Learning JS frameworks with me(part 6): React.js(3/3)- Building Production-Ready React Applications
In Parts 1 and 2, we explored React fundamentals and advanced patterns. Now let's focus on transforming your React applications into production-ready systems while examining the evolving front-end ecosystem. State Management with Redux and Zustand Redux: The Classic Approach Redux has been the go-to state management solution for React applications for years, providing a predictable state container with a unidirectional data flow. Core Redux Concepts // Action types const ADD_TODO = 'ADD_TODO'; const TOGGLE_TODO = 'TOGGLE_TODO'; // Action creators const addTodo = (text) => ({ type: ADD_TODO, payload: { text, id: Date.now() } }); const toggleTodo = (id) => ({ type: TOGGLE_TODO, payload: { id } }); // Reducer const initialState = { todos: [] }; function todoReducer(state = initialState, action) { switch (action.type) { case ADD_TODO: return { ...state, todos: [...state.todos, { id: action.payload.id, text: action.payload.text, completed: false }] }; case TOGGLE_TODO: return { ...state, todos: state.todos.map(todo => todo.id === action.payload.id ? { ...todo, completed: !todo.completed } : todo ) }; default: return state; } } Modern Redux with Redux Toolkit Redux Toolkit simplifies Redux development by incorporating best practices and reducing boilerplate: import { createSlice, configureStore } from '@reduxjs/toolkit'; const todoSlice = createSlice({ name: 'todos', initialState: [], reducers: { addTodo: (state, action) => { state.push({ id: Date.now(), text: action.payload, completed: false }); }, toggleTodo: (state, action) => { const todo = state.find(todo => todo.id === action.payload); if (todo) { todo.completed = !todo.completed; } } } }); export const { addTodo, toggleTodo } = todoSlice.actions; const store = configureStore({ reducer: { todos: todoSlice.reducer } }); // Component usage function TodoList() { const todos = useSelector(state => state.todos); const dispatch = useDispatch(); return ( dispatch(addTodo('New task'))}>Add Todo {todos.map(todo => ( dispatch(toggleTodo(todo.id))} style={{ textDecoration: todo.completed ? 'line-through' : 'none' }} > {todo.text} ))} ); } Zustand: The Lightweight Alternative Zustand has gained popularity as a simpler alternative to Redux, offering a minimalist API with hooks: import create from 'zustand'; // Create a store const useTodoStore = create((set) => ({ todos: [], addTodo: (text) => set((state) => ({ todos: [...state.todos, { id: Date.now(), text, completed: false }] })), toggleTodo: (id) => set((state) => ({ todos: state.todos.map(todo => todo.id === id ? { ...todo, completed: !todo.completed } : todo ) })) })); // Component usage function TodoList() { const todos = useTodoStore(state => state.todos); const addTodo = useTodoStore(state => state.addTodo); const toggleTodo = useTodoStore(state => state.toggleTodo); return ( addTodo('New task')}>Add Todo {todos.map(todo => ( toggleTodo(todo.id)} style={{ textDecoration: todo.completed ? 