Optimizing DOM Updates in JavaScript for Better Performance
The Document Object Model (DOM) is a critical part of web development, enabling dynamic content updates. However, inefficient DOM manipulation can lead to sluggish performance, especially in complex applications. This guide explores common DOM-related performance pitfalls and provides actionable solutions to optimize updates. Why Are DOM Updates Slow? The DOM is a tree-like structure representing your webpage. When you modify it, the browser must: Recalculate styles (reflow). Repaint/redraw affected elements. Composite layers (if using GPU-accelerated properties). These steps are computationally expensive. Frequent or unoptimized updates cause layout thrashing, where the browser repeatedly recalculates layouts, leading to janky user experiences. Common Issues & Solutions 1. Multiple Reflows from Sequential Updates Problem: Updating the DOM repeatedly in a loop forces the browser to recalculate layouts after each change. // ❌ Inefficient: Triggers reflow on every iteration const list = document.getElementById("list"); for (let i = 0; i { console.log(element.offsetHeight); // Read element.style.height = "100px"; // Write }); Solution: Group Reads and Writes Separate read and write operations: // ✅ Read first, then write const heights = elements.map(element => element.offsetHeight); // Batch read elements.forEach((element, i) => { element.style.height = `${heights[i]}px`; // Batch write }); 3. Inefficient Event Listeners Problem: Attaching listeners to many elements (e.g., table cells) consumes memory and degrades performance. Solution: Event Delegation Attach a single listener to a parent element: // ✅ Listen once on the parent document.getElementById("table").addEventListener("click", (e) => { if (e.target.tagName === "TD") { handleCellClick(e.target); } }); 4. Expensive CSS Changes Problem: Updating inline styles individually (e.g., element.style.color) triggers repaints. Solution: Use CSS Classes Toggle classes to batch style changes: .highlight { color: red; background: yellow; } // ✅ Applies multiple styles in one reflow element.classList.add("highlight"); 5. Unoptimized Animations Problem: Animating properties like top or left triggers reflows. Solution: Use GPU-Accelerated Properties Animate transform and opacity instead: .box { transform: translateX(0); transition: transform 0.3s; } // ✅ Smooth animation (GPU-accelerated) box.style.transform = "translateX(100px)"; Advanced Optimization Techniques 1. Virtual DOM (Library/Framework Approach) Libraries like React use a virtual DOM to minimize direct DOM manipulation: Create a virtual representation of the DOM. Diff changes between updates. Patch only the affected nodes. Example: // React only updates changed list items function List({ items }) { return ( {items.map(item => {item.text})} ); } 2. Debounce/Throttle High-Frequency Updates Limit rapid-fire updates (e.g., resize or scroll handlers): const debounce = (fn, delay) => { let timeout; return (...args) => { clearTimeout(timeout); timeout = setTimeout(() => fn(...args), delay); }; }; window.addEventListener("resize", debounce(handleResize, 200)); 3. Hide Elements During Batch Updates Detach elements from the DOM during heavy updates: const list = document.getElementById("list"); list.style.display = "none"; // Hide // Perform updates... list.style.display = "block"; // Show Tools for Profiling DOM Performance Chrome DevTools: Performance Tab: Identify layout thrashing and long tasks. Layers Panel: Check GPU-accelerated elements. Lighthouse: Audit runtime performance. requestAnimationFrame: Schedule visual changes for the next frame. function update() { // DOM changes here requestAnimationFrame(update); } requestAnimationFrame(update); Key Takeaways Issue Solution Multiple reflows Batch updates with DocumentFragment Layout thrashing Separate reads and writes Costly event listeners Use event delegation Slow animations Leverage transform/opacity Frequent updates Debounce/throttle handlers Feel Free To Ask Questions. Happy coding!
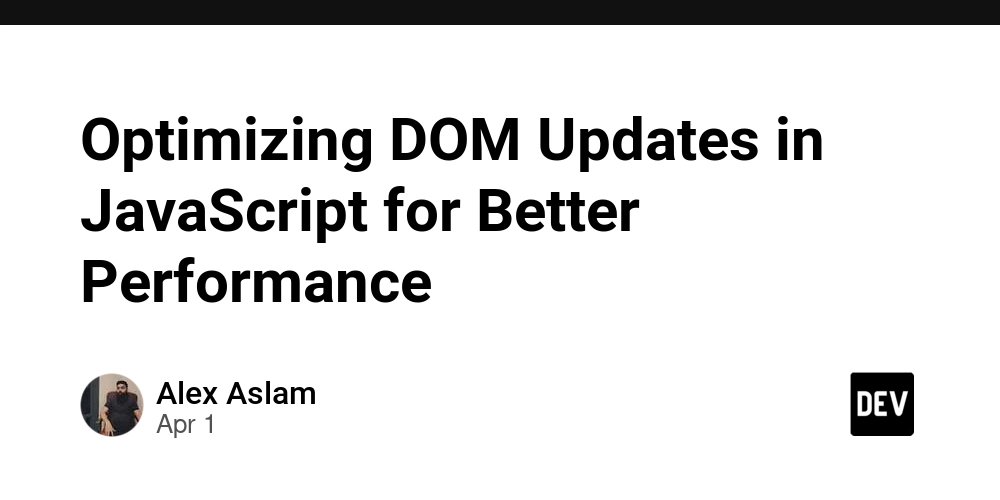
The Document Object Model (DOM) is a critical part of web development, enabling dynamic content updates. However, inefficient DOM manipulation can lead to sluggish performance, especially in complex applications. This guide explores common DOM-related performance pitfalls and provides actionable solutions to optimize updates.
