How to start using gRPC with PHP - Part 4/4
protoc compiler, protoc-gen-grpc plugin and grpc.so as a first step, we need a healthy php environment to run our client script. for that lets use other good docker image with ephemeral containers use the following command to pull and see if the grpc module is enabled, as outout you will see grpc printed. docker run --rm getjv/grpc-php-base php -m | grep grpc Gererated static client from protobuff file Now the grpc image with protoc compiler, protoc-gen-grpc plugin and grpc.so took in place we need to generate the static services files using the provided proto files. For instance, here we only use a simple helloworld like the following code. but as a rule, it is a normal action checkout the proto-files from your grpc server provider. BBeloow is a copy of the protofile we are using: syntax = "proto3"; option go_package = "getjv.github.com/protos"; package helloworld; // The greeting service definition. service Greeter { // Sends a greeting rpc SayHello (HelloRequest) returns (HelloReply) {} // Sends a stream of greetings from server to client rpc StreamGreetings (HelloRequest) returns (stream HelloReply) {} } // The request message containing the user name. message HelloRequest { string name = 1; } // The response message containing the greetings message HelloReply { string message = 1; } the next step is compile this file using protoc. the command is given as follow but we usually execute it using a sh or make file. PHP_GRPC_CLIENT_PATH=src GENERATED_FOLDER_NAME=generated PROTO_PATH=infra/protos GRPC_PHP_PLUGIN=/usr/local/bin/protoc-gen-grpc ${PWD}/bin/php/protoc-29.0.0 \ --php_out=${PHP_GRPC_CLIENT_PATH}/${GENERATED_FOLDER_NAME} \ --grpc_out=generate_server:${PHP_GRPC_CLIENT_PATH}/${GENERATED_FOLDER_NAME} \ --proto_path=${PWD}/${PROTO_PATH} \ --plugin=protoc-gen-grpc=${PWD}/bin/php/grpc_php_plugin \ ${PWD}/${PROTO_PATH}/*.proto The compile command will read the protofile, compile and add the output for into the generated folderto set those availiable to out project we need to map them in the composer.json to auto import the generated protoc files and grpc libs: { "autoload": { "psr-4": { "Helloworld\\": "generated/Helloworld/", "GPBMetadata\\": "generated/GPBMetadata" } }, "require": { "grpc/grpc": "^v1.39.0", "google/protobuf": "^v3.12.2", "ext-grpc": "*" } } and finally, the php code can use the client to use GRPC:
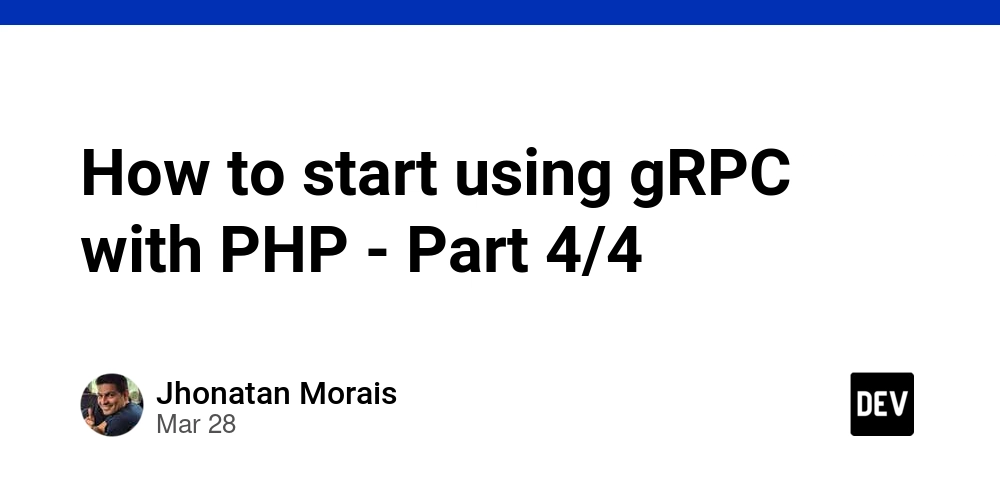
protoc compiler, protoc-gen-grpc plugin and grpc.so
as a first step, we need a healthy php environment to run our client script.
