# Neo4j Tutorial: Establishing Constraints in Graph Databases
Introduction Welcome to this in-depth tutorial on establishing constraints in Neo4j! Constraints are crucial components of any database system, helping to ensure data integrity and consistency. In a graph database like Neo4j, constraints provide a way to enforce rules about the structure and content of your graph, preventing invalid data from being added and maintaining the reliability of your application. In this tutorial, we'll explore the various types of constraints available in Neo4j, learn how to create and manage them, and understand best practices for implementing constraints in your graph database projects. By the end, you'll have a solid understanding of how to use constraints to maintain the quality and consistency of your graph data. Understanding Constraints in Neo4j Constraints in Neo4j serve several important purposes: Ensuring data uniqueness: Preventing duplicate nodes with the same key values Enforcing data existence: Ensuring that required properties are always present Maintaining relationship validity: Making sure relationships connect appropriate nodes Streamlining queries: Optimizing queries that filter on constrained properties Before diving into the details, let's set up a sample database to work with throughout this tutorial. Setting Up Our Sample Database Let's create a sample database representing a simplified e-commerce system with products, categories, customers, and orders. // Create Product nodes CREATE (p1:Product {productId: "P001", name: "Smartphone", price: 699.99, stock: 50}) CREATE (p2:Product {productId: "P002", name: "Laptop", price: 1299.99, stock: 30}) CREATE (p3:Product {productId: "P003", name: "Headphones", price: 149.99, stock: 100}) CREATE (p4:Product {productId: "P004", name: "Tablet", price: 499.99, stock: 45}) CREATE (p5:Product {productId: "P005", name: "Smartwatch", price: 249.99, stock: 60}) // Create Category nodes CREATE (c1:Category {categoryId: "C001", name: "Electronics"}) CREATE (c2:Category {categoryId: "C002", name: "Computers"}) CREATE (c3:Category {categoryId: "C003", name: "Audio"}) CREATE (c4:Category {categoryId: "C004", name: "Wearables"}) // Create Customer nodes CREATE (cust1:Customer {customerId: "CUST001", name: "John Smith", email: "john.smith@example.com"}) CREATE (cust2:Customer {customerId: "CUST002", name: "Jane Doe", email: "jane.doe@example.com"}) CREATE (cust3:Customer {customerId: "CUST003", name: "Robert Johnson", email: "robert.j@example.com"}) CREATE (cust4:Customer {customerId: "CUST004", name: "Emily Wilson", email: "emily.w@example.com"}) // Create Order nodes CREATE (o1:Order {orderId: "ORD001", date: date("2023-01-15"), total: 699.99}) CREATE (o2:Order {orderId: "ORD002", date: date("2023-02-03"), total: 1449.98}) CREATE (o3:Order {orderId: "ORD003", date: date("2023-02-10"), total: 749.98}) CREATE (o4:Order {orderId: "ORD004", date: date("2023-03-05"), total: 249.99}) // Create relationships between Products and Categories MATCH (p:Product {productId: "P001"}), (c:Category {categoryId: "C001"}) CREATE (p)-[:BELONGS_TO]->(c) MATCH (p:Product {productId: "P002"}), (c:Category {categoryId: "C002"}) CREATE (p)-[:BELONGS_TO]->(c) MATCH (p:Product {productId: "P003"}), (c:Category {categoryId: "C003"}) CREATE (p)-[:BELONGS_TO]->(c) MATCH (p:Product {productId: "P004"}), (c:Category {categoryId: "C001"}) CREATE (p)-[:BELONGS_TO]->(c) MATCH (p:Product {productId: "P005"}), (c:Category {categoryId: "C004"}) CREATE (p)-[:BELONGS_TO]->(c) // Create relationships between Customers and Orders MATCH (cust:Customer {customerId: "CUST001"}), (o:Order {orderId: "ORD001"}) CREATE (cust)-[:PLACED]->(o) MATCH (cust:Customer {customerId: "CUST002"}), (o:Order {orderId: "ORD002"}) CREATE (cust)-[:PLACED]->(o) MATCH (cust:Customer {customerId: "CUST003"}), (o:Order {orderId: "ORD003"}) CREATE (cust)-[:PLACED]->(o) MATCH (cust:Customer {customerId: "CUST004"}), (o:Order {orderId: "ORD004"}) CREATE (cust)-[:PLACED]->(o) // Create relationships between Orders and Products MATCH (o:Order {orderId: "ORD001"}), (p:Product {productId: "P001"}) CREATE (o)-[:CONTAINS {quantity: 1}]->(p) MATCH (o:Order {orderId: "ORD002"}), (p:Product {productId: "P001"}) CREATE (o)-[:CONTAINS {quantity: 1}]->(p) MATCH (o:Order {orderId: "ORD002"}), (p:Product {productId: "P003"}) CREATE (o)-[:CONTAINS {quantity: 5}]->(p) MATCH (o:Order {orderId: "ORD003"}), (p:Product {productId: "P004"}) CREATE (o)-[:CONTAINS {quantity: 1}]->(p) MATCH (o:Order {orderId: "ORD003"}), (p:Product {productId: "P003"}) CREATE (o)-[:CONTAINS {quantity: 2}]->(p) MATCH (o:Order {orderId: "ORD004"}), (p:Product {productId: "P005"}) CREATE (o)-[:CONTAINS {quantity: 1}]->(p) Now that we have our sample e-commerce database, let's explore the different types of constraints in Neo4j. Types of Constraints in Neo4j Neo4j supports several types of constraints that can be applied to your graph data: Uniqueness Constrai
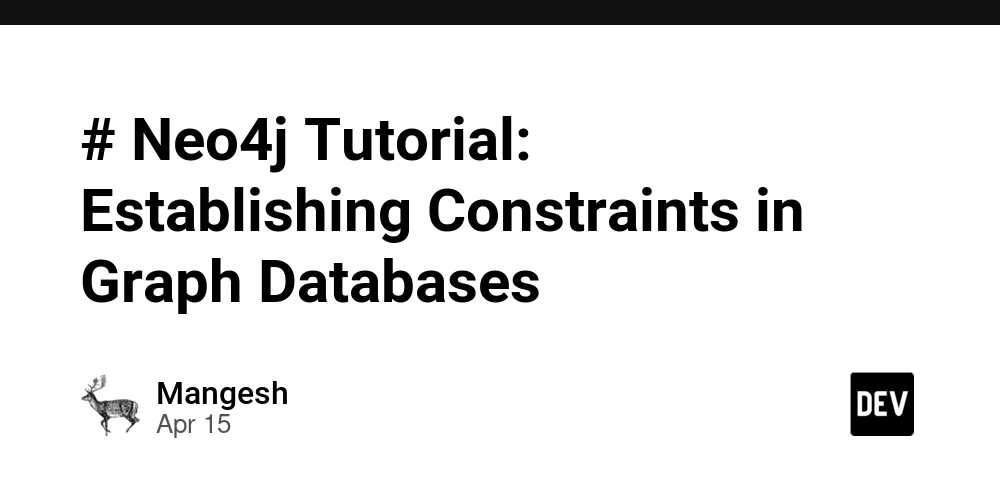
Introduction
Welcome to this in-depth tutorial on establishing constraints in Neo4j! Constraints are crucial components of any database system, helping to ensure data integrity and consistency. In a graph database like Neo4j, constraints provide a way to enforce rules about the structure and content of your graph, preventing invalid data from being added and maintaining the reliability of your application.
In this tutorial, we'll explore the various types of constraints available in Neo4j, learn how to create and manage them, and understand best practices for implementing constraints in your graph database projects. By the end, you'll have a solid understanding of how to use constraints to maintain the quality and consistency of your graph data.
Understanding Constraints in Neo4j
Constraints in Neo4j serve several important purposes:
- Ensuring data uniqueness: Preventing duplicate nodes with the same key values
- Enforcing data existence: Ensuring that required properties are always present
- Maintaining relationship validity: Making sure relationships connect appropriate nodes
- Streamlining queries: Optimizing queries that filter on constrained properties
Before diving into the details, let's set up a sample database to work with throughout this tutorial.
Setting Up Our Sample Database
Let's create a sample database representing a simplified e-commerce system with products, categories, customers, and orders.
