Mastering Rust: Develop a High-Performance CLI Application
Introduction Rust is a modern, high-performance programming language known for its memory safety, speed, and concurrency. It’s perfect for building command-line interface (CLI) applications because it offers control over low-level details without compromising safety. In this blog, we’ll walk you through building a simple CLI application using Rust that shines like neon signs when it comes to performance. Why Rust for CLI Applications? Performance: Rust is blazingly fast, competing with languages like C and C++. Memory Safety: Rust’s ownership model ensures memory safety without needing a garbage collector. Concurrency: Its fearless concurrency model helps avoid data races. Developer Tooling: Cargo, Rust’s package manager and build tool, makes project management straightforward. Getting Started 1. Setting Up Rust First, install Rust using Rustup, the recommended installer: curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh Verify the installation with: rustc --version 2. Creating a New Rust Project We’ll use Cargo to create a new project: cargo new my_cli_app cd my_cli_app 3. Writing Your First CLI App Edit the src/main.rs file with the following code: fn main() { println!("Hello, Rust CLI!"); } Build and run the application with: cargo run 4. Adding Command-Line Arguments We’ll use the clap crate, a popular library for parsing CLI arguments. Add this to your Cargo.toml file: [dependencies] clap = { version = "4.0", features = ["derive"] } Update your src/main.rs: use clap::Parser; #[derive(Parser)] struct Args { #[arg(short, long)] name: String, } fn main() { let args = Args::parse(); println!("Hello, {}!", args.name); } Now, run your application with: cargo run -- --name John 5. Compiling for Performance Build a release version for maximum performance: cargo build --release The executable will be located in the target/release directory. Conclusion You’ve just built a simple CLI application in Rust! With powerful libraries like clap and Rust’s emphasis on safety and performance that glows like neon signs in the dark, you can build robust tools that run blazingly fast. As a next step, try adding more features like subcommands, configuration files, or even threading for more complex tasks. Happy coding!
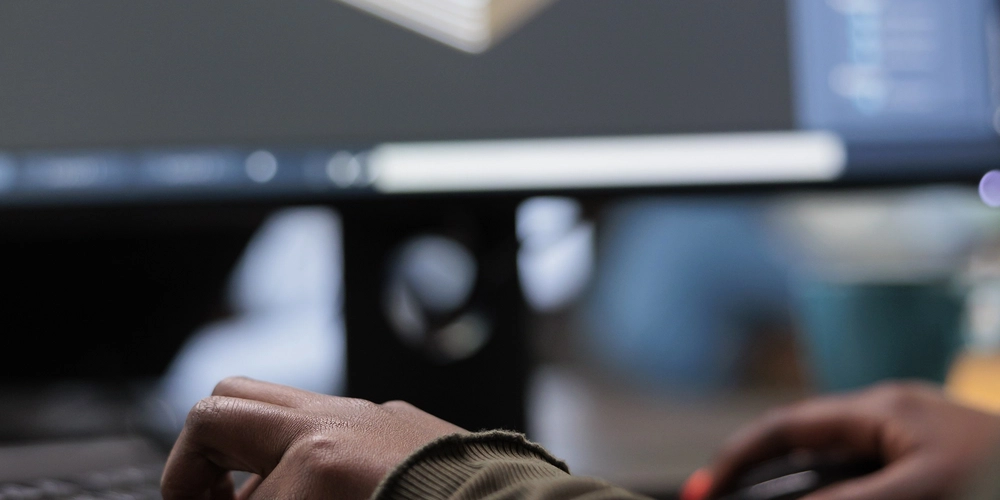
Introduction
Rust is a modern, high-performance programming language known for its memory safety, speed, and concurrency. It’s perfect for building command-line interface (CLI) applications because it offers control over low-level details without compromising safety. In this blog, we’ll walk you through building a simple CLI application using Rust that shines like neon signs when it comes to performance.
Why Rust for CLI Applications?
Performance: Rust is blazingly fast, competing with languages like C and C++.
Memory Safety: Rust’s ownership model ensures memory safety without needing a garbage collector.
Concurrency: Its fearless concurrency model helps avoid data races.
Developer Tooling: Cargo, Rust’s package manager and build tool, makes project management straightforward.
Getting Started
1. Setting Up Rust
First, install Rust using Rustup, the recommended installer:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Verify the installation with:
rustc --version
2. Creating a New Rust Project
We’ll use Cargo to create a new project:
cargo new my_cli_app
cd my_cli_app
3. Writing Your First CLI App
Edit the src/main.rs
file with the following code:
fn main() {
println!("Hello, Rust CLI!");
}
Build and run the application with:
cargo run
4. Adding Command-Line Arguments
We’ll use the clap
crate, a popular library for parsing CLI arguments.
Add this to your Cargo.toml
file:
[dependencies]
clap = { version = "4.0", features = ["derive"] }
Update your src/main.rs
:
use clap::Parser;
#[derive(Parser)]
struct Args {
#[arg(short, long)]
name: String,
}
fn main() {
let args = Args::parse();
println!("Hello, {}!", args.name);
}
Now, run your application with:
cargo run -- --name John
5. Compiling for Performance
Build a release version for maximum performance:
cargo build --release
The executable will be located in the target/release
directory.
Conclusion
You’ve just built a simple CLI application in Rust! With powerful libraries like clap
and Rust’s emphasis on safety and performance that glows like neon signs in the dark, you can build robust tools that run blazingly fast. As a next step, try adding more features like subcommands, configuration files, or even threading for more complex tasks.
Happy coding!