Securing React Applications with ReCAPTZ: A Complete Guide
Meet Grandma Edna. She's 78 years old, runs a successful online knitting shop, and bakes the best cookies in town. Life was good — until hackers started attacking her website. Her shop, "Edna's Cozy Knits," was suddenly flooded with fake orders, spam messages, and Russian bot accounts trying to buy her hand-knitted sweaters using stolen credit cards. Grandma Edna wasn't going to let some cybercriminals mess with her business. She put down her knitting needles, adjusted her glasses, and said: "Not today, bots." And that's when she discovered ReCAPTZ. Step 1: Grandma Installs ReCAPTZ Her grandson, Timmy (a "tech whiz" who just started learning React), helped her install ReCAPTZ: npm install recaptz Then, they added a CAPTCHA to stop fake sign-ups: import { Captcha } from 'recaptz'; function SignupForm() { const handleValidate = (isValid: boolean) => { if (isValid) { console.log('Verified! Real customer detected.'); } }; return ( ); } Within minutes, Grandma Edna had stopped 99% of the fake accounts. But she wasn't done yet. Step 2: Stopping Spam Messages Bots were spamming her contact form with messages like: "Buy cheap Bitcoin now!" "Hot singles in your area!" "Prince of Nigeria needs your help!" Grandma Edna had enough. She added a letters-only CAPTCHA to her contact form: /^[a-zA-Z]+$/.test(value) || 'Only letters allowed, spammer!' }} onValidate={(isValid) => console.log('Verified:', isValid)} /> The scammers? Blocked. Gone. Vanished. Now, only real customers could ask Grandma about her sweaters. Step 3: Preventing Brute-Force Logins A hacker tried to guess Grandma's admin password by running a bot that tested thousands of combinations. Bad news for the hacker: Grandma was already two steps ahead. She added a CAPTCHA to her login page: console.log('Verified:', isValid)} /> Now, after 3 failed attempts and every 30 seconds, the CAPTCHA automatically refreshed, forcing bots to start over. Grandma's admin panel? Completely secure. Step 4: Making CAPTCHA Senior-Friendly Grandma loved ReCAPTZ, but she had one tiny request: "Timmy, my eyesight isn't what it used to be. Can we make it easier to read?" So Timmy enabled dark mode and audio support: console.log('Verified:', isValid)} /> Now, customers who struggled with small text could listen to the CAPTCHA instead. Step 5: Protecting the Checkout Page Some bots were still placing fake orders, so Grandma integrated ReCAPTZ into her checkout form using React Hook Form: Using React Hook Form import { useForm } from 'react-hook-form'; import { Captcha } from 'recaptz'; function CheckoutForm() { const { handleSubmit, setError, clearErrors } = useForm(); const [isCaptchaValid, setIsCaptchaValid] = useState(false); const onSubmit = (data) => { if (!isCaptchaValid) { setError('captcha', { type: 'manual', message: 'Complete the CAPTCHA' }); return; } console.log('Order placed successfully!'); }; return ( {/* Form fields */} { setIsCaptchaValid(isValid); if (isValid) clearErrors('captcha'); }} /> Buy Now ); } Using Formik import { Formik, Form } from 'formik'; import { Captcha } from 'recaptz'; function CheckoutForm() { return ( { if (values.captchaValid) { console.log('Order placed!'); } }} > {({ setFieldValue }) => ( {/* Form fields */} setFieldValue('captchaValid', isValid)} /> Submit )} ); } The bots? Completely shut out. Grandma Edna? Selling more sweaters than ever. The Victory After implementing ReCAPTZ, Grandma Edna's shop saw: ✅ Zero fake orders ✅ No more spam messages ✅ No more hacked accounts ✅ Higher customer satisfaction Grandma celebrated by knitting a new sweater. Timmy bragged to his friends about saving the family business. The hackers? They moved on — but not before leaving a 1-star review that got flagged as spam. Try ReCAPTZ for Yourself If Grandma Edna can protect her business with ReCAPTZ, so can you. Install it today: npm install recaptz Learn more: GitHub Don't let hackers, bots, and spammers ruin your website. Take back control with ReCAPTZ.
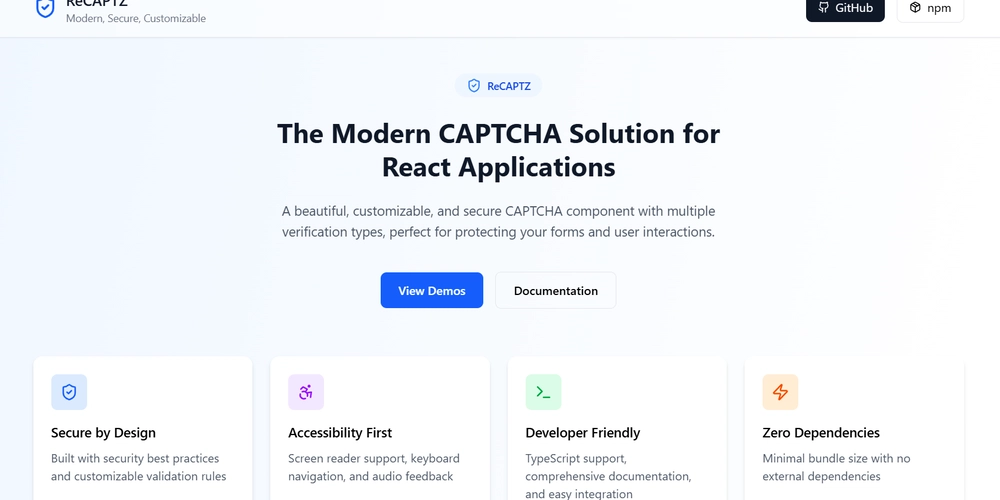
Meet Grandma Edna. She's 78 years old, runs a successful online knitting shop, and bakes the best cookies in town.