// Create Product nodes
CREATE (p1:Product {productId: "P001", name: "Smartphone", price: 699.99, stock: 50})
CREATE (p2:Product {productId: "P002", name: "Laptop", price: 1299.99, stock: 30})
CREATE (p3:Product {productId: "P003", name: "Headphones", price: 149.99, stock: 100})
CREATE (p4:Product {productId: "P004", name: "Tablet", price: 499.99, stock: 45})
CREATE (p5:Product {productId: "P005", name: "Smartwatch", price: 249.99, stock: 60})
// Create Category nodes
CREATE (c1:Category {categoryId: "C001", name: "Electronics"})
CREATE (c2:Category {categoryId: "C002", name: "Computers"})
CREATE (c3:Category {categoryId: "C003", name: "Audio"})
CREATE (c4:Category {categoryId: "C004", name: "Wearables"})
// Create Customer nodes
CREATE (cust1:Customer {customerId: "CUST001", name: "John Smith", email: "john.smith@example.com"})
CREATE (cust2:Customer {customerId: "CUST002", name: "Jane Doe", email: "jane.doe@example.com"})
CREATE (cust3:Customer {customerId: "CUST003", name: "Robert Johnson", email: "robert.j@example.com"})
CREATE (cust4:Customer {customerId: "CUST004", name: "Emily Wilson", email: "emily.w@example.com"})
// Create Order nodes
CREATE (o1:Order {orderId: "ORD001", date: date("2023-01-15"), total: 699.99})
CREATE (o2:Order {orderId: "ORD002", date: date("2023-02-03"), total: 1449.98})
CREATE (o3:Order {orderId: "ORD003", date: date("2023-02-10"), total: 749.98})
CREATE (o4:Order {orderId: "ORD004", date: date("2023-03-05"), total: 249.99})
// Create relationships between Products and Categories
MATCH (p:Product {productId: "P001"}), (c:Category {categoryId: "C001"})
CREATE (p)-[:BELONGS_TO]->(c)
MATCH (p:Product {productId: "P002"}), (c:Category {categoryId: "C002"})
CREATE (p)-[:BELONGS_TO]->(c)
MATCH (p:Product {productId: "P003"}), (c:Category {categoryId: "C003"})
CREATE (p)-[:BELONGS_TO]->(c)
MATCH (p:Product {productId: "P004"}), (c:Category {categoryId: "C001"})
CREATE (p)-[:BELONGS_TO]->(c)
MATCH (p:Product {productId: "P005"}), (c:Category {categoryId: "C004"})
CREATE (p)-[:BELONGS_TO]->(c)
// Create relationships between Customers and Orders
MATCH (cust:Customer {customerId: "CUST001"}), (o:Order {orderId: "ORD001"})
CREATE (cust)-[:PLACED]->(o)
MATCH (cust:Customer {customerId: "CUST002"}), (o:Order {orderId: "ORD002"})
CREATE (cust)-[:PLACED]->(o)
MATCH (cust:Customer {customerId: "CUST003"}), (o:Order {orderId: "ORD003"})
CREATE (cust)-[:PLACED]->(o)
MATCH (cust:Customer {customerId: "CUST004"}), (o:Order {orderId: "ORD004"})
CREATE (cust)-[:PLACED]->(o)
// Create relationships between Orders and Products
MATCH (o:Order {orderId: "ORD001"}), (p:Product {productId: "P001"})
CREATE (o)-[:CONTAINS {quantity: 1}]->(p)
MATCH (o:Order {orderId: "ORD002"}), (p:Product {productId: "P001"})
CREATE (o)-[:CONTAINS {quantity: 1}]->(p)
MATCH (o:Order {orderId: "ORD002"}), (p:Product {productId: "P003"})
CREATE (o)-[:CONTAINS {quantity: 5}]->(p)
MATCH (o:Order {orderId: "ORD003"}), (p:Product {productId: "P004"})
CREATE (o)-[:CONTAINS {quantity: 1}]->(p)
MATCH (o:Order {orderId: "ORD003"}), (p:Product {productId: "P003"})
CREATE (o)-[:CONTAINS {quantity: 2}]->(p)
MATCH (o:Order {orderId: "ORD004"}), (p:Product {productId: "P005"})
CREATE (o)-[:CONTAINS {quantity: 1}]->(p)
Now that we have our sample e-commerce database, let's explore the different types of constraints in Neo4j.
Types of Constraints in Neo4j
Neo4j supports several types of constraints that can be applied to your graph data:
- Uniqueness Constraints: Ensure that a property (or combination of properties) has a unique value across all nodes with a specific label
- Existence Constraints: Ensure that a property exists on all nodes with a specific label or on all relationships of a specific type
- Node Key Constraints: Combine uniqueness and existence constraints to ensure that a combination of properties exists and is unique for all nodes with a specific label
- Property Type Constraints: Ensure that a property has a specific data type
Let's explore each type in detail.
Uniqueness Constraints
Uniqueness constraints ensure that a property value is unique across all nodes with a specific label. This is particularly useful for properties that serve as business keys or identifiers.
Creating a Uniqueness Constraint
The syntax for creating a uniqueness constraint is:
CREATE CONSTRAINT constraint_name IF NOT EXISTS
FOR (node:Label) REQUIRE node.property IS UNIQUE
Let's apply this to our e-commerce database:
// Create a uniqueness constraint on Product.productId
CREATE CONSTRAINT product_id_unique IF NOT EXISTS
FOR (p:Product) REQUIRE p.productId IS UNIQUE
This constraint ensures that no two Product nodes can have the same productId value.
