Why is the Login Failing in My Flutter Web App Release?
When deploying a Flutter web application, you might run into issues, such as the login functionality not working. This can often be perplexing, especially when everything seems to work perfectly in your development environment. Understanding potential pitfalls and implementing solutions can make a significant difference. Understanding the Problem Your login might fail due to several factors, including CORS (Cross-Origin Resource Sharing) settings, incorrect API request headers, or a mismatch in the expected parameters on the server side. Let's dive deeper to clarify why these issues occur and how we can resolve them. CORS and API Requests CORS is a security feature implemented by web browsers to prevent unauthorized requests to your API from a different domain. When you build your Flutter web app and deploy it, the app is accessed from a domain that your API may not recognize. This leads to blocked requests, which can be the root cause of the login issue. Your PHP code snippet outlines how you’re configuring CORS, which is an excellent start. However, let’s ensure that it’s implemented correctly and that your Flutter web app is making requests in a compatible manner. Step-by-Step Solution 1. Confirm CORS Setup in PHP Ensure that your PHP code effectively allows requests from your Flutter app's domain. It looks good overall, but make sure the following configuration is maintained: ini_set('display_errors', 1); error_reporting(E_ALL); $allowed_origins = [ 'https://fbc.mysite.ir', 'https://mysite.ir', 'http://localhost:54992', 'http://localhost:55687', 'http://localhost:5173', 'http://127.0.0.1:5173', ]; $origin = $_SERVER['HTTP_ORIGIN'] ?? ''; if (in_array($origin, $allowed_origins)) { header("Access-Control-Allow-Origin: $origin"); header("Access-Control-Allow-Credentials: true"); } header("Access-Control-Allow-Methods: GET, POST, OPTIONS, PUT, DELETE"); header("Access-Control-Allow-Headers: Content-Type, Authorization"); if ($_SERVER['REQUEST_METHOD'] === 'OPTIONS') { http_response_code(200); exit(); } In the above code, ensure the domains in $allowed_origins include the exact URL of your deployed Flutter app. 2. Flutter Web API Requests Next, double-check how you are making the API requests from your Flutter web application. Here’s a standard way to set up a login request using Dart's http package: import 'dart:convert'; import 'package:http/http.dart' as http; Future login(String username, String password) async { final url = 'https://api.mysite.ir/login'; final response = await http.post( Uri.parse(url), headers: { 'Content-Type': 'application/json', }, body: json.encode({'username': username, 'password': password}), ); if (response.statusCode == 200) { // Handle successful login print('Login successful'); } else { // Handle error response print('Login failed: ${response.body}'); } } Make sure that you are using the correct URL and sending the right parameters in the body of your request. Additionally, the Content-Type header must be set to application/json when sending JSON data. 3. Check for Server-Side Errors If everything seems correct on the Flutter side, look at your PHP code and server logs for any errors or warnings. Debugging the response from your API can pinpoint if the issue is at the server side. You can enhance error handling like this: if (!isset($_POST['username']) || !isset($_POST['password'])) { http_response_code(400); echo json_encode(['error' => 'Username and password required.']); exit(); } This way, your API provides clearer feedback, allowing for easier debugging when login problems occur. Frequently Asked Questions (FAQ) Why is my Flutter web app facing CORS issues? CORS issues often stem from the lack of appropriate headers being set in the server response, especially when deploying on a different domain. How can I debug API request errors in Flutter? Use the print statements to inspect request URLs and responses. Additionally, check your server logs to catch any PHP errors. Are there other security settings I should be aware of? You might also want to verify the SSL/TLS setup for your API, as modern browsers block non-secure calls from secure origin domains. Ensure your API is served over HTTPS. Troubleshooting login issues in your Flutter web application often involves a careful investigation of both client and server setups. By verifying your CORS configurations, correctly implementing API requests, and being vigilant about error logging, you can typically resolve these issues effectively. Remember to always test in an environment that closely resembles your live setup to catch these problems early.
