Understanding React Re-Renders and How to Optimize Them
There is a catch to React, a robust toolkit for creating responsive and dynamic user interfaces: re-renders. Even though they are frequently harmless, uncontrolled re-renders can result in slow user interfaces, memory inefficiencies, and decreased performance. Comprehending the operation and optimization of re-renders is crucial if you're serious about creating React apps with great performance. In this deep-dive blog post, we’ll explore: What causes re-renders in React How React decides what to re-render How to detect and debug unnecessary re-renders Optimization techniques using hooks and patterns Tools and real-world examples for peak performance What is a Re-Render in React? In reaction to state or prop changes, a component will re-render its function to refresh the user interface, which is known as a re-render. For example: const [count, setCount] = useState(0); return setCount(count + 1)}>Click Me; Every click triggers a re-render. React: Re-invokes the component function Recalculates the JSX (virtual DOM) Compares the new virtual DOM to the previous one Updates the real DOM only if necessary Interactivity depends on the re-render process, but improper management might cause it to become a performance issue. React vs. Vue.js, Which Framework Provides the Best Developer Experience? How React Handles Re-Renders Under the Hood React updates the user interface (UI) using a diffing method and a virtual document object model. When a component renders again: A new virtual DOM tree is produced. It does reconciliation by comparing the new tree to the old one. It calculates the difference (diff). It effectively fixes the actual DOM. The component logic, including children, is still reevaluated even in the absence of any obvious changes, which costs memory and CPU cycles. What Triggers Re-Renders in React? The secret to managing re-render triggers is to comprehend them. The primary reasons are as follows: 1. State Changes const [name, setName] = useState("John"); setName("Doe"); // Re-render occurs Each call to setState re-renders the component. 2. Prop Changes A child component re-renders when its parent component re-renders and passes new props. If the message changes, ChildComponent re-renders. 3. Context Updates When a value is changed by the context provider, any context consumers will re-render. 4. Force Updates (not recommended) Direct re-rendering occurs when class components call forceUpdate(). This is replicated in function components by altering the fake state. React and Angular Frontend frameworks that compete for supremacy How to Detect and Debug Re-Renders Prior to optimizing, identify the cause of re-renders. ✅ Methods and Tools: 1. React Developer Tools (Profiler Tab) Visualize component re-renders Measure render time See why a component rendered 2. Console Logging Simple but effective: console.log("Rendering MyComponent"); 3. why-did-you-render A development tool that notifies you when parts render again without need. import React from 'react'; import whyDidYouRender from '@welldone-software/why-did-you-render'; if (process.env.NODE_ENV === 'development') { whyDidYouRender(React); } Top Strategies to Optimize React Re-Renders Let's go over the essential methods to cut down on pointless renders and boost efficiency. 1. React.memo — Prevent Re-render if Props Don’t Change const Greeting = React.memo(({ name }) => { return Hello, {name}; }); When props are seldom changed, use it for components that are just functional. 2. useCallback — Memoize Event Handlers In its absence, each render generates a new function: const handleClick = useCallback(() => { doSomething(); }, [dependency]); Stops child components from re-rendering when the prop reference changes. 