Java Multithreading Explained: Concepts, Examples, and Best Practices
Multithreading is an essential concept in Java that allows multiple threads to run concurrently, making applications more efficient and responsive. In this blog, we will explore Understanding Multithreading in Java, its benefits, real-world applications, and best practices to follow when developing multithreaded programs. What is Multithreading in Java? Multithreading is the ability of a Java program to execute multiple threads simultaneously. A thread is a lightweight sub-process that shares the same memory space with other threads within a process. The Java Virtual Machine (JVM) supports multithreading, making Java a powerful language for developing applications that require parallel execution. Threads help in improving the performance of an application by utilizing the available CPU resources efficiently. Instead of executing tasks sequentially, multithreading allows tasks to be divided into smaller units and executed concurrently, leading to faster execution. Benefits of Multithreading Improved Performance: Multithreading helps in utilizing CPU resources effectively by executing tasks in parallel, reducing overall execution time. Better Responsiveness: Applications, especially GUI-based applications, remain responsive even when performing complex operations. Efficient Resource Utilization: Multiple threads share the same memory and system resources, reducing overhead compared to creating multiple processes. Parallel Processing: Multithreading allows multiple operations to be executed simultaneously, improving efficiency in computation-intensive applications. Asynchronous Execution: Background tasks, such as file operations or network communications, can be performed without blocking the main application thread. Real-World Applications of Multithreading 1. Gaming Applications In modern gaming applications, multiple threads handle different tasks such as rendering graphics, processing player inputs, and executing AI logic simultaneously. This ensures smooth gameplay without lag. 2. Web Servers and Networking Web servers handle thousands of client requests concurrently using multithreading. Each request is processed in a separate thread, improving server efficiency and responsiveness. 3. Financial Applications Stock market applications and banking systems use multithreading to process real-time transactions and update data dynamically without slowing down the user experience. 4. Data Processing and Analysis Big data applications process vast amounts of data efficiently using multithreading. It allows parallel execution of computations, reducing the time needed for data analysis. Challenges in Multithreading Despite its benefits, multithreading also presents challenges, including: Thread Synchronization Issues: When multiple threads access shared resources, race conditions may occur, leading to data inconsistency. Deadlocks: If two or more threads wait indefinitely for each other to release a resource, a deadlock occurs, causing the application to hang. Increased Complexity: Writing and debugging multithreaded programs is more complex than single-threaded applications. Context Switching Overhead: Frequent switching between threads can lead to performance overhead, reducing efficiency. Best Practices for Java Multithreading To maximize the benefits of multithreading while avoiding potential pitfalls, follow these best practices: 1. Use Thread-Safe Data Structures Utilize Java’s built-in thread-safe collections such as ConcurrentHashMap and CopyOnWriteArrayList to prevent concurrent modification issues. 2. Minimize Shared Resources Where possible, reduce the number of shared variables or objects to minimize synchronization overhead and potential conflicts. 3. Implement Synchronization Wisely Use synchronization mechanisms like synchronized blocks, locks, and volatile variables to prevent race conditions while ensuring that they do not introduce unnecessary performance bottlenecks. 4. Use Executor Framework Instead of Creating Threads Manually Java’s ExecutorService provides a higher-level API for managing threads efficiently. It helps in pooling and reusing threads, reducing system overhead. 5. Avoid Deadlocks by Using Lock Ordering If multiple locks are required, always acquire them in a consistent order to prevent circular dependencies leading to deadlocks. 6. Use Atomic Variables for Simple Synchronization Java provides AtomicInteger, AtomicLong, and other atomic classes that support lock-free thread-safe operations, improving performance. 7. Prefer Callable Over Runnable When a thread needs to return a result, prefer using the Callable interface instead of Runnable, as it supports returning values and handling exceptions. 8. Monitor and Optimize Thread Performance Use Java’s ThreadDump, Visua
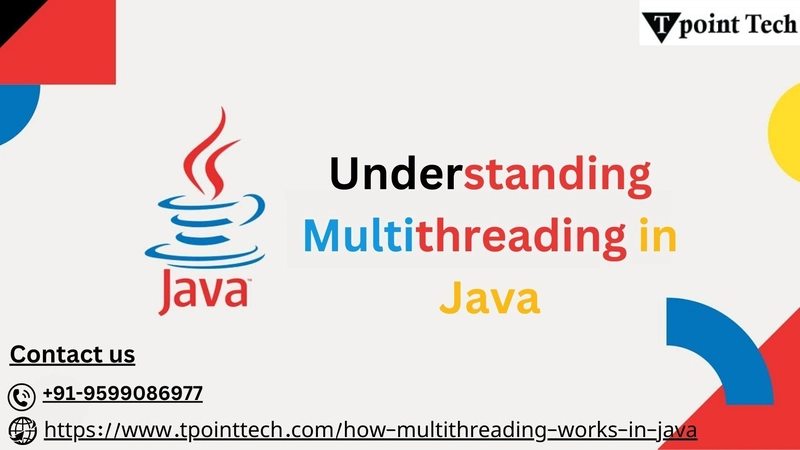
Multithreading is an essential concept in Java that allows multiple threads to run concurrently, making applications more efficient and responsive. In this blog, we will explore Understanding Multithreading in Java, its benefits, real-world applications, and best practices to follow when developing multithreaded programs.