Let's add more uniqueness constraints for our other node types:
// Create a uniqueness constraint on Category.categoryId
CREATE CONSTRAINT category_id_unique IF NOT EXISTS
FOR (c:Category) REQUIRE c.categoryId IS UNIQUE
// Create a uniqueness constraint on Customer.customerId
CREATE CONSTRAINT customer_id_unique IF NOT EXISTS
FOR (cust:Customer) REQUIRE cust.customerId IS UNIQUE
// Create a uniqueness constraint on Order.orderId
CREATE CONSTRAINT order_id_unique IF NOT EXISTS
FOR (o:Order) REQUIRE o.orderId IS UNIQUE
// Create a uniqueness constraint on Customer.email
CREATE CONSTRAINT customer_email_unique IF NOT EXISTS
FOR (cust:Customer) REQUIRE cust.email IS UNIQUE
Testing Uniqueness Constraints
Let's see what happens when we try to violate a uniqueness constraint:
// Attempt to create a Product with an existing productId
CREATE (p:Product {productId: "P001", name: "New Smartphone", price: 599.99, stock: 20})
This query should fail with an error message like:
Node(40) already exists with label `Product` and property `productId` = 'P001'
The constraint prevented us from creating a duplicate product with the same ID.
Existence Constraints
Existence constraints ensure that a property always exists on nodes with a specific label or relationships of a specific type. This is helpful for enforcing mandatory properties.
Creating an Existence Constraint
The syntax for creating an existence constraint is:
CREATE CONSTRAINT constraint_name IF NOT EXISTS
FOR (node:Label) REQUIRE node.property IS NOT NULL
Let's add some existence constraints to our e-commerce database:
// Ensure that all Products have a name
CREATE CONSTRAINT product_name_exists IF NOT EXISTS
FOR (p:Product) REQUIRE p.name IS NOT NULL
// Ensure that all Products have a price
CREATE CONSTRAINT product_price_exists IF NOT EXISTS
FOR (p:Product) REQUIRE p.price IS NOT NULL
// Ensure that all Categories have a name
CREATE CONSTRAINT category_name_exists IF NOT EXISTS
FOR (c:Category) REQUIRE c.name IS NOT NULL
// Ensure that all Customers have a name and email
CREATE CONSTRAINT customer_name_exists IF NOT EXISTS
FOR (cust:Customer) REQUIRE cust.name IS NOT NULL
CREATE CONSTRAINT customer_email_exists IF NOT EXISTS
FOR (cust:Customer) REQUIRE cust.email IS NOT NULL
// Ensure that all Orders have a date and total
CREATE CONSTRAINT order_date_exists IF NOT EXISTS
FOR (o:Order) REQUIRE o.date IS NOT NULL
CREATE CONSTRAINT order_total_exists IF NOT EXISTS
FOR (o:Order) REQUIRE o.total IS NOT NULL
Testing Existence Constraints
Let's see what happens when we try to violate an existence constraint:
// Attempt to create a Product without a name
CREATE (p:Product {productId: "P006", price: 349.99, stock: 25})
This query should fail with an error message like:
Node(47) with label `Product` must have the property `name`
The constraint prevented us from creating a product without the required name property.
Node Key Constraints
Node key constraints combine uniqueness and existence constraints to ensure that a combination of properties exists and is unique for all nodes with a specific label. This is particularly useful for enforcing composite keys.
Creating a Node Key Constraint
The syntax for creating a node key constraint is:
CREATE CONSTRAINT constraint_name IF NOT EXISTS
FOR (node:Label) REQUIRE (node.property1, node.property2, ...) IS NODE KEY
Let's add a node key constraint to our e-commerce database:
// Create a node key constraint on Product name and price
CREATE CONSTRAINT product_name_price_key IF NOT EXISTS
FOR (p:Product) REQUIRE (p.name, p.price) IS NODE KEY
This constraint ensures that the combination of name and price is unique across all Product nodes and that both properties always exist.
Testing Node Key Constraints
Let's see what happens when we try to violate a node key constraint:
// Attempt to create a Product with an existing name and price combination
CREATE (p:Product {productId: "P006", name: "Laptop", price: 1299.99, stock: 15})
This query should fail because we already have a Product node with the name "Laptop" and price 1299.99.
Property Type Constraints
Property type constraints ensure that a property has a specific data type. This helps maintain data consistency.