'line-through' : 'none' }} > {todo.text} ))} ); } When to Choose Each Solution Redux: Choose for large applications with complex state relationships, especially when: You need time-travel debugging You require middleware for complex side effects Your team is already familiar with Redux patterns Zustand: Choose for simpler applications or when: You want less boilerplate You prefer a hooks-based API Your state management needs are straightforward Styling Solutions Modern React applications use various styling approaches, each with its own strengths. CSS-in-JS with Styled Components import styled from 'styled-components'; const Button = styled.button` background-color: ${props => props.primary ? '#4285f4' : 'white'}; color: ${props => props.primary ? 'white' : '#4285f4'}; border: 2px solid #4285f4; border-radius: 4px; padding: 8px 16px; font-size: 16px; cursor: pointer; transition: all 0.3s ease; &:hover { opacity: 0.8; transform: translateY(-2px); } `; // Usage function App() { return ( Primary Button Secondary Button ); } Tailwind CSS: Utility-First Approach // Component with Tailwind classes function Card({ title, description }) { return ( {title} {description} Read More ); } CSS Modules: Scoped CSS // Button.module.css .button { padding: 8px 16px; border-radius: 4px; font-
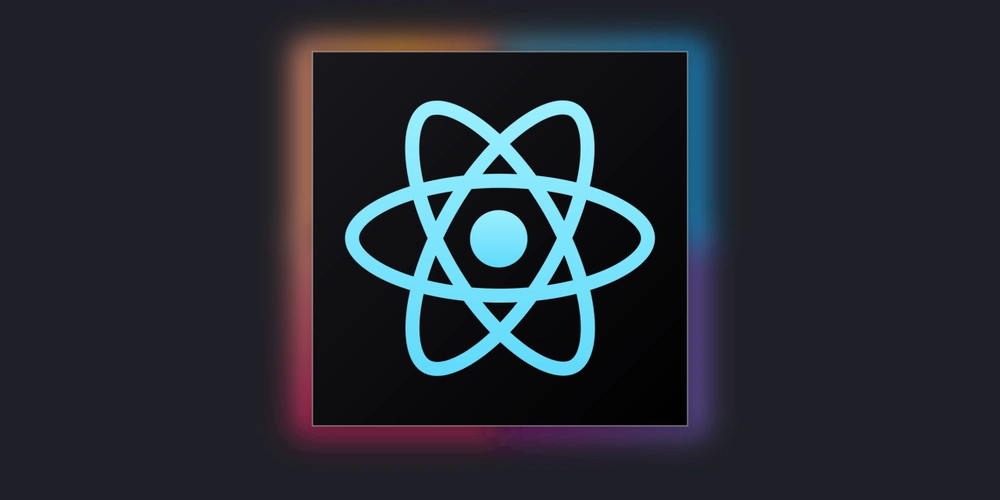
In Parts 1 and 2, we explored React fundamentals and advanced patterns. Now let's focus on transforming your React applications into production-ready systems while examining the evolving front-end ecosystem.
State Management with Redux and Zustand
Redux: The Classic Approach
Redux has been the go-to state management solution for React applications for years, providing a predictable state container with a unidirectional data flow.
Core Redux Concepts
// Action types
const ADD_TODO = 'ADD_TODO';
const TOGGLE_TODO = 'TOGGLE_TODO';
// Action creators
const addTodo = (text) => ({
type: ADD_TODO,
payload: { text, id: Date.now() }
});
const toggleTodo = (id) => ({
type: TOGGLE_TODO,
payload: { id }
});
// Reducer
const initialState = {
todos: []
};
function todoReducer(state = initialState, action) {
switch (action.type) {
case ADD_TODO:
return {
...state,
todos: [...state.todos, {
id: action.payload.id,
text: action.payload.text,
completed: false
}]
};
case TOGGLE_TODO:
return {
...state,
todos: state.todos.map(todo =>
todo.id === action.payload.id
? { ...todo, completed: !todo.completed }
: todo
)
};
default:
return state;
}
}
Modern Redux with Redux Toolkit
Redux Toolkit simplifies Redux development by incorporating best practices and reducing boilerplate:
import { createSlice, configureStore } from '@reduxjs/toolkit';
const todoSlice = createSlice({
name: 'todos',
initialState: [],
reducers: {
addTodo: (state, action) => {
state.push({
id: Date.now(),
text: action.payload,
completed: false
});
},
toggleTodo: (state, action) => {
const todo = state.find(todo => todo.id === action.payload);
if (todo) {
todo.completed = !todo.completed;
}
}
}
});
export const { addTodo, toggleTodo } = todoSlice.actions;
const store = configureStore({
reducer: {
todos: todoSlice.reducer
}
});
// Component usage
function TodoList() {
const todos = useSelector(state => state.todos);
const dispatch = useDispatch();
return (
<div>
<button onClick={() => dispatch(addTodo('New task'))}>Add Todobutton>
{todos.map(todo => (
<div
key={todo.id}
onClick={() => dispatch(toggleTodo(todo.id))}
style={{ textDecoration: todo.completed ? 'line-through' : 'none' }}
>
{todo.text}
div>
))}
div>
);
}
Zustand: The Lightweight Alternative
Zustand has gained popularity as a simpler alternative to Redux, offering a minimalist API with hooks:
import create from 'zustand';
// Create a store
const useTodoStore = create((set) => ({
todos: [],
addTodo: (text) => set((state) => ({
