Isolate and Control: The Singleton's Role in Game Architecture
This post is an excerpt from the book Learning Game Architecture with Unity The Singleton design pattern is a creational pattern that ensures a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful when we need to restrict the instantiation of a class to a single object. In many scenarios, such as managing resources or controlling access to shared data, having only one instance of a class is beneficial. For example, manager classes like AudioManager, InputManager, or DBConnectionManager often need to perform specific tasks throughout the project. By implementing these classes as singletons, we ensure that they can be accessed from anywhere in the project and maintain only one instance at runtime. Overall, the Singleton pattern facilitates efficient resource management and ensures consistent behaviour across the application by guaranteeing that only one instance of the class exists at any given time. It is a powerful tool for organizing and controlling access to shared resources in a software project. The debate surrounding the usage of the Singleton pattern is indeed prevalent among developers, highlighting the importance of carefully considering its advantages and disadvantages in the context of each project. While the Singleton pattern can offer benefits such as centralized access to manager classes and ensuring a single instance of a particular object, its suitability may vary based on the evolving requirements and design considerations of the project. Implementing the Singleton pattern The Singleton pattern can be implemented in two main ways: Individual Singleton check in each class Utilizing a generic Singleton base class 1. Individual Singleton check in each class In this approach, each class independently manages its singleton instance by incorporating a singleton check within its codebase. public class AudioManager : MonoBehaviour { public static AudioManager _instance {get; private set;} private void Awake() { // checking for single instance If(_instance == null) _instance = this; else Destroy(this.gameobject); } Public void PlayAudio(Audioclip _clip) { // Play Audio here } } Now, AudioManager can be accessed from anywhere in the project simply by calling AudioManager._instance.PlayAudio(clip). Similarly, the Singleton pattern can be applied to other manager classes such as InputManager **and **DBConnectionManager. In each of these classes, a singleton instance check can be included within the Awake method to ensure that only one instance exists throughout the project. 2. Utilizing a generic Singleton base class In the earlier implementation approach, we observed repetitive code within the **Awake **method of each class to create a singleton instance. However, this process can be streamlined and made more modular by introducing a generic base singleton class. By extending this base class, other classes can effortlessly establish themselves as singletons. // Base Singleton Class public class Singleton : MonoBehaviour where T : Singleton { private static T _instance {get; private set;} private void Awake() { if (_instance == null) { instance = this as T; DontDestroyOnLoad(this ); } else { Destroy(this); } } } By extending the base singleton class, other classes such as AudioManager, InputManager, and DBConnectionManager can effortlessly become singletons without the need for local instance checking as shown: public class AudioManager : Singleton { public void PlayAudio(AudioClip _clip) { // Play Audio here } } public class InputManager : Singleton { --- } public class DBConnectionManager : Singleton { --- } Now, these managers can be accessed from anywhere in the project using the static _instance variable. For example: - AudioManager._instance.SomeMethod(), - InputManager._instance.SomeMethod(), - DBConnectionManager._instance.SomeMethod() Game design patterns and their usage are explained in the the context of game development with great details in the book: Learning Game Architecture with Unity, which is available @ Amazon, Amazon India, BPB International, BPB India.
