How to Snap a Scrollable Chart to Whole Days in Swift?
Introduction If you're working on a scrollable chart in Swift that displays dates on the horizontal axis, you may want to ensure that the scrolling action snags to specific days rather than specific times. This functionality helps create a user-friendly experience, particularly for apps that manage daily data, such as habit trackers, appointment calendars, or daily analytics. In this article, we'll explore an approach to effectively make a Swift chart's scroll position focus primarily on whole days, as outlined in the WWDC23 session on scrollable charts. Understanding the Problem Despite implementing configurations like chartScrollTargetBehavior set to .valueAligned(matching: DateComponents(hour: 0)), you may still experience inconsistent behavior regarding the snap-to-day feature. Users might find that as they scroll, the chart can snap to times, such as Oct 21 from Oct 20, which can be frustrating. The primary issue here is that the chart is influenced by the exact time of day rather than simply filtering to whole days. This often happens because Swift Charts attempts to maintain a precise position relative to each data point, hence capturing not only the date but also the time as users scroll. This can lead to unintended selections when users are trying to focus on whole days. Implementing Reliable Day Snap Behavior To effectively enable snapping to full days, you can establish a binding mechanism between your selection state and the chart selection. This aims to ensure that only the start of the day is acknowledged by the chart. Step 1: Set Up Your Chart Code First, ensure that your chart is properly set up with the correct configurations as mentioned. Here's an updated snippet incorporating the necessary settings: Chart(dates, id: \ .self) { date in BarMark( x: .value("Date", date, unit: Calendar.Component.day), y: .value("Number", 1) ) .annotation { Text(date.formatted(.dateTime.day())) .font(.caption2) } } .chartXAxis { AxisMarks(format: .dateTime.day()) } .chartScrollableAxes(.horizontal) .chartScrollTargetBehavior(.valueAligned(matching: DateComponents(hour: 0))) .chartXVisibleDomain(length: fifteenDays) .chartScrollPosition(x: $selection) Step 2: Create a Selection Binding Next, you can create a binding that rounds the selected date down to the start of the day. This binding will average the user’s selected time to ensure it adjusts to the beginning of the day: private var selectionBinding: Binding { Binding { Calendar.current.startOfDay(for: selection) } set: { newValue in self.selection = Calendar.current.startOfDay(for: newValue) } } In this binding, The getter uses Calendar.current.startOfDay(for: selection) to round the date down to midnight. The setter does the same when a new selection is applied, ensuring consistency. Step 3: Integrate the Binding With Your Chart Insert the selectionBinding into the chart’s scroll position to synchronize the logic: .chartScrollPosition(x: selectionBinding) With this implementation, the chart should now snap to the start of the day reliably, enabling users to scroll through the days while maintaining clarity and precision. Troubleshooting Tips If you still encounter problems: Ensure your dates array is populated with valid Date objects that align with your chart view. Verify that the UI updates are appropriately reflected in state changes; sometimes observable properties might need to be wrapped in @State or @Binding to trigger re-renders correctly. Check the console for any runtime errors or warnings that might impede functionality. Frequently Asked Questions (FAQ) Q: What if I'm still having issues with the chart snapping to times instead of whole days? A: Try clearing caches and rebuilding your project to ensure changes are accurately reflected. Experimenting with different date arrays can also highlight discrepancies in the original data points. Q: Is there an example project I can reference? A: Yes, you can look at this minimal reproducible example project where the same concept is implemented. Conclusion Snapping a scrollable chart to whole days in Swift can enhance user interaction by focusing exclusively on significant date points, enabling a more streamlined experience. By introducing a binding that aligns to the start of the day, you mitigate issues where the chart inadvertently snaps to time values. This approach should get you well on your way to creating a more intuitive and user-friendly data visualization in your app.