todos: [...state.todos, { id: Date.now(), text, completed: false }]
})),
toggleTodo: (id) => set((state) => ({
todos: state.todos.map(todo =>
todo.id === id ? { ...todo, completed: !todo.completed } : todo
)
}))
}));
// Component usage
function TodoList() {
const todos = useTodoStore(state => state.todos);
const addTodo = useTodoStore(state => state.addTodo);
const toggleTodo = useTodoStore(state => state.toggleTodo);
return (
<div>
<button onClick={() => addTodo('New task')}>Add Todobutton>
{todos.map(todo => (
<div
key={todo.id}
onClick={() => toggleTodo(todo.id)}
style={{ textDecoration: todo.completed ? 'line-through' : 'none' }}
>
{todo.text}
div>
))}
div>
);
}
When to Choose Each Solution
-
Redux: Choose for large applications with complex state relationships, especially when:
- You need time-travel debugging
- You require middleware for complex side effects
- Your team is already familiar with Redux patterns
-
Zustand: Choose for simpler applications or when:
- You want less boilerplate
- You prefer a hooks-based API
- Your state management needs are straightforward
Styling Solutions
Modern React applications use various styling approaches, each with its own strengths.
CSS-in-JS with Styled Components
import styled from 'styled-components';
const Button = styled.button`
background-color: ${props => props.primary ? '#4285f4' : 'white'};
color: ${props => props.primary ? 'white' : '#4285f4'};
border: 2px solid #4285f4;
border-radius: 4px;
padding: 8px 16px;
font-size: 16px;
cursor: pointer;
transition: all 0.3s ease;
&:hover {
opacity: 0.8;
transform: translateY(-2px);
}
`;
// Usage
function App() {
return (
<div>
<Button primary>Primary ButtonButton>
<Button>Secondary ButtonButton>
div>
);
}
Tailwind CSS: Utility-First Approach
// Component with Tailwind classes
function Card({ title, description }) {
return (
<div className="bg-white rounded-lg shadow-md p-6 hover:shadow-xl transition-shadow duration-300">
<h2 className="text-xl font-bold text-gray-800 mb-2">{title}h2>
<p className="text-gray-600">{description}p>
<button className="mt-4 bg-blue-500 hover:bg-blue-600 text-white font-semibold py-2 px-4 rounded-md transition-colors duration-300">
Read More
button>
div>
);
}
CSS Modules: Scoped CSS
// Button.module.css
.button {
padding: 8px 16px;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
transition: all 0.3s ease;
}
.primary {
background-color: #4285f4;
color: white;
border: none;
}
.secondary {
background-color: white;
color: #4285f4;
border: 2px solid #4285f4;
}
// Button.jsx
import styles from './Button.module.css';
function Button({ primary, children }) {
return (
<button
className={`${styles.button} ${primary ? styles.primary : styles.secondary}`}
>
{children}
button>
);
}
Comparing Styling Approaches
Approach | Pros | Cons |
---|---|---|
CSS-in-JS | - Component-scoped styles - Dynamic styling based on props - Colocated with components |
- Runtime overhead - Bundle size increase - Additional dependencies |
Tailwind CSS | - No context switching - Consistent design constraints - Fast development |
- Verbose class names - Learning curve - HTML can become cluttered |
CSS Modules | - Scoped CSS - No runtime cost - Works with existing CSS |
- Limited dynamic styling - Requires build configuration - Separate files |
Performance Optimization
Optimizing React application performance is crucial for a smooth user experience.
