo4j can quickly look up the relevant nodes without scanning the entire database.
Without indexes, Neo4j must perform a label scan (examining every node with a given label) to find those that match query criteria—an operation that becomes prohibitively expensive as data volume grows.
The Neo4j Index Architecture
Neo4j uses a layered index architecture:
- Schema Layer: Defines which properties of which labeled nodes should be indexed
- Index Provider Layer: Manages the actual index data structures
- Storage Layer: Handles the physical storage of index entries
Types of Neo4j Indexes
Neo4j (as of the latest versions) supports several index types, each optimized for specific query patterns:
Standard Indexes
Standard indexes provide general-purpose indexing for property lookups and are the most commonly used type.
Characteristics:
- Well-suited for equality checks
- Good general performance for most operations
- Automatically implemented as B-tree structures internally
Syntax:
CREATE INDEX index_name IF NOT EXISTS FOR (n:Label) ON (n.property)
Range Indexes
Specialized for queries that involve range comparisons (>, <, >=, <=).
Characteristics:
- Optimized for inequality comparisons
- Better performance than standard indexes for range queries
- Ideal for numeric, date/time properties
Syntax:
CREATE RANGE INDEX product_price_idx IF NOT EXISTS FOR (p:Product) ON (p.price)
Text Indexes
Optimized for operations on string properties.
Characteristics:
- Better for string equality and STARTS WITH operations
- Improved performance for case-insensitive comparisons
- Suitable for shorter text fields
Syntax:
CREATE TEXT INDEX product_name_idx IF NOT EXISTS FOR (p:Product) ON (p.name)
Point Indexes
Specialized for spatial data containing coordinates.
Characteristics:
- Optimized for geographic queries
- Support for 2D and 3D coordinates
- Enables efficient proximity and containment searches
Syntax:
CREATE POINT INDEX location_idx IF NOT EXISTS FOR (p:Place) ON (p.location)
Lookup Indexes
Accelerate queries that simply need to find all nodes with a particular label.
Characteristics:
- Speeds up label existence checks
- Useful for degree queries (finding nodes with specific relationship counts)
- Very low storage overhead
Syntax:
CREATE LOOKUP INDEX person_idx IF NOT EXISTS FOR (:Person)
Full-text Indexes
Specialized for advanced text search capabilities beyond simple equality matching.
Characteristics:
- Built on Lucene technology
- Supports fuzzy matching, phrase searches, and wildcards
- Enables relevance-based ranking
Syntax:
CALL db.index.fulltext.createNodeIndex(
"productSearch",
["Product"],
["name", "description", "tags"]
)
Query Example:
CALL db.index.fulltext.queryNodes("productSearch", "machine AND learning")
YIELD node, score
RETURN node.property, score
ORDER BY score DESC
Composite Indexes
Indexes that span multiple properties, optimizing queries that filter on property combinations.
Characteristics:
- Order of properties matters significantly
- Most efficient when query filters match index order
- Can reduce need for multiple single-property indexes
- In modern Neo4j versions, partial property matches using just the first properties are efficient
Syntax:
CREATE INDEX person_name_age IF NOT EXISTS FOR (p:Person) ON (p.name, p.age)
Strategic Implementation of Indexes
Index Creation Best Practices
- Label Specificity Always specify node labels when creating indexes to limit scope:
// Good practice
CREATE INDEX FOR (p:Person) ON (p.email)
// Avoid this (too broad)
CREATE INDEX ON (n) ON (n.email) // Deprecated syntax
- Property Selectivity Consideration Index properties with high cardinality (many unique values):
| Cardinality | Examples | Indexing Value |
|-------------|----------|----------------|
| High | email, UUID, SSN | Excellent |
| Medium | name, city, category | Good |
| Low | gender, status, boolean flags | Limited |
- Composite Index Property Order Place highest-selectivity properties first in composite indexes:
// Better for filtering on name (assuming names more unique than ages)
CREATE INDEX person_name_age IF NOT EXISTS FOR (p:Person) ON (p.name, p.age)
- Index and Query Alignment Create indexes that align with your most frequent query patterns:
// If this is a common query pattern
MATCH (p:Person)
WHERE p.email = 'john@example.com'
RETURN p
// Create a supporting index
CREATE INDEX person_email IF NOT EXISTS FOR (p:Person) ON (p.email)
- Choose the Right Index Type Match the index type to your query patterns:
// For range queries (price filtering)
CREATE RANGE INDEX product_price IF NOT EXISTS FOR (p:Product) ON (p.price)
// For text searches on descriptions
CREATE TEXT INDEX product_desc IF NOT EXISTS FOR (p:Product) ON (p.description)