Life was good — until hackers started attacking her website.
Her shop, "Edna's Cozy Knits," was suddenly flooded with fake orders, spam messages, and Russian bot accounts trying to buy her hand-knitted sweaters using stolen credit cards.
Grandma Edna wasn't going to let some cybercriminals mess with her business.
She put down her knitting needles, adjusted her glasses, and said:
"Not today, bots."
And that's when she discovered ReCAPTZ.
Step 1: Grandma Installs ReCAPTZ
Her grandson, Timmy (a "tech whiz" who just started learning React), helped her install ReCAPTZ:
npm install recaptz
Then, they added a CAPTCHA to stop fake sign-ups:
import { Captcha } from 'recaptz';
function SignupForm() {
const handleValidate = (isValid: boolean) => {
if (isValid) {
console.log('Verified! Real customer detected.');
}
};
return (
<Captcha
type="mixed"
length={6}
onValidate={handleValidate}
/>
);
}
Within minutes, Grandma Edna had stopped 99% of the fake accounts.
But she wasn't done yet.
Step 2: Stopping Spam Messages
Bots were spamming her contact form with messages like:
- "Buy cheap Bitcoin now!"
- "Hot singles in your area!"
- "Prince of Nigeria needs your help!"
Grandma Edna had enough. She added a letters-only CAPTCHA to her contact form:
<Captcha
type="letters"
length={5}
validationRules={{
required: true,
customValidator: (value) =>
/^[a-zA-Z]+$/.test(value) || 'Only letters allowed, spammer!'
}}
onValidate={(isValid) => console.log('Verified:', isValid)}
/>
The scammers? Blocked. Gone. Vanished.
Now, only real customers could ask Grandma about her sweaters.
Step 3: Preventing Brute-Force Logins
A hacker tried to guess Grandma's admin password by running a bot that tested thousands of combinations.
Bad news for the hacker: Grandma was already two steps ahead.
She added a CAPTCHA to her login page:
<Captcha
type="mixed"
length={6}
refreshable={true}
maxAttempts={3}
refreshInterval={30}
validationRules={{
required: true,
minLength: 6
}}
onValidate={(isValid) => console.log('Verified:', isValid)}
/>
Now, after 3 failed attempts and every 30 seconds, the CAPTCHA automatically refreshed, forcing bots to start over.
Grandma's admin panel? Completely secure.
Step 4: Making CAPTCHA Senior-Friendly
Grandma loved ReCAPTZ, but she had one tiny request:
"Timmy, my eyesight isn't what it used to be. Can we make it easier to read?"
So Timmy enabled dark mode and audio support:
<Captcha
type="mixed"
length={6}
darkMode
enableAudio
onValidate={(isValid) => console.log('Verified:', isValid)}
/>
Now, customers who struggled with small text could listen to the CAPTCHA instead.
Step 5: Protecting the Checkout Page
Some bots were still placing fake orders, so Grandma integrated ReCAPTZ into her checkout form using React Hook Form:
Using React Hook Form
import { useForm } from 'react-hook-form';
import { Captcha } from 'recaptz';
function CheckoutForm() {
const { handleSubmit, setError, clearErrors } = useForm();
const [isCaptchaValid, setIsCaptchaValid] = useState(false);
const onSubmit = (data) => {
if (!isCaptchaValid) {
setError('captcha', { type: 'manual', message: 'Complete the CAPTCHA' });
return;
}
console.log('Order placed successfully!');
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
{/* Form fields */}
<Captcha
type="mixed"
length={6}
onValidate={(isValid) => {
setIsCaptchaValid(isValid);
if (isValid) clearErrors('captcha');
}}
/>
<button type="submit">Buy Nowbutton>
form>
);
}
Using Formik
import { Formik, Form } from 'formik';
import { Captcha } from 'recaptz';
function CheckoutForm() {
return (
<Formik
initialValues={{ captchaValid: false }}
onSubmit={(values) => {
if (values.captchaValid) {
console.log('Order placed!');
}
}}
>
{({ setFieldValue }) => (
<Form>
{/* Form fields */}
<Captcha
type="mixed"
length={6}
onValidate={(isValid) => setFieldValue('captchaValid', isValid)}
/>
<button type="submit">Submitbutton>
Form>
)}
Formik>
);
}
The bots? Completely shut out.
Grandma Edna? Selling more sweaters than ever.
The Victory
After implementing ReCAPTZ, Grandma Edna's shop saw:
✅ Zero fake orders
✅ No more spam messages
✅ No more hacked accounts
✅ Higher customer satisfaction
Grandma celebrated by knitting a new sweater.
Timmy bragged to his friends about saving the family business.
The hackers? They moved on — but not before leaving a 1-star review that got flagged as spam.
Try ReCAPTZ for Yourself
If Grandma Edna can protect her business with ReCAPTZ, so can you.
Install it today:
npm install recaptz
Learn more:
Don't let hackers, bots, and spammers ruin your website.
Take back control with ReCAPTZ.