AWS with Python: A Powerful Duo for Cloud Automation
In today's world, cloud computing has become the backbone of digital transformation, and AWS (Amazon Web Services) leads the charge with its powerful and scalable cloud solutions. Now, imagine combining that power with Python, a language known for its simplicity and versatility. The result? A game-changing combination that can automate, scale, and optimize cloud operations like never before. Why Use Python with AWS? Python is one of the most developer-friendly languages, and AWS provides a well-documented SDK for it—Boto3. With Boto3, you can interact with AWS services programmatically, eliminating the need for manual clicks and configurations. This is particularly useful for: • Automating infrastructure deployment • Managing AWS resources • Handling data storage and processing • Monitoring cloud performance Getting Started: Setting Up Python for AWS Before diving into the AWS-Python magic, ensure you have the following: Python Installed: Preferably Python 3.x (you can check with python --version). AWS CLI: This allows you to configure credentials and interact with AWS (aws configure). Boto3 Library: Install it using: pip install boto3 Once you set up these basics, you're ready to communicate with AWS programmatically. Automating AWS Tasks with Python Let's explore some common AWS automation tasks using Python. 1. Creating an S3 Bucket S3 (Simple Storage Service) is AWS’s go-to service for storing objects like images, videos, and backups. Here’s how to create a bucket with Python: import boto3 s3 = boto3.client('s3') bucket_name = "my-unique-bucket-name-12345" s3.create_bucket(Bucket=bucket_name) print(f"Bucket '{bucket_name}' created successfully!") Simple, right? You just created a cloud storage bucket with a few lines of code. 2. Launching an EC2 Instance EC2 (Elastic Compute Cloud) allows you to run virtual servers. You can launch one with the following script: import boto3 ec2 = boto3.resource('ec2') instance = ec2.create_instances( ImageId='ami-12345678', # Replace with a valid AMI ID MinCount=1, MaxCount=1, InstanceType='t2.micro', KeyName='your-key-pair' ) print("EC2 instance launched successfully!") This will spin up a new virtual server, allowing you to host applications or run tasks. 3. Automating AWS Lambda Deployment Lambda lets you run code without provisioning servers. Deploy a Lambda function easily: import boto3 lambda_client = boto3.client('lambda') response = lambda_client.create_function( FunctionName='MyLambdaFunction', Runtime='python3.8', Role='arn:aws:iam::123456789012:role/execution_role', # Replace with your IAM role Handler='lambda_function.lambda_handler', Code={'ZipFile': open('function.zip', 'rb').read()} ) print("Lambda function deployed successfully!") Now you have a serverless function running on AWS! Real-World Use Cases Using Python with AWS opens up endless possibilities, including: • Auto-scaling applications: Adjust computing power based on traffic. • Serverless APIs: Deploy REST APIs using API Gateway and Lambda. • Data processing pipelines: Automate ETL workflows using AWS Glue. • AI/ML model deployment: Deploy machine learning models with AWS SageMaker. Conclusion AWS and Python together offer an incredibly powerful way to manage and automate cloud resources. Whether you're a developer, data scientist, or DevOps engineer, mastering Boto3 can streamline cloud tasks and save hours of manual work. Ready to dive deeper? Start small, automate tasks, and soon, you'll be managing AWS like a pro with Python!