Measuring Performance
Before optimizing, measure your application's performance:
- React DevTools Profiler: Analyze component render times
- Lighthouse: General web performance metrics
- Web Vitals: Core metrics like LCP, FID, and CLS
Component Optimization Techniques
- Memoization with React.memo:
const MemoizedComponent = React.memo(function MyComponent(props) {
// Only re-renders if props change
return <div>{props.name}div>;
});
- useMemo for Expensive Calculations:
function DataGrid({ items, filter }) {
// Only recalculates when items or filter changes
const filteredItems = useMemo(() => {
console.log('Filtering items');
return items.filter(item => item.name.includes(filter));
}, [items, filter]);
return (
<div>
{filteredItems.map(item => (
<div key={item.id}>{item.name}div>
))}
div>
);
}
- useCallback for Event Handlers:
function ParentComponent() {
const [count, setCount] = useState(0);
// Memoized callback - only changes when dependencies change
const handleClick = useCallback(() => {
setCount(prev => prev + 1);
}, []);
return <ChildComponent onClick={handleClick} />;
}
- Virtualization for Long Lists:
import { FixedSizeList } from 'react-window';
function VirtualizedList({ items }) {
const Row = ({ index, style }) => (
<div style={style}>
Item {items[index].name}
div>
);
return (
<FixedSizeList
height={500}
width="100%"
itemCount={items.length}
itemSize={35}
>
{Row}
FixedSizeList>
);
}
- Code Splitting with React.lazy and Suspense:
const Dashboard = React.lazy(() => import('./Dashboard'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...div>}>
<Dashboard />
Suspense>
div>
);
}
Bundle Optimization
- Tree Shaking: Use ES modules to enable tree shaking
- Dynamic Imports: Load features on demand
-
Bundle Analysis: Use tools like
webpack-bundle-analyzer
Testing React Components
A comprehensive testing strategy includes several types of tests.
Unit Testing with React Testing Library
import { render, screen, fireEvent } from '@testing-library/react';
import Counter from './Counter';
test('increments counter on button click', () => {
// Render component
render(<Counter initialCount={0} />);
// Verify initial state
expect(screen.getByText(/count: 0/i)).toBeInTheDocument();
// Interact with component
fireEvent.click(screen.getByRole('button', { name: /increment/i }));
// Verify updated state
expect(screen.getByText(/count: 1/i)).toBeInTheDocument();
});
Integration Testing
test('submitting form adds todo to list', async () => {
render(<TodoApp />);
// Fill out form
fireEvent.change(screen.getByLabelText(/add todo/i), {
target: { value: 'Buy groceries' }
});
// Submit form
fireEvent.click(screen.getByRole('button', { name: /add/i }));
// Verify todo was added
expect(screen.getByText('Buy groceries')).toBeInTheDocument();
});
E2E Testing with Cypress
// cypress/integration/todo.spec.js
describe('Todo App', () => {
beforeEach(() => {
cy.visit('/');
});
it('adds a new todo', () => {
cy.get('[data-testid="todo-input"]').type('Buy groceries');
cy.get('[data-testid="add-button"]').click();
cy.get('[data-testid="todo-list"]').should('contain', 'Buy groceries');
});
it('completes a todo', () => {
// Add a todo first
cy.get('[data-testid="todo-input"]').type('Buy groceries');
cy.get('[data-testid="add-button"]').click();
// Mark as complete
cy.get('[data-testid="todo-item"]').first().click();
cy.get('[data-testid="todo-item"]').first().should('have.class', 'completed');
});
});
Component Storybook
Storybook helps document and visually test components:
// Button.stories.jsx
import Button from './Button';
export default {
title: 'Components/Button',
component: Button,
argTypes: {
variant: {
control: { type: 'select', options: ['primary', 'secondary', 'danger'] }
}
}
};
const Template = (args) => <Button {...args} />;
export const Primary = Template.bind({});
Primary.args = {
variant: 'primary',
children: 'Primary Button'
};
export const Secondary = Template.bind({});
Secondary.args = {
variant: 'secondary',
children: 'Secondary Button'
};
Deployment Strategies
Deploying React applications efficiently requires a thoughtful strategy.