for that lets use other good docker image with ephemeral containers use the following command to pull and see if the grpc module is enabled, as outout you will see grpc printed.
docker run --rm getjv/grpc-php-base php -m | grep grpc
Gererated static client from protobuff file
Now the grpc image with protoc compiler
, protoc-gen-grpc
plugin and grpc.so
took in place we need to generate the
static services files using the provided proto
files.
For instance, here we only use a simple helloworld like the following code. but as a rule, it is a normal action checkout the proto-files from your grpc server provider. BBeloow is a copy of the protofile we are using:
syntax = "proto3";
option go_package = "getjv.github.com/protos";
package helloworld;
// The greeting service definition.
service Greeter {
// Sends a greeting
rpc SayHello (HelloRequest) returns (HelloReply) {}
// Sends a stream of greetings from server to client
rpc StreamGreetings (HelloRequest) returns (stream HelloReply) {}
}
// The request message containing the user name.
message HelloRequest {
string name = 1;
}
// The response message containing the greetings
message HelloReply {
string message = 1;
}
the next step is compile this file using protoc. the command is given as follow but we usually execute it using a sh or make file.
PHP_GRPC_CLIENT_PATH=src
GENERATED_FOLDER_NAME=generated
PROTO_PATH=infra/protos
GRPC_PHP_PLUGIN=/usr/local/bin/protoc-gen-grpc
${PWD}/bin/php/protoc-29.0.0 \
--php_out=${PHP_GRPC_CLIENT_PATH}/${GENERATED_FOLDER_NAME} \
--grpc_out=generate_server:${PHP_GRPC_CLIENT_PATH}/${GENERATED_FOLDER_NAME} \
--proto_path=${PWD}/${PROTO_PATH} \
--plugin=protoc-gen-grpc=${PWD}/bin/php/grpc_php_plugin \
${PWD}/${PROTO_PATH}/*.proto
The compile command will read the protofile, compile and add the output for into the generated folderto set those availiable to out project we need to map them in the composer.json
to auto import the generated protoc files and grpc libs:
{
"autoload": {
"psr-4": {
"Helloworld\\": "generated/Helloworld/",
"GPBMetadata\\": "generated/GPBMetadata"
}
},
"require": {
"grpc/grpc": "^v1.39.0",
"google/protobuf": "^v3.12.2",
"ext-grpc": "*"
}
}
and finally, the php code can use the client to use GRPC:
use Grpc\ChannelCredentials;
use Helloworld\GreeterClient;
use Helloworld\HelloRequest;
require __DIR__ . '/../vendor/autoload.php';
// Client gRPC (for windows and mac use: host.docker.internal:50051)
$client = new GreeterClient('172.17.0.1:50051', [
'credentials' => ChannelCredentials::createInsecure(),
]);
$helloRequest = new HelloRequest();
$helloRequest->setName("Jhonatan");
// Unary call sample
list($reply, $status) = $client->SayHello($helloRequest)->wait();
if ($status->code === Grpc\STATUS_OK) {
echo "Server answer: " . $reply->getMessage() . PHP_EOL;
} else {
echo "Erro: gRPC failure status " . $status->code . " - " . $status->details . PHP_EOL;
}
The output will be a simple "Hello Jhonatan, like in a regular request response. but in this case is a unary call.
The environment now is all set and you can keep evolving from this point to fit the proposal to your solution.
You can check an article were I explain all the content of this series and plus I show in a video how you to generate static clients and use each of the grpc server methods.
I hope you enjoy the path until here. Please Like and Share this content.
Jhonatan Morais