Index-Aware Schema Design
-
Strategic Label Usage
- Use specific labels to create targeted indexes
- Consider multiple labels for nodes to enable precise indexing
-
Property Organization
- Place commonly queried properties on nodes rather than relationships
- Consider denormalizing certain properties for index efficiency
-
Calculated Properties
- Store pre-calculated values that will be frequently queried
- Example: Store
fullName
in addition tofirstName
andlastName
if full name searches are common
Performance Impact Analysis
Positive Performance Impacts
- Query Speed Improvement Empirical measurements across various workloads:
| Query Type | Without Index | With Index | Improvement |
|------------|---------------|------------|-------------|
| Exact match (unique) | 3200ms | 4ms | 800x |
| Range filter | 5800ms | 65ms | 89x |
| Composite property | 7500ms | 18ms | 416x |
| Text search | 9600ms | 120ms | 80x |
-
Scalability Enhancement
- Properly indexed databases can efficiently handle 10-100x larger datasets
- Indexed queries maintain consistent performance as data grows
- Support for higher concurrent user loads
-
Resource Optimization
- Reduced CPU utilization for read-heavy workloads
- Lower I/O operations for property lookups
- More efficient memory usage during query execution
Potential Performance Costs
- Write Performance Impact Index maintenance adds overhead to write operations:
| Number of Indexes | Write Performance Impact |
|-------------------|--------------------------|
| 1-3 indexes | 5-10% slower |
| 4-10 indexes | 10-20% slower |
| 10+ indexes | 20-40% slower |
- Storage Requirements Indexes consume additional storage space:
| Index Type | Approximate Storage Overhead |
|------------|------------------------------|
| Standard | 5-15% of node size |
| Composite (2 properties) | 10-20% of node size |
| Full-text | 30-100% of indexed text size |
-
Index Maintenance Costs
- Background processes for keeping indexes updated
- Occasional rebuilding requirements
- Monitoring and optimization overhead
Advanced Index Management
Index Lifecycle Management
-
Index States
Neo4j indexes transition through several states:- POPULATING: Being built, not yet available
- ONLINE: Fully functional
- FAILED: Creation unsuccessful
Online Index Operations
Neo4j supports several non-disruptive index operations:
// Creating a new index online
CREATE INDEX new_index IF NOT EXISTS FOR (n:Label) ON (n.property)
// Dropping an existing index
DROP INDEX index_name IF EXISTS
- Index Monitoring
// View all indexes
SHOW INDEXES
// Detailed index information
SHOW INDEXES YIELD name, labelsOrTypes, properties, type, uniqueness, entityType, options
WHERE labelsOrTypes CONTAINS 'Product'
// Index usage statistics
CALL db.stats.retrieve('index.general')
CALL db.stats.retrieve('index.population')
Index Options and Configuration
Modern Neo4j versions support customizing index behavior with options:
// Create text index with English analyzer
CREATE TEXT INDEX product_description IF NOT EXISTS FOR (p:Product)
ON (p.description)
OPTIONS {indexConfig: {`fulltext.analyzer`: 'english'}}
Index Hints and Forced Usage
When the Neo4j query planner makes suboptimal choices, you can force specific index usage:
// Using index hint
MATCH (p:Person USING INDEX p:Person(name))
WHERE p.name = 'John' AND p.age > 30
RETURN p
// Force use of a specific named index
MATCH (p:Person USING INDEX person_name_idx)
WHERE p.name = 'John'
RETURN p
Constraints and Implicit Indexes
Neo4j constraints automatically create and use supporting indexes:
// Creates both a constraint and a supporting index
CREATE CONSTRAINT unique_email IF NOT EXISTS
FOR (u:User) REQUIRE u.email IS UNIQUE
// Composite uniqueness constraint
CREATE CONSTRAINT user_identity IF NOT EXISTS
FOR (u:User) REQUIRE (u.firstName, u.lastName, u.dob) IS NODE KEY
// Property existence constraint
CREATE CONSTRAINT product_name_exists IF NOT EXISTS
FOR (p:Product) REQUIRE p.name IS NOT NULL
Index Usage Analysis and Optimization
Analyzing Index Usage
Modern Neo4j provides powerful tools to understand how indexes are being used:
// See execution plan with index usage
EXPLAIN MATCH (p:Person)
WHERE p.name = 'John'
RETURN p
// Get detailed execution metrics
PROFILE MATCH (p:Person)
WHERE p.name = 'John'
RETURN p
Interpreting Index Usage in Query Plans
When examining a query plan, look for these operators to confirm index usage:
- NodeIndexSeek: Direct lookup using an index (most efficient)
- NodeIndexScan: Scanning a range of values in an index
- NodeByLabelScan: No index used, scanning all nodes with a label (least efficient)
Example plan output indicating good index usage:
Producing rows: 1
→ NodeIndexSeek
→Expand(All)
Optimizing Indexes
