Boost Code Reliability with Smart Exception Handling in Python
In the world of programming, errors are inevitable. Whether it’s a user input mistake, a missing file, or a network hiccup, unpredictable issues can disrupt the flow of even the most carefully written applications. That’s where exception handling in Python becomes a vital tool in every developer’s toolkit. More than just fixing bugs, it’s about building applications that are robust, reliable, and ready to face real-world challenges. Understanding how and when to handle exceptions in your code can drastically improve the performance, user experience, and long-term maintainability of your software. In this blog, we’ll explore the power of exception handling in Python and how using it smartly can make your code not only functional but resilient. What Are Exceptions? In programming, exceptions are events that disrupt the normal execution of a program. They typically occur when the system encounters something unexpected, such as invalid input, division by zero, or an unavailable file. Left unaddressed, these errors can cause a program to crash, leaving users frustrated and developers in a scramble to fix issues. Exception handling in Python offers a structured way to detect these issues, respond to them gracefully, and maintain control over your program's behavior. It helps ensure that your application doesn’t break in front of users and provides a pathway to deal with problems sensibly. The Importance of Exception Handling Why is exception handling so crucial? The answer lies in two key aspects of software development: reliability and user experience. Without proper exception handling, errors can result in abrupt terminations or confusing messages that leave users wondering what went wrong. Worse, unhandled exceptions can expose vulnerabilities, corrupt data, or compromise the functionality of a system. Smart exception handling prevents this by anticipating possible failure points and preparing suitable responses. By handling exceptions properly, you give your program a way to recover from unexpected situations without losing data or disrupting user tasks. It’s like having a safety net that catches problems before they escalate. Making Your Code Smarter with Structured Handling Smart exception handling in Python isn’t just about catching errors—it’s about handling them intelligently. That means thinking ahead and designing your code with failure in mind. It involves identifying parts of the code that are prone to failure, deciding which types of exceptions might occur, and writing logic to handle each scenario appropriately. For example, you might log the error for future debugging, display a user-friendly message, or retry the operation automatically. The goal is to ensure that your application can handle unexpected situations without losing its stability or purpose. Benefits of Smart Exception Handling Let’s explore some of the key benefits of implementing thoughtful and structured exception handling in Python: Increased Stability: Programs with well-managed exceptions are less likely to crash. They continue running or fail gracefully when something goes wrong. Better Debugging and Logging: Exception handling allows developers to log useful information when an error occurs, making it easier to trace issues and fix them quickly. Improved User Experience: Instead of showing confusing system errors, you can display clear and helpful messages to guide users when something fails. Maintainability: Code with consistent and well-placed exception handling is easier to read, test, and maintain over time. Security and Integrity: By controlling how exceptions are handled, you can avoid revealing sensitive system details or letting errors escalate into more serious problems. Common Pitfalls to Avoid While exception handling is a powerful tool, it’s important to use it correctly. Poor practices can cause more harm than good. Here are a few things to avoid: Catching every exception without purpose: Broad and vague error handling can hide real problems and make debugging harder. Ignoring exceptions entirely: Silencing an error without logging or taking action means you miss the opportunity to learn and improve your code. Overusing exception handling for flow control: It's better to prevent errors through checks and validations than to rely on catching them every time. Smart exception handling in Python means finding the balance between anticipating errors and writing clear, manageable code that responds to them appropriately. Building Confidence with Fail-Safe Code At its best, exception handling transforms your code from brittle to resilient. It gives you confidence that your program will handle edge cases, unexpected inputs, and system hiccups gracefully. It’s about embracing the unpredictability of the real world and preparing your software to adapt. Especially in larger applications, effective exception handling becomes
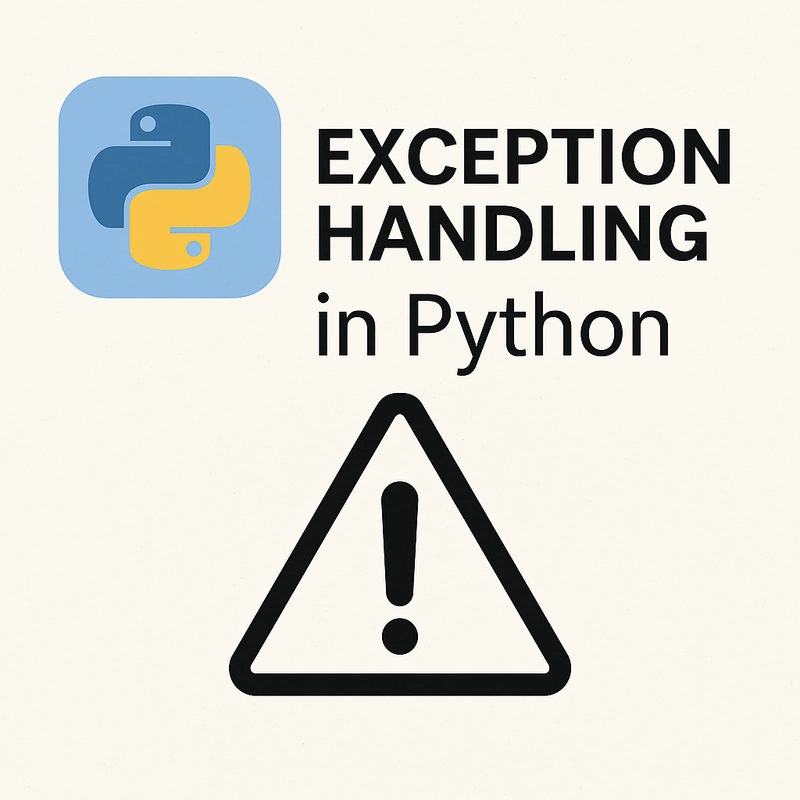
In the world of programming, errors are inevitable. Whether it’s a user input mistake, a missing file, or a network hiccup, unpredictable issues can disrupt the flow of even the most carefully written applications. That’s where exception handling in Python becomes a vital tool in every developer’s toolkit. More than just fixing bugs, it’s about building applications that are robust, reliable, and ready to face real-world challenges.