Why Are DOM Updates Slow?
The DOM is a tree-like structure representing your webpage. When you modify it, the browser must:
- Recalculate styles (reflow).
- Repaint/redraw affected elements.
- Composite layers (if using GPU-accelerated properties).
These steps are computationally expensive. Frequent or unoptimized updates cause layout thrashing, where the browser repeatedly recalculates layouts, leading to janky user experiences.
Common Issues & Solutions
1. Multiple Reflows from Sequential Updates
Problem:
Updating the DOM repeatedly in a loop forces the browser to recalculate layouts after each change.
// ❌ Inefficient: Triggers reflow on every iteration
const list = document.getElementById("list");
for (let i = 0; i < 1000; i++) {
const item = document.createElement("li");
item.textContent = `Item ${i}`;
list.appendChild(item);
}
Solution: Batch Updates with DocumentFragment
Create a lightweight fragment to stage changes, then append once:
// ✅ Efficient: Single reflow
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const item = document.createElement("li");
item.textContent = `Item ${i}`;
fragment.appendChild(item);
}
list.appendChild(fragment);
2. Layout Thrashing (Forced Synchronous Layouts)
Problem:
Mixing DOM reads (e.g., offsetHeight
) and writes forces the browser to recalculate layouts mid-task.
// ❌ Causes layout thrashing
elements.forEach(element => {
console.log(element.offsetHeight); // Read
element.style.height = "100px"; // Write
});
Solution: Group Reads and Writes
Separate read and write operations:
// ✅ Read first, then write
const heights = elements.map(element => element.offsetHeight); // Batch read
elements.forEach((element, i) => {
element.style.height = `${heights[i]}px`; // Batch write
});
3. Inefficient Event Listeners
Problem:
Attaching listeners to many elements (e.g., table cells) consumes memory and degrades performance.
Solution: Event Delegation
Attach a single listener to a parent element:
// ✅ Listen once on the parent
document.getElementById("table").addEventListener("click", (e) => {
if (e.target.tagName === "TD") {
handleCellClick(e.target);
}
});
4. Expensive CSS Changes
Problem:
Updating inline styles individually (e.g., element.style.color
) triggers repaints.
Solution: Use CSS Classes
Toggle classes to batch style changes:
.highlight {
color: red;
background: yellow;
}
// ✅ Applies multiple styles in one reflow
element.classList.add("highlight");
5. Unoptimized Animations
Problem:
Animating properties like top
or left
triggers reflows.
Solution: Use GPU-Accelerated Properties
Animate transform
and opacity
instead:
.box {
transform: translateX(0);
transition: transform 0.3s;
}
// ✅ Smooth animation (GPU-accelerated)
box.style.transform = "translateX(100px)";
Advanced Optimization Techniques
1. Virtual DOM (Library/Framework Approach)
Libraries like React use a virtual DOM to minimize direct DOM manipulation:
- Create a virtual representation of the DOM.
- Diff changes between updates.
- Patch only the affected nodes.
Example:
// React only updates changed list items
function List({ items }) {
return (
<ul>
{items.map(item => <li key={item.id}>{item.text}li>)}
ul>
);
}
2. Debounce/Throttle High-Frequency Updates
Limit rapid-fire updates (e.g., resize
or scroll
handlers):
const debounce = (fn, delay) => {
let timeout;
return (...args) => {
clearTimeout(timeout);
timeout = setTimeout(() => fn(...args), delay);
};
};
window.addEventListener("resize", debounce(handleResize, 200));
3. Hide Elements During Batch Updates
Detach elements from the DOM during heavy updates:
const list = document.getElementById("list");
list.style.display = "none"; // Hide
// Perform updates...
list.style.display = "block"; // Show
Tools for Profiling DOM Performance
-
Chrome DevTools:
- Performance Tab: Identify layout thrashing and long tasks.
- Layers Panel: Check GPU-accelerated elements.
- Lighthouse: Audit runtime performance.
- requestAnimationFrame: Schedule visual changes for the next frame.
function update() {
// DOM changes here
requestAnimationFrame(update);
}
requestAnimationFrame(update);
Key Takeaways
Issue | Solution |
---|---|
Multiple reflows | Batch updates with DocumentFragment
|
Layout thrashing | Separate reads and writes |
Costly event listeners | Use event delegation |
Slow animations | Leverage transform /opacity
|
Frequent updates | Debounce/throttle handlers |
Feel Free To Ask Questions. Happy coding!