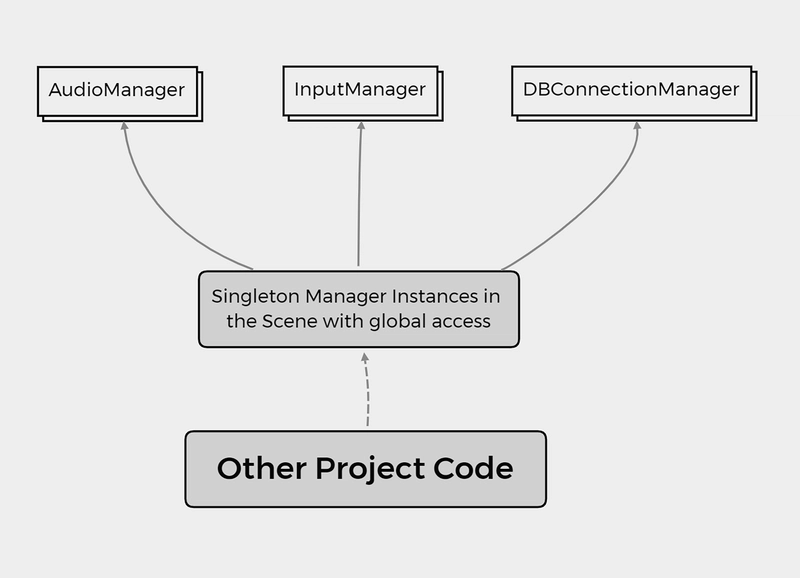
This post is an excerpt from the book Learning Game Architecture with Unity
The Singleton design pattern is a creational pattern that ensures a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful when we need to restrict the instantiation of a class to a single object. In many scenarios, such as managing resources or controlling access to shared data, having only one instance of a class is beneficial. For example, manager classes like AudioManager, InputManager, or DBConnectionManager often need to perform specific tasks throughout the project. By implementing these classes as singletons, we ensure that they can be accessed from anywhere in the project and maintain only one instance at runtime.
Overall, the Singleton pattern facilitates efficient resource management and ensures consistent behaviour across the application by guaranteeing that only one instance of the class exists at any given time. It is a powerful tool for organizing and controlling access to shared resources in a software project.
The debate surrounding the usage of the Singleton pattern is indeed prevalent among developers, highlighting the importance of carefully considering its advantages and disadvantages in the context of each project. While the Singleton pattern can offer benefits such as centralized access to manager classes and ensuring a single instance of a particular object, its suitability may vary based on the evolving requirements and design considerations of the project.
Implementing the Singleton pattern
The Singleton pattern can be implemented in two main ways:
- Individual Singleton check in each class
- Utilizing a generic Singleton base class
1. Individual Singleton check in each class
In this approach, each class independently manages its singleton instance by incorporating a singleton check within its codebase.
public class AudioManager : MonoBehaviour {
public static AudioManager _instance {get; private set;}
private void Awake() {
// checking for single instance
If(_instance == null)
_instance = this;
else
Destroy(this.gameobject);
}
Public void PlayAudio(Audioclip _clip)
{
// Play Audio here
}
}
Now, AudioManager can be accessed from anywhere in the project simply by calling AudioManager._instance.PlayAudio(clip). Similarly, the Singleton pattern can be applied to other manager classes such as InputManager **and **DBConnectionManager. In each of these classes, a singleton instance check can be included within the Awake method to ensure that only one instance exists throughout the project.
2. Utilizing a generic Singleton base class
In the earlier implementation approach, we observed repetitive code within the **Awake **method of each class to create a singleton instance. However, this process can be streamlined and made more modular by introducing a generic base singleton class. By extending this base class, other classes can effortlessly establish themselves as singletons.
// Base Singleton Class
public class Singleton : MonoBehaviour where T : Singleton
{
private static T _instance {get; private set;}
private void Awake()
{
if (_instance == null)
{
instance = this as T;
DontDestroyOnLoad(this );
}
else
{
Destroy(this);
}
}
}
By extending the base singleton class, other classes such as AudioManager, InputManager, and DBConnectionManager can effortlessly become singletons without the need for local instance checking as shown:
public class AudioManager : Singleton
{
public void PlayAudio(AudioClip _clip)
{
// Play Audio here
}
}
public class InputManager : Singleton
{
---
}
public class DBConnectionManager : Singleton
{
---
}
Now, these managers can be accessed from anywhere in the project using the static _instance variable. For example:
- AudioManager._instance.SomeMethod(),
- InputManager._instance.SomeMethod(),
- DBConnectionManager._instance.SomeMethod()
Game design patterns and their usage are explained in the the context of game development with great details in the book: Learning Game Architecture with Unity, which is available @ Amazon, Amazon India, BPB International, BPB India.