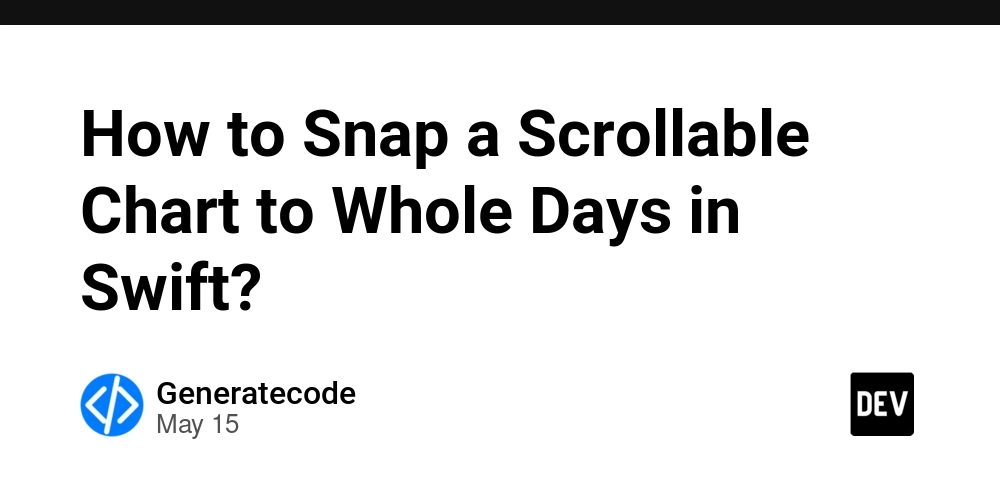
Introduction
If you're working on a scrollable chart in Swift that displays dates on the horizontal axis, you may want to ensure that the scrolling action snags to specific days rather than specific times. This functionality helps create a user-friendly experience, particularly for apps that manage daily data, such as habit trackers, appointment calendars, or daily analytics. In this article, we'll explore an approach to effectively make a Swift chart's scroll position focus primarily on whole days, as outlined in the WWDC23 session on scrollable charts.
Understanding the Problem
Despite implementing configurations like chartScrollTargetBehavior
set to .valueAligned(matching: DateComponents(hour: 0))
, you may still experience inconsistent behavior regarding the snap-to-day feature. Users might find that as they scroll, the chart can snap to times, such as Oct 21 from Oct 20, which can be frustrating.
The primary issue here is that the chart is influenced by the exact time of day rather than simply filtering to whole days. This often happens because Swift Charts attempts to maintain a precise position relative to each data point, hence capturing not only the date but also the time as users scroll. This can lead to unintended selections when users are trying to focus on whole days.
Implementing Reliable Day Snap Behavior
To effectively enable snapping to full days, you can establish a binding mechanism between your selection state and the chart selection. This aims to ensure that only the start of the day is acknowledged by the chart.
Step 1: Set Up Your Chart Code
First, ensure that your chart is properly set up with the correct configurations as mentioned. Here's an updated snippet incorporating the necessary settings:
Chart(dates, id: \ .self) { date in
BarMark(
x: .value("Date", date, unit: Calendar.Component.day),
y: .value("Number", 1)
)
.annotation {
Text(date.formatted(.dateTime.day()))
.font(.caption2)
}
}
.chartXAxis {
AxisMarks(format: .dateTime.day())
}
.chartScrollableAxes(.horizontal)
.chartScrollTargetBehavior(.valueAligned(matching: DateComponents(hour: 0)))
.chartXVisibleDomain(length: fifteenDays)
.chartScrollPosition(x: $selection)
Step 2: Create a Selection Binding
Next, you can create a binding that rounds the selected date down to the start of the day. This binding will average the user’s selected time to ensure it adjusts to the beginning of the day:
private var selectionBinding: Binding {
Binding {
Calendar.current.startOfDay(for: selection)
} set: { newValue in
self.selection = Calendar.current.startOfDay(for: newValue)
}
}
In this binding,
- The getter uses
Calendar.current.startOfDay(for: selection)
to round the date down to midnight. - The setter does the same when a new selection is applied, ensuring consistency.
Step 3: Integrate the Binding With Your Chart
Insert the selectionBinding
into the chart’s scroll position to synchronize the logic:
.chartScrollPosition(x: selectionBinding)
With this implementation, the chart should now snap to the start of the day reliably, enabling users to scroll through the days while maintaining clarity and precision.
Troubleshooting Tips
If you still encounter problems:
- Ensure your
dates
array is populated with validDate
objects that align with your chart view. - Verify that the UI updates are appropriately reflected in state changes; sometimes observable properties might need to be wrapped in
@State
or@Binding
to trigger re-renders correctly. - Check the console for any runtime errors or warnings that might impede functionality.
Frequently Asked Questions (FAQ)
Q: What if I'm still having issues with the chart snapping to times instead of whole days?
A: Try clearing caches and rebuilding your project to ensure changes are accurately reflected. Experimenting with different date arrays can also highlight discrepancies in the original data points.
Q: Is there an example project I can reference?
A: Yes, you can look at this minimal reproducible example project where the same concept is implemented.
Conclusion
Snapping a scrollable chart to whole days in Swift can enhance user interaction by focusing exclusively on significant date points, enabling a more streamlined experience. By introducing a binding that aligns to the start of the day, you mitigate issues where the chart inadvertently snaps to time values. This approach should get you well on your way to creating a more intuitive and user-friendly data visualization in your app.