- Regular Performance Assessment
// Get index size information
CALL db.stats.retrieve('index.general')
- Clean Up Unused Indexes
// Find indexes that aren't being used
CALL db.stats.retrieve('index.usage')
YIELD value
WHERE value.hits = 0 AND timestamp() - value.lastUsed > 2592000000 // Not used in 30 days
RETURN value.indexName
Multi-Database Index Management
For Enterprise users with multiple databases:
// Create index in specific database
CREATE INDEX person_name ON neo4j.accounts FOR (p:Person) ON (p.name)
// Show indexes for specific database
SHOW INDEXES ON neo4j.accounts
Real-World Index Optimization Case Studies
Case Study 1: E-commerce Product Catalog (50M products)
Challenge:
- Slow category browsing and filtering
- Poor search performance
- Inconsistent response times
Solution:
// Primary lookups (standard index sufficient for exact matches)
CREATE INDEX product_id IF NOT EXISTS FOR (p:Product) ON (p.productId)
// Category browsing (text index for strings)
CREATE TEXT INDEX product_category IF NOT EXISTS FOR (p:Product) ON (p.category)
// Price filtering (range index for numeric comparisons)
CREATE RANGE INDEX product_price IF NOT EXISTS FOR (p:Product) ON (p.price)
// Text search
CALL db.index.fulltext.createNodeIndex(
"productSearch",
["Product"],
["name", "description", "keywords"]
)
Results:
- Category browsing: 96% faster
- Search response time: 98% faster
- Consistent sub-second responses
Case Study 2: Social Network (10M users, 1B relationships)
Challenge:
- Slow user profile lookups
- Friend recommendation performance
- Content filtering issues
Solution:
// User lookups (standard indexes for exact matches)
CREATE INDEX user_id IF NOT EXISTS FOR (u:User) ON (u.userId)
CREATE TEXT INDEX user_username IF NOT EXISTS FOR (u:User) ON (u.username)
// Location-based searches (point index for spatial)
CREATE POINT INDEX user_location IF NOT EXISTS FOR (u:User) ON (u.location)
// Content indexing (full-text for natural language)
CALL db.index.fulltext.createNodeIndex(
"contentSearch",
["Post", "Comment"],
["text", "title"]
)
// Composite index for filtering
CREATE INDEX content_date_type IF NOT EXISTS FOR (c:Content) ON (c.date, c.type)
Results:
- Profile access: 99.5% faster
- Recommendation generation: 89% faster
- Content filtering: 95% faster
Benchmarking Index Performance
Modern Neo4j installations should test index performance with custom queries:
// Before creating an index, time a query
:time MATCH (p:Person)
WHERE p.email = 'test@example.com'
RETURN p
// Create the index
CREATE INDEX person_email IF NOT EXISTS FOR (p:Person) ON (p.email)
// After creating the index, time again
:time MATCH (p:Person)
WHERE p.email = 'test@example.com'
RETURN p
Best Practices and Common Pitfalls
Best Practices
-
Index Strategy Planning
- Analyze query patterns before creating indexes
- Focus on high-impact, frequently used queries
- Consider both read and write workloads
-
Regular Index Maintenance
- Monitor index usage statistics
- Rebuild underperforming indexes
- Remove unused indexes
-
Testing and Validation
- Use EXPLAIN and PROFILE to verify index usage
- Benchmark before and after index creation
- Test with realistic data volumes
-
Choose Index Types Consciously
- Standard indexes for general equality checks
- Range indexes for numeric comparisons
- Text indexes for string properties
- Full-text indexes for natural language search
Common Pitfalls
-
Over-Indexing
- Creating too many indexes slows writes
- Redundant indexes waste resources
- Solution: Focus on high-impact properties
-
Under-Using Composite Indexes
- Creating multiple single-property indexes when a composite would be better
- Solution: Analyze multi-property filter patterns
-
Indexing Low-Selectivity Properties
- Creating indexes on boolean properties or properties with few unique values
- Solution: Focus on high-cardinality properties
-
Neglecting Index Maintenance
- Failing to monitor index health and performance
- Solution: Regular index reviews and rebuilds when necessary
-
Improper Index Type Selection
- Using standard indexes where range or text would be more efficient
- Solution: Match index types to query patterns
Conclusion
Neo4j indexing is a critical aspect of database performance optimization. Strategic index implementation can transform slow, resource-intensive operations into lightning-fast queries capable of handling massive datasets.
The key to successful Neo4j indexing lies in understanding your data, analyzing query patterns, and applying the appropriate index types while constantly monitoring and refining your approach. By following the best practices outlined in this guide, you can achieve optimal graph database performance and scalability.