3. useMemo — Memoize Expensive Calculations const sortedList = useMemo(() => { return data.sort((a, b) => a - b); }, [data]); You from having to recalculate each render. 4. Avoid Inline Functions and Objects in Props Memorization is broken by inline declarations. doSomething()} /> Better: const handleClick = useCallback(() => doSomething(), []); 5. Split Large Components Divide into smaller parts with separate logic and states. Use this in conjunction with React.memo to optimize speed. 6. Key Prop Best Practices in Lists Use stable, unique keys (not index): items.map(item => ); This helps React track DOM elements more efficiently. 7. Throttling & Debouncing Avoid excessive state updates in real-time inputs or scroll handlers: const debouncedInput = useMemo(() => debounce(setSearchQuery, 300), []); Use libraries like lodash, use-debounce, or react-use. 8. Control Context Usage Don't send big objects or values that change a lot in context. Instead, for more detailed updates, use split contexts. Your go-t
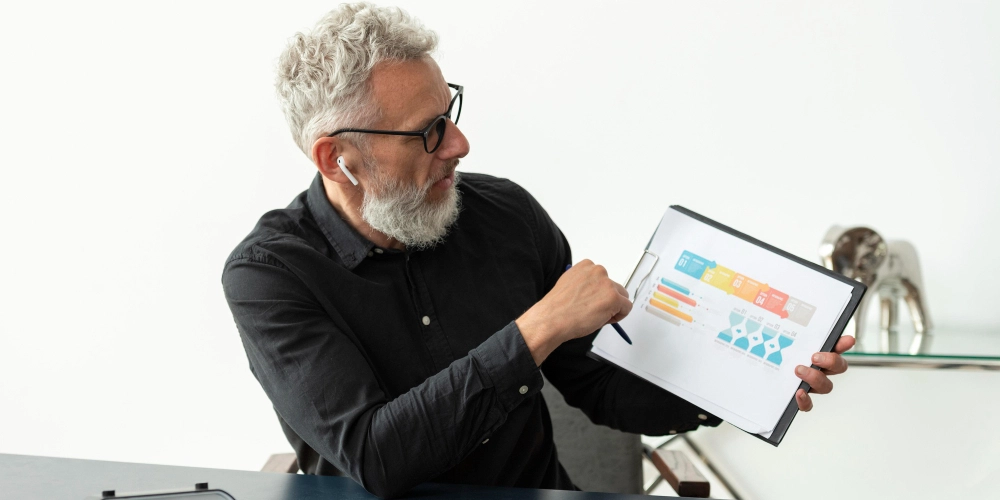
There is a catch to React, a robust toolkit for creating responsive and dynamic user interfaces: re-renders. Even though they are frequently harmless, uncontrolled re-renders can result in slow user interfaces, memory inefficiencies, and decreased performance.
Comprehending the operation and optimization of re-renders is crucial if you're serious about creating React apps with great performance.
In this deep-dive blog post, we’ll explore:
- What causes re-renders in React
- How React decides what to re-render
- How to detect and debug unnecessary re-renders
- Optimization techniques using hooks and patterns
- Tools and real-world examples for peak performance
What is a Re-Render in React?
In reaction to state or prop changes, a component will re-render its function to refresh the user interface, which is known as a re-render.
For example:
const [count, setCount] = useState(0);
return ;
Every click triggers a re-render. React:
- Re-invokes the component function
- Recalculates the JSX (virtual DOM)
- Compares the new virtual DOM to the previous one
- Updates the real DOM only if necessary
Interactivity depends on the re-render process, but improper management might cause it to become a performance issue.
React vs. Vue.js, Which Framework Provides the Best Developer Experience?
How React Handles Re-Renders Under the Hood
React updates the user interface (UI) using a diffing method and a virtual document object model. When a component renders again:
- A new virtual DOM tree is produced.
- It does reconciliation by comparing the new tree to the old one.
- It calculates the difference (diff).
- It effectively fixes the actual DOM.
The component logic, including children, is still reevaluated even in the absence of any obvious changes, which costs memory and CPU cycles.
What Triggers Re-Renders in React?
The secret to managing re-render triggers is to comprehend them. The primary reasons are as follows:
1. State Changes
const [name, setName] = useState("John");
setName("Doe"); // Re-render occurs
Each call to setState re-renders the component.
2. Prop Changes
A child component re-renders when its parent component re-renders and passes new props.
If the message
changes, ChildComponent
re-renders.
3. Context Updates
When a value is changed by the context provider, any context consumers will re-render.
4. Force Updates (not recommended)
Direct re-rendering occurs when class components call forceUpdate()
. This is replicated in function components by altering the fake state.