What is Multithreading in Java?
Multithreading is the ability of a Java program to execute multiple threads simultaneously. A thread is a lightweight sub-process that shares the same memory space with other threads within a process. The Java Virtual Machine (JVM) supports multithreading, making Java a powerful language for developing applications that require parallel execution.
Threads help in improving the performance of an application by utilizing the available CPU resources efficiently. Instead of executing tasks sequentially, multithreading allows tasks to be divided into smaller units and executed concurrently, leading to faster execution.
Benefits of Multithreading
Improved Performance: Multithreading helps in utilizing CPU resources effectively by executing tasks in parallel, reducing overall execution time.
Better Responsiveness: Applications, especially GUI-based applications, remain responsive even when performing complex operations.
Efficient Resource Utilization: Multiple threads share the same memory and system resources, reducing overhead compared to creating multiple processes.
Parallel Processing: Multithreading allows multiple operations to be executed simultaneously, improving efficiency in computation-intensive applications.
Asynchronous Execution: Background tasks, such as file operations or network communications, can be performed without blocking the main application thread.
Real-World Applications of Multithreading
1. Gaming Applications
In modern gaming applications, multiple threads handle different tasks such as rendering graphics, processing player inputs, and executing AI logic simultaneously. This ensures smooth gameplay without lag.
2. Web Servers and Networking
Web servers handle thousands of client requests concurrently using multithreading. Each request is processed in a separate thread, improving server efficiency and responsiveness.
3. Financial Applications
Stock market applications and banking systems use multithreading to process real-time transactions and update data dynamically without slowing down the user experience.
4. Data Processing and Analysis
Big data applications process vast amounts of data efficiently using multithreading. It allows parallel execution of computations, reducing the time needed for data analysis.
Challenges in Multithreading
Despite its benefits, multithreading also presents challenges, including:
- Thread Synchronization Issues: When multiple threads access shared resources, race conditions may occur, leading to data inconsistency.
- Deadlocks: If two or more threads wait indefinitely for each other to release a resource, a deadlock occurs, causing the application to hang.
- Increased Complexity: Writing and debugging multithreaded programs is more complex than single-threaded applications.
- Context Switching Overhead: Frequent switching between threads can lead to performance overhead, reducing efficiency.
Best Practices for Java Multithreading
To maximize the benefits of multithreading while avoiding potential pitfalls, follow these best practices:
1. Use Thread-Safe Data Structures
Utilize Java’s built-in thread-safe collections such as ConcurrentHashMap and CopyOnWriteArrayList to prevent concurrent modification issues.
2. Minimize Shared Resources
Where possible, reduce the number of shared variables or objects to minimize synchronization overhead and potential conflicts.
3. Implement Synchronization Wisely
Use synchronization mechanisms like synchronized blocks, locks, and volatile variables to prevent race conditions while ensuring that they do not introduce unnecessary performance bottlenecks.
4. Use Executor Framework Instead of Creating Threads Manually
Java’s ExecutorService provides a higher-level API for managing threads efficiently. It helps in pooling and reusing threads, reducing system overhead.
5. Avoid Deadlocks by Using Lock Ordering
If multiple locks are required, always acquire them in a consistent order to prevent circular dependencies leading to deadlocks.
6. Use Atomic Variables for Simple Synchronization
Java provides AtomicInteger, AtomicLong, and other atomic classes that support lock-free thread-safe operations, improving performance.
7. Prefer Callable Over Runnable
When a thread needs to return a result, prefer using the Callable interface instead of Runnable, as it supports returning values and handling exceptions.
8. Monitor and Optimize Thread Performance
Use Java’s ThreadDump, VisualVM, and other profiling tools to identify thread-related bottlenecks and optimize performance.
Conclusion
Understanding Multithreading in Java is crucial for building high-performance and scalable applications. It enables parallel processing, improves application responsiveness, and enhances resource utilization. However, implementing multithreading requires careful consideration of synchronization, resource sharing, and best practices to avoid common pitfalls such as race conditions and deadlocks.
By following the best practices outlined in this Java tutorial, developers can efficiently harness the power of multithreading to create robust and scalable applications. Whether developing gaming applications, web servers, or financial systems, mastering multithreading is an invaluable skill for every Java developer.