Understanding how and when to handle exceptions in your code can drastically improve the performance, user experience, and long-term maintainability of your software. In this blog, we’ll explore the power of exception handling in Python and how using it smartly can make your code not only functional but resilient.
What Are Exceptions?
In programming, exceptions are events that disrupt the normal execution of a program. They typically occur when the system encounters something unexpected, such as invalid input, division by zero, or an unavailable file. Left unaddressed, these errors can cause a program to crash, leaving users frustrated and developers in a scramble to fix issues.
Exception handling in Python offers a structured way to detect these issues, respond to them gracefully, and maintain control over your program's behavior. It helps ensure that your application doesn’t break in front of users and provides a pathway to deal with problems sensibly.
The Importance of Exception Handling
Why is exception handling so crucial? The answer lies in two key aspects of software development: reliability and user experience.
Without proper exception handling, errors can result in abrupt terminations or confusing messages that leave users wondering what went wrong. Worse, unhandled exceptions can expose vulnerabilities, corrupt data, or compromise the functionality of a system. Smart exception handling prevents this by anticipating possible failure points and preparing suitable responses.
By handling exceptions properly, you give your program a way to recover from unexpected situations without losing data or disrupting user tasks. It’s like having a safety net that catches problems before they escalate.
Making Your Code Smarter with Structured Handling
Smart exception handling in Python isn’t just about catching errors—it’s about handling them intelligently. That means thinking ahead and designing your code with failure in mind. It involves identifying parts of the code that are prone to failure, deciding which types of exceptions might occur, and writing logic to handle each scenario appropriately.
For example, you might log the error for future debugging, display a user-friendly message, or retry the operation automatically. The goal is to ensure that your application can handle unexpected situations without losing its stability or purpose.
Benefits of Smart Exception Handling
Let’s explore some of the key benefits of implementing thoughtful and structured exception handling in Python:
Increased Stability: Programs with well-managed exceptions are less likely to crash. They continue running or fail gracefully when something goes wrong.
Better Debugging and Logging: Exception handling allows developers to log useful information when an error occurs, making it easier to trace issues and fix them quickly.
Improved User Experience: Instead of showing confusing system errors, you can display clear and helpful messages to guide users when something fails.
Maintainability: Code with consistent and well-placed exception handling is easier to read, test, and maintain over time.
Security and Integrity: By controlling how exceptions are handled, you can avoid revealing sensitive system details or letting errors escalate into more serious problems.
Common Pitfalls to Avoid
While exception handling is a powerful tool, it’s important to use it correctly. Poor practices can cause more harm than good. Here are a few things to avoid:
- Catching every exception without purpose: Broad and vague error handling can hide real problems and make debugging harder.
- Ignoring exceptions entirely: Silencing an error without logging or taking action means you miss the opportunity to learn and improve your code.
- Overusing exception handling for flow control: It's better to prevent errors through checks and validations than to rely on catching them every time.
Smart exception handling in Python means finding the balance between anticipating errors and writing clear, manageable code that responds to them appropriately.
Building Confidence with Fail-Safe Code
At its best, exception handling transforms your code from brittle to resilient. It gives you confidence that your program will handle edge cases, unexpected inputs, and system hiccups gracefully. It’s about embracing the unpredictability of the real world and preparing your software to adapt.
Especially in larger applications, effective exception handling becomes part of the architecture. It ensures that failures in one part of the system don’t take down everything else. Instead, they’re contained, logged, and managed so that users can continue working, and developers can respond with clarity.
Final Thoughts
In the fast-paced, data-heavy, and user-centric world of modern software, reliability isn’t optional—it’s expected. That’s why smart exception handling in Python is more than a programming skill; it’s a discipline that helps you write cleaner, safer, and more professional code.
By anticipating problems and responding to them with thoughtful logic, your applications become more robust and user-friendly. Whether you're building simple scripts or complex systems, smart exception handling ensures your code stands strong in the face of errors.
Start seeing errors not as failures, but as opportunities to catch, learn, adapt, and build software that’s ready for anything.