Creating a Property Type Constraint
The syntax for creating a property type constraint is:
CREATE CONSTRAINT constraint_name IF NOT EXISTS
FOR (node:Label) REQUIRE node.property IS :: TYPE
Let's add some property type constraints to our e-commerce database:
// Ensure that Product price is a float
CREATE CONSTRAINT product_price_type IF NOT EXISTS
FOR (p:Product) REQUIRE p.price IS :: FLOAT
// Ensure that Product stock is an integer
CREATE CONSTRAINT product_stock_type IF NOT EXISTS
FOR (p:Product) REQUIRE p.stock IS :: INTEGER
// Ensure that Order date is a date
CREATE CONSTRAINT order_date_type IF NOT EXISTS
FOR (o:Order) REQUIRE o.date IS :: DATE
Testing Property Type Constraints
Let's see what happens when we try to violate a property type constraint:
// Attempt to create a Product with a non-integer stock value
CREATE (p:Product {productId: "P007", name: "Speaker", price: 89.99, stock: "fifty"})
This query should fail because the stock property must be an integer, not a string.
Managing Constraints
Neo4j provides commands to view, modify, and drop constraints.
Viewing Existing Constraints
To see all constraints in your database:
SHOW CONSTRAINTS
This command returns information about all constraints, including their names, types, and the properties they apply to.
Dropping Constraints
To remove a constraint:
DROP CONSTRAINT constraint_name
For example:
DROP CONSTRAINT product_name_price_key
This removes the node key constraint on Product name and price.
Best Practices for Using Constraints
Here are some best practices to follow when implementing constraints in Neo4j:
- Use naming conventions: Give your constraints meaningful names that indicate what they enforce
- Don't over-constrain: Only add constraints for properties that truly need them
- Consider performance implications: Constraints can impact write performance, so use them judiciously
- Combine with indexes: Uniqueness constraints automatically create indexes, but consider adding indexes for other frequently queried properties
- Plan for constraint violations: In your application code, handle potential constraint violations gracefully
- Use the IF NOT EXISTS clause: This prevents errors when trying to create constraints that already exist
- Implement constraints early: Add constraints during database setup rather than after loading data
Practical Examples
Let's explore some practical use cases for constraints in our e-commerce database.
Example 1: Preventing Duplicate Emails
We've already added a uniqueness constraint on Customer.email, but let's see how this helps in a real-world scenario:
// Attempt to update a customer's email to one that already exists
MATCH (cust:Customer {customerId: "CUST003"})
SET cust.email = "jane.doe@example.com"
This update should fail because another customer already has this email address, preventing potential issues like duplicate accounts or misdirected communications.
Example 2: Ensuring Complete Product Information
With our existence constraints on Product name and price, we can be confident that all products in our database have the essential information needed for display and purchase:
// Create a new valid product
CREATE (p:Product {
productId: "P007",
name: "Bluetooth Speaker",
price: 79.99,
stock: 40
})
This query succeeds because it includes all required properties, ensuring data consistency for downstream applications.
Example 3: Maintaining Data Type Integrity
Our property type constraints ensure that numerical operations work as expected:
// Calculate total value of inventory
MATCH (p:Product)
RETURN sum(p.price * p.stock) AS TotalInventoryValue
This calculation works correctly because our constraints ensure that price is always a float and stock is always an integer.
Advanced Constraint Scenarios
Combining Constraints with Procedures
Neo4j procedures can be used alongside constraints for more complex validation:
// Using APOC to validate email format
CALL apoc.trigger.add(
'validateEmail',
'MATCH (c:Customer) WHERE id(c) = event.id AND NOT apoc.text.regexMatch(c.email, "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$") CALL apoc.util.validate(true, "Invalid email format", [0]) RETURN count(*)',
{phase: 'before'}
)
This trigger uses APOC procedures to validate email formats beyond what standard constraints can enforce.
Working with Relationship Constraints
While Neo4j currently doesn't support constraints directly on relationships, we can use node constraints to enforce relationship validity:
// Ensure that order totals match the sum of product prices
MATCH (o:Order)-[c:CONTAINS]->(p:Product)
WITH o, sum(c.quantity * p.price) AS calculatedTotal
WHERE o.total <> calculatedTotal
RETURN o.orderId, o.total, calculatedTotal
This query identifies orders where the total doesn't match the sum of the contained products, helping maintain data integrity.
Conclusion
Constraints are powerful tools for maintaining data quality and consistency in Neo4j graph databases. By implementing appropriate uniqueness, existence, node key, and property type constraints, you can prevent data inconsistencies and ensure that your graph database remains reliable and trustworthy.
In this tutorial, we've explored the different types of constraints available in Neo4j, learned how to create and manage them, and seen how they can be applied in practical scenarios. By following best practices and understanding how constraints work, you can build robust graph database applications that maintain data integrity even as your database grows and evolves.