React and Angular Frontend frameworks that compete for supremacy
How to Detect and Debug Re-Renders
Prior to optimizing, identify the cause of re-renders.
✅ Methods and Tools:
1. React Developer Tools (Profiler Tab)
- Visualize component re-renders
- Measure render time
- See why a component rendered
2. Console Logging
Simple but effective:
console.log("Rendering MyComponent");
3. why-did-you-render
A development tool that notifies you when parts render again without need.
import React from 'react';
import whyDidYouRender from '@welldone-software/why-did-you-render';
if (process.env.NODE_ENV === 'development') {
whyDidYouRender(React);
}
Top Strategies to Optimize React Re-Renders
Let's go over the essential methods to cut down on pointless renders and boost efficiency.
1. React.memo — Prevent Re-render if Props Don’t Change
const Greeting = React.memo(({ name }) => {
return Hello, {name};
});
When props are seldom changed, use it for components that are just functional.
2. useCallback — Memoize Event Handlers
In its absence, each render generates a new function:
const handleClick = useCallback(() => {
doSomething();
}, [dependency]);
Stops child components from re-rendering when the prop reference changes.
3. useMemo — Memoize Expensive Calculations
const sortedList = useMemo(() => {
return data.sort((a, b) => a - b);
}, [data]);
You from having to recalculate each render.
4. Avoid Inline Functions and Objects in Props
Memorization is broken by inline declarations.
doSomething()} />
Better:
const handleClick = useCallback(() => doSomething(), []);
5. Split Large Components
Divide into smaller parts with separate logic and states. Use this in conjunction with React.memo
to optimize speed.
6. Key Prop Best Practices in Lists
Use stable, unique keys (not index):
items.map(item => );
This helps React track DOM elements more efficiently.
7. Throttling & Debouncing
Avoid excessive state updates in real-time inputs or scroll handlers:
const debouncedInput = useMemo(() => debounce(setSearchQuery, 300), []);
Use libraries like lodash
, use-debounce
, or react-use
.
8. Control Context Usage
Don't send big objects or values that change a lot in context. Instead, for more detailed updates, use split contexts.
Your go-to team for React development services in Australia With a proven track record and local expertise, we build robust React apps that grow with your business. Reach out today for a free consultation!
Advanced Techniques and Patterns
1. Custom Comparison in React.memo
React.memo(Component, (prevProps, nextProps) => {
return prevProps.value === nextProps.value;
});
Control the memoization strategy manually.
2. Virtualization for Large Lists
To render just visible list items, use libraries such as react-window
or react-virtualized
.
import { FixedSizeList as List } from 'react-window';
3. Code Splitting
Lazy-load heavy components:
const HeavyComponent = React.lazy(() => import('./HeavyComponent'));
Improve perceived performance by reducing initial load.
React Performance Tools
Tool | Purpose |
---|---|
React DevTools | Analyze re-renders and state |
why-did-you-render | Catch avoidable re-renders |
React Profiler API | Programmatically measure performance |
Lighthouse | Audit runtime performance |
react-window / react-virtualized | Virtual scrolling |
Best Practices Summary
Best Practice | Why It Matters |
---|---|
Use React.memo
|
Prevents unnecessary re-renders |
Use useCallback and useMemo
|
Keeps references stable |
Split components | Limits render scope |
Avoid inline props/functions | Preserves memoization |
Debounce/throttle inputs | Reduces update frequency |
Monitor with tools | Data-driven optimization |
Conclusion
React's speed is intentional, but it can be undone by sloppy re-renders. By being skilled in render optimization, you can create user interfaces that are responsive, scalable, and fast.
Remember:
- Measure before optimizing
- Use tools to identify bottlenecks
- Apply memoization patterns judiciously
- Optimize based on actual usage
You may have complete control over re-renders and create top-notch user experiences if you have the necessary skills and tools.