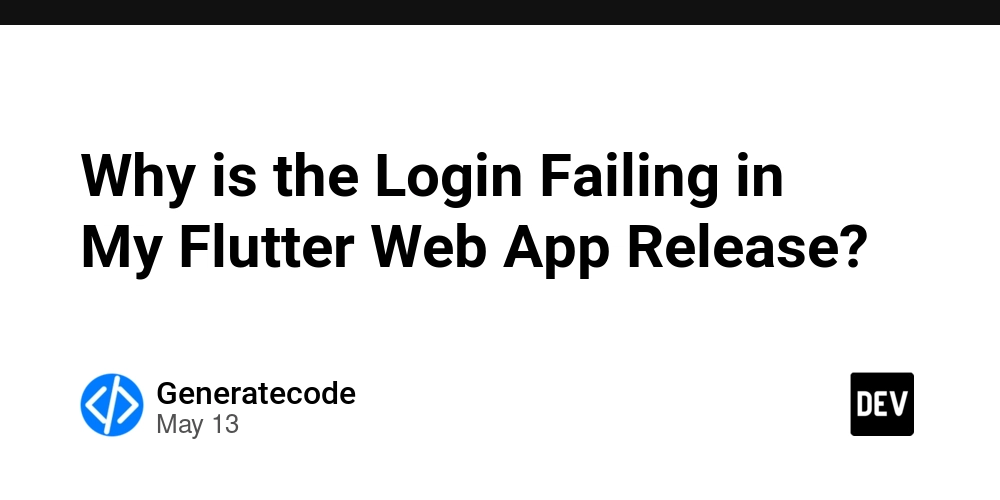
When deploying a Flutter web application, you might run into issues, such as the login functionality not working. This can often be perplexing, especially when everything seems to work perfectly in your development environment. Understanding potential pitfalls and implementing solutions can make a significant difference.
Understanding the Problem
Your login might fail due to several factors, including CORS (Cross-Origin Resource Sharing) settings, incorrect API request headers, or a mismatch in the expected parameters on the server side. Let's dive deeper to clarify why these issues occur and how we can resolve them.
CORS and API Requests
CORS is a security feature implemented by web browsers to prevent unauthorized requests to your API from a different domain. When you build your Flutter web app and deploy it, the app is accessed from a domain that your API may not recognize. This leads to blocked requests, which can be the root cause of the login issue.
Your PHP code snippet outlines how you’re configuring CORS, which is an excellent start. However, let’s ensure that it’s implemented correctly and that your Flutter web app is making requests in a compatible manner.
Step-by-Step Solution
1. Confirm CORS Setup in PHP
Ensure that your PHP code effectively allows requests from your Flutter app's domain. It looks good overall, but make sure the following configuration is maintained:
ini_set('display_errors', 1);
error_reporting(E_ALL);
$allowed_origins = [
'https://fbc.mysite.ir',
'https://mysite.ir',
'http://localhost:54992',
'http://localhost:55687',
'http://localhost:5173',
'http://127.0.0.1:5173',
];
$origin = $_SERVER['HTTP_ORIGIN'] ?? '';
if (in_array($origin, $allowed_origins)) {
header("Access-Control-Allow-Origin: $origin");
header("Access-Control-Allow-Credentials: true");
}
header("Access-Control-Allow-Methods: GET, POST, OPTIONS, PUT, DELETE");
header("Access-Control-Allow-Headers: Content-Type, Authorization");
if ($_SERVER['REQUEST_METHOD'] === 'OPTIONS') {
http_response_code(200);
exit();
}
In the above code, ensure the domains in $allowed_origins
include the exact URL of your deployed Flutter app.
2. Flutter Web API Requests
Next, double-check how you are making the API requests from your Flutter web application. Here’s a standard way to set up a login request using Dart's http
package:
import 'dart:convert';
import 'package:http/http.dart' as http;
Future login(String username, String password) async {
final url = 'https://api.mysite.ir/login';
final response = await http.post(
Uri.parse(url),
headers: {
'Content-Type': 'application/json',
},
body: json.encode({'username': username, 'password': password}),
);
if (response.statusCode == 200) {
// Handle successful login
print('Login successful');
} else {
// Handle error response
print('Login failed: ${response.body}');
}
}
Make sure that you are using the correct URL and sending the right parameters in the body of your request. Additionally, the Content-Type
header must be set to application/json
when sending JSON data.
3. Check for Server-Side Errors
If everything seems correct on the Flutter side, look at your PHP code and server logs for any errors or warnings. Debugging the response from your API can pinpoint if the issue is at the server side. You can enhance error handling like this:
if (!isset($_POST['username']) || !isset($_POST['password'])) {
http_response_code(400);
echo json_encode(['error' => 'Username and password required.']);
exit();
}
This way, your API provides clearer feedback, allowing for easier debugging when login problems occur.
Frequently Asked Questions (FAQ)
Why is my Flutter web app facing CORS issues?
CORS issues often stem from the lack of appropriate headers being set in the server response, especially when deploying on a different domain.
How can I debug API request errors in Flutter?
Use the print
statements to inspect request URLs and responses. Additionally, check your server logs to catch any PHP errors.
Are there other security settings I should be aware of?
You might also want to verify the SSL/TLS setup for your API, as modern browsers block non-secure calls from secure origin domains. Ensure your API is served over HTTPS.
Troubleshooting login issues in your Flutter web application often involves a careful investigation of both client and server setups. By verifying your CORS configurations, correctly implementing API requests, and being vigilant about error logging, you can typically resolve these issues effectively. Remember to always test in an environment that closely resembles your live setup to catch these problems early.