What is an IIFE in JavaScript and Why Would You Use It?
In JavaScript, you might have seen a function that seems to call itself right after it's defined. That’s called an IIFE, short for Immediately Invoked Function Expression. It might look weird at first, but it has some interesting use cases. Let’s explore what it is, how it works, and when to use it. What is an IIFE? An IIFE is a function that runs immediately after it’s defined. The main idea is to create a new scope without polluting the global one. Here’s how it looks: (function () { console.log('This runs immediately!'); })(); Or, with arrow function syntax: (() => { console.log('Arrow IIFE runs immediately!'); })(); Why Does This Work? In JavaScript, when you define a function like: function sayHi() {} …it’s just a declaration, and it won’t run until you call it. But to make the function run immediately, you need to turn it into an expression. That’s why we wrap it in parentheses: The first () turns the function into a function expression. The second () calls it. Common Use Cases Avoiding Global Scope Pollution IIFEs help keep variables inside their own scope so they don’t interfere with other parts of your code. (function () { const secret = 'I stay local'; console.log(secret); // Works here })(); console.log(secret); // Error: secret is not defined Running Setup Code Once If you want to run some initialization logic right away (like setting up configs), IIFE can be a clean solution. const app = (() => { const config = { env: 'production' }; console.log('App is starting...'); return { getConfig: () => config }; })(); Using await in Non-Async Environments (Async IIFE) In environments where you can't use top-level await (like older Node.js or CommonJS modules), an async IIFE allows you to run asynchronous code with await. (async () => { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); })(); This is super useful for running async logic without needing to define and then manually call a separate async function. IIFEs are a handy JavaScript pattern that allow you to execute functions immediately while keeping variables scoped locally. While not as common in ES6+ codebases thanks to modules and let/const, they're still worth knowing — especially when you stumble across them in older code or want a quick isolated block. Console You Later!
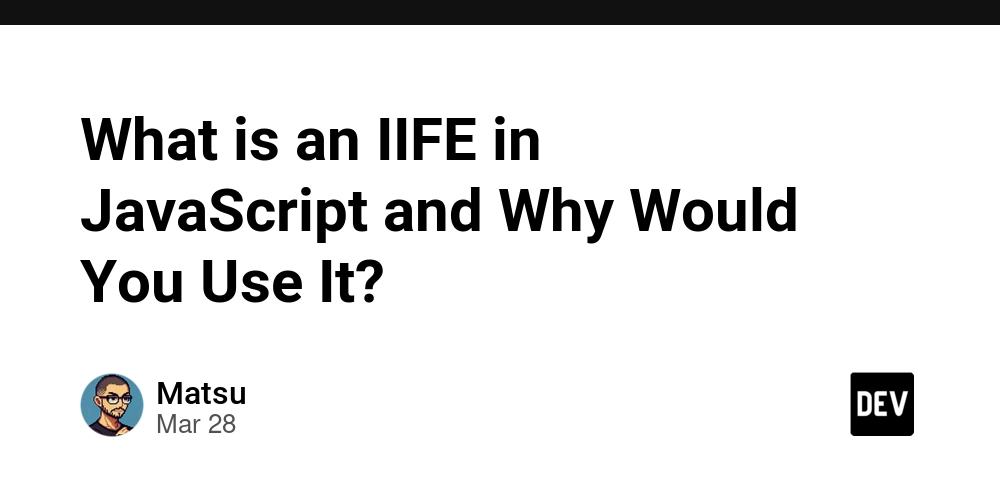
In JavaScript, you might have seen a function that seems to call itself right after it's defined. That’s called an IIFE, short for Immediately Invoked Function Expression. It might look weird at first, but it has some interesting use cases. Let’s explore what it is, how it works, and when to use it.
What is an IIFE?
An IIFE is a function that runs immediately after it’s defined. The main idea is to create a new scope without polluting the global one.
Here’s how it looks:
(function () { console.log('This runs immediately!'); })();
Or, with arrow function syntax:
(() => { console.log('Arrow IIFE runs immediately!'); })();
Why Does This Work?
In JavaScript, when you define a function like:
function sayHi() {}
…it’s just a declaration, and it won’t run until you call it.
But to make the function run immediately, you need to turn it into an expression. That’s why we wrap it in parentheses:
- The first () turns the function into a function expression.
- The second () calls it.
Common Use Cases
- Avoiding Global Scope Pollution IIFEs help keep variables inside their own scope so they don’t interfere with other parts of your code.
(function () { const secret = 'I stay local'; console.log(secret); // Works here })();
console.log(secret); // Error: secret is not defined
- Running Setup Code Once If you want to run some initialization logic right away (like setting up configs), IIFE can be a clean solution.
const app = (() => { const config = { env: 'production' }; console.log('App is starting...'); return { getConfig: () => config }; })();
- Using await in Non-Async Environments (Async IIFE) In environments where you can't use top-level await (like older Node.js or CommonJS modules), an async IIFE allows you to run asynchronous code with await.
(async () => { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); })();
This is super useful for running async logic without needing to define and then manually call a separate async function.
IIFEs are a handy JavaScript pattern that allow you to execute functions immediately while keeping variables scoped locally. While not as common in ES6+ codebases thanks to modules and let/const, they're still worth knowing — especially when you stumble across them in older code or want a quick isolated block.
Console You Later!