Static Site Deployment
For standard SPAs, static deployment is common:
- Build Process:
# Create optimized production build
npm run build
-
Popular Hosting Options:
- Vercel
- Netlify
- GitHub Pages
- AWS S3 + CloudFront
Automating Deployment with GitHub Actions:
name: Deploy React App
on:
push:
branches: [ main ]
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Build
run: npm run build
- name: Deploy to Netlify
uses: netlify/actions/cli@master
with:
args: deploy --prod
env:
NETLIFY_AUTH_TOKEN: ${{ secrets.NETLIFY_AUTH_TOKEN }}
NETLIFY_SITE_ID: ${{ secrets.NETLIFY_SITE_ID }}
Server-Side Rendering Deployment
For Next.js and similar frameworks:
- Build and Start:
npm run build
npm run start
- Container-Based Deployment:
FROM node:18-alpine
WORKDIR /app
COPY package*.json ./
RUN npm ci
COPY . .
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
-
Serverless Deployment:
- Vercel (optimized for Next.js)
- AWS Amplify
- Netlify with server functions
CI/CD Best Practices
- Automated Testing: Run tests before deployment
- Environment Variables: Securely manage environment-specific config
- Progressive Rollouts: Use canary deployments to test changes
- Monitoring: Implement application monitoring post-deployment
The Evolving Front-End Ecosystem
The Perceived Decline of React
While React remains extremely popular, several factors have contributed to a shift in the front-end ecosystem:
- Bundle Size Concerns: React's bundle size can be significant compared to newer alternatives
- Complexity Overhead: The React ecosystem has grown increasingly complex
- Performance Challenges: React's virtual DOM approach has performance limitations
- Meta-framework Dominance: Many React applications now use Next.js or similar, rather than "pure" React
Rising Alternative Frameworks
Several frameworks have emerged as strong alternatives:
Svelte: Compile-Time Reactivity
Svelte shifts the work from runtime to compile time, resulting in smaller bundles and better performance:
let count = 0;
function increment() {
count += 1;
}
Vue 3: Progressive Framework
Vue offers similar component-based architecture with a gentler learning curve:
<template>
template>
<script setup>
import { ref } from 'vue';
const count = ref(0);
function increment() {
count.value++;
}
script>
Solid.js: Reactive Primitives
Solid.js offers React-like JSX with fine-grained reactivity:
import { createSignal } from 'solid-js';
function Counter() {
const [count, setCount] = createSignal(0);
return (
<button onClick={() => setCount(count() + 1)}>
Clicks: {count()}
button>
);
}
Qwik: Resumable Applications
Qwik aims to solve the JavaScript payload problem with resumability:
import { component$, useSignal } from '@builder.io/qwik';
export const Counter = component$(() => {
const count = useSignal(0);
return (
<button onClick$={() => count.value++}>
Clicks: {count.value}
button>
);
});
The Future of Front-End Development
The front-end ecosystem continues to evolve with several emerging trends:
- Islands Architecture: Selective hydration of interactive components
- Resumable Applications: Frameworks that can pause and resume execution
- Zero-Bundle JavaScript: Server-first approaches that minimize client JS
- Streaming Server Rendering: Progressive rendering of content
- Edge Computing: Moving rendering closer to users
Where React Fits Today
Despite challenges, React remains relevant for several reasons:
- Massive Ecosystem: Thousands of libraries, tools, and resources
- Industry Adoption: Widespread use in enterprise applications
- Developer Familiarity: Large pool of experienced React developers
- Meta-framework Innovation: Next.js and similar frameworks address many core limitations
Conclusion: Building Production-Ready React Applications
Building production-ready React applications in today's landscape requires:
- Framework Awareness: Understanding React's strengths and limitations versus alternatives
- Focused State Management: Choosing the right solution for your specific needs
- Performance Optimization: Implementing best practices from the start
- Comprehensive Testing: Covering unit, integration, and E2E scenarios
- Efficient Deployment: Leveraging modern CI/CD and hosting platforms
While the front-end ecosystem continues to evolve, the core principles of component composition, state management, and performance optimization remain relevant regardless of framework choice. The best developers understand the tradeoffs between different approaches and choose the right tool for each specific project.
Whether you continue with React or explore alternatives like Svelte, Vue, or Solid.js, focusing on user experience, performance, and maintainability will serve you well in the rapidly changing world of front-end development.