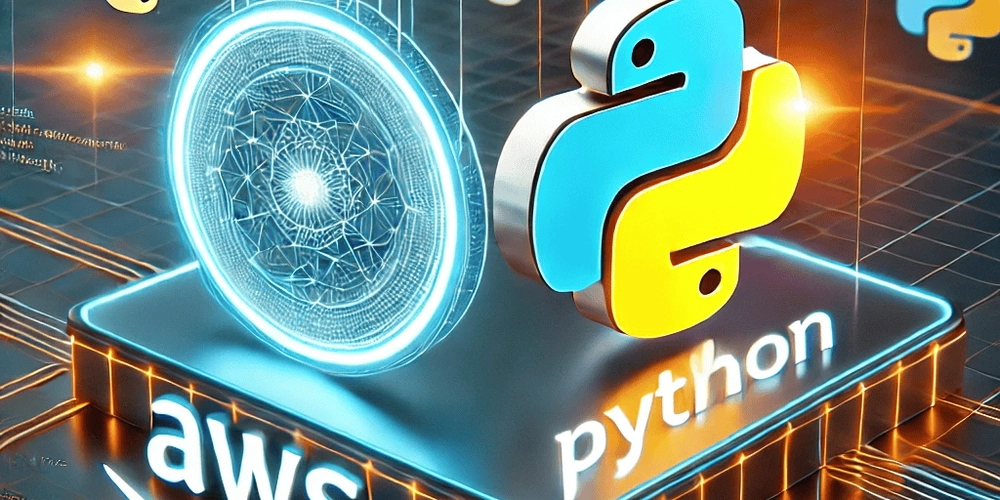
In today's world, cloud computing has become the backbone of digital transformation, and AWS (Amazon Web Services) leads the charge with its powerful and scalable cloud solutions. Now, imagine combining that power with Python, a language known for its simplicity and versatility. The result? A game-changing combination that can automate, scale, and optimize cloud operations like never before.
Why Use Python with AWS?
Python is one of the most developer-friendly languages, and AWS provides a well-documented SDK for it—Boto3. With Boto3, you can interact with AWS services programmatically, eliminating the need for manual clicks and configurations. This is particularly useful for:
• Automating infrastructure deployment
• Managing AWS resources
• Handling data storage and processing
• Monitoring cloud performance
Getting Started: Setting Up Python for AWS
Before diving into the AWS-Python magic, ensure you have the following:
Python Installed: Preferably Python 3.x (you can check with python --version).
AWS CLI: This allows you to configure credentials and interact with AWS (aws configure).
Boto3 Library: Install it using:
pip install boto3
Once you set up these basics, you're ready to communicate with AWS programmatically.
Automating AWS Tasks with Python
Let's explore some common AWS automation tasks using Python.
1. Creating an S3 Bucket
S3 (Simple Storage Service) is AWS’s go-to service for storing objects like images, videos, and backups. Here’s how to create a bucket with Python:
import boto3
s3 = boto3.client('s3')
bucket_name = "my-unique-bucket-name-12345"
s3.create_bucket(Bucket=bucket_name)
print(f"Bucket '{bucket_name}' created successfully!")
Simple, right? You just created a cloud storage bucket with a few lines of code.
2. Launching an EC2 Instance
EC2 (Elastic Compute Cloud) allows you to run virtual servers. You can launch one with the following script:
import boto3
ec2 = boto3.resource('ec2')
instance = ec2.create_instances(
ImageId='ami-12345678', # Replace with a valid AMI ID
MinCount=1,
MaxCount=1,
InstanceType='t2.micro',
KeyName='your-key-pair'
)
print("EC2 instance launched successfully!")
This will spin up a new virtual server, allowing you to host applications or run tasks.
3. Automating AWS Lambda Deployment
Lambda lets you run code without provisioning servers. Deploy a Lambda function easily:
import boto3
lambda_client = boto3.client('lambda')
response = lambda_client.create_function(
FunctionName='MyLambdaFunction',
Runtime='python3.8',
Role='arn:aws:iam::123456789012:role/execution_role', # Replace with your IAM role
Handler='lambda_function.lambda_handler',
Code={'ZipFile': open('function.zip', 'rb').read()}
)
print("Lambda function deployed successfully!")
Now you have a serverless function running on AWS!
Real-World Use Cases
Using Python with AWS opens up endless possibilities, including:
• Auto-scaling applications: Adjust computing power based on traffic.
• Serverless APIs: Deploy REST APIs using API Gateway and Lambda.
• Data processing pipelines: Automate ETL workflows using AWS Glue.
• AI/ML model deployment: Deploy machine learning models with AWS SageMaker.
Conclusion
AWS and Python together offer an incredibly powerful way to manage and automate cloud resources. Whether you're a developer, data scientist, or DevOps engineer, mastering Boto3 can streamline cloud tasks and save hours of manual work.
Ready to dive deeper? Start small, automate tasks, and soon, you'll be managing AWS like a pro with Python!